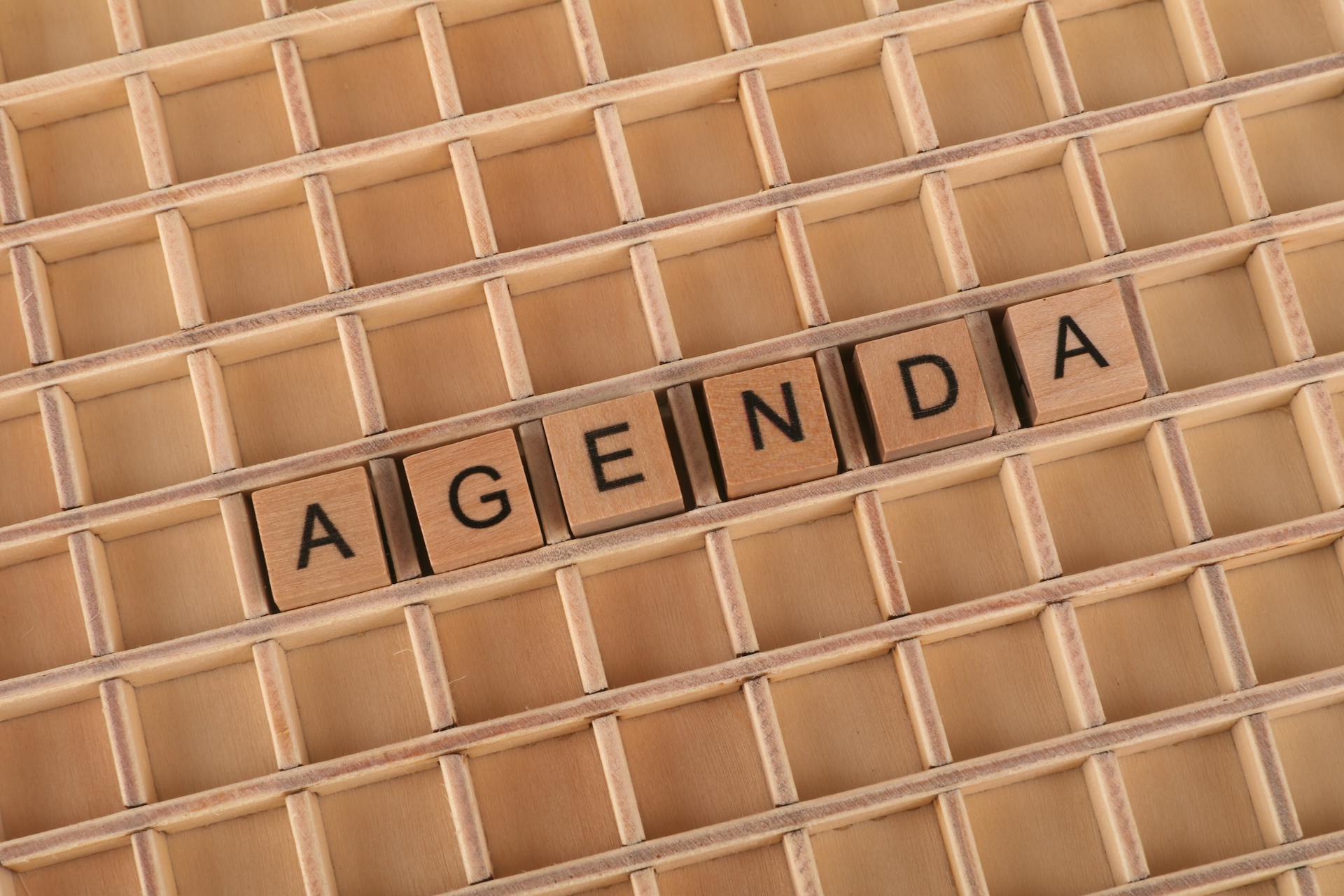
Grid search is a method used to find the optimal parameters for a machine learning model. It involves systematically trying out different combinations of parameters to see which one performs best.
Grid search is a brute force approach, meaning it tries every possible combination of parameters. This can be computationally expensive, especially for models with many parameters.
