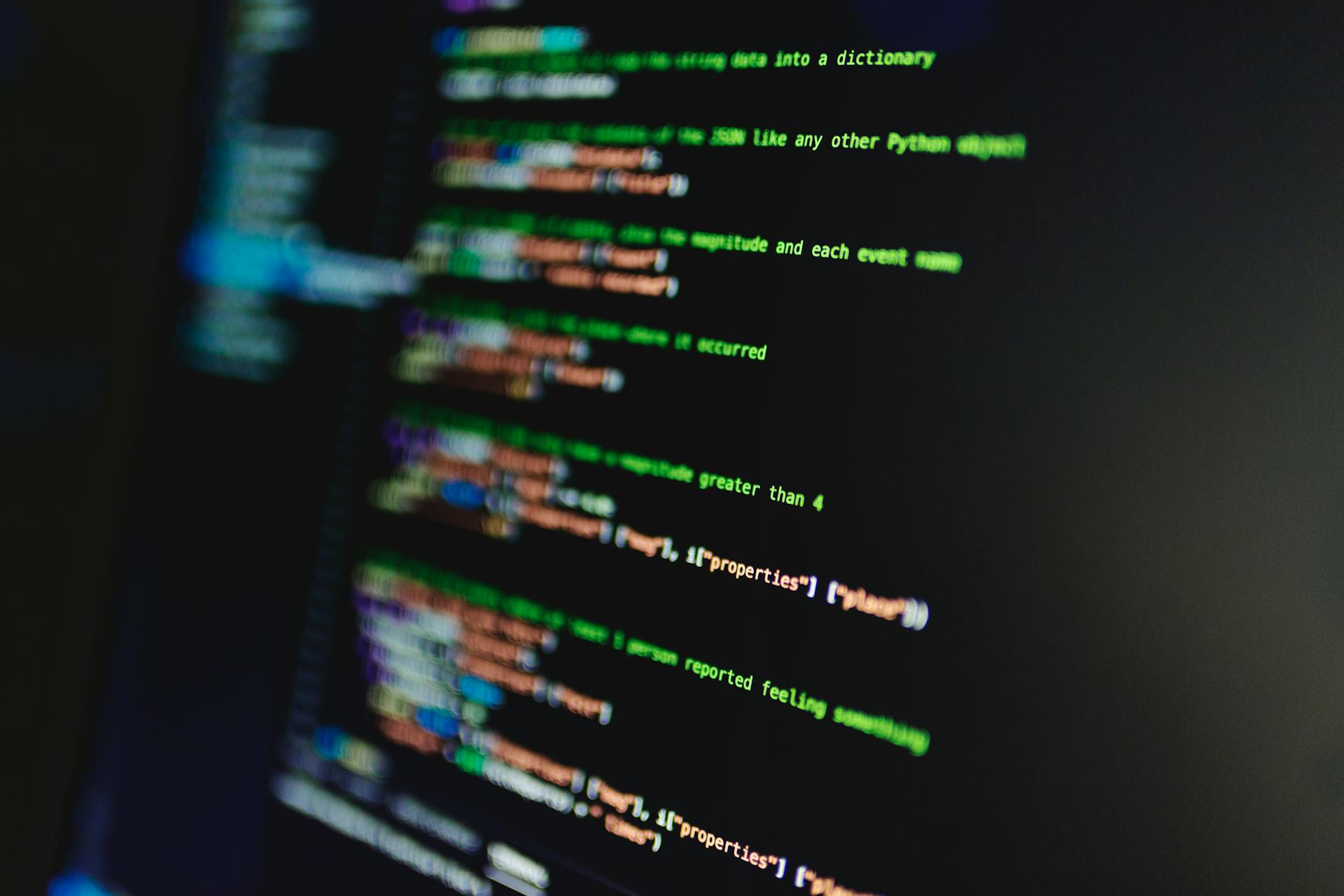
To start coding Google's Go Golang programming language from scratch, you'll need a basic understanding of programming concepts.
