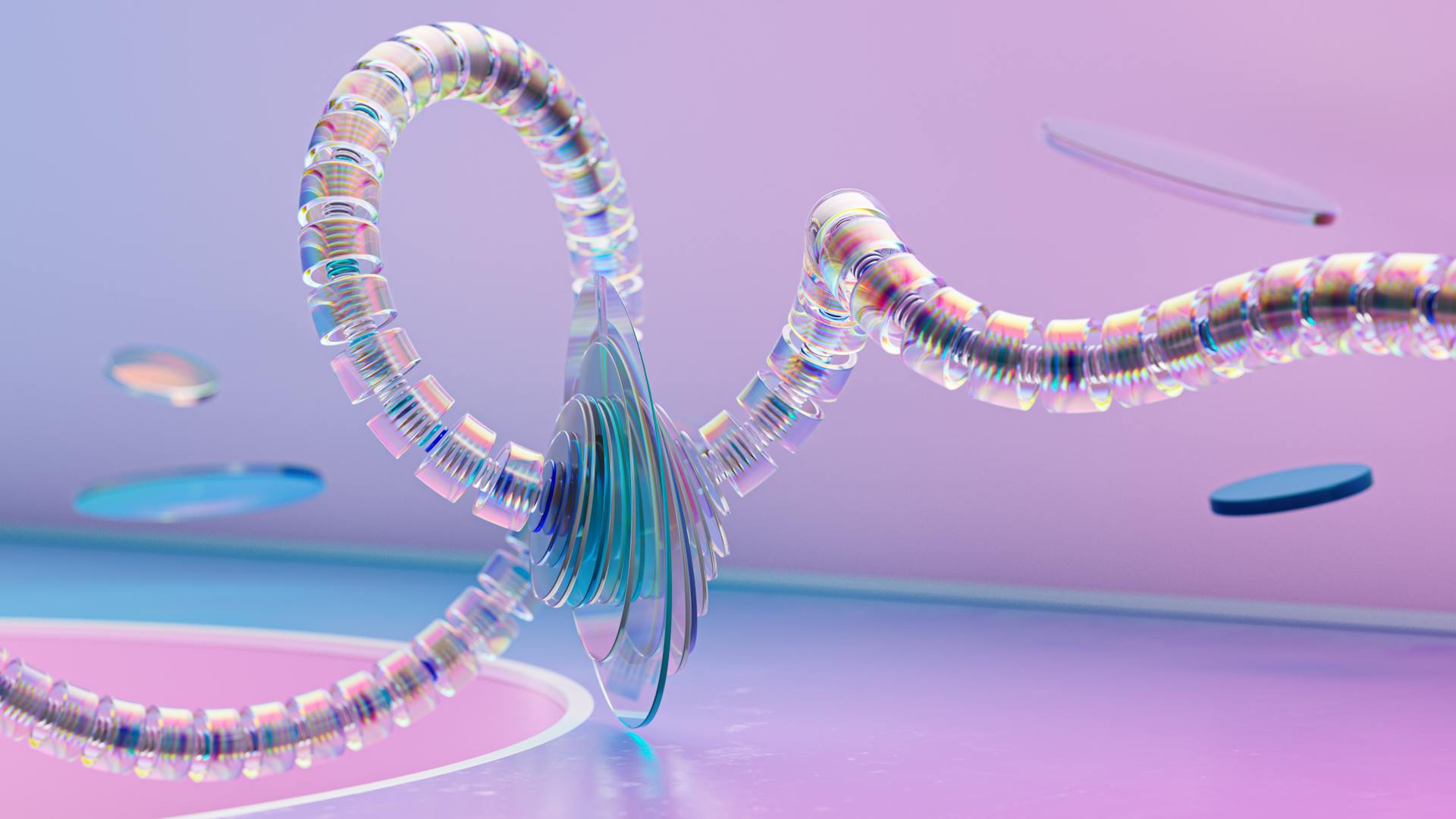
The bounded buffer problem is a classic issue in operating systems that can be frustrating to deal with. It occurs when a producer thread tries to add data to a buffer that is already full, causing the system to hang or crash.
Producers and consumers are two types of threads involved in this problem. Producers add data to the buffer, while consumers remove data from it. The problem arises when the producer thread tries to add data to a buffer that is already full.
In a real-world scenario, this could happen in a printer queue where the printer is trying to print a document, but there's no paper left in the tray. The printer is the producer, and the paper tray is the buffer.
To avoid this problem, a synchronization mechanism is needed to ensure that only one producer can add data to the buffer at a time. This can be achieved using locks or semaphores.
Problem Description
The Bounded Buffer Problem is a classic synchronization challenge that arises when multiple threads access a shared resource. It involves a producer that generates data and a consumer that processes it.
The producer and consumer must coordinate their activities to prevent data overwrite and ensure data isn't read prematurely. This is crucial in maintaining data integrity during buffer access.
A finite-sized array or storage space, known as the buffer, holds the items generated by the producer. The buffer has a limited capacity, and the producer must wait for the consumer to consume an item if the buffer is full.
If the buffer is empty, the consumer must wait for the producer to fill the buffer with at least one item. This synchronization is essential to prevent data loss or corruption.
The buffer's capacity is a critical factor in the Bounded Buffer Problem. To manage this capacity efficiently, we employ three semaphores: empty, full, and buffer manipulation.
Here's a breakdown of these semaphores:
By using these semaphores, we can synchronize the producer and consumer threads, ensuring that the buffer's capacity is respected and data integrity is maintained.
