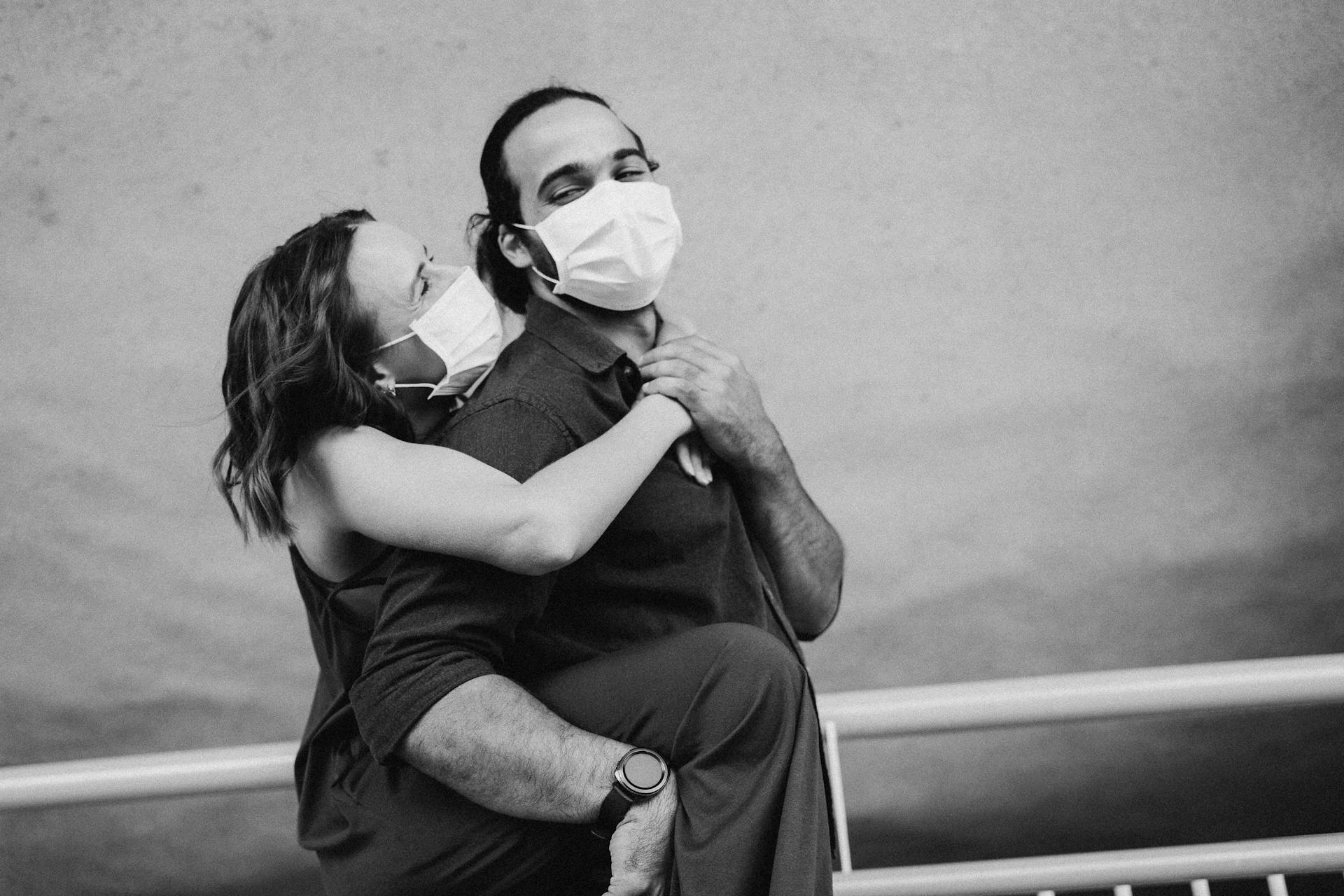
Hugging Face Datasets is a powerful tool for creating and managing datasets. It allows you to easily load, manipulate, and transform datasets for use in machine learning models.
With Hugging Face Datasets, you can create features for datasets using the `dataset.map()` method. This method applies a transformation function to each element in the dataset.
To create a feature, you'll need to define a transformation function that takes an element from the dataset as input and returns the desired feature as output. For example, if you're working with a text dataset, your transformation function might extract the text from a JSON object.
Expand your knowledge: Long Text Summarization Huggingface
Getting Started
To start creating a feature for a dataset on Hugging Face, you'll need to install the Transformers library and the datasets library, which can be done using pip with the command `pip install transformers datasets`.
Hugging Face provides a simple way to load datasets using the `load_dataset` function, which allows you to load a dataset from a local file or a remote repository.
On a similar theme: Huggingface Transformers Model Loading Slow
The `load_dataset` function takes the dataset name as an argument, and you can specify the version of the dataset you want to load.
You can also use the `cache` argument to cache the dataset locally, which can be useful for large datasets.
To create a feature for a dataset, you'll need to use the `create_feature` function, which takes the dataset and a list of feature names as arguments.
The `create_feature` function will automatically create the necessary columns for the features you specify, and you can use the `set_format` function to specify the data type and format for each feature.
You can also use the `add_column` function to add a new column to the dataset, which can be useful for creating a new feature.
By following these steps, you can easily create a feature for a dataset on Hugging Face and start working with your data.
For more insights, see: How to Use Huggingface Models
Create a Dataset
Creating a dataset with š¤ Datasets is a breeze, and it comes with a host of advantages, including fast loading and processing, streaming enormous datasets, and memory-mapping.
You can easily create a dataset using low-code approaches, which reduces the time it takes to start training a model. In many cases, it's as easy as dragging and dropping your data files into a dataset repository on the Hub.