Go is statically typed, which means the compiler checks the types of variables at compile time, not at runtime. This results in fewer errors and more efficient code.
You can download the Go SDK from the official Go website, which includes the Go compiler and other essential tools.
The Go installation process is straightforward, and you can follow the installation instructions on the official Go website.
Take a look at this: Get Started with Programming
Getting Started
Getting Started with Go is a great place to begin your coding journey. You can set up and use Go on your computer for larger development projects.
To get started, you'll need to set up Visual Studio Code for Go. This will make your Golang development experience easy and fun by helping you with formatting, error checking, and code navigation. With Visual Studio Code setup for Go, you’re now ready to start coding. You can launch your Go files and take use of the Go extension’s robust capabilities, and experience intelligent code completion.
Related reading: Generative Ai for Code
Beginner's Guide
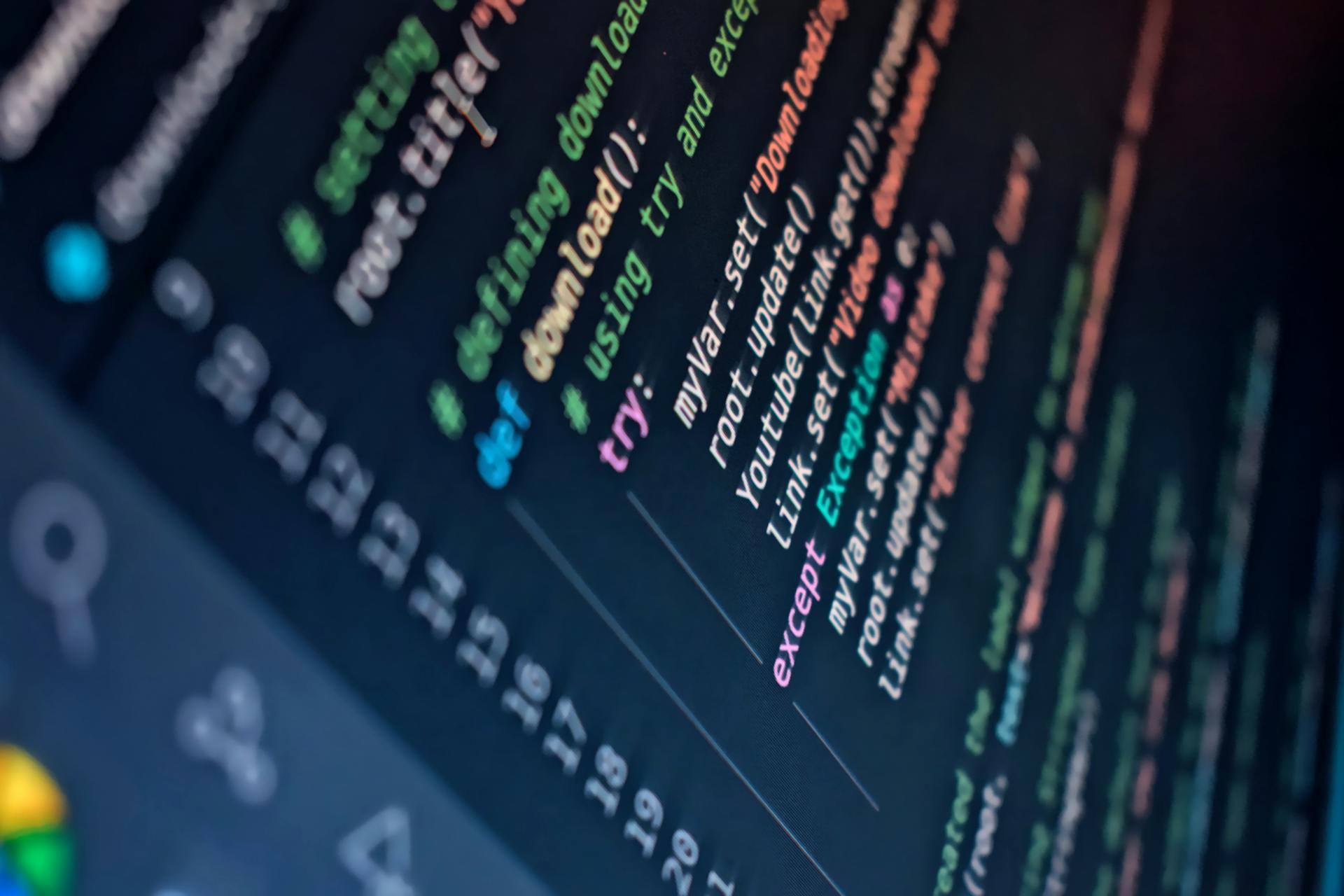
As a beginner, it's essential to start with the basics of Go. You'll need to learn about Go Variables, which are the fundamental building blocks of any programming language.
