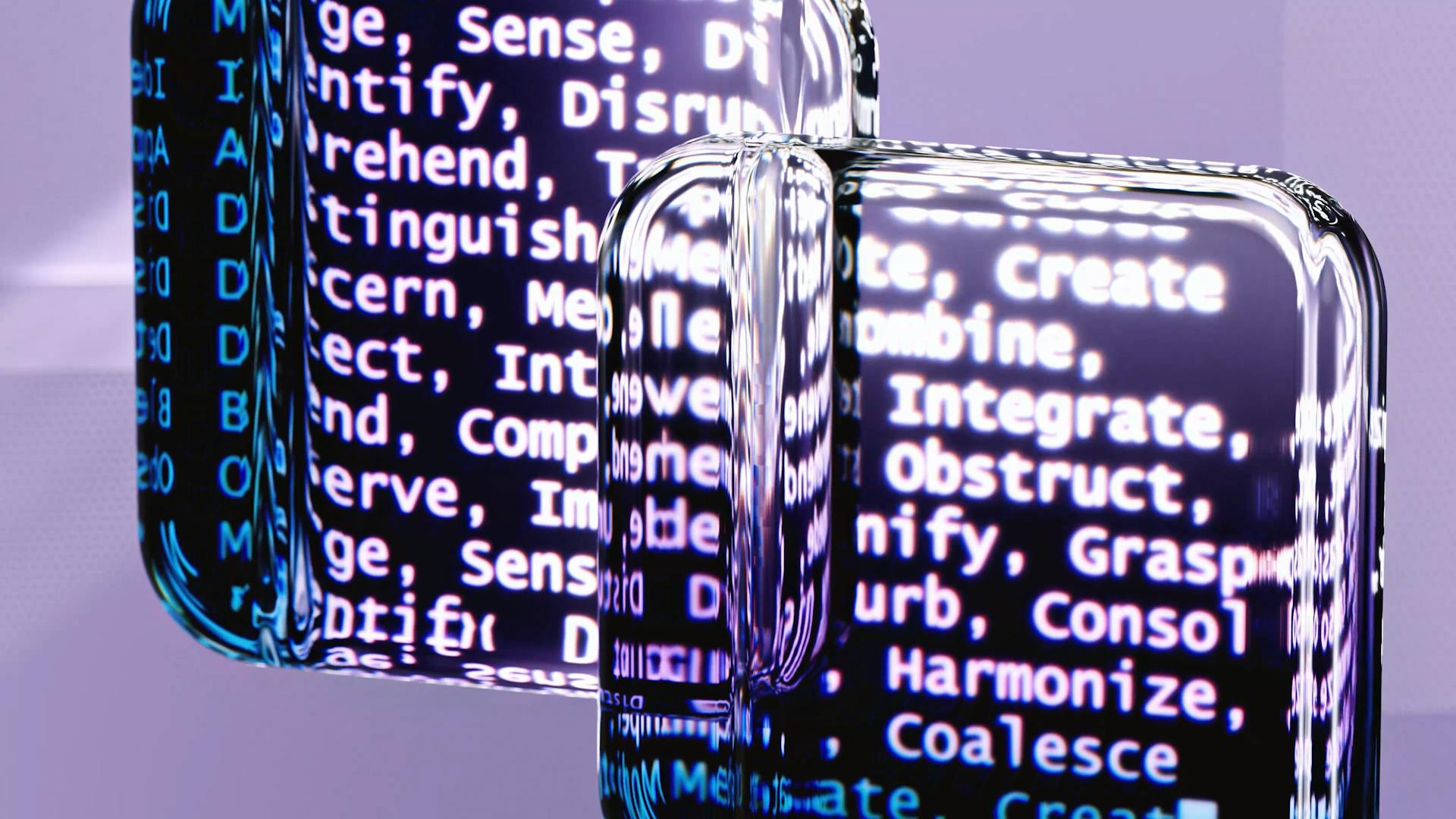
Multi Head Attention is a technique used in PyTorch to improve the performance of neural networks. It's a way to allow the network to focus on different parts of the input data simultaneously.
By using multiple attention heads, the network can capture more complex relationships between the input data. This is particularly useful for tasks like machine translation and text summarization.
In PyTorch, Multi Head Attention is implemented using the `nn.MultiHeadAttention` module. This module takes in several parameters, including the number of attention heads and the dimensionality of the input data.
The `nn.MultiHeadAttention` module is a key component of many state-of-the-art models, including the Transformer model.
Expand your knowledge: Masked Multi Head Attention
Transformer Architecture
The Transformer architecture is a popular and widely used neural network architecture for natural language processing tasks. It was first introduced in the paper "Transformer: A Novel Neural Network Architecture for Language Understanding" by Jakob Uszkoreit in 2017.
The Transformer architecture is based on the idea of self-attention, which allows the model to focus on the most relevant parts of the input sequence. This is in contrast to traditional recurrent neural networks (RNNs), which process the input sequence sequentially.
