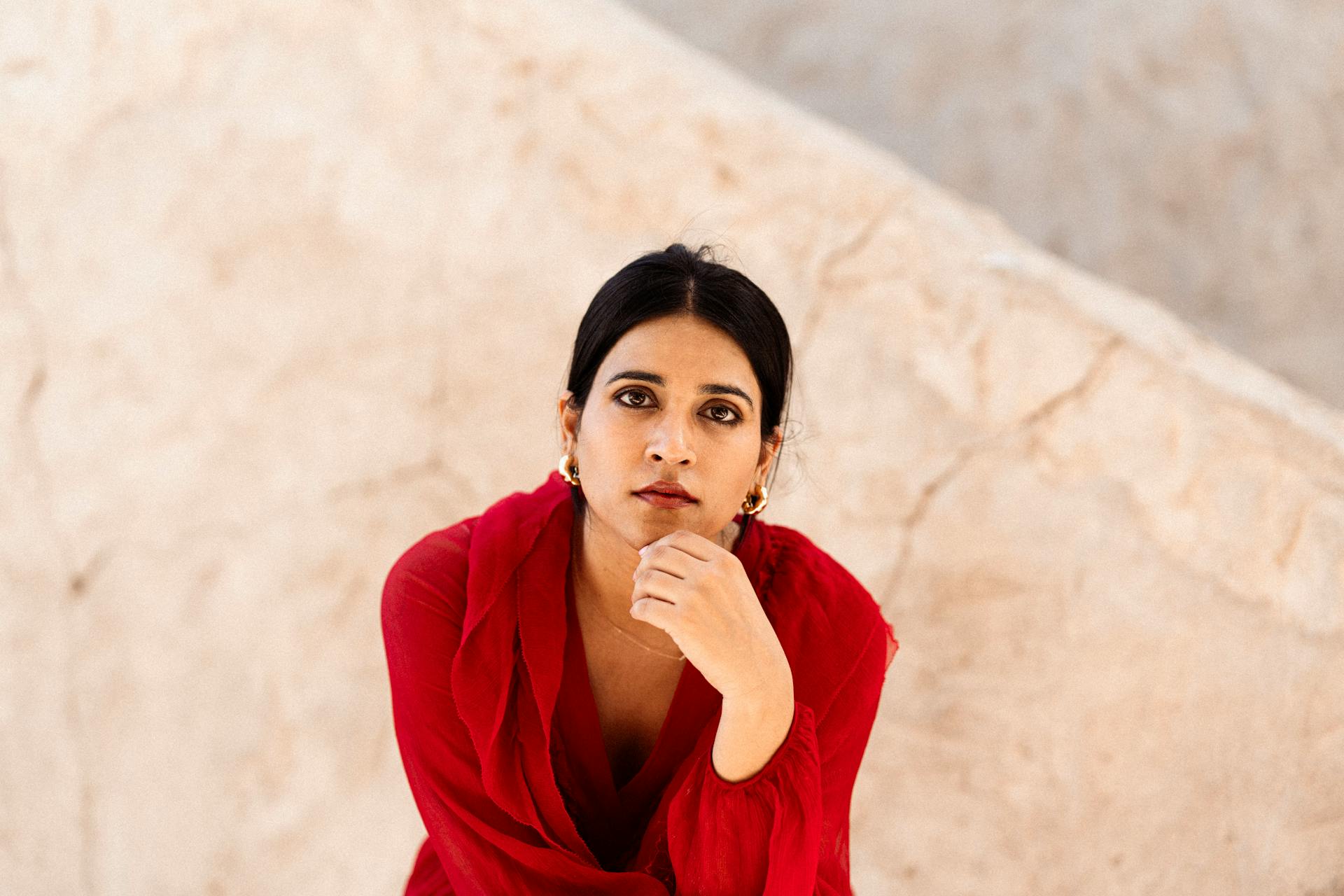
Geometric computation is a field that deals with the mathematical and computational aspects of geometric objects and shapes. It's a fundamental concept in computer graphics, engineering, and architecture.
At its core, geometric computation involves the manipulation and analysis of geometric data, such as points, curves, and surfaces. This can include tasks like mesh generation, surface reconstruction, and collision detection.
Geometric computation is used in a wide range of applications, from computer-aided design (CAD) software to video games and special effects. It's a crucial tool for visualizing and analyzing complex geometric data.
In the next section, we'll dive deeper into the basics of geometric computation, exploring topics like geometric algebra and computational geometry.
You might like: Geometric Feature Learning
Geometric Computation Basics
Parametric curves and surfaces are the most important instruments in numerical computational geometry, used for curve and surface modeling and representation.
Bézier curves, spline curves, and surfaces are examples of parametric curves and surfaces.
The level-set method is an important non-parametric approach in computational geometry.
You might enjoy: Computational Learning Theory
Application areas of computational geometry include the shipbuilding, aircraft, and automotive industries.