Go Variables are used to store and manipulate data in your code. You'll want to understand how to declare, assign, and use variables effectively.
Go Data Types are also crucial to grasp, as they determine the type of data a variable can hold. You'll learn about the different types, such as integers, floats, and strings.
To get started with Go, you'll need to understand Go Operators, which are used to perform operations on variables and data types. You'll learn about basic operators like arithmetic, comparison, and logical operators.
Go Functions are also a vital part of any programming language. You'll learn how to declare, define, and use functions to perform specific tasks in your code.
Here's a quick rundown of the key concepts you'll cover in this beginner's guide:
You'll also learn about Go Variable Scope, which determines the visibility and accessibility of variables in your code. This is essential to avoid confusion and errors.
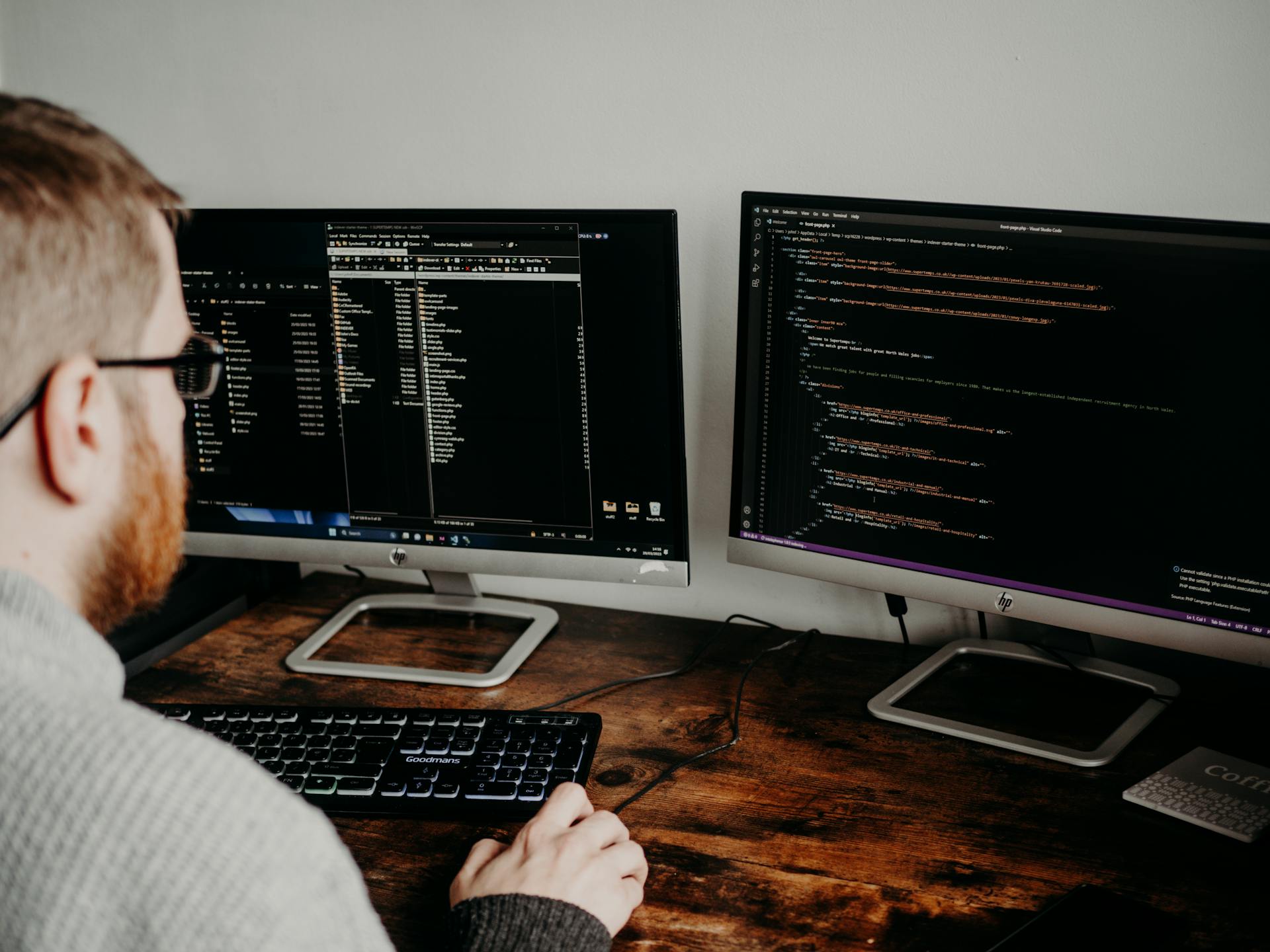
Go Packages are also a crucial concept to understand, as they help you organize and structure your code. You'll learn how to create and use packages effectively.
Finally, you'll learn about Go Pointers and Functions, which are used to manipulate memory and perform complex operations. You'll also cover Go Pointers to Struct, Go Interface, Go Empty Interface, Go Type Assertions, and Go Errors, which are all essential concepts to master as a Go developer.
By the end of this beginner's guide, you'll have a solid foundation in Go and be ready to tackle more advanced topics, such as Goroutine and Go Channel.
Why to Learn?
Learning Go is a great investment of your time, and here's why:
Go's efficient performance makes it perfect for developing high-performance applications. This means your code will run smoothly and quickly, even with large amounts of data.
With Go's concise syntax and easy design, your code will be easy to read and maintain. This is especially important for large projects where collaboration is key.
