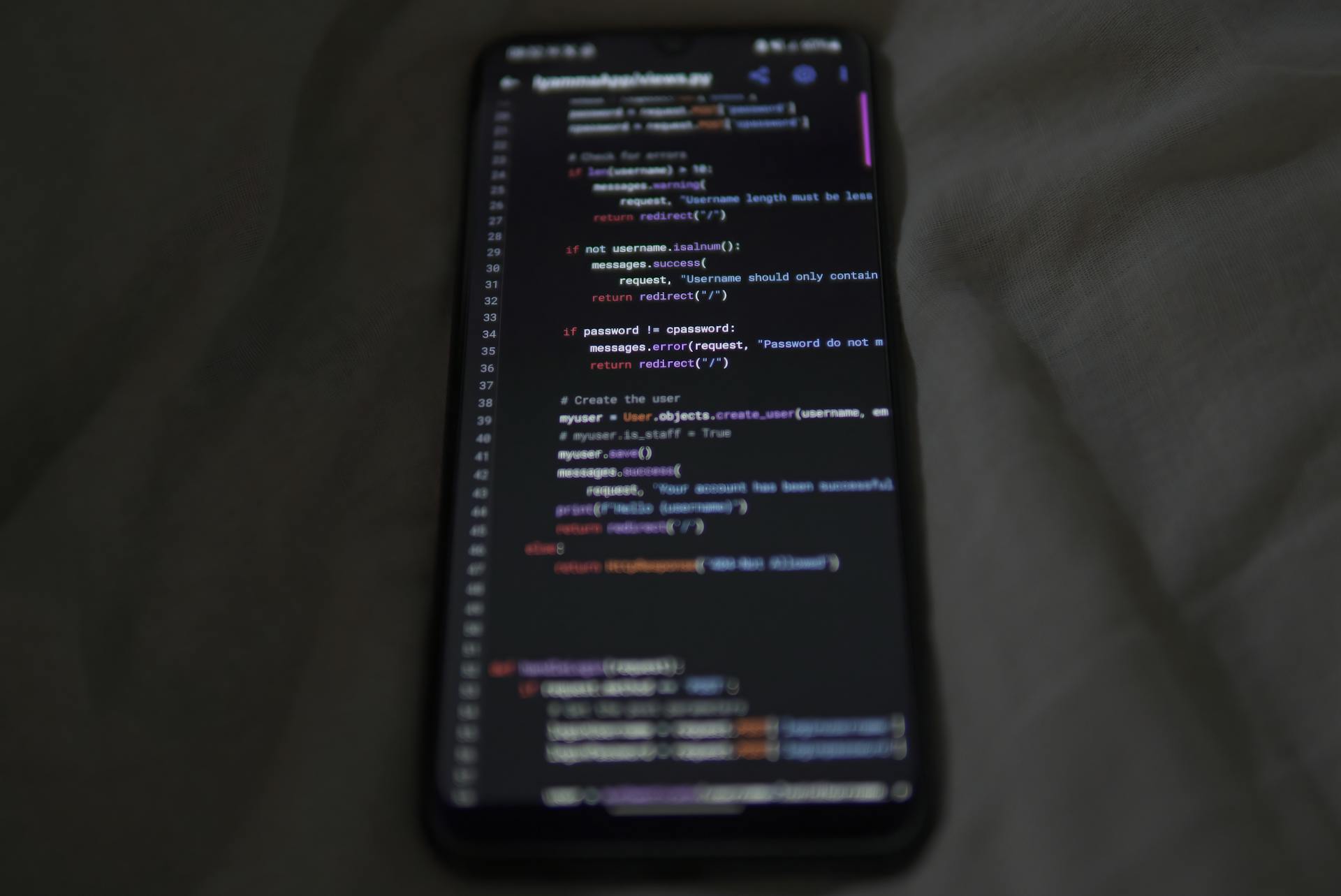
Random shuffle dataset is a crucial step in machine learning, ensuring that data is properly mixed up and representative of the real world. This helps prevent overfitting and improves model performance.
To achieve this in Python using Hugging Face, you'll need to import the necessary libraries, including transformers and datasets. Specifically, you'll want to use the Dataset class from datasets, which provides a simple way to load and manipulate datasets.
The Dataset class is the core of the Hugging Face library, allowing you to load and preprocess data for your machine learning model. By using this class, you can easily load datasets from various sources, including CSV files and online repositories.
