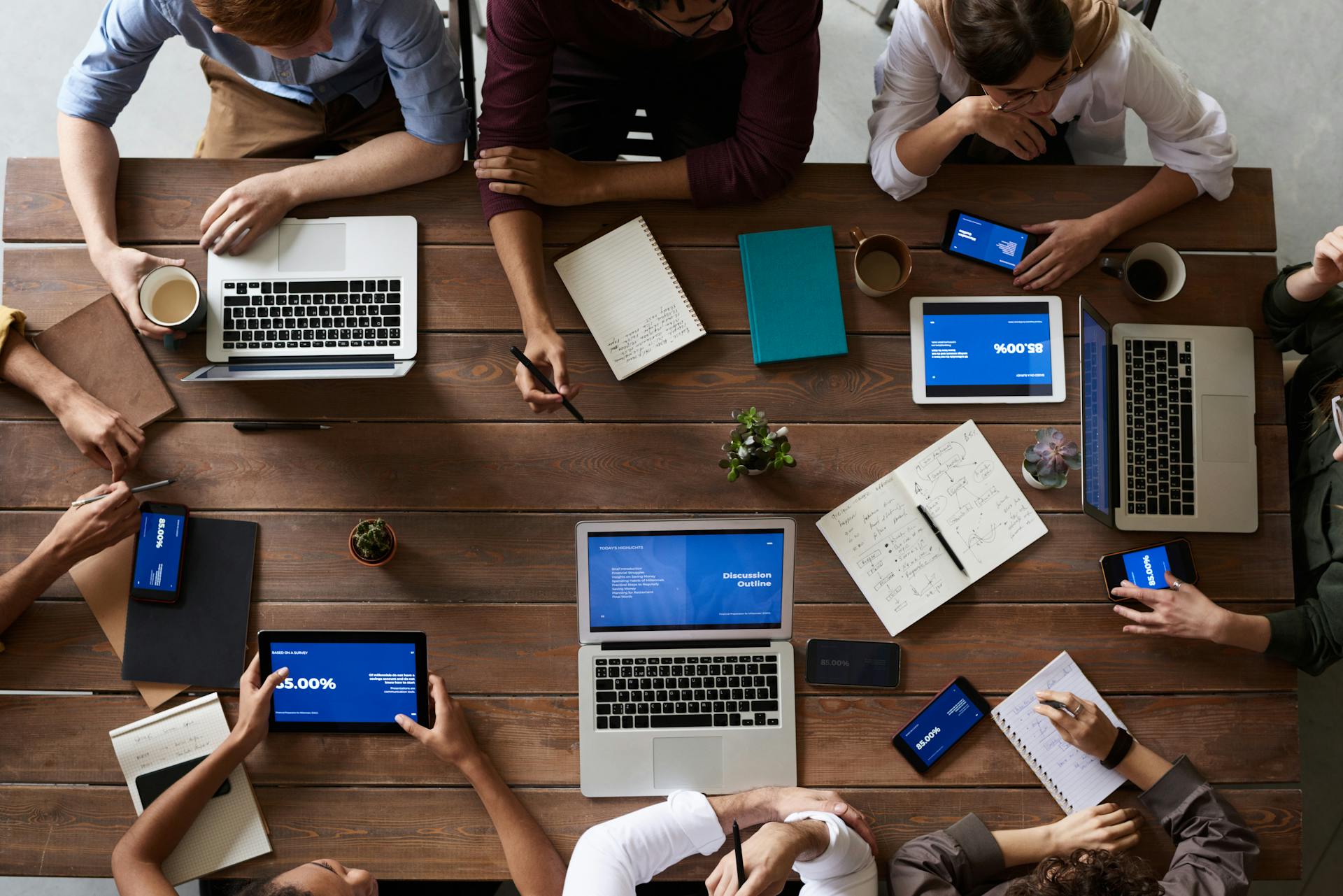
The Dining Philosophers Problem is a classic example of a synchronization issue that can occur in threaded programming. This problem was first introduced by Edsger Dijkstra in 1965 as a thought experiment to illustrate the challenges of coordinating multiple threads accessing shared resources.
In the Dining Philosophers Problem, five philosophers sit around a table with five plates of food and five chopsticks. Each philosopher needs two chopsticks to eat, but there are only five chopsticks available. This creates a situation where multiple threads (the philosophers) are competing for shared resources (the chopsticks).