Solution Approach
The solution to the bounded buffer problem lies in using a combination of semaphores and a mutex. A mutex, or mutual exclusion, is used to create a critical section where only one thread can access the buffer at a time.
Here's a step-by-step approach to solving the problem:
1. The mutex ensures that the producer and consumer threads have mutually exclusive access to the buffer.
2. If the consumer tries to retrieve data when the buffer is empty, it will go to the waiting stage because the full semaphore won't allow it to decrease its value.
3. The producer thread checks if the slots are empty, and if so, calls the wait() function, decreasing the value of the empty semaphore by 1.
4. The producer then accesses the critical section, ensuring that it's the only one accessing the buffer.
5. After increasing the value of the full semaphore by calling the signal() function, the producer allows the consumer to access the buffer.
6. If the slots get filled, the empty semaphore becomes 0, and the producer goes to the waiting stage.
A key aspect of this solution is the use of semaphores to control access to the buffer. Two semaphores are used: one to count the filled locations in the buffer and another to count the empty locations.
Concurrency Issues
Concurrency issues are a major concern when dealing with the bounded buffer problem. They can lead to race conditions, where multiple processes access and manipulate shared data concurrently, resulting in unpredictable and incorrect outcomes.
Concurrency is when an application juggles multiple tasks at once, either with threads or separate processes. This can be useful for handling multiple requests concurrently, like in a web server.
Parallelism, on the other hand, is perfect for crunching big numbers, making computations faster and more efficient. It's like having a heavy-duty multi-threaded friend.
To solve concurrency issues, we need synchronization methods to keep threads in line and prevent data corruption. This is where synchronization primitives like mutexes and semaphores come in.
Here are the key differences between mutexes and semaphores:
In the context of the bounded buffer problem, we use two counting semaphores (full and empty) and a mutex to ensure safe and efficient access to the shared buffer. This helps prevent race conditions and ensures the integrity of the shared data.
Real World Example
In a restaurant scenario, the kitchen staff are like producers, preparing dishes that customers have ordered. They add these orders to a shared buffer, the restaurant's order queue or ticket system.
The shared buffer is a critical component, as it holds the orders until the waitstaff or servers can retrieve them and deliver them to the respective tables.
In this scenario, the waitstaff or servers act as consumers, retrieving orders from the shared buffer and delivering them to the customers.
This is a classic example of the producer-consumer problem, where multiple producers are adding items to a shared buffer, and multiple consumers are retrieving items from the buffer.
Here are some key takeaways from this example:
- Producers: Kitchen staff, adding orders to the shared buffer.
- Shared Buffer: Restaurant's order queue or ticket system.
- Consumers: Waitstaff or servers, retrieving orders from the shared buffer.
This example highlights the importance of managing access to shared resources, ensuring that producers don't overwhelm the buffer and that consumers can retrieve items efficiently.
Analysis and Explanation
The bounded buffer problem is a classic issue that requires careful management of shared resources. In a scenario where multiple producers and consumers are accessing a shared buffer, synchronization is crucial to prevent race conditions.
To ensure that producers deposit data and consumers retrieve it correctly, we need to implement a semaphore to block producers when the buffer is full. This semaphore should have an initial value equal to the buffer size, as mentioned in Example 1. This is because the buffer is empty initially, and we want to allow that number of producers to pass through before blocking them.
Producers must wait until the buffer is not full, deposit their data, and then notify consumers that the buffer is not empty. Similarly, consumers must wait until the buffer is not empty, retrieve a data item, and then notify producers that the buffer is not full.
Here's a step-by-step breakdown of the process:
- Producers wait on the empty semaphore before adding an item to the buffer.
- Consumers wait on the full semaphore before retrieving an item from the buffer.
- Both producers and consumers lock the mutex to enter the critical section and access the buffer.
- Producers add an item to the buffer and increment the full semaphore.
- Consumers remove an item from the buffer and increment the empty semaphore.
This ensures that producers and consumers access the buffer safely and efficiently.
Concurrency Techniques
Concurrency Techniques are essential for handling multiple tasks simultaneously, ensuring smooth operation and preventing data corruption. This is especially true for the bounded buffer problem, where multiple threads need to access shared resources.
Concurrency is not the same as parallelism. Concurrency is about juggling multiple tasks at once, while parallelism is about dividing complex tasks among multiple threads or processing units. This distinction is crucial when deciding which technique to use.
In the context of the bounded buffer problem, concurrency is used to handle multiple producer and consumer threads. These threads need to access shared resources, such as the buffer, without causing conflicts.
Mutexes and semaphores are two synchronization primitives used to coordinate access to shared resources. Mutexes provide mutual exclusion, allowing only one thread to access a shared resource at a time. Semaphores, on the other hand, control access to a shared resource by allowing a specific number of threads to access it simultaneously.
Here are some key differences between mutexes and semaphores:
In the Lamport solution, busy waiting is used in the thread instead of waiting in the scheduler. However, this solution neglects the impact of scheduler thread switch at inconvenient times. Atomic read-modify-write operations can solve this problem.
Sources
- https://en.wikipedia.org/wiki/Producer%E2%80%93consumer_problem
- https://pages.mtu.edu/~shene/NSF-3/e-Book/SEMA/TM-example-buffer.html
- https://blog.jyotiprakash.org/bounded-buffer-problem
- https://medium.com/@ashaymotiwala/tackling-the-bounded-buffer-problem-aka-producer-consumer-problem-using-semaphores-42b4b22f2448
- https://favtutor.com/blogs/producer-consumer-problem
Featured Images: pexels.com