Wolfram Geometric Computation provides a comprehensive set of tools and solutions for modeling, analyzing, visualizing, and synthesizing geometry.
State-of-the-art geometric algorithms, such as triangulations, convex hulls, and data structures, are available in Wolfram Geometric Computation.
Convex hulls, Delaunay meshes, and other geometric algorithms are part of the extensive collection of geometric algorithms in Wolfram Geometric Computation.
To get started with geometric computation, you can begin with the basics and build your way up to more advanced concepts.
Here are some essential concepts to get you started:
- Parametric curves and surfaces
- Level-set method
- Triangulations, convex hulls, and data structures
- Convex hulls, Delaunay meshes, and other geometric algorithms
Algorithms and Techniques
Computational geometry relies on efficient algorithms to solve geometric problems. One such algorithm is the Sweep line algorithm, which solves problems like finding the closest pair of points or line segment intersection by sweeping a line or plane across the geometry.
A key aspect of computational geometry is the development of algorithms for solving problems stated in terms of basic geometrical objects. These problems include finding the convex hull of a set of points, which can be done using algorithms like Graham's and Chan's, with time complexities of O(n log n) and O(n log h), respectively.
Geometric query problems, such as range searching and point location, require preprocessing the search space to efficiently answer queries. The time and space required for preprocessing and querying are crucial in estimating the computational complexity of these problems.
- Range searching: Preprocess a set of points to efficiently count the number of points inside a query region.
- Point location problem: Given a partitioning of the space into cells, produce a data structure that efficiently tells in which cell a query point is located.
- Nearest neighbor: Preprocess a set of points to efficiently find which point is closest to a query point.
- Ray tracing: Given a set of objects in space, produce a data structure that efficiently tells which object a query ray intersects first.
Combinatorial Computational
Combinatorial computational geometry is a fascinating field that deals with the development of efficient algorithms and data structures for solving geometric problems. It's like trying to find the shortest route between two points on a map, but instead of just finding the shortest distance, you're also trying to figure out how to do it in the most efficient way possible.
Some of the primary goals of research in combinatorial computational geometry include finding efficient algorithms for solving problems stated in terms of basic geometrical objects, such as points, line segments, polygons, and polyhedra. This involves developing algorithms that can solve problems quickly and accurately, even with large amounts of data.
One classic result in computational geometry is the Closest pair problem, which involves finding the two points in a set of points that are closest to each other. This problem seems simple, but it's actually quite complex, and there are many different algorithms that have been developed to solve it. Some of these algorithms take O(n log n) time, while others take O(n) expected time.
Another important problem in combinatorial computational geometry is the Convex Hull problem, which involves finding the smallest convex set that contains a given set of points. This problem has many practical applications, such as in computer graphics and robotics.
Here are some key algorithms and techniques used in combinatorial computational geometry:
- Sweep line algorithm: This algorithm is used to solve a wide range of geometric problems, including computing the intersection of two lines or planes and triangulating a polygon.
- Graham's Algorithm: This algorithm is used to compute the Convex Hull of a set of points and takes O(n log n) time.
- Chan's Algorithm: This algorithm is an improvement over Graham's Algorithm and takes O(n log h) time, where h is the number of points in the Convex Hull.
- Triangulation and Partitioning: This technique involves dividing a large geometric structure into smaller, more manageable pieces, such as triangles.
These are just a few examples of the many algorithms and techniques used in combinatorial computational geometry. By understanding these concepts, you can develop more efficient and effective solutions to complex geometric problems.
Grading
Grading is a straightforward process in this course. You'll be assessed on your understanding of algorithms and techniques.
Homeworks make up 80% of your grade. This is a significant portion of the course, so make sure to stay on top of your assignments.
A project is also a part of the grading, accounting for 20% of your overall grade. You have the option to implement a Delaunay triangulation or discuss an alternative with your instructor.
Here's a breakdown of the grading: 80% for the homeworks.20% for the project.
Programming Languages
Geometric computation is a fascinating field that requires careful attention to detail.
Multiple programming languages can be used for geometric computation, including Java and JavaScript.
Java and JavaScript are both used in the examples provided, with Java's code snippet demonstrating the implementation of a line intersection algorithm in a class-based structure.
The JavaScript example, on the other hand, shows a more concise implementation of the same algorithm using functions.
Both Java and JavaScript implementations require careful calculation of the intersection point, taking into account the denominators and numerators of the equations.
The denominators are used to determine if the lines are parallel or not, while the numerators help calculate the intersection point.
Here are the programming languages used in the examples:
- Java
- JavaScript
Java
Java is a popular programming language that's widely used in various industries. It's known for its platform independence, which means you can run Java code on any device that has a Java Virtual Machine (JVM) installed.
Java has a vast collection of libraries and tools that make it easy to develop complex applications. For example, the Java Standard Edition (Java SE) library includes classes for working with graphics, sound, and networking.
One of the key features of Java is its object-oriented design. In Java, you can create objects that represent real-world entities, such as a point in space or a line on a graph.
