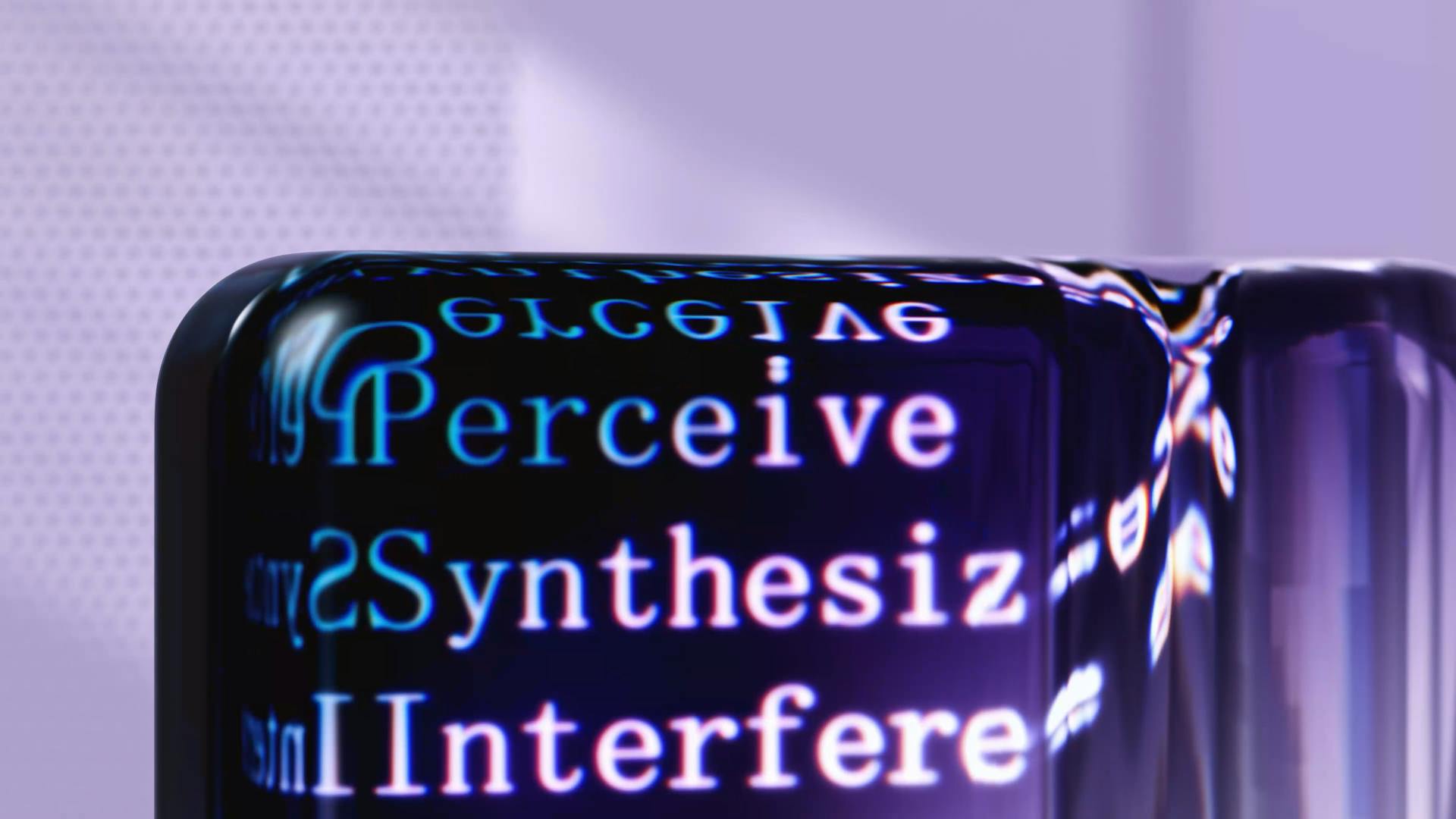
Fine tuning Llama Hugging Face involves a multi-step process. First, you'll need to install the necessary libraries, including Transformers and Hugging Face's library.