A fresh viewpoint: Dataset Huggingface Modify Class Label
Data Preparation
Loading data from a dataset can be a challenge, especially when you want to use Keras methods like fit() and predict(). You can load individual samples and batches just by indexing into your dataset, but this won't work.
Instead, you can convert your dataset to a tf.data.Dataset using the to_tf_dataset() method. This class covers a wide range of use-cases and can be created from Tensors in memory or using a load function to read files on disc or external storage.
If this caught your attention, see: How to Use Huggingface Model in Python
The tf.data.Dataset class allows you to transform your data arbitrarily with the map() method, or methods like batch() and shuffle() can be used to create a dataset that's ready for training. These methods don't modify the stored data in any way.
The entire data preprocessing pipeline can be compiled in a tf.data.Dataset, which allows for massively parallel, asynchronous data loading and training. However, graph compilation can be a limitation, particularly for Hugging Face tokenizers.
To get around this limitation, you can pre-process the dataset as a Hugging Face dataset, where arbitrary Python functions can be used, and then convert to tf.data.Dataset afterwards using to_tf_dataset() to get a batched dataset ready for training.
On a similar theme: Proximal Gradient Methods for Learning
Shuffling the Dataset
Shuffling the Dataset is a crucial step in machine learning, and Hugging Face's datasets library makes it easy to do so. You can shuffle your dataset using the datasets.IterableDataset.shuffle() method, which fills a buffer of size buffer_size and randomly samples examples from this buffer.
To achieve perfect shuffling, you need to set buffer_size to be greater than the size of your dataset. However, this will download the full dataset in the buffer, which may not be feasible for large datasets.
Here are some key points to keep in mind when shuffling your dataset:
- Setting buffer_size to be greater than the size of your dataset will download the full dataset in the buffer.
- For larger datasets that are sharded into multiple files, datasets.IterableDataset.shuffle() also shuffles the order of the shards.
You can also split your dataset by taking or skipping the first n examples using datasets.IterableDataset.take() or datasets.IterableDataset.skip(). However, keep in mind that using take or skip prevents future calls to shuffle from shuffling the dataset shards order.
Split Your Dataset
You can split your dataset using the `take` and `skip` methods.
Using `take` creates a new dataset with the first n examples, while `skip` creates a dataset with the rest of the examples. This is done using `datasets.IterableDataset.take()` and `datasets.IterableDataset.skip()` respectively.
Iterating on a new dataset created with `skip` will take some time to start, as it has to iterate over the skipped examples first.
To prevent future calls to `shuffle` from shuffling the dataset shards order, it's advised to shuffle the dataset before splitting using `take` or `skip`.
Here are some key considerations for splitting your dataset:
- Using `take` (or `skip`) prevents future calls to `shuffle` from shuffling the dataset shards order.
- Iterating on a new dataset created with `skip` will take some time to start.
Alternatively, you can use `datasets.Dataset.train_test_split()` to create train and test splits, allowing you to adjust the relative proportions or absolute number of samples in each split.
Shuffling the Dataset
Shuffling the dataset can be a bit tricky, but don't worry, I've got you covered. You can shuffle your dataset using the datasets.IterableDataset.shuffle() method, which fills a buffer of size buffer_size and randomly samples examples from this buffer.
The selected examples in the buffer are replaced by new examples, maintaining the buffer size. For perfect shuffling, you need to set buffer_size to be greater than the size of your dataset, which can be a challenge for large datasets.
In fact, if your dataset is sharded into multiple files, datasets.IterableDataset.shuffle() also shuffles the order of the shards. This is a nice feature that ensures the entire dataset is shuffled, not just the individual shards.
However, if you've already fixed the order of the shards using datasets.IterableDataset.skip() or datasets.IterableDataset.take(), the order of the shards will be kept unchanged.
To reshuffle the dataset at each epoch, you can specify a different seed each time you shuffle. For example, if you're using datasets.IterableDataset.shuffle() with a seed, you can use an effective seed of seed+epoch to shuffle the dataset differently each time.
Here are some key things to keep in mind when shuffling your dataset:
- Shuffle buffer size: Set buffer_size to be greater than the size of your dataset for perfect shuffling.
- Shard order: datasets.IterableDataset.shuffle() shuffles the order of the shards, unless you've fixed the order using skip() or take().
- Reshuffling: Use an effective seed of seed+epoch to shuffle the dataset differently each time.
- Splitting: Shuffle the dataset before splitting using take() or skip() to prevent shuffling the shard order.