The goal of grid search is to find the combination of parameters that results in the best performance. This is typically measured by a metric such as accuracy or mean squared error.
Grid search can be implemented using libraries such as scikit-learn in Python.
What is Grid Search
Grid search is a simple yet powerful technique used to find the best combination of parameters in a machine learning model. It involves systematically varying each parameter across a range of values and evaluating the model's performance at each point.
A grid search can be computationally expensive, especially for models with many parameters. For example, if a model has 5 parameters, each with 10 possible values, the total number of combinations to try is 10^5, or 100,000.
Discover more: Grid Search Python
The goal of a grid search is to find the combination of parameters that yields the best performance, which is typically measured by metrics such as accuracy, precision, or recall. This can be a time-consuming process, but it can also provide valuable insights into the relationships between parameters and performance.
Grid search is often used in combination with other techniques, such as cross-validation, to ensure that the model is not overfitting or underfitting the training data. By systematically varying the parameters and evaluating the model's performance, grid search can help identify the optimal combination of parameters for a given problem.
Implementing Grid Search
Implementing Grid Search is a straightforward process, especially with libraries like Scikit-learn, which provides a built-in GridSearchCV function. This function allows users to specify the model, the hyperparameter grid, and the cross-validation strategy.
Grid Search in Scikit-learn's GridSearchCV is a technique used to search and find the optimal combination of hyperparameters for a given model. It systematically explores a predefined set of hyperparameter values, creating a “grid” of possible combinations.
Suggestion: Machine Learning Hyperparameter
The GridSearchCV methodically explores various combinations of hyperparameter values within a predetermined grid. This grid establishes the potential values for each hyperparameter. Cross-Validation is used by GridSearchCV to assess the performance of each combination of hyperparameters.
You can define a parameter grid for a support vector machine (SVM) model and use GridSearchCV to automatically evaluate each combination. The results can then be accessed to determine the best hyperparameters, which can be used to retrain the model for final evaluation.
Here are the steps to implement Grid Search in Scikit-learn:
1. Define the model and the hyperparameter grid
2. Use GridSearchCV to explore the hyperparameter space
3. Evaluate each combination of hyperparameters using cross-validation
4. Select the best combination of hyperparameters based on the evaluation results
By following these steps, you can implement Grid Search in Scikit-learn and find the optimal combination of hyperparameters for your model.
GridSearchCV is a powerful tool for hyperparameter tuning, and it can be used in conjunction with other techniques such as cross-validation to improve the accuracy of your model.
Here is an example of how to use GridSearchCV to tune the hyperparameters of a Support Vector Machine (SVM) model:
```
param_grid = {'kernel': ['linear', 'rbf', 'poly'], 'C': [1, 10, 100]}
grid_search = GridSearchCV(estimator=SVM(), param_grid=param_grid, cv=5)
grid_search.fit(X, y)
print(grid_search.best_params_)
```
This code defines a parameter grid for the SVM model, uses GridSearchCV to explore the hyperparameter space, and prints the best combination of hyperparameters found during the search.
Worth a look: Grid Search Hyperparameter Tuning
Grid Search Techniques
Grid search is a hyperparameter tuning technique used to find the optimal combination of model parameters. It involves defining a grid of possible values for the hyperparameters and evaluating the model's performance for each combination. The caret package in R is used to perform grid search, as seen in Example 1.
The trainControl() function is used to define the method of cross-validation to be carried out and the search type, which can be either "grid" or "random". The tuneGrid argument is used to specify the tuning parameters and applies grid search CV on them. In Example 1, the trainControl() function is used to specify the CV technique, which is K-fold cross-validation with 5 folds.
A grid search can be customized by specifying a tuning grid, as shown in Example 1. The gbmGrid is created using the expand.grid() function, which defines the possible values for the hyperparameters. The max_depth and nrounds hyperparameters are specified with possible values of 3, 5, 7 and 1 to 10 times 50, respectively.
A different take: Python Grid Search Cv
Randomized Parameter Optimization
Randomized Parameter Optimization is a method that can be more efficient than Grid Search, particularly when the hyperparameter space is large. It randomly samples a specified number of combinations from the hyperparameter space, leading to faster results.