One of the key features of the Transformer architecture is its ability to handle long-range dependencies in the input sequence. This is achieved through the use of self-attention mechanisms, which allow the model to attend to any part of the input sequence simultaneously.
Here are some popular resources for learning more about the Transformer architecture:
- Transformer: A Novel Neural Network Architecture for Language Understanding (Jakob Uszkoreit, 2017)
- The Illustrated Transformer (Jay Alammar, 2018)
- Attention? Attention! (Lilian Weng, 2018)
- Illustrated: Self-Attention (Raimi Karim, 2019)
- The Transformer family (Lilian Weng, 2020)
The Transformer Architecture
The Transformer architecture is a popular choice in the field of natural language processing (NLP). It's so well-known that PyTorch has a module called nn.Transformer.
The Transformer architecture was first introduced in the paper "Transformer: A Novel Neural Network Architecture for Language Understanding" by Jakob Uszkoreit in 2017. This paper focused on the application of the Transformer in machine translation.
The Transformer architecture is based on self-attention mechanisms, which allow the model to focus on different parts of the input sequence in parallel. This is explained in more detail in the blog post "Attention? Attention!" by Lilian Weng, which covers attention mechanisms in various domains, including vision.
Check this out: Attention Mechanisms
The Transformer architecture consists of an encoder and a decoder. The encoder takes in the input sequence and outputs a continuous representation of the input. This is explained in the blog post "The Illustrated Transformer" by Jay Alammar, which provides a clear and intuitive explanation of the Transformer architecture.
Here are some notable blog posts about the Transformer architecture:
- "Transformer: A Novel Neural Network Architecture for Language Understanding" (Jakob Uszkoreit, 2017)
- "The Illustrated Transformer" (Jay Alammar, 2018)
- "Attention? Attention!" (Lilian Weng, 2018)
- "Illustrated: Self-Attention" (Raimi Karim, 2019)
- "The Transformer family" (Lilian Weng, 2020)
Scaled Dot Product
The Scaled Dot Product is a key component of the Transformer Architecture, allowing for efficient and parallelizable computations.
This technique is used in the Self-Attention mechanism, which enables the model to weigh the importance of different input elements relative to each other.
The Scaled Dot Product is computed by taking the dot product of the query and key vectors, then scaling the result by the square root of the key vector's dimensionality.
This scaling factor is necessary to prevent the dot product from dominating the self-attention weights, which would cause the model to focus too much on a single input element.
The Self-Attention mechanism uses the scaled dot product to compute a weighted sum of the input elements, allowing the model to attend to different parts of the input sequence simultaneously.
Explore further: Scaled Dot Product Attention
Attention Basics
Attention is a fundamental concept in deep learning, particularly in transformer architectures.
The attention mechanism helps the model focus on specific parts of the input sequence when generating the output.
It's based on the idea that not all parts of the input are equally important for generating the output.
In PyTorch, attention is implemented using the `torch.nn.MultiHeadAttention` class.
The attention mechanism can be thought of as a weighted sum of the input elements, where the weights are learned during training.
Attention Mechanism
The Attention Mechanism is a key component of the Multi-Head Attention model in PyTorch. It allows the model to focus on specific parts of the input sequence when generating output.
The Attention Mechanism calculates a weighted sum of the input sequence, where the weights are determined by the similarity between the input and the query. This is done using the dot product of the input and query vectors.
This process is repeated for each head of the multi-head attention model, resulting in a total of H output vectors. The output vectors are then concatenated to form the final output.
The Attention Mechanism is particularly useful for tasks such as machine translation, where the model needs to focus on specific words or phrases in the input sequence to generate accurate translations.
A fresh viewpoint: What Is Multi Head Attention
Transformer Encoder
The Transformer Encoder is a crucial component in the Transformer architecture, which is a popular neural network model used for natural language processing tasks. It's a multi-layered architecture that allows the model to attend to different parts of the input sequence simultaneously.
To implement a Transformer Encoder Layer in PyTorch, you can use the `nn.MultiheadAttention` module, which is part of the PyTorch library. This module provides a way to perform self-attention, which is a key component of the Transformer architecture.
Here's a step-by-step example of how to use `nn.MultiheadAttention` in a practical scenario: You define the `TransformerEncoderLayer` class.You instantiate an object of this class with specific parameters.You create some dummy input data with a shape of (sequence length, batch size, embedding dimension).You pass the dummy input through the encoder layer.You print the shape of the output to ensure it matches the expected shape.
Transformer Encoder
The Transformer Encoder is a crucial component of the Transformer architecture, and it's used for sequence-to-sequence tasks such as machine translation.
In the Transformer architecture, the Transformer Encoder Layer is a single layer of the transformer encoder, which uses nn.MultiheadAttention for self-attention and includes feedforward neural networks, layer normalization, and dropout for regularization.
