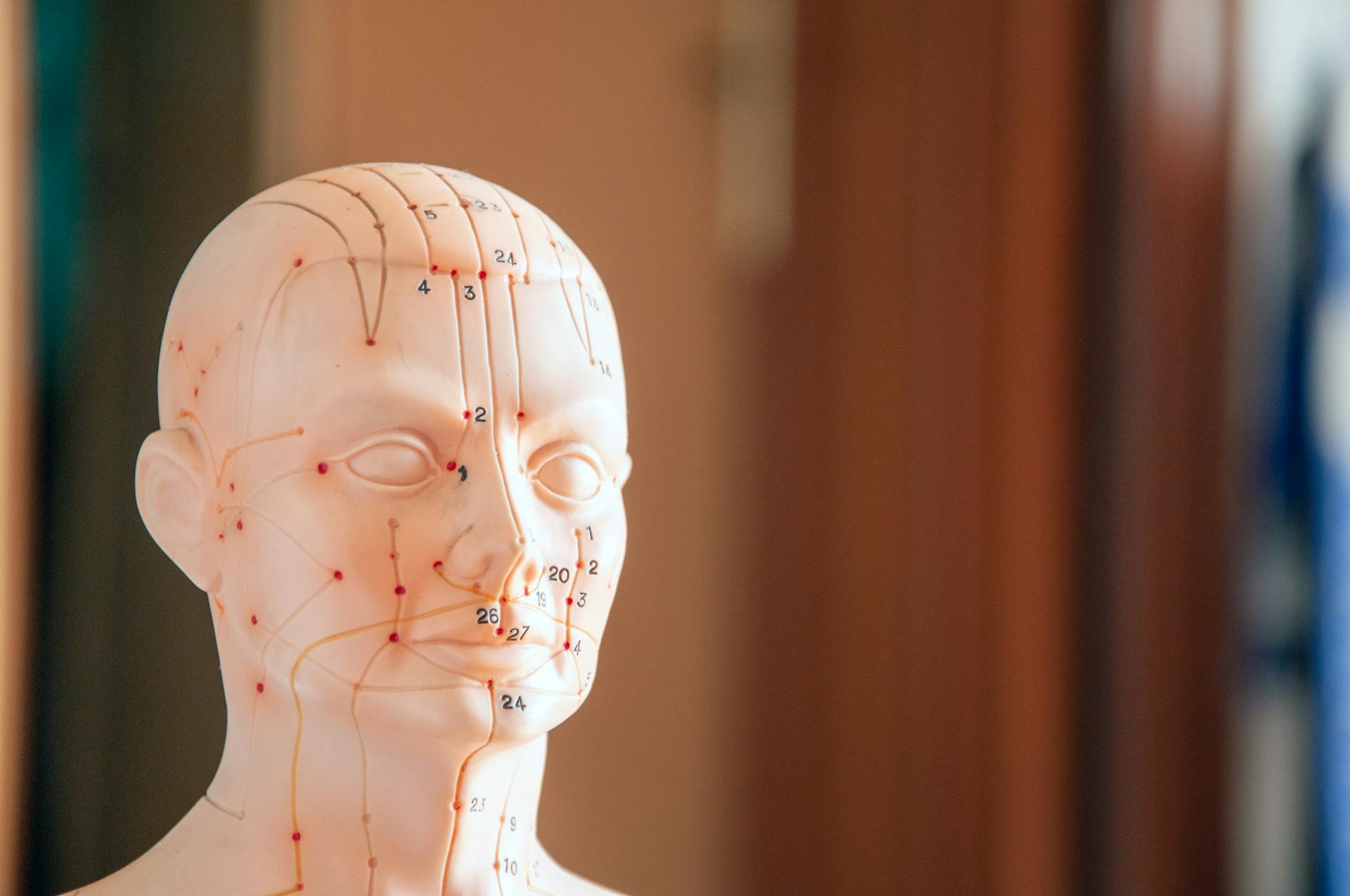
Grokking object-oriented design is a game-changer for system design skills.
It allows you to break down complex systems into smaller, manageable parts, making them easier to understand and maintain.
By understanding the principles of object-oriented design, you can create systems that are more flexible and scalable.
This is especially important in software development, where systems often need to adapt quickly to changing requirements.
Object-oriented design helps you achieve this by using concepts like inheritance and polymorphism to create reusable code.
Inheritance allows you to create new classes based on existing ones, reducing code duplication and making it easier to modify the system.
Polymorphism enables you to write code that can work with different types of objects, making your system more flexible and adaptable.
Worth a look: Grokking the System Design Intervie
What Is
Object-Oriented Design (OOD) is a programming technique that solves software problems by building a system of interrelated objects.
It makes use of the concepts of classes and objects to model real-world entities and their interactions.
A system architecture that is modular, adaptable, and simple to understand and maintain is produced using OOD.
A fresh viewpoint: Grokking System Design Fundamentals Pdf
OOD Principles
OOD Principles are the foundation of good object-oriented design. Encapsulation is key, bundling data with methods that operate on the data, restricting direct access and protecting object integrity.
