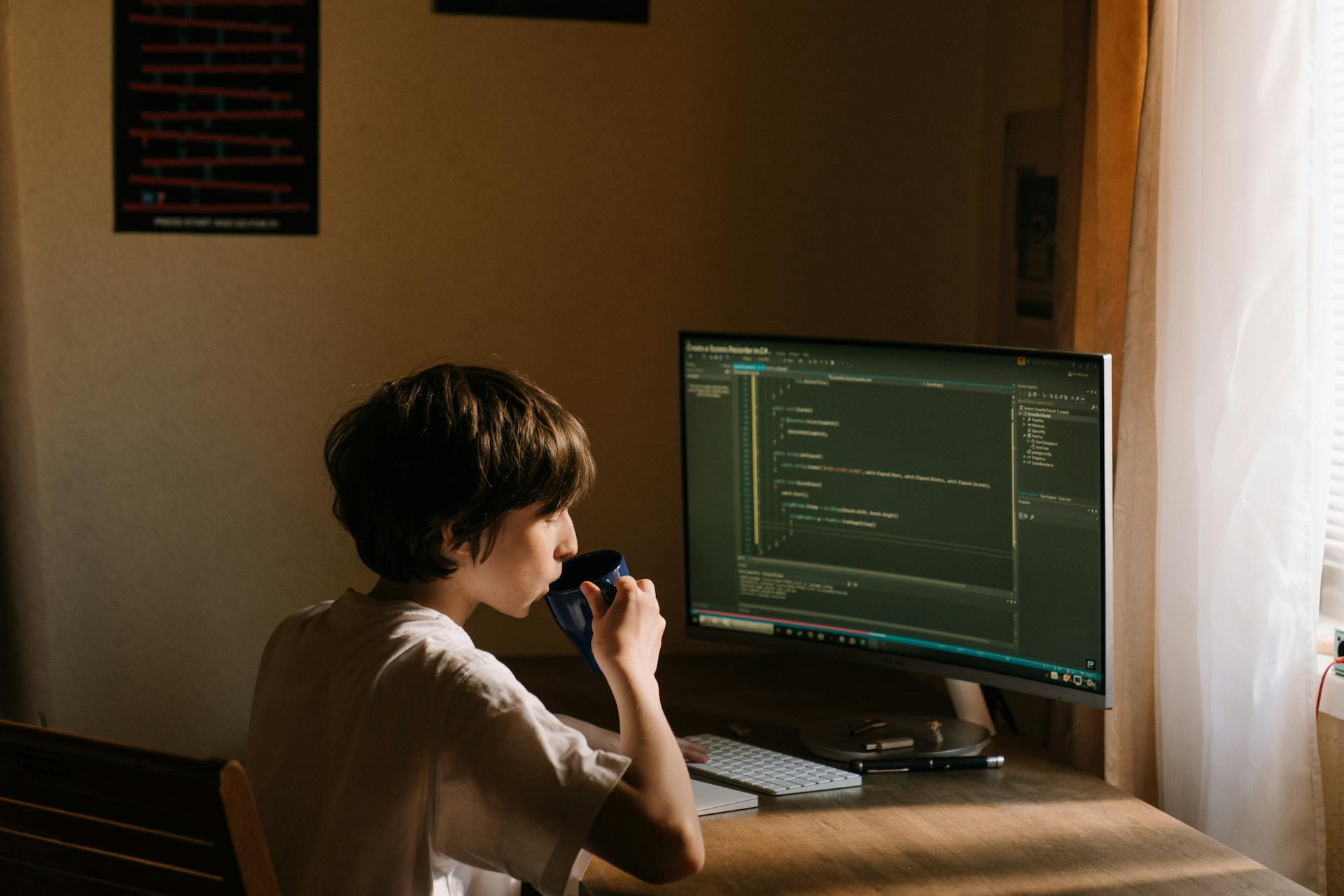
Welcome to Compsci 161! This course is a 10-week semester-long class that covers the basics of computer programming in Python. It's a great introduction to the world of computer science.
The course is designed for beginners, with no prior programming experience required. You'll learn the fundamentals of programming through hands-on exercises and projects.
You can expect to spend around 12-15 hours per week on coursework, including lectures, lab sessions, and homework assignments.
Course Information
Compsci 161 is an introduction to computer programming, specifically designed for students planning to major in computer science or related fields.
The course focuses on developing problem-solving strategies to design and implement algorithms in a modern programming language, currently Java.
You'll have the opportunity to apply problem-solving skills to real-world scenarios, which will help you become proficient in designing and implementing algorithms.
The course is ideal for students who want to gain a solid foundation in computer programming and prepare for more advanced courses in computer science.
By the end of the course, you'll be able to write efficient and effective algorithms in Java, which is a valuable skill in the field of computer science.
