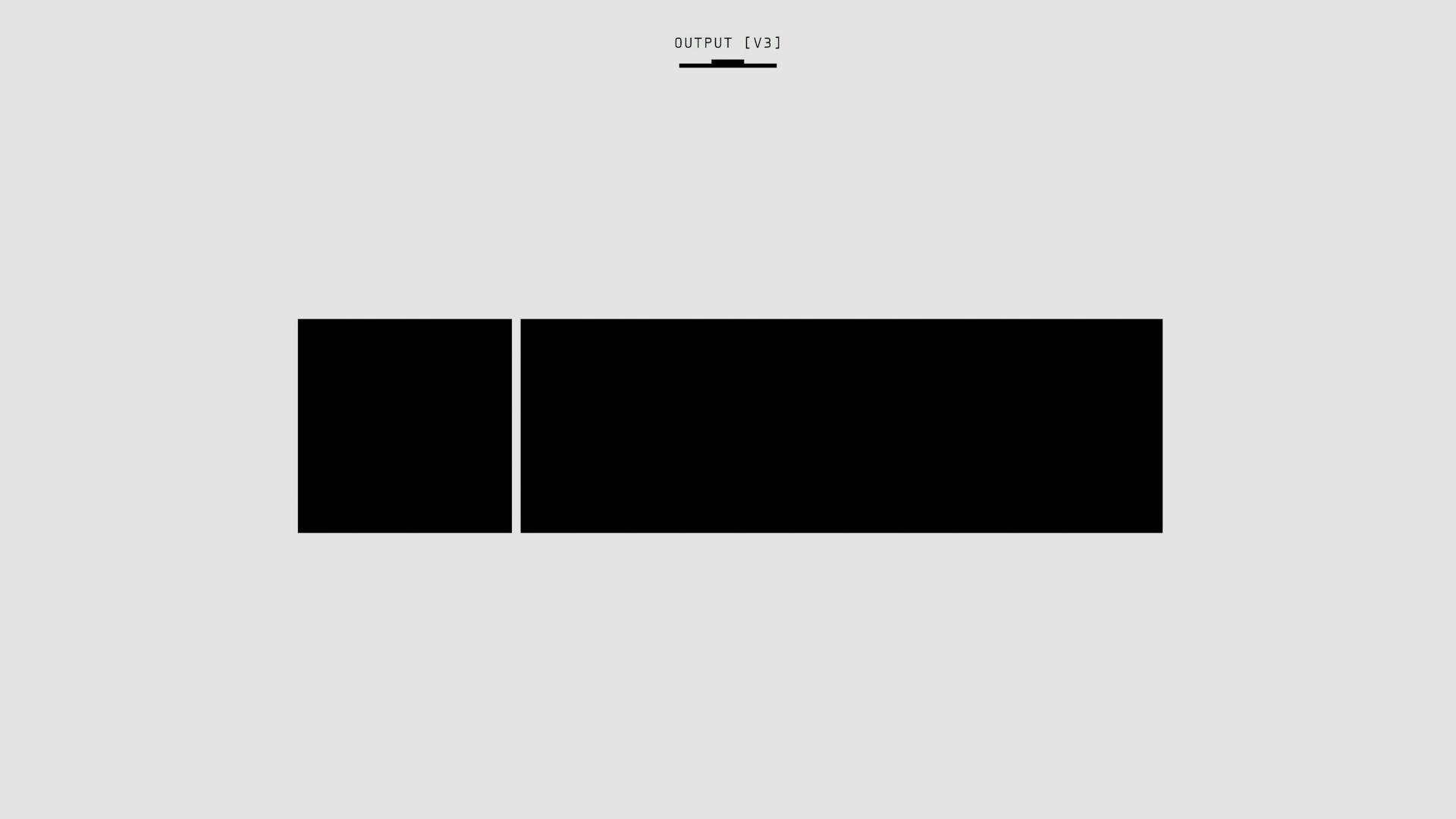
Grokking algorithms is a journey, not a destination. It starts with understanding the basics of computer science, such as data structures like arrays and linked lists.
