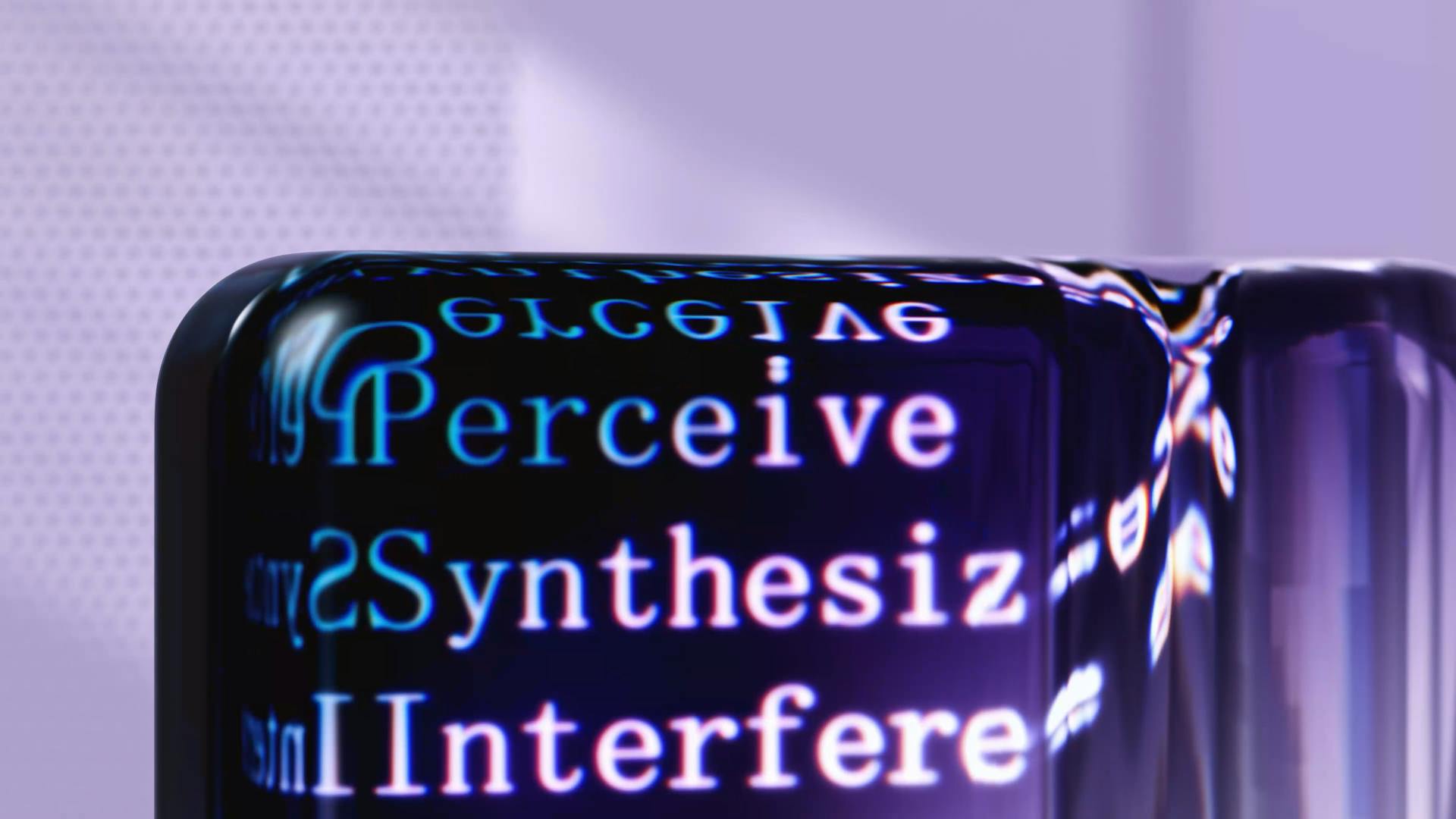
In TensorFlow, hidden layers are a crucial part of neural networks, allowing them to learn complex patterns in data.
A hidden layer is essentially a layer of neurons that processes the input data, transforming it into a more abstract representation that can be used by the next layer.
The number of hidden layers in a neural network can significantly impact its performance, with more layers often allowing for more complex models.
In TensorFlow, you can create a hidden layer using the `tf.keras.layers.Dense` layer, specifying the number of units and activation function.
Recommended read: Neural Network vs Generative Ai
TensorFlow Setup
TensorFlow Setup is where you'll start building your neural network with hidden layers. TensorFlow provides a high-level API called Keras for this purpose.
To use the Sequential API in TensorFlow, you'll need to create a neural network with hidden layers. This API is a convenient way to build and train models without worrying about the underlying architecture.
With Keras, you can easily set up hidden layers in your neural network, making it a great choice for beginners and experts alike.
Take a look at this: Tensorflow Transfer Learning
Setting Up TensorFlow
TensorFlow provides a high-level API called Keras for building neural networks with hidden layers.