For another approach, see: Are Large Language Models Generative Ai
Go's concurrent programming capabilities allow you to run multiple activities simultaneously, making it ideal for tasks that require multitasking. This can significantly speed up development and deployment.
Go's scalability is top-notch, thanks to its lightweight goroutines and efficient memory usage. This means you can build large-scale applications without worrying about performance issues.
Go's rapid compilation speeds make development and deployment a breeze. You'll be able to test and iterate on your code quickly, without waiting for long compilation times.
The Go community is strong and open-source, offering a wide range of libraries and developer support. This means you'll have access to a wealth of resources to help you learn and grow as a developer.
Whether you're working on a Windows, macOS, or Linux project, Go's cross-platform capabilities make it easy to transfer your code between different environments.
If you're interested in building server-side applications, Go is a great choice. Its strengths in this area make it perfect for web servers and microservices.
Go's static typing feature helps detect problems during compilation, reducing the risk of issues during runtime. This means you can catch errors early and avoid costly bugs.
Lastly, learning Go is a smart move for your future career. As the popularity of Go continues to grow, having proficiency in this language will make you a more attractive candidate to potential employers.
A fresh viewpoint: Unlock Iphone without Codes
Variables & Types
Variables in Go are used to organize and store data, and they can be declared with the var keyword. The compiler can automatically deduce their type or define it explicitly.
To declare a variable, you can use the var keyword followed by the variable name and its type. However, Go also provides an operator for quick declaration, which allows you to declare multiple variables in a single line.