RandomizedSearchCV implements a randomized search over parameters, where each setting is sampled from a distribution over possible parameter values. This has two main benefits over an exhaustive search: a budget can be chosen independent of the number of parameters and possible values, and adding parameters that do not influence the performance does not decrease efficiency.
You can specify how parameters should be sampled using a dictionary, similar to specifying parameters for GridSearchCV. A computation budget, being the number of sampled candidates or sampling iterations, is specified using the n_iter parameter.
For each parameter, you can either specify a distribution over possible values or a list of discrete choices (which will be sampled uniformly). The scipy.stats module contains many useful distributions for sampling parameters, such as expon, gamma, uniform, loguniform, or randint.
To take full advantage of the randomization, it's essential to specify a continuous distribution for continuous parameters, such as C. This way, increasing n_iter will always lead to a finer search.
A continuous log-uniform random variable is the continuous version of a log-spaced parameter. For example, to specify the equivalent of C from above, you can use loguniform(1,100) instead of [1,10,100].
Here are some key points to keep in mind when comparing randomized search and grid search for hyperparameter estimation:
- Randomized search can yield comparable or even better performance than grid search, particularly when the hyperparameter space is large.
- Randomized search does not require exhaustive evaluation, leading to faster results.
- Specifying a continuous distribution for continuous parameters is essential to take full advantage of the randomization.
Bergstra, J. and Bengio, Y., Random search for hyper-parameter optimization, The Journal of Machine Learning Research (2012), provides a comprehensive overview of randomized search for hyperparameter optimization.
Optimal Parameters
Grid search is a method of hyperparameter tuning that allows you to search over a grid of parameter settings to find the optimal combination that results in the best performance.
Randomized search over parameters is another method that can be used, where each setting is sampled from a distribution over possible parameter values.
A continuous log-uniform random variable is the continuous version of a log-spaced parameter, and is an important distribution to specify for continuous parameters.
Specifying how parameters should be sampled is done using a dictionary, very similar to specifying parameters for GridSearchCV.
A budget can be chosen independent of the number of parameters and possible values when using randomized search, which is a significant advantage over an exhaustive search.
Adding parameters that do not influence the performance does not decrease efficiency when using randomized search.
The number of sampled candidates or sampling iterations is specified using the n_iter parameter.
To specify a continuous random variable that is log-uniformly distributed between 1e0 and 1e3, you can use the loguniform function.
Here are some key benefits of using randomized search over grid search:
- A budget can be chosen independent of the number of parameters and possible values.
- Adding parameters that do not influence the performance does not decrease efficiency.
Here are some common distributions used in randomized search:
- expon
- gamma
- uniform
- loguniform
- randint
These distributions can be used to sample parameters from a distribution over possible values.
Analyzing Results
The cv_results_ attribute contains useful information for analyzing the results of a search. It can be converted to a pandas dataframe with df=pd.DataFrame(est.cv_results_).
Each row corresponds to a given parameter combination (a candidate) and a given iteration. The iteration is given by the iter column. The n_resources column tells you how many resources were used.
The best parameter combination is the one that has reached the last iteration with the highest score. In the example above, the best parameter combination is {'criterion':'log_loss','max_depth':None,'max_features':9,'min_samples_split':10} since it has reached the last iteration (3) with the highest score: 0.96.
The cv_results_ attribute of HalvingGridSearchCV and HalvingRandomSearchCV is similar to that of GridSearchCV and RandomizedSearchCV, with additional information related to the successive halving process.
Here's a breakdown of the columns in the cv_results_ attribute:
Alternatives and Robustness
Grid search can be a robust method, but it's not foolproof. Some parameter settings may result in a failure to fit one or more folds of the data.
By default, the score for those settings will be np.nan, indicating a failed fit. This can be controlled by setting error_score to "raise" to raise an exception if one fit fails, or for example error_score=0 to set another value for the score of failing parameter combinations.