You can use the Sequential API to create a neural network with hidden layers in TensorFlow.
TensorFlow's Keras API is designed to be user-friendly and easy to use, making it a great choice for beginners.
To get started with building neural networks in TensorFlow, you'll need to import the necessary libraries and set up your environment.
TensorFlow provides a variety of tools and resources to help you learn and work with the platform, including tutorials and documentation.
Discover more: Hidden Layers in Neural Networks
If Categorical
When dealing with categorical output, TensorFlow uses the softmax function to ensure the output is a valid probability distribution. This is especially important when you have multiple classes, like in the example with four classes ($M=4$).
The softmax function is applied to the last layer of the neural network, and it's used to convert the raw output into a probability distribution. In the case of binary output, the sigmoid function is used instead.
On a similar theme: Gumbel Softmax Reparameterization Trick
For example, if you have four classes, the output would look something like this: $y_1 = \sigma(a^{(3)}_1)$, $y_2 = \sigma(a^{(3)}_2)$, $y_3 = \sigma(a^{(3)}_3)$, and $y_4 = \sigma(a^{(3)}_4)$. This means the output is a probability distribution over all four classes.
The softmax function is a great way to ensure the output is a valid probability distribution, but it can be a bit tricky to implement.
You might enjoy: Claude 3 Context Window
Neural Network Basics
A neural network is made up of layers, and each layer has its own number of nodes. The first layer has J nodes, and the input vector X has L elements.
The weights for each node in the first layer are stored in a vector W, and the biases are stored in a vector b. We can absorb b into X by adding a column of ones to X, making it a matrix of size JxL+1.
The affine transformation is then calculated as a = W^T X, where a is a vector of size J. The output from each node is then evaluated using a non-linear function σ, resulting in a vector u of size J.
If this caught your attention, see: Code First Girls
Let's consider a simple example with 3 input points, 2 hidden layers, and 2 nodes in each layer. This is a linear model, and the mathematical relationship between input and output remains the same.
However, we can add another layer to the network, creating a hidden layer. The value of each neuron in the hidden layer is calculated using the same formula as the output of a linear model.
The neurons in the next layer are then calculated using the hidden layer's neuron values as inputs. This allows the model to recombine the input data using another set of parameters, enabling it to learn nonlinear relationships.
See what others are reading: Hidden Technical Debt in Machine Learning Systems
Code Explanation
In neural networks, the input layer defines the shape of the input data, which in this case is a flattened 28×28 image. The input shape is defined as (784,).
When designing hidden layers, two critical decisions need to be made: the number of neurons and the activation function. In the given example, two hidden layers are used, one with 128 neurons and the other with 64 neurons, both using the ReLU activation function.
ReLU is commonly used for hidden layers because it efficiently avoids vanishing gradient problems. The output layer, on the other hand, uses the softmax activation function for classification tasks, which converts raw scores into probabilities for each class.
Here's a summary of the activation functions used in the example:
Code Explanation
The input layer of a neural network is defined by its shape, which in this case is (784,), representing a flattened 28×28 image.
The model contains two hidden layers with 128 and 64 neurons, respectively, both using the ReLU activation function. This activation function is commonly used for hidden layers because it efficiently avoids vanishing gradient problems.
The output layer of a neural network uses the softmax activation function for classification tasks, which converts raw scores into probabilities for each class.
You need to make critical decisions when designing hidden layers, such as choosing the number of neurons and the activation function.
The affine function transforms inputs into outputs, and a simpler sigmoid function can be defined using the logistic function: σ(z) = 1 / (1 + e^(-z)).
The sigmoid function is a family of functions, and the logistic function is just one member of that family.
Changing the weights and biases (w and b) of the sigmoid function can affect its shape and flexibility, but it may not always match the actual function.
The output layer of a neural network is simply the logistic function, which can only have so much flexibility.
Exercise 2
In Exercise 2, a hidden layer containing four neurons was added to the model. This layer was used to calculate the value of the four hidden-layer nodes and the output node for the input values $x_1 = 1.00$, $x_2 = 2.00$, and $x_3 = 3.00$.
The calculations performed on the hidden-layer nodes were linear, consisting of multiplication and addition operations. This is because each node in the hidden layer was performing a linear calculation.
The output node's calculation was also linear, as it was performing a linear calculation on the output of linear calculations. This means the model cannot learn nonlinearities.
Modifying the model parameters can affect the hidden-layer node values and the output value. Reviewing the Calculations panel can show how these values were calculated.
Sources
- https://www.slideshare.net/slideshow/hidden-layers-in-neural-networks-code-examples-tensorflow/271579198
- https://learnindata.com/hidden-layers-in-neural-networks-code-examples-tensorflow/
- https://pythonprogramminglanguage.com/tensorflow-neural-network/
- https://harvard-iacs.github.io/2019-CS109A/labs/lab11/notebook/
- https://developers.google.com/machine-learning/crash-course/neural-networks/nodes-hidden-layers
Featured Images: pexels.com