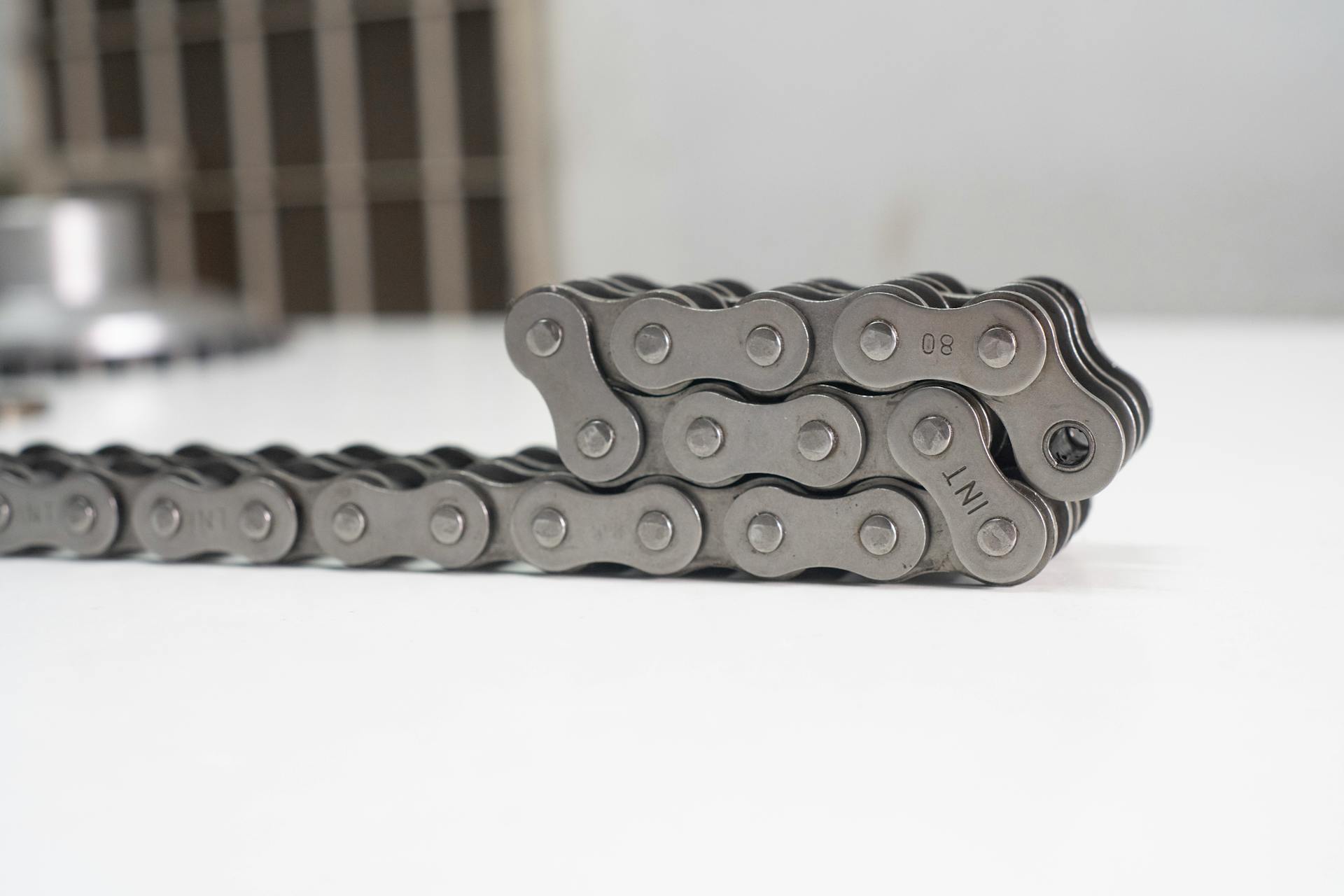
Data structures are the building blocks of computer science, and understanding them is crucial for any aspiring programmer.
Arrays are a fundamental data structure that store a collection of elements of the same data type in contiguous memory locations. They are used extensively in various applications, including sorting and searching algorithms.
A hash table is a data structure that stores key-value pairs in an array using a hash function to map keys to indices. This allows for efficient lookup, insertion, and deletion of elements.
In Compsci 61b, we learned that a stack is a Last-In-First-Out (LIFO) data structure that follows the principle of last element added is the first one to be removed. This makes it ideal for applications like parsing and evaluating postfix expressions.
Linked lists are a dynamic data structure where each element points to the next element, allowing for efficient insertion and deletion of elements at any position. They are commonly used in scenarios where data is frequently added or removed.
A fresh viewpoint: Elements in Statistical Learning
Data Structure Fundamentals
Data Structure Fundamentals are the building blocks of programming, and understanding them is crucial for writing efficient code.
Arrays are a type of data structure that store multiple values of the same type in a single variable.
Arrays are used to store a collection of elements of the same data type, such as integers, floats, or strings.
Linked Lists are a type of data structure where elements are stored as separate objects, each pointing to the next element.