Benefits of Using
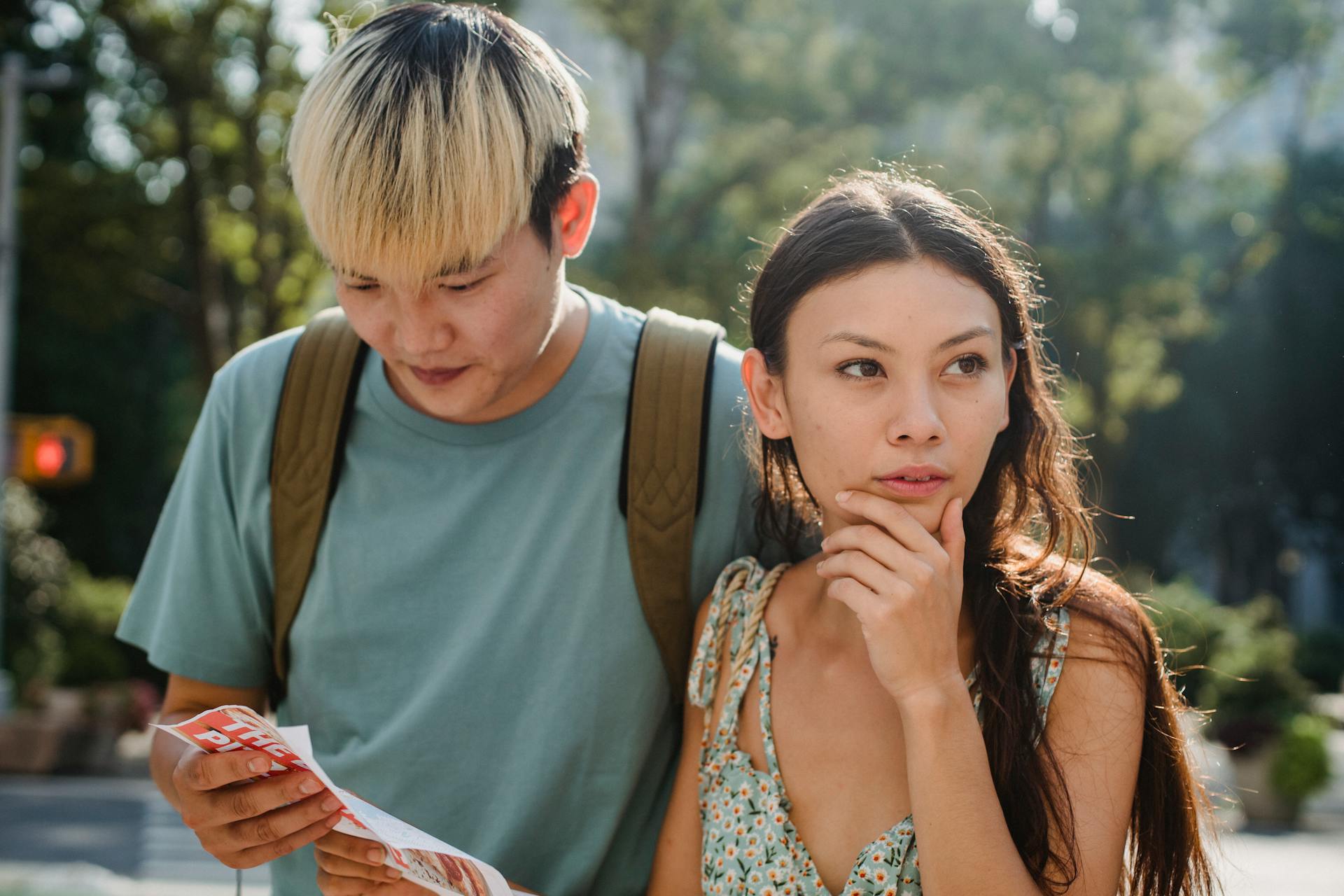
Grid Search is a reliable option for hyperparameter tuning because it evaluates every possible combination of hyperparameters, increasing the likelihood of finding the optimal settings for a given model.
One of the primary benefits of using Grid Search is its exhaustive nature, which can lead to significant improvements in model accuracy and performance. This thorough approach provides a clear methodology for hyperparameter tuning, making it accessible for both novice and experienced data scientists.
