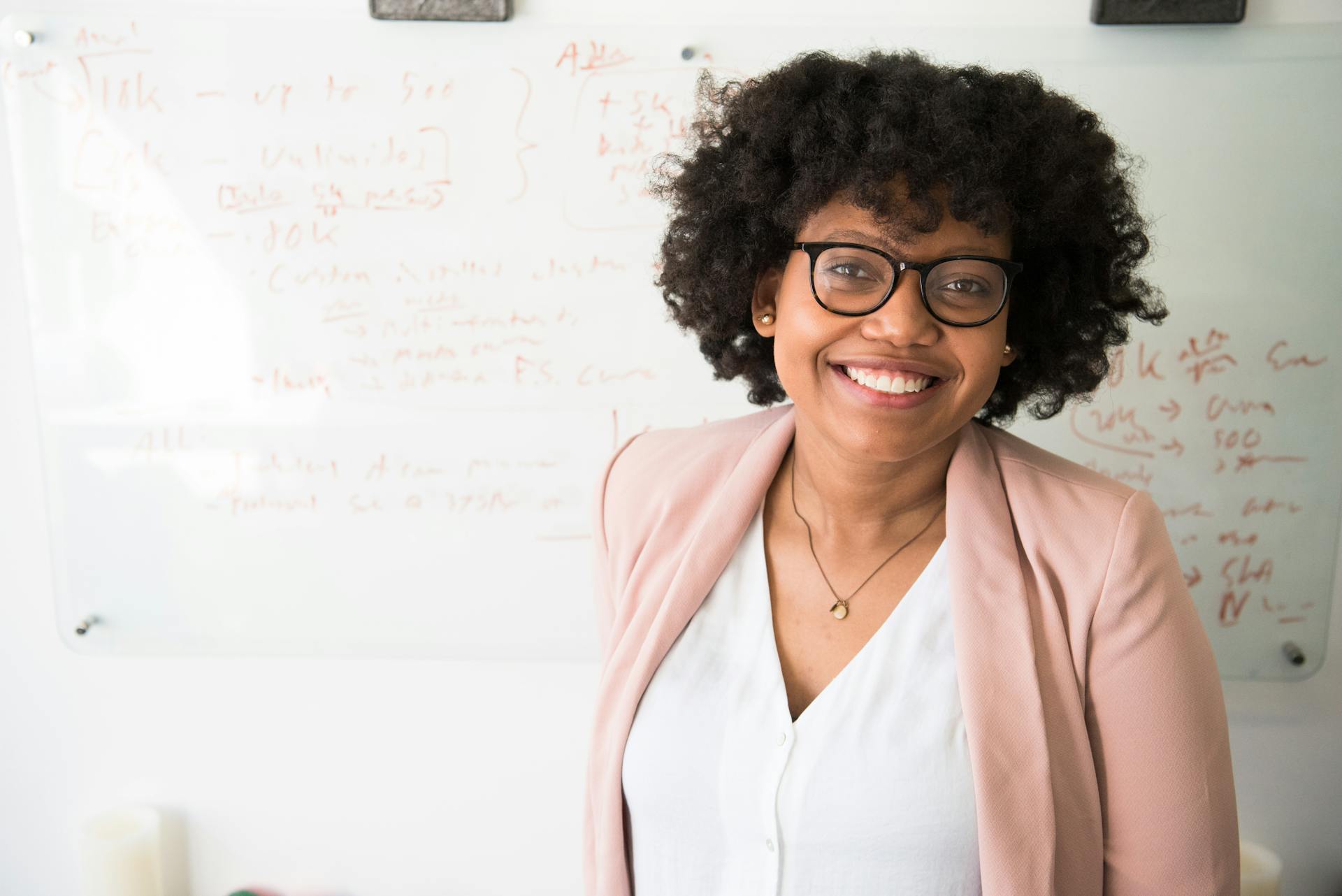
Grokking the coding interview is a skill that can be learned with practice and patience. It's a process of breaking down complex problems into manageable parts, and identifying the essential patterns that underlie them.