Stacks and Queues are types of data structures that follow the Last-In-First-Out (LIFO) and First-In-First-Out (FIFO) principles, respectively.
Trees are a type of data structure that organize elements in a hierarchical structure, with each node having a value and zero or more child nodes.
Graphs are a type of data structure that consist of nodes or vertices connected by edges, allowing for efficient traversal and manipulation of data.
Understanding CS
CS 61B is a pivotal course in computer science education, laying the foundation for advanced studies and career success. It's like constructing your programming toolkit, filled with essential instruments you'll consistently utilize throughout your computer science journey.
Check this out: Books about Computer Science
The course focuses on data structures and algorithms, emphasizing practical application using Java. You'll learn how to choose the most effective data structures and algorithms for different situations, optimizing program performance. This knowledge is highly transferable, making it valuable beyond individual programming languages.
CS 61B covers three main areas: data structures, algorithms, and software engineering. Data structures include arrays, linked lists, trees, and hash tables, while algorithms cover sorting and searching. Software engineering principles and practices focus on designing, developing, and maintaining high-quality software.
Here are some key data structures you'll learn about in CS 61B:
- Arrays
- Linked Lists (singly-linked, doubly-linked, and circular)
- Binary Trees (binary search trees and expression trees)
- Hash Tables
- Graph Structures
Historical Live QA
Historical Live QA has shown us that IntLists can be tricky to work with. In order to use an IntList correctly, the programmer must understand and utilize recursion even for simple list related tasks.
IntLists are especially hard to use because they require a good grasp of recursion. This can be a challenge for beginners who are still learning the basics of programming.
Programmers have struggled with IntLists for a long time, and it's not uncommon to see them cause frustration and confusion.
What Is CS About?
CS 61B is a course that delves into the core of computer science, exploring how we structure and manipulate data to solve complex problems. It assumes a basic understanding of programming, building upon concepts introduced in CS 61A. The course uses Java as the primary language, but the focus is on applying Java to understand broader concepts of data structures and algorithms.
Students in CS 61B will gain facility with Java programming, become familiar with fundamental data structures and algorithms, and learn techniques for constructing programs of moderate size using Java. They'll learn how to choose the most effective data structures and algorithms for different situations, optimizing program performance.
The course covers three main areas: data structures, algorithms, and software engineering. Data structures include arrays, linked lists, trees, and hash tables. Algorithms involve step-by-step procedures for solving problems and manipulating data, such as sorting and searching algorithms.
Here's a breakdown of the course's focus:
The course aims to equip students with the skills to recognize when data structures and algorithms are applicable to a problem, and to evaluate their relative advantages and disadvantages. By the end of the course, students will understand the distinction between a specification or interface and an implementation, and be able to use a specification expressed as a set of procedure headers with comments.
Prerequisites and Preparation
To tackle CS 61B, you'll need to have a solid foundation in programming concepts. CS 61A or ENGIN 7 is a required prerequisite, introducing fundamental programming concepts like logic, recursion, object-oriented programming, and basic data structures.
Practical programming experience is highly recommended, not just for CS 61B, but for any programming course. Any coding experience outside of coursework, such as personal projects, strengthens your foundational skills.
To give you a better idea of what's required, here's a breakdown of the prerequisites and their importance:
Having prior coding experience, even through personal projects, can greatly benefit you in CS 61B. This hands-on experience will make the transition smoother and facilitate a deeper understanding of the material, especially when working with Java.
Mastering Algorithms: The Computation Recipes
Algorithms are the backbone of computer science, and understanding them is crucial for solving computational problems. In CS 61B, we dive into the world of algorithms, where we learn to manipulate data to provide solutions.
Take a look at this: Grokking Algorithms 2nd Edition Pdf
Searching is a fundamental concept in algorithms, where we locate specific information within a dataset. This is essential in real-world applications, such as finding a specific file on your computer or searching for a particular product online.
Sorting is another vital algorithm that arranges data in a specific order. This is useful for tasks like organizing a list of names or numbers in ascending or descending order.
Graph Traversal is a more complex algorithm that navigates and explores graph structures. This is essential for tasks like finding the shortest path between two cities or identifying the most efficient route for a delivery truck.
Here are the three key algorithms we cover in CS 61B:
- Searching: Locating specific information within a dataset.
- Sorting: Arranging data in a specific order.
- Graph Traversal: Navigating and exploring graph structures.
Frequently Asked Questions
What language is cs61b taught in?
CS61B is taught in Java, where students learn programming fundamentals, data structures, and algorithms.
What are the 4 data structures?
There are four main data structure types: linear, tree, hash, and graph. These fundamental structures form the building blocks of computer programming and data organization.
What are the 8 data structures?
Our data structures include arrays, linked lists, stacks, queues, hash tables, trees, heaps, and graphs, each serving a unique purpose in organizing and manipulating data. Understanding these fundamental data structures is essential for building efficient and effective software solutions.
What is cs 61B?
CS 61B is a course that teaches Java programming, data structures, and algorithms to help students build moderate-sized programs. It's a comprehensive course that covers the fundamentals of computer science.
Featured Images: pexels.com