The problem is that each philosopher will wait for the other philosopher to release their chopsticks before attempting to eat, but since all philosophers are waiting for each other, no one can eat. This creates a deadlock situation where all threads are blocked, and no progress is made.
In a real-world scenario, this problem can occur in a multi-threaded application where multiple threads are accessing shared resources, such as a database or a file. If not properly synchronized, this can lead to a deadlock situation where the application becomes unresponsive.
Problem Statement
Imagine five friends sitting at a table, each with their own plate and a fork between each plate. They're trying to eat spaghetti, but there's a catch - they need two forks to eat it, and they can only hold one fork at a time.
Each friend can only eat when they have both a left and right fork, and they'll put down both forks after they finish eating. The problem is, how can they design a system so that no one starves?
The spaghetti is eaten with two forks, and each philosopher can only eat when they have both a left and right fork. This means that two forks will only be available when their two nearest neighbors are thinking, not eating.
A philosopher can only eat their spaghetti when they have both a left and right fork, which they can only get when their neighbors are thinking.
Problem Overview
The dining philosophers problem is a classic example of a system state that can lead to no progress being possible. It's a challenging problem that requires careful consideration of sequence and access.
Imagine a group of philosophers sitting around a table with two forks each, trying to eat. The problem is designed to illustrate the challenges of avoiding deadlock, a system state where no progress is possible.
The problem can arise when each philosopher is instructed to behave in a certain way, such as thinking unless the left fork is available and then picking it up. This can lead to a situation where each philosopher holds the fork to their left, waiting for the other fork to be available.
Other types of sequence and access problems include resource starvation, mutual exclusion, and livelock. These problems can have significant consequences, such as system crashes or data loss.
Solutions Overview
To solve the dining philosophers problem, we need to understand that negating at least one of the four necessary conditions for a deadlock to occur is key.
A solution that negates mutual exclusion or non-preemption can give a valid solution, but most theoretical treatments assume those assumptions are non-negotiable.
Negating resource holding is a common approach to solving the problem, as it allows a philosopher to release a fork while waiting for the second.
Circular waiting is another condition that can be attacked, but often both resource holding and circular waiting are targeted in a solution.
A solution that negates both resource holding and circular waiting is considered a strong approach to avoiding deadlocks in the dining philosophers problem.
Dijkstra's Algorithm
Dijkstra's Algorithm is a problem-solving technique that can be used to find the shortest path between nodes in a graph.
It was developed by Edsger W. Dijkstra in 1959, a Dutch computer scientist who also worked on the dining philosophers problem.
The algorithm is particularly useful for solving problems like the dining philosophers problem, where multiple processes need to access shared resources in a coordinated manner.
Dijkstra's Algorithm works by assigning a distance value to each node, and then iteratively updating these values to find the shortest path.
Dijkstra's
Dijkstra's solution is a clever approach to the classic Dining Philosophers problem. It negates resource holding by requiring philosophers to pick up both forks or wait, never holding exactly one fork outside of a critical section.
To accomplish this, Dijkstra's solution uses one mutex, one semaphore per philosopher, and one state variable per philosopher. This setup makes the solution more complex than the resource hierarchy solution.
The use of a function called test() is a key aspect of Dijkstra's solution. This function is used in the take_forks() and put_forks() functions to make the solution deadlock-free.
Dijkstra's solution is a testament to the power of creative problem-solving. By using a combination of mutexes, semaphores, and state variables, Dijkstra was able to create a solution that is both efficient and deadlock-free.
Tanenbaum's changes to Dijkstra's solution are a notable improvement. The C++20 version of Dijkstra's solution with Tanenbaum's changes is a great example of how to implement this solution in practice.