To begin, you need to grasp the concept of time and space complexity, which is crucial for evaluating algorithm efficiency. A good starting point is to learn about Big O notation, which helps you analyze algorithms' performance.
As you progress, you'll encounter sorting algorithms like Bubble Sort and Selection Sort, which are essential for organizing data. These algorithms have an average time complexity of O(n^2), making them inefficient for large datasets.
Practice is key to mastering algorithms, and you can start by solving problems on platforms like LeetCode or HackerRank.
Recommended read: Grokking Data Structures
Algorithms Fundamentals
Grokking algorithms is a great way to learn about common algorithms, and the book is fully illustrated, making it a friendly guide.
The book covers search, sort, and graph algorithms, which are essential for any programmer to know.
Over 400 pictures with detailed walkthroughs make it easy to understand complex concepts.
You'll learn about performance trade-offs between algorithms, which is crucial for writing efficient code.
The book uses Python-based code samples, which is a great language for beginners.
Here's a quick summary of what you'll learn:
- Covers search, sort, and graph algorithms
- Over 400 pictures with detailed walkthroughs
- Performance trade-offs between algorithms
- Python-based code samples
If you're new to programming, you might appreciate that this book makes math fun and easy!
Data Structures
As you start to explore the world of algorithms, it's essential to understand the basics of data structures. Arrays and linked lists are two fundamental data structures that are commonly used in programming.
Arrays are great for sequential access, allowing you to quickly read and write data in a linear fashion.
Arrays
Arrays are a type of data structure where data is stored contiguously in memory.
This means that if you need to add more data, everything has to be moved to a different location that will allow it to be stored contiguously, which can be slow.
Inserting data into the middle of an array requires moving everything else to the right to make space for the new item, which can be a time-consuming process.
Adding data can be quite slow, even if you reserve slots for future use, as those slots will be wasted if they're not used.
Deleting data from an array is also slow, as everything has to be moved up to fill the empty space.
Linked Lists
Linked Lists are a type of data structure that stores data in a way that's different from arrays. Each item in a linked list stores the address of the next item in the list.
This makes it possible to save data anywhere in memory, which solves the problem of needing to store everything together. You can think of it like a chain of connected boxes, where each box has a note with the address of the next box.
Reading data from a linked list is slow because you have to access all the previous items to get to the last one. I've seen this in action when working with large datasets, and it's not exactly the most efficient process.
Adding and deleting items in a linked list is fast, though, because it only requires changing the address of the next item in the list. This is a big advantage when working with dynamic data that's constantly changing.
The Stack
The Stack is a fundamental data structure that follows a simple yet powerful principle: Last In, First Out (LIFO). This means that items are added at the top and removed from the top, making it a great tool for tasks that require a specific order of operations.
Items are added to the top of the stack using a process called push, and removed from the top using a process called pop. This is in contrast to a queue, which is a First In, First Out (FIFO) data structure.
A classic example of the stack in action is in the calculation of the factorial of a number. As we'll see in the next section, the call stack is used to manage the recursive calls involved in this calculation.
Here's a simple breakdown of the stack's behavior:
The stack's LIFO behavior means that the most recently added item is always the first one to be removed. This can be a bit counterintuitive at first, but it's a crucial aspect of how the stack works.
The stack's simplicity and efficiency make it a valuable tool in a wide range of applications, from mathematical calculations to programming tasks.
Frequently Asked Questions
Do grokking algorithms cover data structures?
Yes, Grokking Algorithms covers various data structures such as arrays, lists, hash tables, trees, and graphs. This comprehensive coverage will help you master the fundamentals of computer science and programming.
What language do grokking algorithms use?
Grokking Algorithms uses Python as its primary example language. Dive into the world of algorithms with Python as your guide.
Featured Images: pexels.com