Limitations
Grid Search has some significant limitations that can't be ignored. The most notable drawback is its computational cost, which can grow exponentially with large datasets or complex models.
This means that training the model for each combination can take a very long time, leading to long wait times for results. In some cases, it can even be impractical to use Grid Search due to its high computational requirements.
Grid Search may not always find the best hyperparameter configuration, particularly if the grid is not fine-grained enough or if the parameter space is large and complex. This can lead to suboptimal results and a lot of unnecessary trial and error.
Alternatives to Brute Force
Grid Search is a brute force approach to hyperparameter tuning, but it's not the only option. Grid Search evaluates every possible combination of hyperparameters, which can be time-consuming and inefficient, especially when dealing with a large number of parameters.
Grid Search can be compared to Random Search, which randomly samples a specified number of combinations from the hyperparameter space. This approach can lead to faster results and comparable or even better performance than Grid Search.
Randomized Search is another alternative that implements a randomized search over parameters, where each setting is sampled from a distribution over possible parameter values. This method has two main benefits: a budget can be chosen independent of the number of parameters and possible values, and adding parameters that don't influence performance doesn't decrease efficiency.
Randomized Search can be specified using a dictionary, similar to Grid Search, and a computation budget can be set using the n_iter parameter. For each parameter, a distribution over possible values or a list of discrete choices can be specified, which can be sampled uniformly.
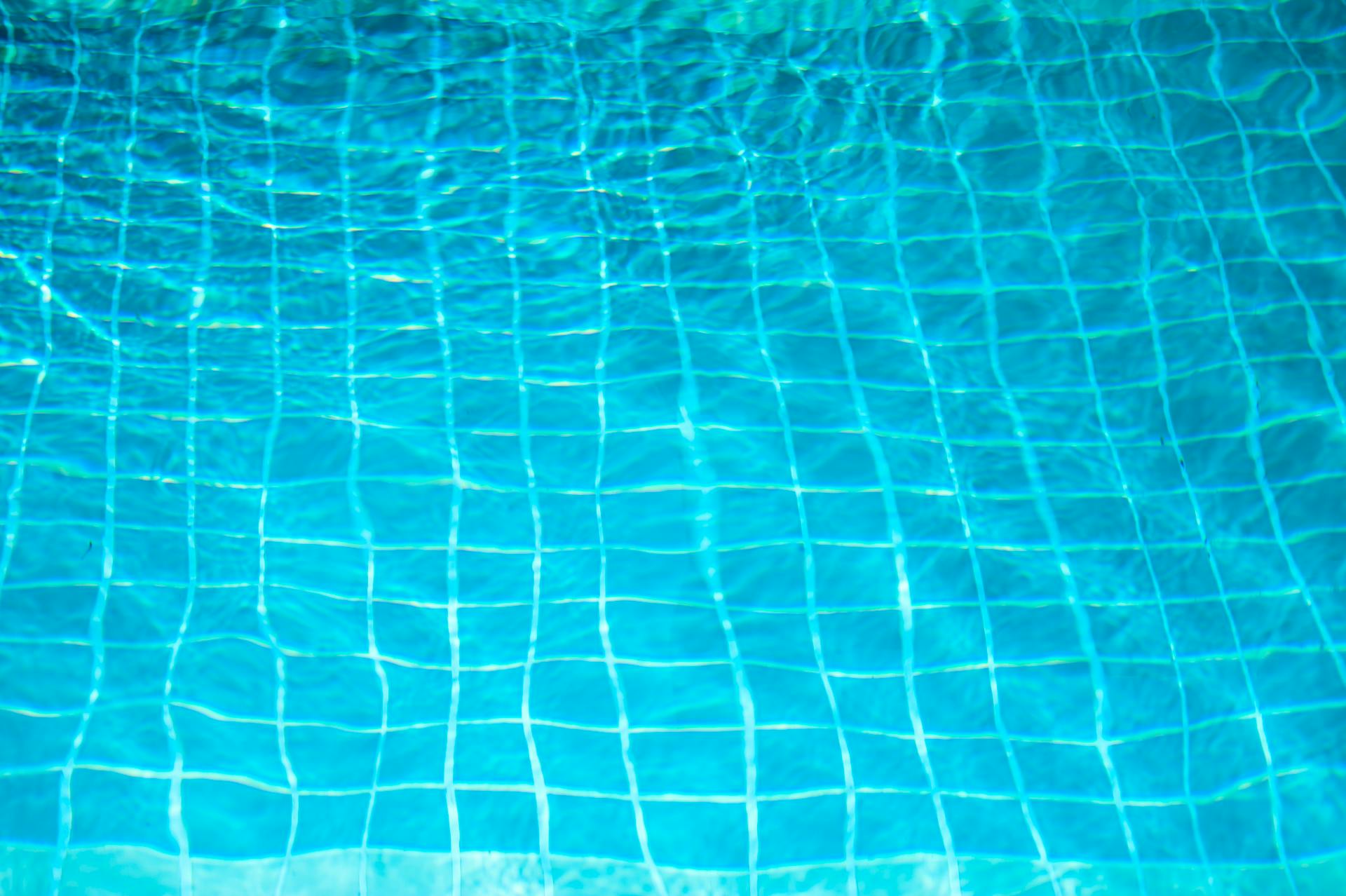