Echo Lisp
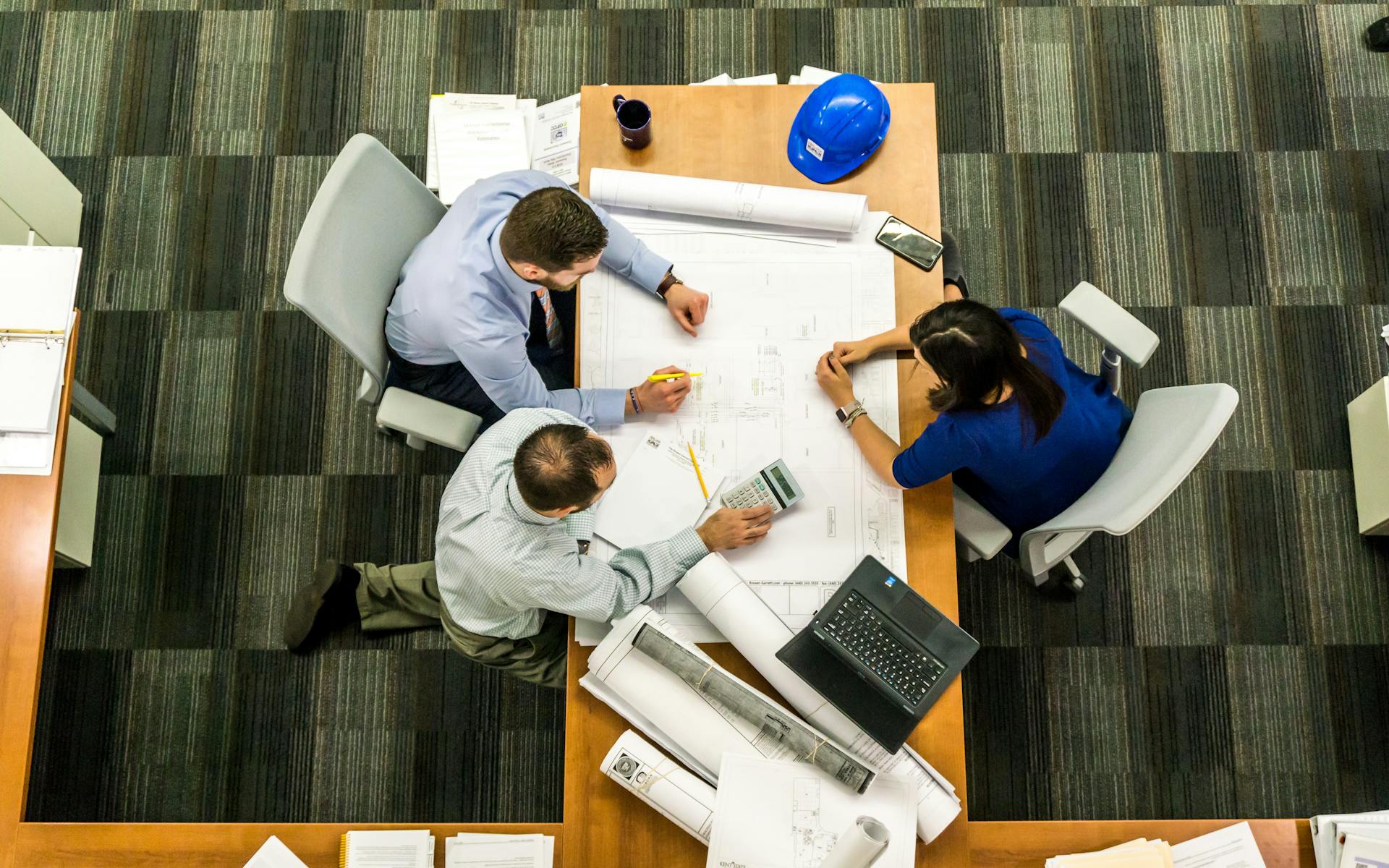
Echo Lisp is a programming language that uses a unique approach to synchronization, inspired by the classic dining philosophers problem. This problem is a great example of how to avoid deadlocks in concurrent programming.
The solution involves introducing a laquais who checks that no more than 4 philosophers are sitting at the same time, preventing deadlocks. This is a clever way to ensure that the program doesn't get stuck.
In Echo Lisp, synchronization occurs when the eat routine is called, which requires exclusive access to all separate forks. The resources are released when the routine terminates, ensuring that the program can continue running smoothly.
The example uses numbers to identify the philosophers, making it easy to vary the number of philosophers. This is a great feature for testing and experimenting with different scenarios.
Deadlocks are avoided by always getting locks on forks with lower numbers first, which is a simple yet effective solution. This approach ensures that the program runs efficiently and avoids getting stuck.