Abstraction is a crucial principle, simplifying complex systems by modeling classes that fit the problem domain, highlighting essential features while hiding unnecessary details. This makes it easier to work with complex systems.
Inheritance is a powerful tool, establishing a hierarchy between classes that allows derived classes to inherit properties and behaviors from base classes, promoting code reuse and extension. This helps keep code organized and efficient.
Polymorphism enables objects to be treated as instances of their parent class, allowing one interface to be used for a general class of actions, improving flexibility and integration. This makes it easier to write code that can work with different types of objects.
Composition is another important principle, building complex objects by combining simpler ones, promoting reuse and flexible designs. This helps keep code modular and easy to maintain.
Readers also liked: Machine Learning Systems Design
The SOLID Principles are a set of five principles that help guide object-oriented design:
- S - Single responsibility principle: A class should have only one reason to change.
- O - Open/closed principle: A class should be open for extension but closed for modification.
- L - Liskov substitution principle: Derived classes should be substitutable for their base classes.
- I - Interface segregation principle: A client should not be forced to depend on interfaces it does not use.
- D - Dependency inversion principle: High-level modules should not depend on low-level modules, but both should depend on abstractions.
OOD Concepts
OOD Concepts are the building blocks of object-oriented design. They help create systems that are reliable, expandable, and maintainable.
Encapsulation is a fundamental principle of OOD, which bundles data with methods that operate on the data, restricting direct access to some components and protecting object integrity. This ensures controlled access to the internal state of an object.
Abstraction involves hiding complex implementation details and showing only the essential features of the object. This helps manage complexity by allowing the designer to focus on interactions at a higher level.
Inheritance is a key concept in OOD, which establishes a hierarchy between classes, allowing derived classes to inherit properties and behaviors from base classes. This promotes code reuse and extension.
Polymorphism enables objects to be treated as instances of their parent class, allowing one interface to be used for a general class of actions. This improves flexibility and integration.
Related reading: Grokking Data Structures
Composition is another important concept in OOD, which builds complex objects by combining simpler ones. This promotes reuse and flexible designs.
Here are the key principles of OOD:
- Encapsulation: Bundling data with methods that operate on the data, restricting direct access to some components and protecting object integrity.
- Abstraction: Simplifying complex systems by modeling classes appropriate to the problem domain, highlighting essential features while hiding unnecessary details.
- Inheritance: Establishing a hierarchy between classes, allowing derived classes to inherit properties and behaviors from base classes, promoting code reuse and extension.
- Polymorphism: Enabling objects to be treated as instances of their parent class, allowing one interface to be used for a general class of actions, improving flexibility and integration.
- Composition: Building complex objects by combining simpler ones, promoting reuse and flexible designs.
Understanding these concepts is essential for effective OOD, and they form the foundation of creating reliable, scalable, and maintainable systems.
UML Diagrams
UML diagrams are a crucial part of object-oriented design, and they're used to visualize the structure and behavior of a system. They help developers understand the relationships between classes, objects, and interactions.
One of the most common types of UML diagrams is the Class Diagram, which shows the static structure of a system. It's like a blueprint of the system's classes, attributes, and relationships. In a Class Diagram, you'll see classes represented as rectangles, with attributes listed inside and relationships between classes shown as lines.
A Class Diagram can be used to identify the main classes of a system, such as the ones in the Parking Lot System, which includes classes like ParkingRate, ExitPanel, and ParkingFloor. Similarly, the Online Stock Brokerage System has classes like Account, Customer, Admin, and Guest, which represent the different types of users interacting with the system.
Another type of UML diagram is the Activity Diagram, which shows the flow of control in a system. It's like a flowchart that illustrates the steps involved in a use case. Activity diagrams are used to model workflow or business processes and internal operations.
For example, in the Movie Ticket Booking System, the Activity Diagram shows the steps involved in searching movies, creating/modifying/viewing bookings, making payments, and assigning seats. Similarly, in the Online Shopping System, the Activity Diagram illustrates the steps involved in adding/updating products, searching products, adding/removing product items to the shopping cart, and checking out to buy product items.
Sequence Diagrams are another type of UML diagram that shows the interactions between objects over time. They're used to model the dynamic behavior of a system, and they help developers understand the sequence of events that occur in a system.
Here's a summary of the main UML diagrams:
- Class Diagram: shows the static structure of a system, including classes, attributes, and relationships.
- Activity Diagram: shows the flow of control in a system, illustrating the steps involved in a use case.
- Sequence Diagram: shows the interactions between objects over time, modeling the dynamic behavior of a system.
By using these UML diagrams, developers can create a clear and concise representation of a system's structure and behavior, making it easier to understand and work with the system.
Sources
- https://realtoughcandy.com/grokking-the-object-oriented-design-interview/
- https://www.sartiano.it/marco/diigo/System%20Design/Grokking%20the%20Object%20Oriented%20Design%20Interview.html
- https://www.educative.io/courses/grokking-the-low-level-design-interview-using-ood-principles
- https://loadcourse.com/library/educative-grokking-the-object-oriented-design-interview/
- https://www.geeksforgeeks.org/oops-object-oriented-design/
Featured Images: pexels.com