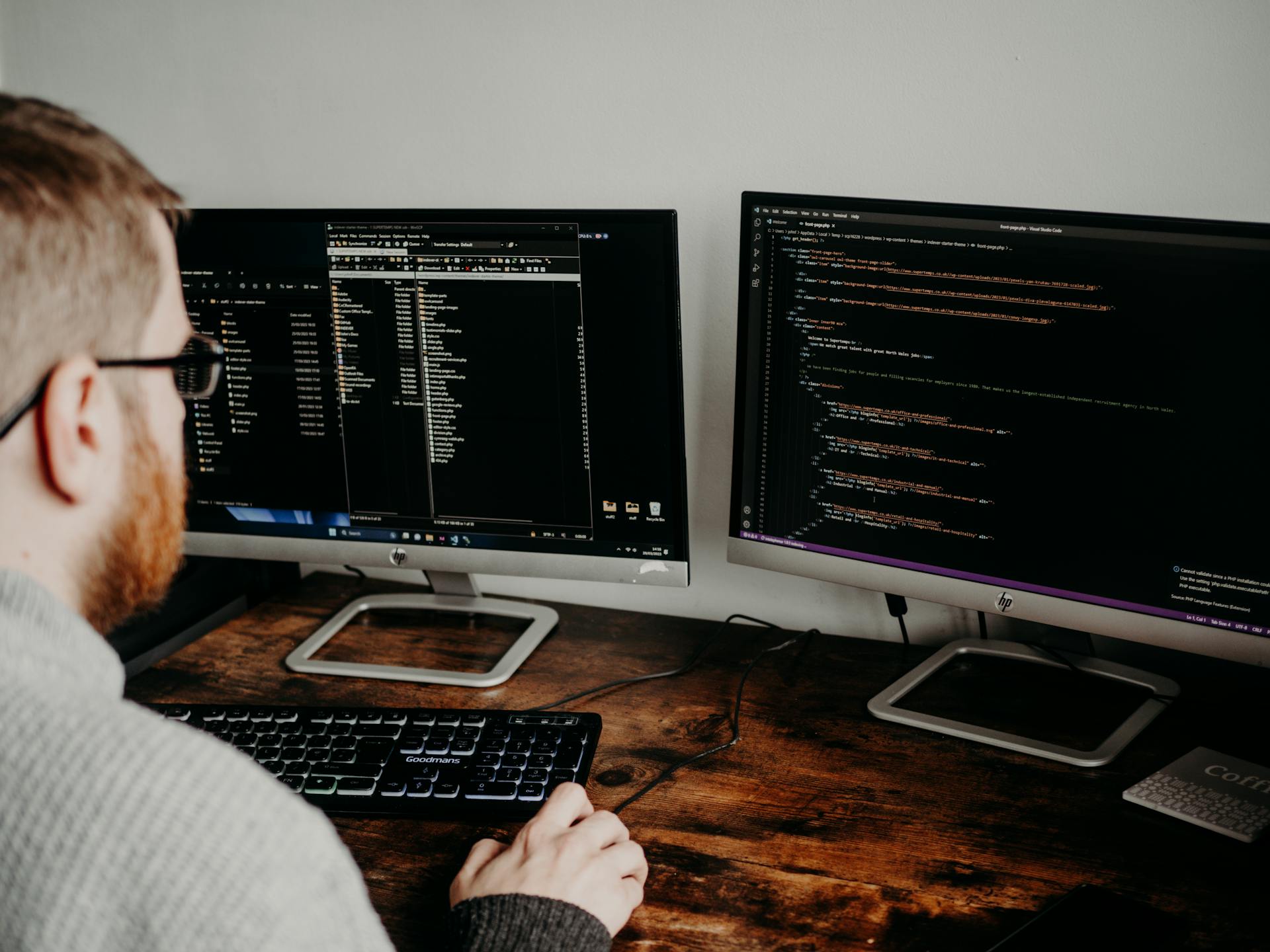
Pursuing a Bachelor's in Computer Science is a fantastic way to kickstart your tech career. The program typically takes four years to complete and covers a wide range of topics, including data structures, algorithms, computer systems, and software engineering.
