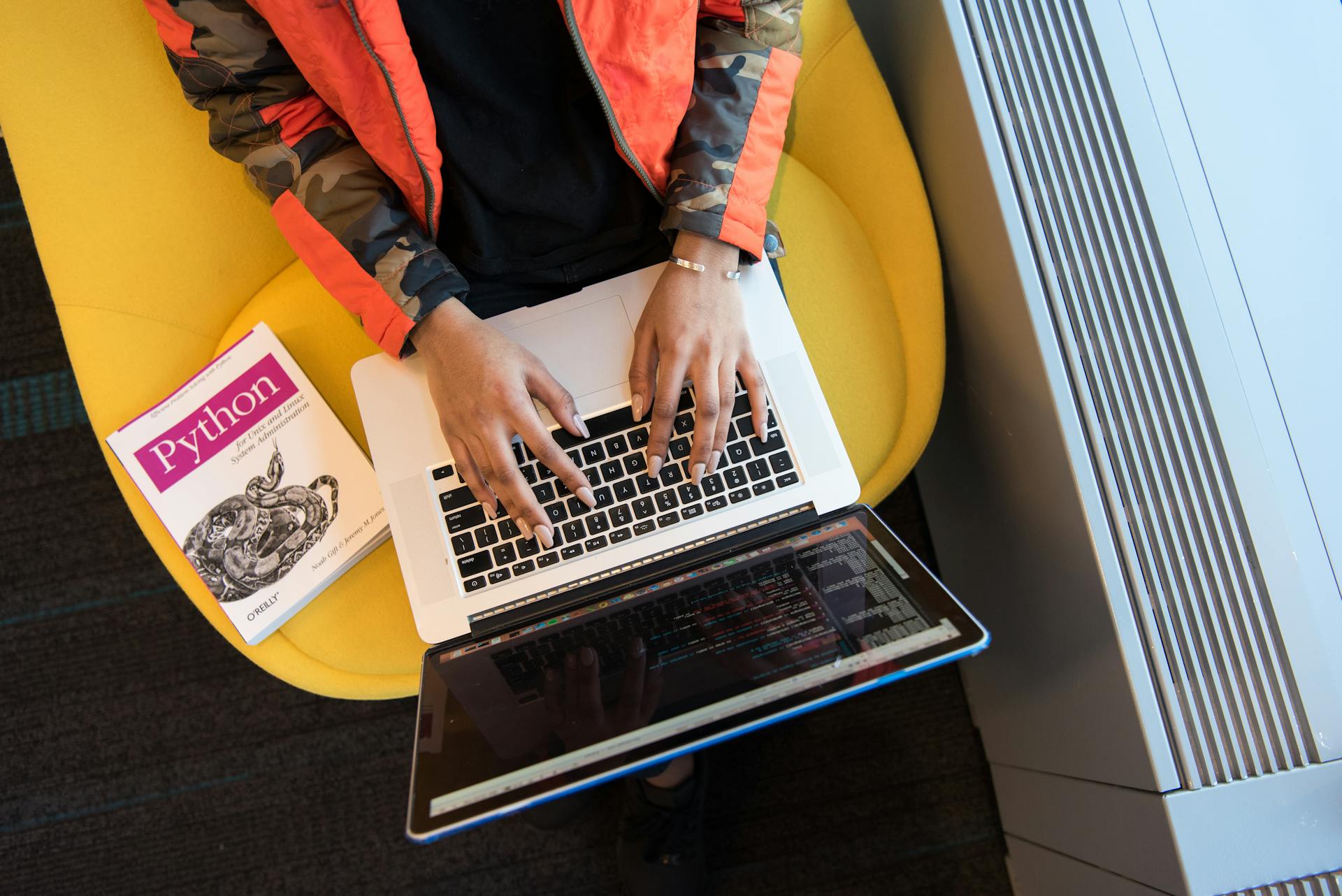
Data preprocessing is a crucial step in any data analysis project, and Python is an excellent language for handling it. You can load your data into a Pandas DataFrame using the `read_csv` function.
First, you need to import the necessary libraries, such as Pandas and NumPy. Pandas is used for data manipulation and analysis, while NumPy is used for numerical computations. In the article, we'll be using Pandas to handle our data.
Next, we'll explore how to handle missing values in your data. The `isnull` function can be used to identify missing values, and the `dropna` function can be used to remove them. For example, if you have a DataFrame with missing values in the 'Age' column, you can drop those rows using `df.dropna(subset=['Age'])`.
To get a better understanding of your data, you can use the `info` function to print a concise summary of the DataFrame, including the index dtype and column dtypes. This is especially useful when dealing with large datasets.
Tools and Libraries
Python is a programming language that supports countless open source libraries for data preprocessing. These libraries can compute complex operations with a single line of code.
The scikit-learn library package is particularly useful for imputing missing values, and can be used with just one line of code. Autumunge is another great tool, built as a python library platform, that prepares tabular data for direct application of machine learning algorithms.
R is a framework mostly used for research/academic purposes, and has multiple packages that support data preprocessing steps considerably. Data preprocessing with R is made easier with its various packages.
Weka and RapidMiner are both software that support data mining and data preprocessing, with in-built tools and machine learning models for intelligent mining.