To fine tune Llama Hugging Face, start by loading a pre-trained model. This can be done using the AutoModelForSequenceClassification class from the Transformers library, which loads the pre-trained model and its configuration.
For example, you can load the Llama model using the following code: `model = AutoModelForSequenceClassification.from_pretrained('facebook/llama-13b')`. This loads the pre-trained Llama model and its configuration.
Expand your knowledge: Is Huggingface Transformers Model Good
Challenges with LLaMa
Fine-tuning a LLaMa 70B model can be a daunting task, but understanding the challenges that come with it can help you prepare and overcome them. One of the main challenges is the massive amount of CPU RAM required to load the pre-trained model, which can lead to processes being terminated.
The LLaMa 70B model requires 70*4*8 GB ~ 2TB of CPU RAM, which is a staggering amount of memory. To put this into perspective, a typical laptop might have 8-16 GB of RAM, so trying to load the entire model into memory is a recipe for disaster.
A unique perspective: How to Load a Model in Mixed Precision in Huggingface
Saving intermediate checkpoints using FULL_STATE_DICT with CPU offloading on rank 0 is another challenge that can result in NCCL Timeout errors due to indefinite hanging during broadcasting. This can lead to a lot of wasted time and frustration.
To give you a better idea of the challenges you might face, here are the three main challenges mentioned in the article:
- FSDP wraps the model after loading the pre-trained model, requiring massive amounts of CPU RAM.
- Saving entire intermediate checkpoints using FULL_STATE_DICT with CPU offloading on rank 0 can result in NCCL Timeout errors.
- Improving speed and reducing VRAM usage is crucial to train faster and save compute costs.
Pre-processing and Configuration
To pre-process our dataset, we'll use a function to format our prompts with hashtags to delimit each part. This will help us create a uniform format for our prompts.
We'll then use our model tokenizer to process these prompts into tokenized ones. This step is crucial to ensure our dataset is suitable for fine-tuning the language model.
To create input sequences of uniform length, we'll use functions to process our dataset. This maximizes efficiency and minimizes computational overhead.
The goal is to create input sequences that don't exceed the model's maximum token limit. This ensures our dataset is compatible with the model.
Take a look at this: How to Use Huggingface Models
We'll define the base model for fine-tuning as NousResearch/Llama-2-7b-chat-hf. This will be the foundation for our fine-tuning process.
The dataset we'll use is mlabonne/guanaco-llama2-1k. This dataset will be used to fine-tune the base model.
We'll provide a name for the new model, which will be created after fine-tuning. This name will help us identify the new model and distinguish it from the base model.
A different take: Fine-tuning Huggingface Model with Custom Dataset
LLaMA Overview and Key Concepts
LLaMA is a large language model that can be fine-tuned for specific tasks or domains. Fine-tuning involves adapting the pre-trained model to perform better on new data.
The fine-tuning process adjusts the weights of LLaMA's neural network, enabling it to make better predictions or generate more accurate responses. This is achieved by training the model on a new dataset that is more focused on the desired task or domain.
Fine-tuning LLaMA is a key concept in LLM fine tuning, which involves adapting pre-trained models to perform specific tasks or understand particular domains better.
Expand your knowledge: Pre Trained vs Fine Tune
LLaMA 2 Overview
LLaMA 2 is an open source large language model (LLM) introduced by Meta in 2023, offering a range of model variants with varying capacities. It's part of the LLaMA family, which includes models with 7 billion to 70 billion parameters.
The number of parameters determines the capacity to learn from data and generate responses, with more parameters generally resulting in more nuanced and complex capabilities. LLaMA 2 has been trained on an extensive dataset of 2 trillion tokens, allowing it to consider context lengths up to 4,096 tokens.
This is double the context length of its predecessor, LLaMA 1. LLaMA 2 also features models specifically fine-tuned for certain applications, such as LLaMA Chat and Code LLaMA.