Some common distributions used in Randomized Search include the exponential, gamma, uniform, and loguniform distributions, which can be found in the scipy.stats module. By using these distributions, you can specify how parameters should be sampled and take advantage of the randomization.
Here are some key differences between Grid Search and Randomized Search:
By using Randomized Search, you can obtain similar accuracy in a fraction of the time it takes for Grid Search, as demonstrated in the example where the random search took only 36 seconds compared to the grid search, which took 4 minutes and 34 seconds.
Best Practices
Limiting the number of hyperparameters being tuned at once can help reduce computational costs.
Starting with a coarse grid and gradually refining it based on initial results allows for a more focused search on the most promising areas of the hyperparameter space.
Using parallel processing can significantly speed up the Grid Search process, enabling faster evaluations of multiple combinations simultaneously.
Robustness to Failure
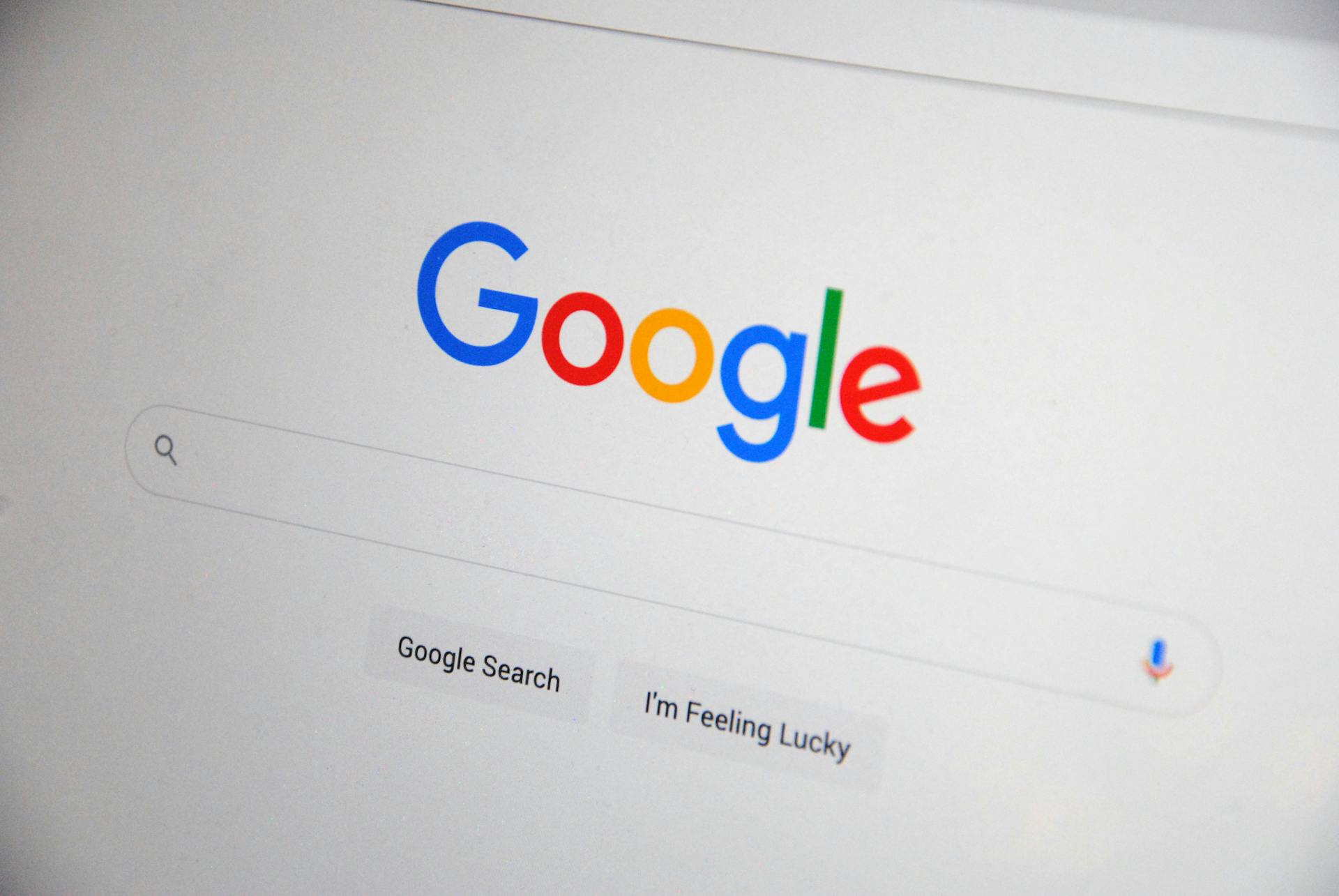
Robustness to failure is a crucial aspect of any model. Some parameter settings may result in a failure to fit one or more folds of the data.
By default, the score for those settings will be np.nan. This can be controlled by setting error_score to "raise" to raise an exception if one fit fails.
Setting error_score to 0 will set another value for the score of failing parameter combinations.
Scikit-Learn and Cross-Validation
Cross-validation is a crucial aspect of GridSearchCV in scikit-learn. It helps evaluate model performance by dividing the dataset into training and validation sets.
The most popular type of Cross-validation is K-fold Cross-Validation, which is an iterative process that divides the train data into k partitions. Each iteration keeps one partition for testing and the remaining k-1 partitions for training the model.