Resource Hierarchy
The resource hierarchy solution is a clever way to prevent deadlocks in the dining philosophers problem. This solution assigns a partial order to the resources, ensuring that all resources are requested in order and no two unrelated resources are used by a single unit of work at the same time.
In this solution, the resources (forks) are numbered 1 through 5, and each philosopher will always pick up the lower-numbered fork first, then the higher-numbered fork. For example, if four of the five philosophers simultaneously pick up their lower-numbered forks, only the highest-numbered fork will remain on the table, so the fifth philosopher will not be able to pick up any fork.
The resource hierarchy solution avoids deadlocks, but it's not always practical, especially when the list of required resources is not completely known in advance. For instance, if a unit of work holds resources 3 and 5 and then determines it needs resource 2, it must release 5, then 3 before acquiring 2, and then it must re-acquire 3 and 5 in that order.
The resource hierarchy solution is not fair, as it may prevent some philosophers from eating if they are slow to take a fork. To achieve fairness, a solution must guarantee that each philosopher will eventually eat, no matter how slowly that philosopher moves relative to the others.
Here's a summary of the resource hierarchy solution:
The resource hierarchy solution is a good starting point for understanding how to prevent deadlocks in the dining philosophers problem. However, it's essential to consider other solutions, such as using an array of mutexes or imposing a linear ordering on the semaphores, to achieve fairness and efficiency.
Fair
The dining philosophers problem is all about fairness. Imposing a linear ordering on the semaphores can help prevent deadlock, but it's not a perfect solution. By requiring each thread to wait on semaphores in a specific order, we can avoid the circular wait that causes deadlock.
For example, thread 4 must wait on semaphore 0 before semaphore 4, which prevents it from blocking other threads. This is shown in Table 8.3, where thread 4 is blocked from the start, allowing thread 3 to proceed.