One key pattern to recognize is the "Two Pointers" technique, which is used to solve problems that involve arrays or linked lists. This technique is especially useful when dealing with problems that require comparing elements or finding a specific element in a list.
As you practice solving problems, you'll find that certain patterns start to emerge. For example, the "Sliding Window" technique is often used to solve problems that involve finding a subarray or substring within a larger array or string.
By recognizing these essential patterns, you'll be able to approach problems with a sense of familiarity and confidence, and tackle even the most challenging coding interview questions with ease.
16 Essential Patterns Every Programmer Should Learn
Grokking the coding interview requires mastering essential patterns that can help you tackle complex problems with ease. These patterns are the building blocks of coding interviews, and understanding them can make a huge difference in your preparation.
One of the most important patterns is the Two Pointer technique, which is covered in 20 lessons. This technique is a fundamental concept that can be applied to various problems, including arrays and linked lists.
The Sliding Window pattern is another essential concept that is covered in 12 lessons. This pattern is useful for problems that require you to process a subset of data within a larger dataset.
In addition to these patterns, the course also covers the Merge Intervals pattern, which is useful for problems that involve merging overlapping intervals.
Here are 16 essential coding patterns every programmer should learn:
By mastering these 16 essential coding patterns, you'll be well-prepared to tackle a wide range of coding interview problems and land your dream job.
Algorithms
Algorithms are the heart of coding interviews, and understanding how they work is crucial to acing the process. In a typical coding interview, you'll be asked to solve problems using algorithms, so it's essential to have a solid grasp of the basics.
For more insights, see: Pdf Grokking Algorithms
The average coding interview problem has a time complexity of O(n), where n is the size of the input. This means that the algorithm's running time increases linearly with the size of the input. For example, a simple sorting algorithm like bubble sort has a time complexity of O(n^2), making it inefficient for large datasets.
To improve your algorithmic skills, focus on practicing with problems that have a time complexity of O(n) or better. This will help you develop a sense of how to optimize your solutions and reduce the time complexity of your algorithms.
Reverse Linked List
The Reverse Linked List pattern is a clever way to reverse the nodes of a linked list without using extra space. This is achieved by manipulating pointers directly, resulting in O(1) space complexity.
To accomplish this, you'll need to carefully handle the pointers to ensure the nodes are correctly reversed. This pattern requires initial practice to get a feel for how the pointers need to be manipulated.
A key benefit of this pattern is its memory efficiency, making it a great choice for scenarios where memory is limited. However, it can be a bit tricky to get right, so be sure to take your time and work through some examples.
Here are some examples of problems that involve the Reverse Linked List pattern:
- Reverse linked list (Educative.io)
- Problem Challenge 1: Tree Diameter (Leetcode)
Sliding Window
The Sliding Window pattern is a fundamental technique used to solve problems involving arrays and strings. It's a powerful tool for finding the maximum or minimum sum of a subarray, or identifying the smallest subarray with a given sum.
The Sliding Window pattern is often used to solve problems that involve finding a specific pattern within a larger array or string. For example, finding the longest substring with K distinct characters is a classic problem that can be solved using this pattern.
Here are some examples of problems that can be solved using the Sliding Window pattern:
- Maximum Sum Subarray of Size K (easy)
- Smallest Subarray with a given sum (easy)
- Longest Substring with K Distinct Characters (medium)
- Fruits into Baskets (medium)
The Sliding Window pattern is particularly useful when dealing with arrays and strings, as it allows us to efficiently traverse the data and find the desired solution. By using this pattern, we can avoid unnecessary iterations and improve the performance of our algorithms.
Some common applications of the Sliding Window pattern include finding the maximum or minimum sum of a subarray, identifying the smallest subarray with a given sum, and finding the longest substring with K distinct characters.
Modified Binary Search
Modified Binary Search is a powerful pattern that efficiently handles searches in sorted arrays. It adapts classic binary search to prevent integer overflow and optimize searching boundaries.
This pattern is particularly useful for searching large datasets, as seen in problems like Search in a Sorted Infinite Array and Search a 2D Matrix. By using modified binary search, you can reduce the time complexity of these searches from O(n) to O(log n).
