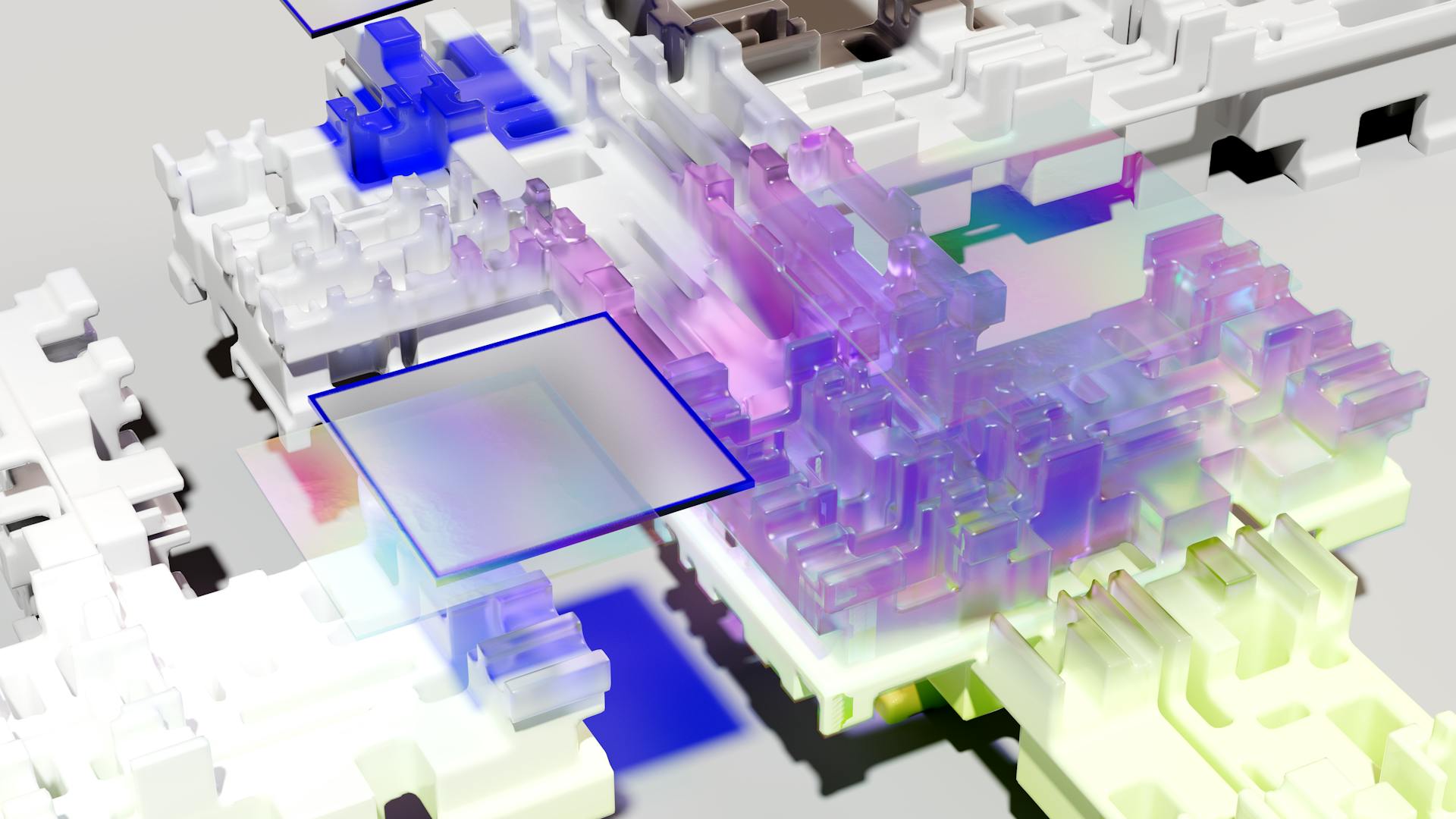
Grokking data structures is not just about memorizing formulas and algorithms, it's about understanding the underlying concepts that make them work. A data structure is a way to organize and store data in a way that makes it efficient to access and manipulate.
Arrays are a fundamental data structure that can be used to store a collection of elements of the same data type. In fact, arrays are so versatile that they can be used to implement other data structures like stacks and queues.
In the context of arrays, indexing is a crucial concept that allows us to access a specific element within the array. Indexing is based on the concept of zero-based indexing, where the first element is at index 0.
Understanding the basics of data structures like arrays and indexing is essential for building more complex data structures like linked lists and trees.
For more insights, see: Compsci 61b
Data Structure Fundamentals
Data structures are the building blocks of programming, and understanding them is crucial for any aspiring coder. A data structure is a way to organize and store data in a program, making it easier to access and manipulate.
Arrays are a type of data structure that store multiple values of the same type in a single variable. They are often used to store a collection of items, such as a list of names or a set of numbers.
Linked lists, on the other hand, are a type of data structure that store a sequence of nodes, each containing a value and a reference to the next node. This allows for efficient insertion and deletion of nodes at any position in the list.
In summary, data structures are essential for any programmer to master, and understanding the basics of arrays and linked lists is a great starting point.
Deletion
Deletion can be a challenging operation, especially when dealing with arrays.