The TransformerEncoderLayer class implements a single layer of a transformer encoder, and it's instantiated with specific parameters. The class can be used to create a transformer encoder with multiple layers.
To implement a Transformer Encoder Layer, you can use the TransformerEncoderLayer class, which is a practical scenario to illustrate the usage of nn.MultiheadAttention.
Here's a step-by-step guide to implementing a Transformer Encoder Layer:
1. Define the TransformerEncoderLayer class.
2. Instantiate an object of this class with specific parameters.
3. Create some dummy input data with a shape of (sequence length, batch size, embedding dimension).
4. Pass the dummy input through the encoder layer.
5. Print the shape of the output to ensure it matches the expected shape.
Here are some additional resources to learn more about the Transformer Encoder:
- Transformer: A Novel Neural Network Architecture for Language Understanding (Jakob Uszkoreit, 2017) - The original Google blog post about the Transformer paper, focusing on the application in machine translation.
- The Illustrated Transformer (Jay Alammar, 2018) - A very popular and great blog post intuitively explaining the Transformer architecture with many nice visualizations. The focus is on NLP.
- Attention? Attention! (Lilian Weng, 2018) - A nice blog post summarizing attention mechanisms in many domains including vision.
- Illustrated: Self-Attention (Raimi Karim, 2019) - A nice visualization of the steps of self-attention. Recommended going through if the explanation below is too abstract for you.
- The Transformer family (Lilian Weng, 2020) - A very detailed blog post reviewing more variants of Transformers besides the original one.
Positional Encoding
The Transformer Encoder's positional encoding is a clever way to add position information to the input features. This is necessary because the Multi-Head Attention block is permutation-equivariant, meaning it can't tell the difference between an input's position in the sequence.
The position encoding is implemented using sine and cosine functions of different frequencies. These functions are applied to the input features to create a pattern that can be identified by the network.
The specific pattern chosen by Vaswani et al. uses sine and cosine functions of different frequencies, ranging from 2π to 10000⋅2π. This allows the model to easily attend to relative positions.
The positional encoding is added to the original input features, and the resulting values are concatenated for all hidden dimensions. This creates a position information that can be used by the model.
The code for the positional encoding is taken from the PyTorch tutorial about Transformers on NLP and adjusted for our purposes. It's a great example of how to implement this technique in practice.
The positional encoding can be visualized to get a better understanding of the pattern. This visualization shows the sine and cosine waves with different wavelengths that encode the position in the hidden dimensions.
Key Components
The key components of the Transformer Encoder's MultiheadAttention module are crucial to its functionality.
The embed_dim parameter determines the total dimension of the model, which is a critical aspect of the Transformer Encoder's architecture.
The num_heads parameter specifies the number of parallel attention heads, allowing the model to process multiple aspects of the input data simultaneously.
The dropout parameter controls the dropout probability on attention weights, which helps prevent overfitting and improve the model's robustness.
Bias is added to the input/output projection layers when the bias parameter is set to True.
When add_bias_kv is True, bias is added to the key and value sequences, which can enhance the model's ability to learn complex relationships.
The kdim and vdim parameters specify the total number of features for keys and values, respectively, which are used in the attention mechanism.
The batch_first parameter determines the order of the input and output tensors, with a default value of False (seq, batch, feature) unless set to True (batch, seq, feature).
Here are the key components of the MultiheadAttention module summarized:
Parameter | Description |
---|---|
embed_dim | Total dimension of the model |
num_heads | Number of parallel attention heads |
dropout | Dropout probability on attention weights |
bias | Whether to add bias to input/output projection layers |
add_bias_kv | Whether to add bias to key and value sequences |
kdim and vdim | Total number of features for keys and values, respectively |
batch_first | Determines the order of input and output tensors |
Frequently Asked Questions
What is the difference between single head and multihead attention?
Single head attention focuses on one position at a time, whereas multihead attention allows the model to jointly attend to multiple positions simultaneously, enhancing its ability to process complex information. This key difference is a major factor behind the success of the Transformer model.
What is MHA in machine learning?
Multi-Head Attention (MHA) is a key operator in the Transformer architecture that enables models to focus on different parts of the input data simultaneously. It's a crucial component in many machine learning frameworks, including PyTorch, and has revolutionized the field of natural language processing.
Sources
- https://uvadlc-notebooks.readthedocs.io/en/latest/tutorial_notebooks/tutorial6/Transformers_and_MHAttention.html
- https://www.geeksforgeeks.org/how-to-use-pytorchs-nnmultiheadattention/
- https://pytorch.org/docs/stable/generated/torch.ao.nn.quantizable.MultiheadAttention.html
- https://pytorch.org/torchtune/stable/generated/torchtune.modules.MultiHeadAttention.html
- https://runebook.dev/en/docs/pytorch/generated/torch.nn.multiheadattention
Featured Images: pexels.com