The linear ordering also affects the last thread, which must wait on semaphore 0 before semaphore N-1. This is done by adding a single if statement, as shown in Code Listing 8.28. The thread identifier and semaphores are used to enforce this order.
In the example, thread 4 is blocked from the start because it must wait on semaphore 0, which is already decremented by thread 0. This prevents the circular wait that would cause deadlock.
Table Diner Capacity
The dining philosophers problem can be a real challenge, but one solution is to limit the number of diners at the table. By allowing a maximum of n-1 philosophers to sit down at any time, the last philosopher must wait for someone to finish dining before they can "sit down" and request access to any fork.
This approach negates the circular wait, guaranteeing at least one philosopher may always acquire both forks, allowing the system to make progress. It's a clever solution that ensures the table can always accommodate one more diner.
To implement this solution, a semaphore is used to limit the number of concurrent accesses. This requires that one of the seats at the table must always remain unoccupied. This clever trick prevents the circular wait and ensures the system can always make progress.
Table Diner Capacity Limit
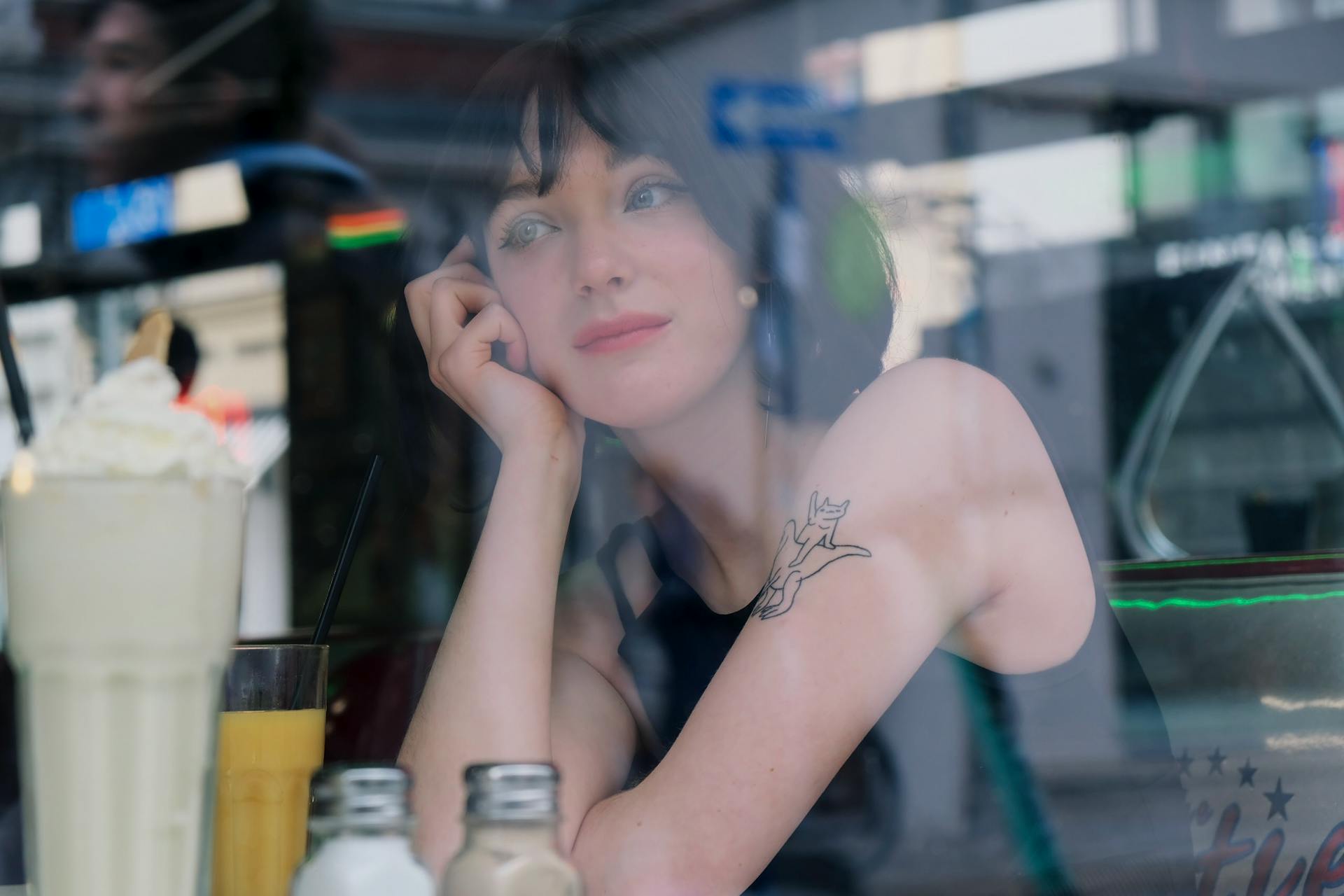
Limiting the number of diners at a table is a clever solution to the dining philosophers problem. By allowing a maximum of n-1 philosophers to sit down at any time, the last philosopher must wait for someone to finish dining before they can "sit down" and request access to any fork.
This approach negates the circular wait, guaranteeing at least one philosopher may always acquire both forks, allowing the system to make progress.
To implement this solution, a multiplexing semaphore can be used to limit the number of concurrent accesses. This would require one of the seats at the table to always remain unoccupied.
A single additional semaphore (can_sit) is created and initialized to N-1 for N semaphores, preventing all N semaphores from being decremented by the first call to sem_wait(). This ensures that there must be at least one semaphore that can be decremented by the second call, guaranteeing one thread enters the critical section.