GridSearchCV performs cross-validation while training the model, making it a time-consuming process. The process records the performance of the model in each iteration and gives the average of all the performance at the end.
The performance measure used in GridSearchCV is specified by the 'scoring' parameter, which can be 'r2' for regression models or 'precision' for classification models.
GridSearchCV along with cross-validation takes huge time cumulatively to evaluate the best hyperparameters, but it provides a robust assessment of model accuracy.
Putting It Together
When you've found the best combination of hyperparameters, you can retrieve it using the clf.best_params_ attribute. This gives you the best combination of tuned parameters for your model.
The best score of your Random Forest Classifier is stored in clf.best_score_. This score represents the average cross-validated score of your model.
As you work with grid search, you'll likely need to save and load user states across page requests. This is where the "Preserves users' states across page requests" feature comes in handy.
The IT Engineering Graduate who wrote the example code was currently pursuing a Post Graduate Diploma in Data Science. This shows that grid search is a valuable tool for data science professionals.
Machine Learning and Grid Search
Grid Search is a technique used in machine learning to automate the hyperparameter tuning process. This ensures that preprocessing steps and model training are executed in a cohesive manner, enhancing reproducibility and simplifying the process of hyperparameter optimization.
GridSearchCV is a tool from the scikit-learn library used for hyperparameter tuning in machine learning, which automates the process of finding the optimal combination of hyperparameters for a given machine learning model.
Hyperparameters manage how a machine learning model learns, and Grid Search systematically explores various combinations of hyperparameter values within a predetermined grid. This grid establishes the potential values for each hyperparameter.