Here are some key applications and their characteristics:
- LLaMA Chat: optimized for dialogue use cases and trained on over 1 million human annotations to enhance conversational abilities
- Code LLaMA: focuses on code generation, supporting multiple programming languages like Python, Java, and C++, and trained on a massive corpus of 500 billion tokens of code
LLM Key Concepts
Fine-tuning large language models involves adapting the pre-trained model to perform specific tasks or understand particular domains better. This is achieved by training the model on a new dataset that is more focused on the desired task or domain.
The fine-tuning process adjusts the weights of the model's neural network, enabling it to make better predictions or generate more accurate responses based on the new data.
Fine-tuning large language models is a crucial step in achieving accurate results. It allows the model to learn from new data and adapt to specific tasks or domains.
The key concept of fine-tuning is to adjust the weights of the model's neural network. This enables the model to make better predictions or generate more accurate responses.
Large language models can be fine-tuned for specific tasks or domains by training them on a new dataset. This process enables the model to learn from the new data and adapt to the specific task or domain.
The fine-tuning process involves adjusting the weights of the model's neural network. This is achieved by training the model on a new dataset that is more focused on the desired task or domain.
You might enjoy: How to Fine Tune Llm on Custom Data
Fine-Tuning LLaMa
Fine-tuning LLaMa involves several challenges, including the need for significant CPU RAM, which can lead to out-of-memory errors. This is especially true when using the FSDP (Fully Sharded Data Parallelism) method, which can require up to 2TB of CPU RAM for a 70B model.
To overcome this challenge, you can use a codebase like the one found on GitHub, which provides a solution to the FSDP issue. Additionally, you can use Parameter-Efficient Fine-Tuning (PEFT) methods like LoRA or QLoRA, which reduce the need for updating all model parameters. LoRA, for example, modifies only the weights of certain layers, while QLoRA quantizes the parameters to reduce memory usage.
To fine-tune LLaMa, you'll need to adjust the learning rate, batch size, and number of training epochs. You can also use Supervised Fine-Tuning (SFT), which involves training the model on a smaller, task-specific dataset under human supervision. This process helps the model adapt to specific tasks or domains, such as medical data analysis.
You might like: Architecture for Users to Fine Tune Lora
Here are some common challenges and solutions for fine-tuning LLaMa:
- FSDP issue: use a codebase like the one found on GitHub
- PEFT methods: use LoRA or QLoRA to reduce parameter updates
- Learning rate, batch size, and epochs: adjust these parameters to optimize training
- SFT: use a smaller, task-specific dataset for supervised fine-tuning
You can also test the fine-tuned model using the pipeline function, which generates text based on a prompt. This helps evaluate the model's performance on the new data.
Addressing Challenge 2
Addressing Challenge 2 requires choosing the right state dict type when creating FSDP config. SHARDED_STATE_DICT saves shard per GPU separately, making it quick to save or resume training from an intermediate checkpoint.
Using SHARDED_STATE_DICT instead of FULL_STATE_DICT can speed up the training process significantly. FULL_STATE_DICT, on the other hand, gathers the whole model on CPU before saving it, which can be a bottleneck.
To save the final checkpoint as the whole model state dict, a specific code snippet is used. This code is necessary to ensure that the model's state is properly saved and can be easily loaded later.
For more insights, see: Huggingface save Model
Create Bitsandbytes Configuration
To create a bitsandbytes configuration, we need to load our LLM in 4 bits, which will allow us to divide the used memory by 4 and import the model on smaller devices.
You might enjoy: How to Fine Tune Gpt 4
We choose to apply bfloat16 compute data type and nested quantization for memory-saving purposes. This will help us reduce the model's size without compromising its performance.
By setting up the bitsandbytes configuration, we can use the print_trainable_parameters() helper function to see how many trainable parameters are in the model.
The LoRa model is expected to have fewer trainable parameters compared to the original one, which is a good thing since we want to perform fine-tuning.