There are two main ways to create a dataset: Folder-based builders and from_ methods. Folder-based builders are great for quickly creating an image or audio dataset with several thousand examples.
Here are the two Folder-based builders:
The from_ methods are used for creating datasets from local files.
Dataset Configuration
Creating a dataset with Hugging Face's š¤ Datasets library is a great way to work with your own data. It confers all the advantages of the library to your dataset, including fast loading and processing, stream enormous datasets, and more.
You can easily create a dataset using š¤ Datasets low-code approaches, which reduces the time it takes to start training a model. This is especially useful when you need to create a dataset quickly.
There are two main ways to create a dataset: Folder-based builders for quickly creating an image or audio dataset, and from_ methods for creating datasets from local files.
A configuration is a specific version or subset of your dataset, like having different flavors of the same ice cream. Each configuration can have its own set of parameters, like language, size, or specific features.
You can create multiple configurations for your dataset using Hugging Face datasets BuilderConfig, but for this example, we'll create only one configuration. To create a custom configuration class, you can inherit from datasets.BuilderConfig, as shown in the code example for the rice crop diseases dataset.
Here are some key points to consider when creating a dataset configuration:
- Each configuration can have its own set of parameters.
- Configurations make your dataset more flexible and easier to use in various scenarios.
- You can create multiple configurations using Hugging Face datasets BuilderConfig.
Working with Data
You can create a dataset from data in Python dictionaries using the from_ methods. The from_generator() method is the most memory-efficient way to create a dataset from a generator, especially useful for large datasets that may not fit in memory.
The from_dict() method is a straightforward way to create a dataset from a dictionary, but it's not suitable for image or audio datasets. To create an image or audio dataset, chain the cast_column() method with from_dict() and specify the column and feature type.
To generate samples, you'll need to transform your raw data into a format that can be easily processed by ML models. This involves tasks like cleaning the data, handling missing values, and splitting the data into appropriate chunks. You might also need to balance your dataset if you're dealing with classification problems.
From Python Dictionaries
You can create a dataset from data in Python dictionaries using the from_ methods in the datasets library. The from_generator() method is the most memory-efficient way to create a dataset from a generator, which is especially useful when working with large datasets that may not fit in memory.
One way to create a generator is by using a function that yields data, like this: def gen(): yield {"pokemon": "bulbasaur", "type": "grass"}; yield {"pokemon": "squirtle", "type": "water"}. This allows you to create a dataset from a generator-based IterableDataset.
For another approach, see: Create with Confidence Using Generative Ai
Alternatively, you can use the from_dict() method to create a dataset from a dictionary, like this: ds = Dataset.from_dict({"pokemon": ["bulbasaur", "squirtle"], "type": ["grass", "water"]}). This is a straightforward way to create a dataset from a dictionary, but it's worth noting that to create an image or audio dataset, you'll need to chain the cast_column() method with from_dict() and specify the column and feature type.
Here are the two main methods for creating a dataset from a dictionary:
- from_generator(): most memory-efficient way to create a dataset from a generator
- from_dict(): straightforward way to create a dataset from a dictionary
To create an image or audio dataset, use the cast_column() method with from_dict() and specify the column and feature type. For example, to create an audio dataset, you would use: audio_dataset = Dataset.from_dict({"audio": ["path/to/audio_1", ..., "path/to/audio_n"]}).cast_column("audio", Audio()).
If this caught your attention, see: How to Use Huggingface Models in Python
Generating Samples
Generating samples is a crucial step in building a dataset for machine learning models. It involves transforming raw data into a format that's easily processed by these models.
Cleaning the data is a vital part of this process. Handling missing values and splitting the data into chunks are also essential tasks. Balancing the dataset is necessary for classification problems.
Check this out: Ollama Huggingface Models
The DatasetBuilder._generate_examples method reads and parses data files using the file path provided by gen_kwargs in _split_generator(). It loads the data files and extracts the columns.
This function yields a tuple of id and an example of the dataset. It iterates through each image in the directories, assigning labels according to their respective directory name. The function finally yields the label and the image as a PIL image.
Dataset Management
Creating a dataset with š¤ Datasets confers all the advantages of the library to your dataset, including fast loading and processing, stream enormous datasets, memory-mapping, and more.
You can easily and rapidly create a dataset with š¤ Datasets low-code approaches, reducing the time it takes to start training a model. This can be as easy as dragging and dropping your data files into a dataset repository on the Hub.
š¤ Datasets provides two main features: documentation and dataset sharing on the Hub.
The library is designed to let the community easily add and share new datasets.
š¤ Datasets originated from a fork of the awesome TensorFlow Datasets and the HuggingFace team wants to thank the TensorFlow Datasets team for building this amazing library.
Here are the main ways to create a dataset with š¤ Datasets:
- Folder-based builders for quickly creating an image or audio dataset
- from_ methods for creating datasets from local files
To add a new dataset to the Hub, you can follow a detailed step-by-step guide, which includes how to upload a dataset using your web browser or Python, and also how to upload it using Git.
You might enjoy: Hugging Face Upload Model
Frequently Asked Questions
What is a feature of a dataset?
A feature of a dataset is a measurable property that can be used to describe or predict something, such as a variable or attribute. Understanding features is key to building accurate models in machine learning and statistics.
Sources
- https://huggingface.co/docs/datasets/en/create_dataset
- https://github.com/huggingface/datasets
- https://medium.com/@netrajpatil12mati/how-to-create-a-dataset-loading-script-using-hugging-face-datasets-52c6834e89a0
- https://blog.min.io/integrating-minio-hugging-face-datasets/
- https://machinelearningknowledge.ai/introduction-tutorial-to-hugging-face-datasets-library/
Featured Images: pexels.com