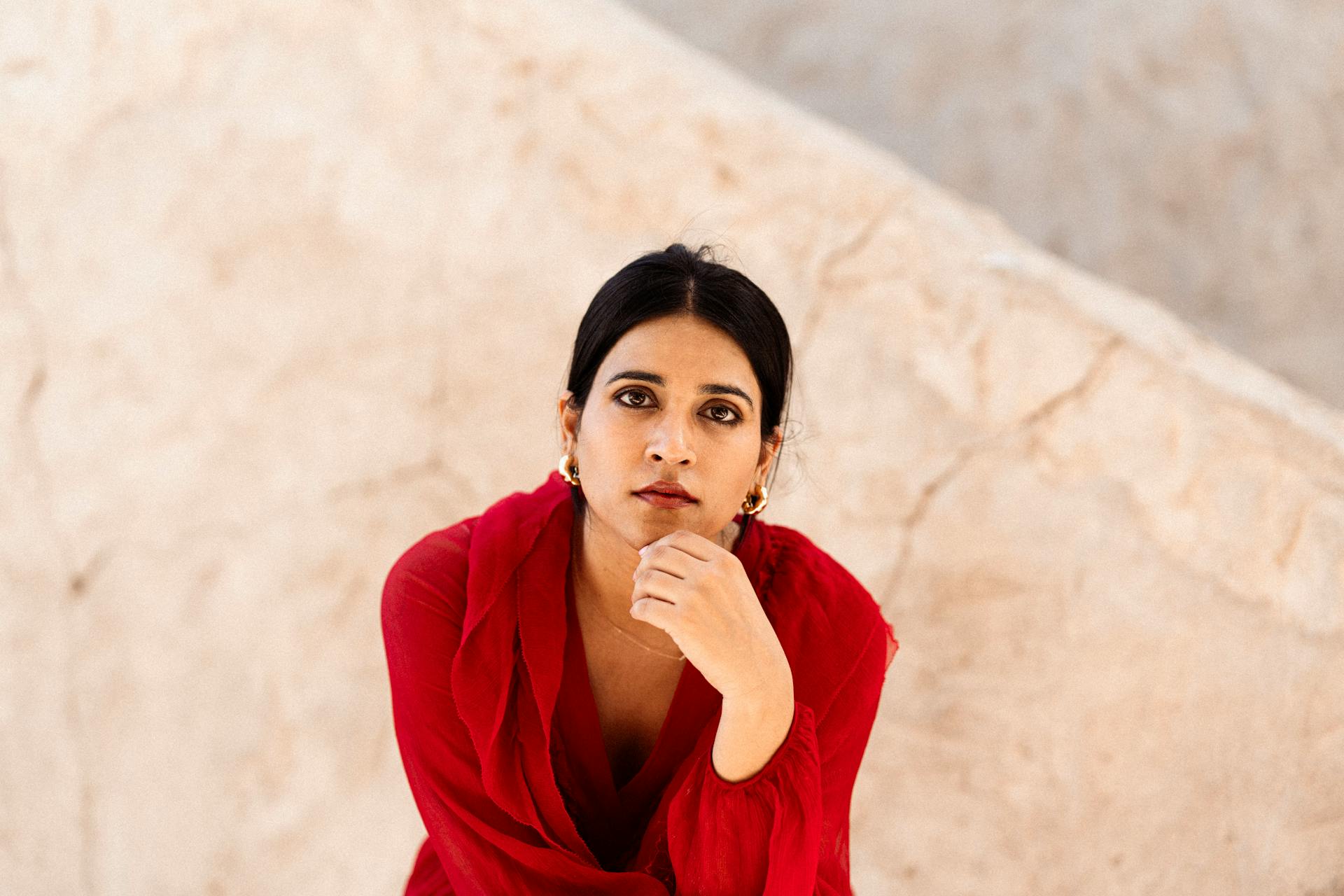
TensorFlow transfer learning is a powerful technique that can save you a ton of time and effort when building deep learning models. By leveraging pretrained models, you can get started with your project much faster.
Pretrained models are essentially pre-trained neural networks that have already been trained on a large dataset. This means you can use them as a starting point for your own project, fine-tuning them to fit your specific needs.