Here's a breakdown of how this approach works:
Simple with Statistics
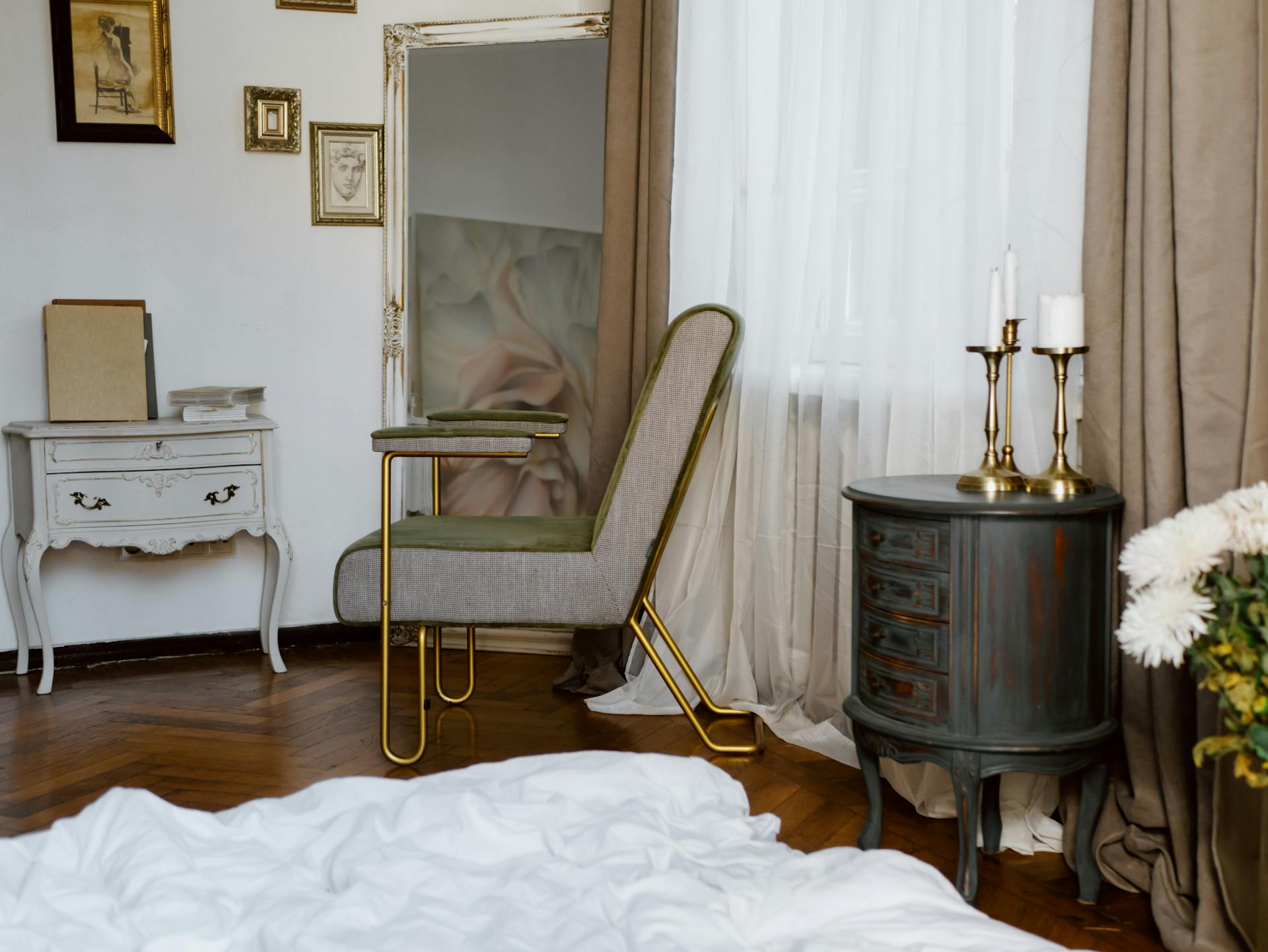
The Simple with Statistics solution is a great way to approach the dining philosophers problem, and it's actually quite simple. This solution allows a philosopher to take only two forks at once, or none at all, which eliminates the possibility of deadlock.
By making each fork into a channel, and guarding the eating process by two forks, this solution achieves several benefits. No deadlock occurs, and no "livelock" (trying to pick up and put down forks forever) happens either.
The mean time of waiting while hungry is not bounded and grows very slowly (logarithmically) with time. This means that the longer you wait, the longer you'll have to wait for your turn to eat.
Here's a breakdown of the statistics printed by this solution:
This solution supports only a fixed set of philosophers, since all channels are declared statically. More philosophers need more lines of code, which can be a limitation in certain situations.
Chandy/Misra Algorithm
The Chandy/Misra algorithm is a solution to the dining philosophers problem that allows for arbitrary agents to contend for an arbitrary number of resources. It's a completely distributed solution that requires no central authority after initialization.
This algorithm was proposed in 1984 by K. Mani Chandy and J. Misra. It's a bit more complex than Dijkstra's solution, but it's more flexible and can handle a large degree of concurrency.
Here's a step-by-step breakdown of how the Chandy/Misra algorithm works:
- For every pair of philosophers contending for a resource, create a fork and give it to the philosopher with the lower ID.
- When a philosopher wants to use a set of resources, they must obtain the forks from their contending neighbors by sending request messages.
- When a philosopher with a fork receives a request message, they keep the fork if it's clean, but give it up when it's dirty.