Lists are generally more efficient, as they only require changing the previous element's pointer.
In a list, deleting an element is a relatively straightforward process.
You simply update the pointer of the previous element to skip over the deleted element.
This is in contrast to arrays, where every element needs to be shifted up when one is deleted.
For instance, Netflix's algorithm works similarly, making lists a more appealing choice for deletion operations.
This is because lists adapt more easily to changing data, making them a more practical choice for many use cases.
Data Structure Fundamentals
Data structures are a key concept in computer science, and they're introduced to students as part of a high school or college computer science curriculum.
Java is an excellent choice for coding interviews because it's a popular and widely used programming language in the industry, effectively supporting core data structures and algorithms.
To crack Java coding interviews, you need to thoroughly understand the basics, including OOP principles, Java data structures, exception handling, and multithreading.
Practice coding problems on platforms like Educative, LeetCode, and HackerRank to apply these concepts effectively and improve your problem-solving skills.
Coding patterns are common strategies or techniques for solving recurring coding problems asked in coding interviews, including two pointer techniques, sliding windows, backtracking, greedy algorithms, and dynamic programming.
For your interest: Data Science vs Ai vs Ml
Data structures are abstract and can be difficult to visualize, but drawing them on paper can help make the concepts clearer and the solutions more accessible.
To learn data structures in a fun and effective way, it's helpful to have a basic understanding of programming and to be able to visualize the concepts.
In a typical textbook, data structures are explained with pages and pages of text and code, but this book provides vivid pictures of data structures to help you learn in a more engaging way.
This book is designed for students who have a basic understanding of programming and want to take their skills to the next level, as well as for new graduates and junior programmers who need to refresh their memory and prepare for coding interviews.
A fresh viewpoint: Get Started with Programming
Searching and Sorting
Searching and sorting are fundamental concepts in data structures that help us find and organize data efficiently. Binary search is a great example of this, where we can find an element in a sorted list in just log_2_n steps, compared to simple search which takes n steps.
The key to binary search is to start from the middle of the list and eliminate half the numbers with each step. This is how we can find the desired element in just a few steps. For instance, if we're searching for a number between 50 and 100, we can start by finding the middle, which is 75, and then keep cutting the range in half until we find the number.
Quicksort is another popular sorting algorithm that uses a recursive approach to sort arrays efficiently. It works by selecting a pivot element, partitioning the array into smaller arrays, and then recursively sorting these arrays until the entire array is sorted. The average runtime of quicksort is O(n log n), but the worst case is O(n²) time.
Here's a comparison of the time complexities of simple search, binary search, and quicksort:
Binary Search
Binary search is a clever way to find an element in a sorted list. It works by repeatedly dividing the search space in half, starting from the middle of the list.
This approach is much faster than simple search, which checks every single index in the list until it finds the desired element. In fact, binary search can find an element in just log_2_n steps, whereas simple search takes n steps.
The key to binary search is finding the middle of the search space and deciding whether to cut the list in half or not. For example, if we're searching a range of 50-100, the middle is 75, but we can cut again to 63 or 57 to find the element more quickly.
This process of elimination is what makes binary search so efficient.
Quicksort
Quicksort is a sorting algorithm that's generally faster than selection sort and is often used. It uses a recursive algorithm structure for fast and proper sorting.
The process of quicksort involves picking an element from the array, known as a pivot, and then binning the smaller and larger elements into two separate arrays through a process called partitioning.
You can think of quicksort like a recursive process that continues until you get sorted arrays, which can then be concatenated to get the final output.
Quicksort has an average runtime of O(n log n), but the worst case is O(n²) time.
In practice, quicksort is faster because it meets the average case substantially more than the worst case.
The pivot matters in quicksort, and you want to choose both arrays to be as small as possible. Choosing the middle or a random number is a good approach.
Here are some key facts about quicksort:
- Quicksort has an average runtime of O(n log n).
- The worst case runtime of quicksort is O(n²) time.
- Quicksort is generally faster in practice due to its average case performance.
- The pivot choice can significantly impact quicksort's performance.
In the best-case scenario, quicksort takes O(n log n) time, which is achieved when the height of the call stack is O(log n) and each level takes O(n) time.
Breadth-First Search
Breadth-First Search is a powerful algorithm for solving shortest-path problems. It's a crucial tool in graph theory, helping us navigate complex connections between nodes.
To solve a problem using breadth-first search, we first need to model it as a graph. A graph is simply a way to set connections between nodes and edges. Nodes are the points of connection, and edges are the lines that connect them.
There are two main questions we can ask about a graph: is there a path from one node to another, and what is the shortest path between them? Breadth-first search can help us answer these questions.
Here are the two main steps to solve a problem using breadth-first search:
- Model the problem as a graph
- Solve the problem using breadth-first search
By following these steps, we can efficiently find the shortest path between two nodes in a graph.
Sources
- https://www.educative.io/courses/grokking-coding-interview
- https://www.lavivienpost.com/grokking-data-structures/
- https://srianumakonda.medium.com/understanding-data-structures-with-grokking-algorithms-57005ce03a0
- https://blackstonebookstore.com/book/9781633436992
- https://www.target.com/p/grokking-data-structures-by-marcello-la-rocca-paperback/-/A-91953862
Featured Images: pexels.com