With TensorFlow, you can easily load and use these pretrained models, thanks to its built-in support for transfer learning. This makes it a breeze to get started with transfer learning, even if you're new to deep learning.
By using a pretrained model, you can skip the time-consuming process of training a neural network from scratch.
For another approach, see: Grid Search Tensorflow
What Is TensorFlow Transfer Learning?
TensorFlow transfer learning is all about leveraging the power of pre-trained models to speed up your development process. This technique allows you to reuse the feature representations from a pre-trained model, so you don't have to train a new model from scratch.
Pre-trained models are usually trained on massive datasets that are a standard benchmark in the computer vision frontier. These models can be used directly in making predictions on new tasks or integrated into the process of training a new model.
The advantage of pre-trained models is that they are generic enough for use in other real-world applications. For example, models trained on ImageNet can be used in real-world image classification problems.
You can use pre-trained models to initialize the weights of a new model, especially when you have a small training dataset. This can lead to lower training time and lower generalization error.
Here are some examples of how pre-trained models can be used in real-world applications:
- Models trained on ImageNet can be used in real-world image classification problems, such as classifying insects.
- Pre-trained word embeddings like GloVe can be used to hasten the development process in natural language processing problems.
When to Use Transfer Learning
You need a lot of data to train a model with high accuracy, but in the real world, you're unlikely to have a dataset as large as the ImageNet dataset, which contains over 1 million images. This is where transfer learning comes in.
You might like: Random Shuffle Dataset Python Huggingface
You might have the data, but not the compute resources to train a model on it. For example, training a model on a large dataset like ImageNet requires a lot of resources and time, which can take days or weeks. This is where pre-trained models can save you precious time.
Here are the key reasons to use transfer learning:
- Training models with high accuracy requires a lot of data.
- You might not have the compute resources needed to train models on huge datasets.
- Even with the resources, you still have to wait for days or weeks to train such a model.
Why Use?
Transfer learning is a game-changer when you're working with limited data. You can train a model from scratch, but it's likely to overfit horribly with just 100 images of cats and dogs.
Training models with high accuracy requires a lot of data, like the ImageNet dataset with over 1 million images. You're unlikely to have such a large dataset in the real world.
Using transfer learning saves you precious time, even if you have the compute resources to train a model on a huge dataset. It can take days or weeks to train such a model.
Here are the main reasons why you want to use transfer learning:
- Training models with high accuracy requires a lot of data.
- You might not have the compute resources needed to train models on huge datasets.
- Even with compute resources, training a model on a huge dataset takes a long time.
When Doesn't Work
Transfer learning won't work when the high-level features learned by the bottom layers aren't sufficient to differentiate the classes in your problem. For example, a pre-trained model may be great at identifying a door but not whether it's closed or open.
You'll need to use the low-level features instead of the high-level features in this case, which means you'll have to retrain more layers of the model or use features from earlier layers.
When datasets are not similar, features transfer poorly. This can be a challenge, especially if you're trying to adapt a pre-trained model to a completely new domain.
Removing some layers from the pre-trained model can also make transfer learning unlikely to work. This is because it reduces the number of trainable parameters, which can result in overfitting.
Discover more: Hidden Layers
How to Implement Transfer Learning
To implement transfer learning, you need to create a base model from a pre-trained architecture such as ResNet or Xception.
You can download the pre-trained weights, but if you don't, you'll have to train the model from scratch. The base model will usually have more units in the final output layer than you require, so you'll need to remove it.
Freezing the base model layers is crucial, especially if they contain BatchNormalization layers, as updating them will destroy what the model has already learned.
How to Implement?
Implementing transfer learning involves several key steps. The first step is to instantiate a base model using a pre-trained architecture such as ResNet or Xception. You can also download the pre-trained weights for the model.
The next step is to remove the final output layer of the base model, as it will not be compatible with your problem. This is because the base model is pre-trained on a different task and has more units in the final output layer than you require.
To do this, you can use the `include_top=False` argument when creating the base model. This will exclude the top layers of the model, including the final output layer.
Once you have created the base model, you can freeze its layers so that they are not updated during the training process. This is important because the base model has already learned the features of the pre-training task, and you want to preserve this knowledge.