Most Bachelor's in Computer Science programs are accredited by the Computing Accreditation Commission of ABET, which ensures that the program meets rigorous standards. This accreditation is a significant advantage when applying for jobs or graduate programs.
A Bachelor's in Computer Science program usually requires students to complete a set of core courses, which can include data structures, computer systems, and software engineering. These courses provide a solid foundation in computer science principles and prepare students for more advanced topics.
Many colleges and universities offer online or part-time Bachelor's in Computer Science programs, which can be a great option for students who need flexibility in their schedules.
For another approach, see: Informatics Engineering
What Is
Computer science is a fascinating field that explores the theory and application of computational systems. It's a blend of many fields, including mathematics, electrical engineering, and robotics.
Computer science has a rich history that dates back to World War II, when several countries developed electronic digital computing to gain a technological upper hand. After the war, computer science continued to grow and evolve.
The field of computer science encompasses many areas, including software design, algorithms, data structures, and human-computer interaction. This is because computer science is an interdisciplinary field that draws on knowledge from various disciplines.
Here are some of the key areas of focus in computer science:
- Algorithms
- Data structures
- Artificial intelligence
- Cybersecurity
- Discrete algebra
- Human-computer interaction
A bachelor's degree in computer science can equip you with the skills and knowledge needed to pursue a career in this field. With a degree in hand, you'll be well-prepared to tackle complex problems and develop innovative solutions.
Intriguing read: Compsci Degree Planner
Binary Search Tree and Data Structures
A binary search tree is a data structure that's particularly well suited for "search" scenarios, where you need to rapidly access data. It's also known as a BST.
Consider reading: Compsci 61b Data Structures
In a binary search tree, every node has a value, and both children are nodes just like the root, with values that are smaller or larger than its value. Every value in a node's left tree is smaller, and every value in its right tree is larger.
Values that are equal can go either way, but it's best to be consistent, like putting them on the left. If any of these rules are violated, then your tree isn't a binary search tree.
Binary Search Tree
A binary search tree is a data structure that's particularly well-suited for "search" scenarios. It's made up of nodes, each with a value and pointers to its left and right children.
The tree is ordered by value, so whenever you insert a new value, it will be inserted in a sorted fashion. This means that every value in a node's left tree is smaller than its value, and every value in its right tree is larger.
The binary part of the name means there are at most two children nodes per node. This is in contrast to a LinkedList, which only has one next pointer.
Every node has a value, and both children are nodes just like the root. They can have 0-2 children and will always have a value. Values that are equal can go either way, but it's best to be consistent.
If the rules of the binary search tree are violated, then it's not a binary search tree. All of the rules must be followed 100% of the time, which makes it really fast to use in certain scenarios.
The algorithm for look ups is simple: go left or right depending if it's smaller or bigger, and then you'll find it. The average case for look ups is O(log n), and the best case is O(1) if someone asks for the root.
Delete
Deleting a node from a Binary Search Tree (BST) is a bit more complex than inserting or searching, but it's still relatively efficient.
To delete a node, you need to replace its value with the smallest child in the right child's subtree, which is also known as the least child in the right subtree.
You find this node by following right children in the right subtree until you hit the last one, which is just one hop in this case.
This replacement doesn't change the overall structure of the tree, so the Big O of this operation remains O(log n).
In the worst-case scenario, you might need to hop more than one time to find the least child in the right subtree, but the time complexity is still the same.
As you can see, deleting a node from a BST is a bit more involved than other operations, but it's still a vital part of maintaining the tree's integrity.
The key takeaway is that you need to replace the deleted node's value with the smallest child in the right subtree, and then move the deleted node's right child to the new node's left child.
Worst Case
In the worst case scenario, a Binary Search Tree (BST) can be severely unbalanced, leading to poor performance. This happens when you add elements in a specific order, like a sorted list of numbers.
The worst case BST is created when you add elements in order, which can be disastrous for a BST. Unfortunately, a BST has no built-in mechanism to handle this.
A classic example of this is when you add a sorted list of numbers to a BST, which can result in a severely unbalanced tree. This is why you'll never use BSTs directly yourself.
There are, however, variations of BSTs that are designed to deal with these worst-case scenarios.