Here's a simple example of how you can create a Point class in Java:
```
class Point {
double x, y;
Point(double x, double y) {
this.x = x;
this.y = y;
}
}
```
This code defines a Point class with two fields, x and y, which represent the coordinates of a point in space. The constructor takes two arguments, x and y, which are used to initialize the fields.
See what others are reading: Code Org Ap Computer Science Principles
In Java, you can also create classes that represent more complex objects, such as a Line. Here's an example of how you can define a Line class:
```
class Line {
Point p, q;
Line(Point p, Point q) {
this.p = p;
this.q = q;
}
}
```
This code defines a Line class with two fields, p and q, which represent the endpoints of a line. The constructor takes two arguments, p and q, which are used to initialize the fields.
Java has a built-in method for checking if two lines intersect, called doIntersect. This method takes two Line objects as arguments and returns a boolean value indicating whether the lines intersect.
Here's an example of how you can use the doIntersect method to check if two lines intersect:
```
public class Main {
public static void main(String[] args) {
Line l1 = new Line(new Point(0, 0), new Point(1, 1));
Line l2 = new Line(new Point(0, 1), new Point(1, 0));
if (doIntersect(l1, l2)) {
// Function call
} else {
System.out.println("Lines do not intersect");
}
}
A fresh viewpoint: Copilot New Computer Ai
}
```
In this example, we create two Line objects, l1 and l2, and then use the doIntersect method to check if they intersect. If the lines intersect, we can use the computeIntersection method to find the intersection point.
Here's an example of how you can use the computeIntersection method to find the intersection point:
```
public class Main {
public static void main(String[] args) {
Line l1 = new Line(new Point(0, 0), new Point(1, 1));
Line l2 = new Line(new Point(0, 1), new Point(1, 0));
if (doIntersect(l1, l2)) {
Point p = computeIntersection(l1, l2);
System.out.println("Intersection point: (" + p.x + ", " + p.y + ")");
} else {
System.out.println("Lines do not intersect");
}
}
}
```
In this example, we use the computeIntersection method to find the intersection point of the two lines, and then print the result to the console.
Here's an interesting read: How to Use Ai in Computer
Python
Python is an excellent language for implementing the sweep line algorithm, as demonstrated in the provided code example. The algorithm assumes that the objects being swept are not overlapping in the same event point, which is a crucial assumption to avoid algorithm failure.
The algorithm's implementation in Python involves defining classes for Point and Line, which are then used to check for intersections and compute the intersection point. This is achieved through the use of functions like doIntersect and computeIntersection.
One key aspect of the algorithm's implementation is the use of mathematical formulas to determine whether two lines intersect and, if so, compute the intersection point. This is evident in the computeIntersection function, where the formulas for the intersection point are derived from the line equations.
The algorithm's performance can be affected by the need for sorting and searching operations, which can have a high computational cost. However, this is a general consideration and not specific to the Python implementation.
Here are some key points to note about the Python implementation:
- The algorithm assumes that the objects being swept are not overlapping in the same event point.
- The implementation involves defining classes for Point and Line.
- The algorithm uses mathematical formulas to determine intersection points.
- Sorting and searching operations can affect the algorithm's performance.
Overall, the Python implementation of the sweep line algorithm provides a clear and concise example of how to approach this problem in a programming language.
Multi-Paradigm Modeling
Multi-Paradigm Modeling is a powerful approach to geometric modeling that allows you to model regions using various methods, including meshes, constructive solid geometry, and implicit representation.
You can combine and transform these regions to create new ones, making it a versatile tool for a wide range of applications.
One of the key benefits of Multi-Paradigm Modeling is that it provides a comprehensive set of tools and solutions, tailored to everyone's needs from beginners to advanced users.
Here are some specific guides that can help you get started with Multi-Paradigm Modeling:
- Guide to Mesh Modeling
- Guide to Solid Modeling
- Guide to Geometry Modeling
By using these guides, you can learn how to model geometric regions using different methods and create complex shapes with ease.
Frequently Asked Questions
What is 3D computational geometry?
Computational geometry in 3D involves designing and analyzing algorithms to solve geometric problems in three dimensions, with applications in various fields. It's a branch of computer science that uses elegant techniques to tackle complex geometric challenges.
What is computational geometry vs computer graphics?
Computational geometry is the theoretical foundation for geometric computations, while computer graphics is the practical application of algorithms to display geometry on a computer screen
Sources
- https://en.wikipedia.org/wiki/Computational_geometry
- https://www.wolfram.com/language/core-areas/geometry/index.php.en
- https://www.geeksforgeeks.org/what-is-computational-geometry-and-how-is-it-applied-in-solving-geometric-problems/
- https://medium.com/@harshitsikchi/exploring-computational-geometry-where-to-start-33ccfcbe09f4
- https://people.eecs.berkeley.edu/~jrs/274/
Featured Images: pexels.com