- After a philosopher is done eating, all their forks become dirty. If another philosopher had previously requested one of the forks, the philosopher that has just finished eating cleans the fork and sends it.
This solution also solves the starvation problem by giving preference to the most "starved" processes. The clean/dirty labels act as a way of giving preference to the most starved processes, and a disadvantage to processes that have just "eaten".
The Chandy/Misra algorithm guarantees that deadlock cannot occur by negating circular waiting. However, if the system is initialized to a perfectly symmetric state, like all philosophers holding their left side forks, then the graph is cyclic at the outset, and their solution cannot prevent a deadlock.
Mutex Implementation
Using an array of mutexes can prevent deadlocking in the Dining Philosophers Problem. This approach ensures that the philosopher's forks are seized as an atomic operation, preventing deadlocks.
A more direct approach to modeling forks is to use sync.Mutex objects, as they are specifically designed for mutually exclusive use. This makes it clear and efficient to implement fork use in the Dining Philosophers Problem.
In some cases, using channels for synchronization can be sufficient, but the sync library has additional functions that can make it easier to model common operations, such as waiting for concurrent tasks to finish. This is where sync.WaitGroup comes in, which is directly implemented for this purpose.
Array of Mutexes
Modeling forks with an array of mutexes is a viable approach to avoid deadlocks in the Dining Philosophers problem. This method uses an array object to prevent the philosopher from seizing his forks from the subset as an atomic operation.
Using an array of mutexes allows for efficient modeling of forks, ensuring that a philosopher can't grab his forks simultaneously. The array object acts as a single unit, preventing concurrent access to the same fork.
The atomic operation of the array object is key to preventing deadlocks, as it ensures that the philosopher can't access multiple forks at the same time. This approach is particularly useful in the Dining Philosophers problem, where fork use is mutually exclusive.
In this implementation, the array of mutexes is used to synchronize access to the forks, making it easier to manage concurrent access. By using an array of mutexes, you can avoid the complexities of synchronization and focus on the logic of the problem.
Rust
Rust is a great language to implement mutexes in, as we can use Dijkstra's solution to prevent deadlocks in the Dining Philosophers Problem.
This solution involves making a single diner "left-handed", meaning all diners except one pick up the chopstick to their left and then the chopstick to their right.
The remaining diner performs this in reverse, which prevents the deadlock that can occur when all diners are left-handed.
This approach is effective in Rust, where we can easily implement the solution to avoid deadlocks.
By using this approach, we can ensure that our mutex implementation in Rust is deadlock-free and efficient.
Frequently Asked Questions
What is the solution of the Dining Philosophers problem?
The solution to the Dining Philosophers problem is to limit the number of philosophers competing for chopsticks, ensuring at least one can acquire them. This approach prevents the deadlock scenario that occurs when all philosophers wait for chopsticks simultaneously.
What is the Dining Philosophers problem in go?
The Dining Philosophers problem in Go is a synchronization challenge where multiple philosophers simultaneously try to pick up forks to eat, demonstrating the need for coordination and mutual exclusion in concurrent programming. This problem is often used to illustrate the dangers of deadlocks and the importance of proper synchronization techniques.
Why might a group of dining philosophers starve?
A group of philosophers may starve if they all follow the same simultaneous action of grabbing the left chopstick and waiting for the right one, creating a deadlock. This scenario illustrates the classic problem of the Dining Philosophers, a thought experiment in computer science and philosophy.
What is the deadlock in the dining philosophers problem?
A deadlock occurs when all philosophers are holding their forks to the left, causing them to wait indefinitely for the other fork to become available. This is a classic example of a sequence and access problem in computer science.
How do we describe the starvation situation for the dining philosophers problem?
The dining philosophers problem leads to starvation due to a circular wait, where each philosopher waits for another to release a fork. This deadlock situation causes the philosophers to starve, unable to eat.
Sources
- https://en.wikipedia.org/wiki/Dining_philosophers_problem
- https://rosettacode.org/wiki/Dining_philosophers
- https://w3.cs.jmu.edu/kirkpams/OpenCSF/Books/csf/html/DiningPhil.html
- http://workcraft.org/tutorial/model/dining_philosophers/start
- https://www.adit.io/posts/2013-05-11-The-Dining-Philosophers-Problem-With-Ron-Swanson.html
Featured Images: pexels.com