You can freeze the layers by setting the `trainable` attribute to `False`. This will prevent the weights in those layers from being updated during training.
Next, you need to add new trainable layers that will turn the old features into predictions on the new dataset. This is important because the pre-trained model is loaded without the final output layer.
You can add these new layers as needed, but most importantly, you need to define a final dense layer with units corresponding to the number of outputs expected by your model.
Here's a summary of the steps involved in implementing transfer learning:
By following these steps, you can successfully implement transfer learning and adapt a pre-trained model to your specific problem.
Using GloVe Embeddings
Using GloVe Embeddings is a crucial step in implementing transfer learning for natural language processing. You can download pre-trained GloVe embeddings, which have already been trained on a massive corpus of text.
To get started, you'll need to extract the pre-trained embeddings into a temporary folder. This will give you access to the word vectors that you can use to create your own embedding layer.
You can load the Glove embeddings and append them to a dictionary, which will serve as a lookup table for your word vectors. This dictionary will help you create an embedding matrix for each word in your training set.
The embedding vector for a word like "bakery" will be represented by a specific vector, and if a word isn't found in the dictionary, it will be represented by a zero vector.
To create the model, you can use this embedding layer, which will allow your model to learn from the pre-trained word vectors. Bidirectional LSTMs are often used to ensure that information is passed both forward and backward.
Here are a few popular pre-trained word embeddings you can consider:
- GloVe(Global Vectors for Word Representation) by Stanford
- Google’s Word2vec trained on around 1000 billion words from Google News
- Fasttext English vectors
Using Pretrained Models
Using pre-trained models can save you a lot of time and effort in your TensorFlow transfer learning projects. You can leverage a pre-trained model from TensorFlow trained on similar tasks, which is beneficial when you don't have enough annotated data to train from scratch.
To use a pre-trained model, you need to ensure the model's input size matches the original training conditions for effective transfer. If your input size doesn't match, you'll need to add a step to resize your input to the required size.
The number of layers to reuse and retrain depends on the task at hand. You can reuse the early and central layers, while only retraining the latter layers, which is a common approach in transfer learning in CNN.
A unique perspective: Confusion Matrix Tensorflow
Pretrained Models in Keras
Keras offers a wide range of pre-trained models that can be used for transfer learning. These models are trained on large datasets and can be fine-tuned for specific tasks.
There are more than two dozen pre-trained models available from Keras, which are served via Keras applications. You can download these models and use them for image tasks.
Each pre-trained model comes with pre-trained weights that are downloaded automatically when you download the model. These weights are stored in `~/.keras/models/`.
You can initialize the MobileNet architecture trained on ImageNet, which is just one example of the many pre-trained models available.
The number of layers to reuse and retrain is determined by the task, so it's essential to research and choose the right model for your specific needs.
Additional reading: Transfer Learning Keras
Pretrained Word Embeddings
Pretrained word embeddings are a game-changer for natural language processing problems. They can save you a ton of time and computational resources.
You can consider using pre-trained word embeddings like GloVe, Google's Word2vec, or Fasttext English vectors. These are all well-established options that have been trained on massive datasets.
GloVe, for example, was trained on a dataset of 6 billion words, while Google's Word2vec was trained on around 100 billion words from Google News. That's a lot of text!
Using pre-trained word embeddings can give you a significant boost in performance, especially if you're working with limited data.
Expand your knowledge: Generative Ai Human Creativity and Art Google Scholar
Image Classification with Transfer Learning
Image classification with transfer learning is a powerful technique that can be used when you don't have enough data to train a model from scratch. By leveraging pre-trained models from TensorFlow, you can adapt them to your specific task and achieve good results.
You can use pre-trained models to solve image and text problems, and the process involves six steps specific to transfer learning. One popular pre-trained model for image classification is Xception, trained on the ImageNet dataset, which contains over 1 million images and 1000 classes.
To fine-tune a pre-trained model, you can start by loading the model and then freeze the original layers while making the top layers trainable. This is known as the transfer learning phase, and it's an effective way to adapt the model to your specific task.