Data Preparation
Data Preparation is a crucial step in any data analysis project. You want to make sure your data is clean and ready for use.
To start, checking for missing values is essential. According to the data, you can count the number of missing values in each column using a simple function. This will give you an idea of where the problems are.
Missing values can be a major issue, but identifying them is the first step to fixing them. By counting the number of missing values in each column, you can determine which columns need the most attention.
Step 2: Load
Loading a dataset is the first step in any data preparation process. This involves importing the data from a file into your Python environment.
You can use the pandas library to load a CSV file, as shown in the example: `df = pd.read_csv('Geeksforgeeks/Data/diabetes.csv')`. This line of code reads the data from the specified file and stores it in a DataFrame object called `df`.
The `print(df.head())` statement is then used to display the first few rows of the DataFrame, giving you an idea of what the data looks like.
Here's a quick rundown of the data loading process:
The box plots in the example code provide a visual representation of the data distribution for each column. This can help you identify any issues with the data, such as outliers or skewness.
Checking the Info
Data preparation is all about understanding what you're working with. To do that, you need to know the data types of each column in your DataFrame.
This command displays the data types of each column: `Checking the data types of the columns`. It's a quick way to see if everything is in the right format.
If you're new to Pandas, you might be wondering what kind of data structures it offers. Pandas provides two primary data structures: Series and DataFrame.
Here are the main features of Pandas:
- Data cleaning and transformation
- Data aggregation
- Data merging and joining
- Time series analysis
To change the data type of a column, you can use this code: `Changing the data type of a column`. This is useful if you need to convert a column to a different data type, like float.
Checking the Shape
You can get the number of rows and columns in your DataFrame by checking its shape. To get the number of rows and columns in your DataFrame, use the shape attribute.
The shape attribute returns a tuple with two values: the number of rows and the number of columns. For example, if your DataFrame has 5 rows and 3 columns, the shape attribute will return (5, 3).
Renaming
Renaming is an essential step in data preparation, allowing you to give your columns meaningful names that accurately reflect their content. This makes it easier to understand and work with your data.
To change the name of a column, you can simply rename it.
Data Cleaning
Data cleaning is a crucial step in data preprocessing, and it involves several techniques to ensure the quality and accuracy of our data. Removing duplicates is one of the first steps in data cleaning, which helps to eliminate redundant data and reduce the risk of errors.
Handling missing data is another important aspect of data cleaning, as it can significantly impact the accuracy of our models. To count the number of missing values in each column, we can use a simple function or method.
Here are some common techniques used to handle missing data:
- Removing duplicates
- Handling missing data
- Handling outliers
- Standardizing or normalizing data
- Encoding categorical data
- Feature selection
- Handling data errors
Handling outliers is also crucial in data cleaning, as they can skew our results and affect the performance of our models. One common method for handling outliers is to drop them, but this should be done with caution as it can lead to biased results.
In Example 3, we see how to drop outliers using the interquartile range (IQR) method, which is a common technique used to identify and remove outliers. The IQR method calculates the lower and upper bounds of the data, and any data points that fall outside of these bounds are considered outliers.
Checking the Number
You need to know how many missing values are lurking in your data, and it's easy to do with a simple command. Checking the number of missing values in each column is a crucial step in the data cleaning process.
The command to count the number of missing values in each column is straightforward: it's the same one used to check the data types of the columns.
This code displays the data types of each column in the DataFrame, and it's also used to check the number of missing values in each column.
The number of missing values in each column can vary greatly, and it's essential to identify and address these gaps in your data.
Dropping
Dropping columns is a crucial step in data cleaning, and it's actually quite straightforward. You can remove specific columns from a DataFrame with just a few lines of code.
In some cases, you might have unnecessary columns that are taking up space and slowing down your analysis. For example, if you're working with a large dataset, you might have a column that's just a copy of another column, and you can drop it to save space.
To drop specific columns, you can use the drop() function. This function takes in the column names as arguments and removes them from the DataFrame. For instance, if you have a DataFrame with columns A, B, and C, you can drop column B like this.
Dropping columns can also help you avoid confusion and errors in your analysis. If you have duplicate or redundant columns, dropping them can help you work with cleaner and more accurate data.
Imputation
Imputation is a crucial step in data cleaning. It involves replacing missing values in our data with substituted values.
The substituted value is commonly the mean, median, or mode of the data for that column.
Data imputation is a way to deal with missing data, which is a common issue in data cleaning. We can use the mean, median, or mode to fill in the gaps.
Here are some common methods for imputation:
- Mean imputation: Replacing missing values with the mean of the column
- Median imputation: Replacing missing values with the median of the column
- Mode imputation: Replacing missing values with the most frequent value in the column
Imputation can be a simple yet effective way to deal with missing data, but it's essential to choose the right method for the job.
Sources
- https://neptune.ai/blog/data-preprocessing-guide
- https://www.geeksforgeeks.org/data-preprocessing-machine-learning-python/
- https://github.com/PacktPublishing/Hands-On-Data-Preprocessing-in-Python
- https://dzone.com/articles/machine-learning-with-python-data-preprocessing
- https://levelup.gitconnected.com/mastering-data-preprocessing-in-python-pandas-23-clear-examples-013df80b95a3
Featured Images: pexels.com