Intriguing read: Grokking Algorithms Second Edition
Submission and Grading
Make sure to upload your project directory to the LabB submission folder on hedwig by the end of the lab. Only one partner needs to upload the code, so coordinate with your partner to avoid duplication.
The lab is due at the start of class the morning after lab, so don't wait until the last minute to submit. You can find help on uploading to the correct folder in the previous lab.
This assignment will be graded on four criteria: drawing a house, modifying the Pyramid class, code formatting, and submitting a lab partner evaluation. The grading breakdown is as follows:
Remember that it's easy to get partial credit, so turn in whatever you have by the due date.
Submission
Make sure both your names are on both the classes you've modified, and upload the entire project directory to the LabB submission folder on hedwig.
Only one partner needs to upload the code, so be sure to coordinate with your partner if you're working together.
Make sure you upload your work to the correct folder, as stated in the lab instructions.
The lab is due at the start of class the morning after lab, so plan accordingly to meet the deadline.
For your interest: Comp Sci Lab
Grading
Grading is a crucial aspect of this assignment, and understanding how it will be evaluated can help you prepare and submit your work confidently.
The grading criteria are as follows: submitted a HouseDrawing class that draws a house accounts for 40% of the grade.
To ensure you get the most out of your submission, remember that partial credit is available, so don't hesitate to turn in whatever you have before the deadline.
The HouseDrawing class is the first major component of the grading criteria, and it's worth 40% of the total grade.
The second major component is a modified Pyramid class that draws a pyramid at any specified size, which accounts for 50% of the grade.
Code formatting is also crucial, and 5% of the grade is allocated for submitting code that is formatted correctly, including good indentation, names on the files, and reasonable comments.
To make formatting easier, you can select "Edit > Autolayout" from the BlueJ menu to automatically fix the indentation.
Lastly, submitting a lab partner evaluation is worth 5% of the grade.
Discover more: Compsci Major
Assignment Details
For this lab, you'll need to write programs that instruct a turtle on how to produce two different drawings. You can produce these programs in either order, but make sure you do them both!
You'll need to submit your solution, and then log onto Moodle to submit the Lab B Partner Evaluation.
Assignment Details
You'll need to write two different programs that instruct a turtle on how to produce two different drawings.
These programs can be written in any order, but make sure to complete both.
For the submission, you'll need to log onto Moodle and submit your solution.
Both partners need to submit the Lab B Partner Evaluation after submitting your solution.
Recommended read: Best Comp Sci Masters Programs
Necessary Files
To complete this assignment, you'll need to download a specific file. The LabB.zip file contains the necessary project files, including the Turtle class, which has already been written for you.
The Turtle class is a pre-made component that you'll be building upon. You'll also find a Pyramid class in the project, which is where you'll need to focus your efforts.
The project is contained within the LabB.zip file, so make sure to extract it once you've downloaded it.
Analysis and Optimization
To determine the efficiency of an algorithm, we need to analyze its running time. This is done by comparing the algorithm's running time to a known function, g(n), using the Big O notation. In problem set 1, we learned that f(n) is O(g(n)) if f(n) ≤ cg(n) for some constants c > 0 and n0 ≥ 0, and for all n > n0.
For example, if we have f(n) = n4 + 9n3 + 4n + 7 and g(n) = n4, we can show that f(n) is O(g(n)) by finding a value of c that makes cg(n) greater than or equal to f(n). A simple way to do this is to add the coefficients of f(n) to get c.
To rank functions in order from smallest to largest asymptotic running time, we can categorize them into constant, poly-log, polynomial, exponential, and worse. This makes it easier to sort the functions into their respective categories. In problem set 1, we saw that a string can be considered a min-heap if it is arranged in alphabetical order.
Intriguing read: Dining Philosophers Problem
Here's a rough guide to help you categorize functions:
In problem set 1, we also saw that an array can be considered a complete tree and a heap if the heap property applies. If we arrange the characters in the string "BEDPOST" in alphabetical order, we get a min-heap. Drawing this as a tree/heap is a useful way to visualize the structure of the string.
Frequently Asked Questions
Is cs 161 easy?
Yes, CS 161 is considered an accessible class with a gentle learning curve. Success in the class requires consistent effort to watch lectures and experiment with provided examples.
What is compsci 61A?
CS 61A focuses on abstraction, allowing programmers to think conceptually about problems rather than low-level computer hardware. It's a foundational course that sets the stage for more advanced software engineering.
Sources
- https://www.millersville.edu/computerscience/curriculum/courses/csci-161.php
- https://ics.uci.edu/~dillenco/compsci161/
- https://www.coursehero.com/sitemap/schools/234-University-of-California-Berkeley/courses/448448-COMPSCI161/
- https://faculty.washington.edu/joelross/courses/archive/f13/cs161/lab/b/
- https://www.studocu.com/row/document/hebei-university-of-science-and-technology/advanced-macroeconomics/exam-1-sample-solutions/43088557
Featured Images: pexels.com