Using Random Augmentation
Random augmentation is a technique used to artificially introduce sample diversity in the training dataset. This is crucial for training machine learning models, especially deep learning models, as it helps in improving the model's ability to generalize well to new, unseen data.
By applying random transformations to each image, such as random horizontal flipping or small random rotations, you can expose the model to different aspects of the training data. This helps slow down overfitting.
Random rotation is done in the function build_datasets. This function is designed to enhance the diversity of the training dataset.
Introducing randomness in the augmentations allows each image in the dataset to potentially contribute a unique learning experience, reducing the model's chance of overfitting. By doing so, you can improve the model's ability to generalize well to new, unseen data.
Random data augmentation is a good practice to follow when you don't have a large image dataset. It helps introduce sample diversity in the training images, making the model more robust and less prone to overfitting.
Curious to learn more? Check out: Conditional Random Fields
Image
Image classification with transfer learning is a powerful technique that allows you to leverage pre-trained models to solve image classification problems. You can use pre-trained models like Xception, trained on the ImageNet dataset, to classify your favorite pets, such as cats and dogs.
You might enjoy: Binary Classifier
The first step is to convert your images to RGB format using a function like _convert_to_rgb, which ensures that the input grayscale images have an explicit channel dimension and duplicates the grayscale values across the three required RGB channels.
To prepare and organize your image data, you can use the build_datasets function, which creates TensorFlow datasets from the provided tensors of training and testing images and labels, shuffles both the training and test datasets, and separates a portion of the training dataset to form a validation dataset.
The build_datasets function also incorporates data augmentation, which involves operations like random rotations to help the model generalize better by training on a wider variety of data. This is especially useful when you don't have enough data to train a model from scratch.
You can use a pre-trained model like ResNet50, which was trained on the ImageNet dataset, to classify new images. ImageNet is an extensive collection of images that have been used to train models, including ResNet50, with over 1 million images and 1000 classes in this dataset.
Here's a summary of the steps involved in using a pre-trained model for image classification:
- Convert your images to RGB format
- Prepare and organize your image data using the build_datasets function
- Use a pre-trained model like ResNet50 to classify new images
By following these steps, you can leverage the power of transfer learning to solve image classification problems with ease.
Training and Evaluation
Training a model from scratch can be a daunting task, especially when dealing with limited data. You can train a model on a related task with ample data and transfer the learned model to solve your original problem.
TensorFlow is capable of utilizing all 32 cores of a computer at an average of more than 80% capacity, making it an efficient tool for training deep neural networks. The high level of development maturity of TensorFlow enables it to handle complex tasks with ease.
To evaluate a model's performance, it's essential to test it on a validation set. This step can help you identify areas where the model needs improvement and fine-tune it accordingly.
Training Phase with Fashion and Cifar
During the training phase, TensorFlow can utilize all 32 cores of the computer, averaging over 80% capacity. This is due to the high level of development maturity of TensorFlow.
The images used for training are often difficult to classify, even for a human observer. This highlights the complexity of the task.
TensorFlow is capable of visualizing predictions of test data after a certain number of epochs. In one example, after 15 epochs, the model's accuracy and loss were still not satisfactory.
A retraining session with a reduced learning rate can improve the model's accuracy and loss. In one case, after retraining for 5 epochs, only one image was not recognized correctly.
Evaluating the
Evaluating the model is a crucial step in the training process.
You can test the model's performance on the validation set once it's fine-tuned.
The validation accuracy starts at a high value because you're using a pre-trained model.
To test the model, you'll want to take a sample question-context pair and tokenize it.
The fine-tuned model can then generate an answer to the question.
The tokenizer decodes the output back into human-readable text so you can see the answer.
Related reading: Transfer Learning vs Fine Tuning
Customizing Transfer Learning
You can use a pre-trained model to classify your favorite pets, cats and dogs, even with a small dataset.
The Xception architecture, trained on the ImageNet dataset, is a good choice for this task.
To apply transfer learning properly, you should follow the steps specific to transfer learning, which include selecting a pre-trained model and training it with Keras.
You can start training the model with Keras once you've chosen your pre-trained model, such as the Xception architecture.
Fine-tuning the model is an essential step in the process, allowing you to adapt the pre-trained model to your specific task.