One key advantage of modified binary search is its ability to handle edge cases, such as searching for the ceiling or floor of a number, as seen in Ceiling of a Number and Minimum Difference Element. This is achieved by using the floor and ceiling functions to determine the optimal search boundaries.
Here are some key applications of modified binary search:
- Search in a Sorted Infinite Array (Leetcode)
- Search a 2D Matrix (Leetcode)
- Ceiling of a Number (Geeksforgeeks)
- Minimum Difference Element (Geeksforgeeks)
By mastering modified binary search, you'll be able to tackle a wide range of search problems efficiently and effectively.
Two Pointers
The two pointers technique is a game-changer for optimizing algorithms, especially when working with ordered data structures. It eliminates the need for nested loops and reduces time complexity from O(n²) to O(n).
This method is particularly effective for finding elements that meet specific conditions in arrays or linked lists. By using two pointers, you can iterate through the data structure efficiently.
The two pointers technique is often used in combination with other algorithms, such as the reverse linked list pattern, which requires careful pointer handling and initial practice. This combination can lead to significant memory efficiency and improved performance.
Here's a list of some common use cases for the two pointers technique:
By mastering the two pointers technique, you can tackle a wide range of problems and improve your algorithmic skills. With practice, you'll become more comfortable using this technique to solve complex problems efficiently.
Stack
A stack is a fundamental data structure that can be used to implement a variety of algorithms. It's a Last-In-First-Out (LIFO) data structure, meaning the last item added to the stack is the first one to be removed.
Consider reading: Code First Girls
You can use a stack to reverse a string, which is a common problem in programming. The idea is to push each character of the string onto the stack, and then pop each character off the stack to build the reversed string.
One of the most well-known applications of a stack is in parsing expressions, such as evaluating the validity of balanced parentheses. This is a classic problem that can be solved using a stack to keep track of the opening parentheses.
Here are some common operations you can perform on a stack:
- Push: Add an item to the top of the stack
- Pop: Remove the item from the top of the stack
- Peek: Look at the item at the top of the stack without removing it
A stack can also be used to convert decimal numbers to binary. The idea is to repeatedly divide the decimal number by 2 and push the remainder onto the stack, and then pop each remainder off the stack to build the binary representation.
The following table summarizes some of the applications of a stack:
A stack can also be used to find the next greater element in a sequence of numbers. This problem is often referred to as the "Next Greater Element" problem.
Languages Used to Solve Questions
Java, Python, C++, and JavaScript are the languages used to solve the coding questions in this course.
Each problem presented has been solved using these four languages, totaling 325 lessons and 353 coding playgrounds.
These languages are commonly used in coding interviews and are essential for any aspiring programmer to master.
The course's comprehensive approach to teaching these languages makes it an excellent resource for those looking to improve their coding skills.
Frequently Asked Questions
Is Grokking the System Design Interview enough?
Grokking the System Design Interview is a valuable resource, but it's just one piece of the puzzle - a comprehensive study plan should include additional learning and hands-on experience. Supplementing the course with real-world learning is essential for true mastery.
How long does it take to finish grokking the coding interview?
Finish the course in 2-4 weeks with intense study (3-4 hours/day) and a strong background in data structures and algorithms
Is grokking algorithm good for LeetCode?
Yes, Grokking Algorithm is a highly recommended resource for LeetCode, as it's often paired with Blind 75 to help you tackle medium-level problems and prepare for L3-L4 FAANG interviews.
How many problems are in Grokking the Coding Interview?
Grokking the Coding Interview contains 99 essential coding interview challenges, carefully curated with hiring managers at top tech companies. Master these challenges to boost your coding interview prep and land your dream job.
Sources
- https://www.educative.io/courses/grokking-coding-interview
- https://github.com/dipjul/Grokking-the-Coding-Interview-Patterns-for-Coding-Questions
- https://interviewnoodle.com/review-of-grokking-the-coding-interview-course-6b389601705f
- https://www.codinginterview.com/coding-interview-patterns
- https://medium.com/javarevisited/is-grokking-the-coding-interview-pattern-in-java-on-educative-worth-it-8bb3ad4e1daa
Featured Images: pexels.com