CS Bachelor's Skills and Requirements
A computer science bachelor's degree can provide a solid foundation in technical, practical, theoretical, and soft skills. Students can learn programming languages like Java or Python, and develop skills in areas such as algorithms, data structures, and artificial intelligence.
The skills you acquire in a computer science program depend on your concentration, program emphasis, and choice of BS or BA degree. Common computer science skills include theory and mathematics of computation, problem analysis, decision loops, variables, and technical writing.
Here are some of the specific skills you can expect to learn in a computer science program:
- Theory and mathematics of computation
- Problem analysis
- Programming languages, such as Java or Python
- Decision loops, variables, and how to write functions
- Technical writing
- Algorithms
- Data structures
- Artificial intelligence
- Cybersecurity
In addition to these technical skills, you'll also learn soft skills like communication, time management, attention to detail, and collaboration.
Are Bachelor's Degrees Expensive?
The cost of a Bachelor's degree in Computer Science can be a significant concern for many students. As of the 2020-21 school year, the average net price for one year of college at public institutions stood at $14,700 and $28,400 at private nonprofit institutions, according to the National Center for Education Statistics.
Tuition for online computer science programs can vary, but it can run from $130-$550 per credit. Online degrees may be more affordable than on-campus programs, but other factors also affect the cost of higher education.
Curious to learn more? Check out: Ai in Computer Science
Large public universities often charge less than small private colleges, so your location can impact your tuition rate. Your state residency may also determine your tuition rate at a public university.
Some schools offer subscription-based programs, which allow you to complete assignments at your own pace. Highly motivated students can save money by completing coursework quickly.
You can also use financial aid options like scholarships, grants, military or veterans funding, or employer tuition assistance to help cover the costs.
Scholarships for Bachelor's
Many private companies and foundations offer scholarships for computer science majors, which can help alleviate the financial burden of pursuing this degree. Typically, applicants must meet the donor organization's criteria for support and often have to compete against other qualified applicants.
Some scholarships may support computer science majors broadly, while others only fund students in specific concentrations, such as cybersecurity or software development. The list of scholarships for computer science majors is not comprehensive, but it offers an example of relevant scholarships for students in this major.
Consider reading: Ai Computer Scientist
The Betty Stevens Frecknall Scholarship supports students pursuing computer science or other related majors, with a focus on those who are U.S. citizens or residents enrolled full time in accredited institutions. Applicants must have one semester or more of college credits and a GPA of 3.0 or better.
Lockheed Martin awards $10,000 to 200 recipients studying engineering or computer science each year, with the option to renew the scholarship up to three times, totaling $40,000. An applicant must hold a 2.5 GPA or higher and be willing to intern with Lockheed Martin to qualify.
The Palantir Future Scholarship supports students from racial and ethnic groups historically underrepresented in technology careers, who can be first-year, second-year, or third-year students in accredited colleges in the U.S., Mexico, or Canada.
A computer science degree can open doors to many dynamic careers, with the BLS projecting that employment in computer and information technology will grow faster than average from 2022-2032.
CS Bachelor's Skills
A CS bachelor's degree can open doors to many dynamic careers, with a median annual wage of $104,920 as of May 2023. Students can learn technical, practical, theoretical, and soft skills in these programs.
You can learn theory and mathematics of computation, problem analysis, programming languages like Java or Python, decision loops, variables, and how to write functions. Technical writing is also a valuable skill to acquire.
Soft skills like communication, time management, attention to detail, and collaboration are essential in any field. These skills will help you work effectively with others and manage your time wisely.
You can also develop skills in areas like algorithms, data structures, artificial intelligence, cybersecurity, discrete algebra, and human-computer interaction.
Here's a breakdown of common computer science skills:
- Theory and mathematics of computation
- Problem analysis
- Programming languages, such as Java or Python
- Decision loops, variables, and how to write functions
- Technical writing
Soft skills include:
- Communication
- Time management
- Attention to detail
- Collaboration
Students also learn skills in areas like algorithms, data structures, artificial intelligence, cybersecurity, discrete algebra, and human-computer interaction.
A BA in computer science emphasizes the liberal arts, while a BS focuses on math, science, and technology. Either degree can be helpful, but a BS in computer science is more likely to open doors to specialized careers or advanced degrees.