Here are some examples of variables declared and used in Go:
- Playground
- Hello World
- Overview of packages
- operator for quick declaration
- The word "var"
- searching style
- Null value
- The fmt file
- Developing original type
- Not casting, but conversion
A variable's type determines the value allocated to it when it is defined but not explicitly initialized. This is known as a zero value, and it's an important concept to understand when working with variables in Go.
Multiple Variables
Declaring multiple variables is a straightforward process in Go. You can use the var keyword with parentheses to declare multiple variables at once.
For example, you can declare multiple variables like this: `var (x int, y float64, z string)`. This declares three variables: x, y, and z, with their respective types.
Declaring multiple variables can make your code more concise and easier to read. It's a good practice to declare variables with their types, as it helps the compiler catch any type-related errors.
Here's a simple example of declaring multiple variables: `var (a, b, c int)`. This declares three integer variables: a, b, and c.
Variables in
Variables in Go are used to organize and store data. The var keyword is used to construct them, and the compiler can either automatically deduce their type or define them explicitly.
You can declare multiple variables at once using the var keyword with parentheses, as shown in Example 4: Multiple Variables. This can be a convenient way to initialize multiple variables with the same type.
The var keyword is used to declare variables, and it can be used with or without parentheses. For example, you can declare a single variable like this: var x = 5.
In Go, you can also use the "var" keyword to declare multiple variables with different types. For example: var a string = "Hello"; var b int = 10.
Here are some examples of variables declared and used in Go:
- Playground
- Hello World
- Overview of packages
- operator for quick declaration
- The word "var"
- searching style
- Null value
- The fmt file
- Developing original type
- Not casting, but conversion
Note that the type of a variable can be automatically deduced by the compiler if the variable is initialized with a value, or it can be explicitly defined using the var keyword.
Example 5: Constants
Constants are a type of variable that can't be changed after they're declared. They must be assigned a value at the time of declaration.
In programming, constants are declared using the const keyword. This keyword is used consistently throughout the code to indicate that a variable can't be modified.
Constants can be used to store values that don't need to change, such as the value of pi or the speed of light. These values can be used in calculations without worrying about them being altered.
For example, if you're writing a program to calculate the area of a circle, you can use a constant for the value of pi to ensure accuracy.
Zero Values
In Go, a variable's type determines the value allocated to it when it is defined but not explicitly initialized. This means that if you create a variable without assigning a value, it will receive a default value based on its type.
For example, a variable of type int will receive a value of 0, which is known as a zero value. This is similar to how a variable of type string will receive an empty string "" as its zero value.
In Go, the zero value is not just a default value, but a specific value that is allocated to the variable based on its type. This is important to keep in mind when working with variables, especially when you're not explicitly initializing them.
Strings in
Strings in Go are expressed using double quotes ("...") to represent a succession of characters. They are handled as immutable, meaning their contents cannot be modified once a string is formed.
In Go, strings are declared and initialized using the short variable declaration (:=) syntax, as seen in the example where the variable greeting is initialized with the string “Hello, Golang!”.
The fmt.Sprintf function is used for string formatting, returning a formatted string without printing it to the console.
Strings in Go have a zero value, which is determined by their type, but this is a topic covered in a separate section on variables.
Unicode Support
Go's Unicode support is excellent, allowing you to work with a wide range of international characters in your strings.
You can represent phrases like the Japanese phrase “Hello, World!” using Unicode characters in your Go strings.
Go has fundamental procedures for working with strings, which are covered in the examples provided.
These procedures will help you efficiently manage and deal with strings in your Go programs.
Sources
- https://courseflix.net/course/learn-how-to-code-google-s-go-golang-programming-language
- https://stackskills.com/p/learn-google-go-golang-programming-for-beginners
- https://www.programiz.com/golang
- https://elearnprogramming.com/products/learn-how-to-code-googles-go-golang-programming-language
- https://golang.withcodeexample.com/blog/golang-tutorial-for-beginners/
Featured Images: pexels.com