Fine-Tuning LLaMa
Fine-tuning LLaMa 2 is a process that requires careful consideration of various parameters and techniques. The Guanaco dataset from HuggingFace, which provides examples of 175 language tasks, is a great starting point for this process.
To fine-tune LLaMa 2, you'll need to use a Jupyter notebook with access to a GPU and sufficient memory. This will allow you to run the full script and experiment with different fine-tuning approaches.
The Supervised Fine-Tuning (SFT) approach is a great way to adapt LLaMa 2 to specific tasks or domains. By fine-tuning the model on a smaller, task-specific dataset under human supervision, you can enhance its applicability and accuracy.
Take a look at this: Fine Tune Ai
Parameter-Efficient Fine-Tuning (PEFT) is another technique that can be used to fine-tune LLaMa 2 without updating all of the model's parameters. This is achieved by focusing on a small subset of the model's parameters, making the fine-tuning process more efficient and less resource-intensive.
LoRA (Low-Rank Adaptation) is a popular method used in PEFT that involves modifying only the weights of certain layers within the model. This is done by applying low-rank matrices to transform these weights during the forward pass of the model.
The LoraConfig class specifies settings for Parameter-Efficient Fine-Tuning (PEFT), including parameters like lora_alpha, lora_dropout, r, and bias. These parameters define the architecture and behavior of the LoRA layers used for efficient fine-tuning.
By fine-tuning LLaMa 2 using SFT or PEFT, you can adapt the model to specific tasks or domains and enhance its accuracy and applicability. This is particularly useful for tasks that require a high level of domain-specific knowledge, such as medical data analysis.
The Guanaco dataset has 534,530 entries, providing a rich source of data for fine-tuning LLaMa 2. This dataset is specifically designed for English grammar analysis, natural language understanding, cross-lingual self-awareness, and explicit content recognition.
Fine-tuning LLaMa 2 can be a computationally intensive process, requiring significant computational power and memory. However, by using techniques like PEFT and LoRA, you can make the process more efficient and less resource-intensive.
A different take: Fine Tune Llama 2
Reinforcement Learning from Human Feedback
Reinforcement Learning from Human Feedback is an advanced fine-tuning technique used to further refine the performance of language models like LLaMA2.
This method involves training the model using feedback derived from human interactions, which serves as a reward signal that guides the model to learn which types of responses are preferred or more accurate in given contexts.
The model's objective is to maximize the positive feedback it receives, effectively aligning its responses more closely with human expectations and preferences.
Human evaluators interact with the model by providing inputs and then rating or correcting the outputs generated by the model, helping the model to understand nuances and subtleties in human communication.
RLHF is particularly useful for improving the model's performance in complex, subjective tasks such as conversation generation, ethical reasoning, or creative writing.
By using RLHF, the model can generate more appropriate, context-sensitive, and human-like responses, effectively refining its performance and making it more useful in real-world applications.
Suggestion: Huggingface Rlhf
Test the
Now that we've fine-tuned LLaMa, it's time to test its capabilities. The pipeline function is a high-level utility for text generation that we can use to test the model.
To test the model, we simply need to use the pipeline function with a simple prompt to generate text. This will give us an idea of how well the model has adapted to the new data.
The output will reflect the model's performance and give us an idea of how well it's learned from the new data. This is an essential step in fine-tuning LLaMa, and it's what makes the pipeline function so useful.
By testing the model, we can see firsthand how well it's adapted to the new data and make any necessary adjustments. This is where the fine-tuning process really comes together.
Worth a look: Hugging Face Text Summarization
Sources
- https://huggingface.co/blog/ram-efficient-pytorch-fsdp
- https://blog.ovhcloud.com/fine-tuning-llama-2-models-using-a-single-gpu-qlora-and-ai-notebooks/
- https://www.run.ai/guides/generative-ai/llama-2-fine-tuning
- https://www.analyticsvidhya.com/blog/2023/10/hugging-face-fine-tuning-tutorial/
- https://myscale.com/blog/how-to-fine-tune-llm-from-huggingface/
Featured Images: pexels.com