To become proficient in computer science, you'll need to take courses like Computer Science I and II, Mathematical Methods for Computer Science, Calculus I and II, Data Structures, Advanced Programming, and Computational Structures.
For more insights, see: Bs Compsci
CS Program and Course Information
The University of London's online Computer Science degree is a comprehensive program that will equip you with the skills you need to thrive in the tech industry. You'll master sought-after programming, mathematical, and computing skills through practical project-based modules.
The program offers a range of specialisms, including Data Science, Web and Mobile Development, or Machine Learning and AI. You'll learn to use a range of programming languages, including Python and C++, which are in high demand in the industry.
Here are some of the courses you can expect to take in the program:
The program is designed to give you the skills and knowledge you need to succeed in a range of roles in the tech industry, which is expected to grow by 15% this decade.
CS Capstone Courses
CS capstone courses are a crucial part of a computer science program, providing students with the opportunity to apply their knowledge and skills to real-world problems.
CS capstone courses are designed to be hands-on and project-based, allowing students to work on a team, examine multiple design alternatives, and provide significant written, oral, and visual deliverables.
Some examples of CS capstone courses include CS 4284: Systems & Networking Capstone, CS 4274: Secure Computing Capstone, and CS 4414: Issues in Scientific Computing (when taught by CS faculty).
These courses are often taken in the final year of a CS program and are designed to help students develop skills in areas such as problem analysis, programming languages, and technical writing.
Here are some examples of CS capstone courses:
- CS 4284: Systems & Networking Capstone
- CS 4274: Secure Computing Capstone
- CS 4414: Issues in Scientific Computing (when taught by CS faculty)
- CS 4624: Multimedia, Hypertext & Information Access
- CS 4634: Design of Information
- CS 4644: Creative Computing Studio
- CS 4664: Data-Centric Computing Capstone
- CS 4704: Software Engineering Capstone
- CS 4784: Human-Computer Interaction Capstone
- CS 4884: Computational Biology & Bioinformatics Capstone
Individual CS 4994 Undergraduate Research projects can also be counted for capstone credit with prior approval from the Associate Department Head, provided they meet the characteristics of a capstone course.
External Electives
As you explore the various courses offered in the CS program, you may be wondering about the options for external electives. The University of London's online Computer Science degree allows you to choose a learning path to focus on IT career specialisms, such as Data Science, Web and Mobile Development, or Machine Learning and AI.
You can choose from a range of programming languages, including Python and C++, which are in high demand in the industry. In fact, the US is expected to see a 15% growth in the tech industry this decade.
The Virginia Tech CS department plans to offer various courses through Spring 2025, including CS 1064, Intro to Programming in Python, CS 2114, Software Design and Data Structures, and CS 4824, Machine Learning.
Computer science majors must take at least one course from an approved list of capstone courses, which includes CS 4624, Multimedia/Hypertext, CS 4664, Data-Centric Computing Capstone, and CS 4784, Human-Computer Interaction Capstone.
Here is a list of some of the capstone courses:
- CS 4624: Multimedia/Hypertext
- CS 4664: Data-Centric Computing Capstone
- CS 4784: Human-Computer Interaction Capstone
Frequently Asked Questions
What does BST mean coding?
BST stands for Binary Search Tree, a data structure where nodes' left children have lower values than their own, enabling efficient searching and sorting in code
Is a BST an ADT?
A binary search tree (BST) is an example of an abstract data type (ADT), which is a collection of operations with a specific meaning, rather than just a physical data structure. Learn more about the key differences between ADTs and data structures on Wikipedia.
What are the rules for BST?
In a Binary Search Tree (BST), elements are arranged in a specific order where left nodes have smaller values than their parent nodes, and right nodes have larger values. This rule ensures efficient searching and organization of data in the tree.
Sources
- https://btholt.github.io/complete-intro-to-computer-science/binary-search-tree/
- https://www.computerscience.org/degrees/bachelors/
- https://www.coursera.org/degrees/bachelor-of-science-computer-science-london
- https://bulletin.georgetown.edu/schools-programs/college/degree-programs/computer-science/
- https://website.cs.vt.edu/Undergraduate/courses.html
Featured Images: pexels.com