Broaden your view: Mlops Architecture
Improving Transfer Learning
Fine-tuning is an optional step in transfer learning that can improve the performance of your model, but it can also lead to overfitting if not done properly.
To avoid overfitting, you can retrain the model or part of it using a low learning rate. This will prevent significant updates to the gradient, which can result in poor performance.
A low learning rate is especially important when working with large models and small datasets, as it can help prevent overfitting.
Curious to learn more? Check out: High Bias Low Variance
There are several ways to further improve the performance of your model through fine-tuning, including data augmentation, use of transfer learning techniques, optimization, experimenting with hyperparameters, and leveraging TPUs or multi-GPU training.
Here are some specific techniques you can try:
- Data Augmentation: Paraphrase questions or slightly modify the context to create more training samples.
- Use of Transfer Learning Techniques: Explore other transfer learning techniques like Parameter Efficient Fine-Tuning (PEFT).
- Optimization: Try using more advanced optimizers like AdamW or LAMB for better convergence.
- Experiment with Hyperparameters: Use a small validation set to tune learning rate, number of epochs, and dropout rates.
- Leverage TPUs or Multi-GPU Training: Speed up the training process with large datasets or models.
Ways to Improve
Fine-tuning is an optional step in transfer learning that can improve the performance of your model. However, since you have to retrain the entire model, you'll likely overfit.
To avoid overfitting, retrain the model or part of it using a low learning rate. This prevents significant updates to the gradient, which can result in poor performance.
Using a callback to stop the training process when the model has stopped improving is also helpful. This ensures that the model doesn't continue to make updates that can lead to overfitting.
There are several ways you can further improve the performance of your model:
- Data Augmentation: This involves using techniques like paraphrasing questions or slightly modifying the context to create more training samples.
- Transfer Learning Techniques: Explore other techniques like Parameter Efficient Fine-Tuning (PEFT), which allows fine-tuning of smaller subsets of the model's parameters.
- Optimization: Try using more advanced optimizers like AdamW or LAMB for better convergence.
- Experiment with Hyperparameters: You can experiment with hyperparameters like learning rate, number of epochs, and dropout rates.
- Leverage TPUs or Multi-GPU Training: If you're working with a large dataset or model, consider using TPUs or multiple GPUs to speed up the training process.
By implementing these strategies, you can improve the performance of your transfer learning model and achieve better results in your project.
Benefits
Transfer learning offers several benefits that make it an attractive approach for many applications.
One of the main advantages is that it significantly reduces training time, often by days or even weeks.
Training a neural model from scratch can be a lengthy process, but transfer learning leverages pre-trained models to achieve strong performance with limited training data.
This is particularly useful in fields like natural language processing, where vast labeled datasets are available.
Transfer learning in CNN also enhances neural network performance in many cases, making it a valuable tool for improving model accuracy.
With transfer learning, you can work effectively with limited data, which is a game-changer in many real-world applications.
Check this out: Hidden Layers in Neural Networks Code Examples Tensorflow
Frequently Asked Questions
Which model is best for transfer learning?
For transfer learning, the VGG Family is a popular choice due to its simplicity and robustness, making it a great starting point for many applications. However, the ResNet model is often preferred for its ability to handle complex tasks and large datasets.
What is the difference between finetuning and transfer learning?
Transfer learning uses a pre-trained model's knowledge on a large dataset, while fine-tuning allows the model to learn specific features from a larger dataset
What is the difference between transfer learning and Pretrained model?
Transfer learning fine-tunes a pre-trained model on a new task, whereas a pre-trained model is used as-is when the new task is similar to the original one. The key difference lies in how the model is adapted to the new task
Sources
- https://neptune.ai/blog/transfer-learning-guide-examples-for-images-and-text-in-keras
- https://www.analyticsvidhya.com/blog/2021/10/understanding-transfer-learning-for-deep-learning/
- https://medium.com/@alfred.weirich/transfer-learning-with-keras-tensorflow-an-introduction-51d2766c30ca
- https://tensorflow.rstudio.com/guides/keras/transfer_learning
- https://www.pluralsight.com/resources/blog/ai-and-data/llms-tensorflow-keras-hugging-face
Featured Images: pexels.com