Grid Search uses Cross-Validation to assess the performance of each combination of hyperparameters by dividing the data into folds, training the model on certain folds, and testing on the rest of the folds. This process is repeated for all folds and hyperparameter combinations.
GridSearchCV helps in automating the process of hyperparameter tuning, enhancing model performance, and avoiding manual trial-and-error. It systematically explores a predefined set of hyperparameter values, creating a “grid” of possible combinations.
The primary purpose of GridSearchCV is to identify the optimal hyperparameters for a machine learning model by evaluating each combination on various dataset sections and determining the best settings for the model. This process ensures the model operates optimally without excessive computational costs.
Grid Search Cross-Validation (GridSearchCV) is a technique used in machine learning to search and find the optimal combination of hyperparameters for a given model. It systematically explores a predefined set of hyperparameter values, creating a “grid” of possible combinations.
GridSearchCV helps in automating the process of hyperparameter tuning, enhancing model performance, and avoiding manual trial-and-error.
Cross-Validation and Model Selection
Cross-validation is a crucial step in the grid search process, and it's essential to understand how it works. It's a technique used to evaluate the performance of a model by dividing the data into training and validation sets.
To perform cross-validation, you need to split your data into two parts: a development set and an evaluation set. The development set is used to train the model, while the evaluation set is used to test its performance. This is done to prevent overfitting, where the model becomes too complex and performs well only on the training data.
GridSearchCV uses cross-validation to evaluate the performance of each combination of hyperparameters. It divides the data into k partitions, trains the model on k-1 partitions, and tests its performance on the remaining partition. This process is repeated for all k partitions, and the average performance is calculated.
Here are the key points to remember about cross-validation in GridSearchCV:
- It's used to evaluate the performance of a model by dividing the data into training and validation sets.
- It prevents overfitting by testing the model on unseen data.
- It's an iterative process that repeats for all k partitions.
- The average performance is calculated to get a comprehensive evaluation.
By using cross-validation, GridSearchCV can provide a robust assessment of model accuracy, but it can also be time-consuming. However, the benefits of cross-validation far outweigh the costs, as it helps to identify the best combination of hyperparameters for your model.
Conclusions
Grid Search CV is a crucial model selection step that should be performed after Data Processing tasks.
It's essential to leverage sklearn grid search cv to ensure that the grid search is exhaustive, providing the best possible model performance.
Grid Search CV is a powerful tool in scikit-learn for hyperparameter tuning, particularly with models like RandomForestClassifier.
It systematically searches for optimal parameters, enhancing performance through effective cross-validation (CV) in Random Forest hyperparameter tuning.
GridSearchCV is not a library itself, but rather a class provided by the popular Python machine learning library scikit-learn (sklearn).
By utilizing GridSearchCV, you can efficiently explore different hyperparameter combinations and optimize your model's performance.
Grid search is a method for hyperparameter optimization that systematically evaluates all possible combinations of hyperparameter values within a predefined grid to find the best-performing set of hyperparameters.
It's a straightforward approach but can be computationally expensive for models with many hyperparameters.
GridSearchCV guarantees finding the best within the grid but can be computationally expensive.
It's always good to compare the performances of Tuned and Untuned Models to ensure you're getting the best results.
This will cost us the time and expense, but it will surely give us the best results.
Frequently Asked Questions
What is the difference between grid search and random search?
Grid search evaluates all possible combinations systematically, while random search balances exploration and efficiency by randomly sampling hyperparameters. This difference affects the trade-off between thoroughness and computational cost.
What is GridSearchCV used for?
GridSearchCV is a technique used to find the best combination of model parameters through cross-validation, optimizing model performance. It's a powerful tool for hyperparameter tuning in machine learning.
Sources
- https://statisticseasily.com/glossario/what-is-grid-search/
- https://scikit-learn.org/1.5/modules/grid_search.html
- https://pyimagesearch.com/2021/05/17/introduction-to-hyperparameter-tuning-with-scikit-learn-and-python/
- https://www.projectpro.io/recipes/tune-hyper-parameters-grid-search-r
- https://www.analyticsvidhya.com/blog/2021/06/tune-hyperparameters-with-gridsearchcv/
Featured Images: pexels.com