By following these tips, you'll be able to shuffle your dataset like a pro and get the most out of your machine learning models!
Data Augmentation and Manipulation
Data augmentation is a powerful technique that can be applied to your dataset using batch processing. You can even generate additional examples by augmenting your dataset with new words for a masked token in a sentence.
To do this, you can load the RoBERTA model through the 🤗 Transformer FillMaskPipeline, which allows you to generate top replacements for a masked word. This model can be used to randomly select a word to mask in the sentence and return the original sentence along with the top two replacements.
By applying the function over the whole dataset using datasets.Dataset.map(), you can augment each original sentence with three alternatives, effectively increasing the size of your dataset. For example, in the first sentence, the word "distorting" is augmented with "withholding", "suppressing", and "destroying".
Readers also liked: Top Machine Learning Applications at Fin Tech Companies
Sort, Select, Split, Shard
Sorting a dataset can be a crucial step in data manipulation, allowing you to rearrange the structure of your data in a more organized way.
You can sort a dataset by using various methods, such as sorting by a specific column or in a specific order.
Shuffling a dataset can be useful for creating a random order, which can be helpful when you need to train a machine learning model on a dataset that's too large to fit in memory.
Shuffling is especially useful when you're working with very large datasets that need to be split into smaller chunks.
Splitting a dataset is another important step in data manipulation, allowing you to create separate train and test splits for your machine learning model.
Sharding a dataset can be useful for dividing a very large dataset into smaller, more manageable chunks.
By sharding your dataset, you can make it easier to work with and analyze, especially when you're dealing with extremely large datasets.
Data Augmentation
Data augmentation can be a powerful tool to increase the size of your dataset, making it easier to train accurate models. By using batch processing, you can generate additional examples, like replacing a word in a sentence with three alternative options.
With the RoBERTA model, you can even augment your dataset with additional examples, such as generating top two replacements for a masked token in a sentence. This can be achieved by using the 🤗 Transformer FillMaskPipeline.
You can create a function to randomly select a word to mask in the sentence, and use datasets.Dataset.map() to apply the function over the whole dataset. For example, RoBERTA can augment a random word with three alternatives, like the word "distorting" in the first sentence, which is replaced with "withholding", "suppressing", and "destroying".
Saving and Exporting
Saving and Exporting your Dataset is a crucial step in working with Hugging Face's Datasets library. You can save your dataset by providing the path to the directory you wish to save it to.
Check this out: Huggingface save Model
After saving your dataset, you can easily reload it later using datasets.load_from_disk(). I've found this to be super convenient when working on multiple projects simultaneously.
Datasets supports exporting as well, so you can work with your dataset in other applications. Here are some supported file formats you can export to:
Exporting your dataset to a CSV file is as simple as calling datasets.Dataset.to_csv() with the desired file path.
Hugging Face Hub and Data Loading
You can load datasets from the Hugging Face Hub without a loading script by creating a dataset repository and uploading your data files. This allows you to load datasets directly from the Hub using the load_dataset() function.
To load a dataset from the Hub, you need to provide the repository namespace and dataset name. For example, you can load a dataset from a demo repository by providing the namespace and dataset name. Some datasets may have multiple versions based on Git tags, branches, or commits, and you can specify the version you want to load using the revision parameter.
Recommended read: Huggingface Load Model from S3
You can also use the data_files parameter to map data files to specific splits like train, validation, and test, or use the split parameter to map a data file to a specific split. This can be useful if you don't want to load the entire dataset at once, as loading a large dataset like C4 can take a long time.
Take a look at this: How to Use Hugging Face Models
Hugging Face Hub
You can load a dataset from the Hugging Face Hub without a loading script by creating a dataset repository and uploading your data files. This allows you to use the load_dataset() function to load the dataset directly.
To load a dataset from the Hub, you need to provide the repository namespace and dataset name. For example, you can load the files from a demo repository.
Datasets on the Hub may have multiple versions based on Git tags, branches, or commits. You can specify the dataset version you want to load using the revision parameter.
If you don't specify which data files to use, load_dataset() will return all the data files, which can take a long time for large datasets like C4, which is approximately 13TB of data.
You can also load a specific subset of the files with the data_files or data_dir parameter, which can accept a relative path that resolves to the base path corresponding to where the dataset is loaded from.
Data Loading
Data loading can be a challenge, especially when working with large datasets. You can load individual samples and batches just by indexing into your dataset, but this won't work if you want to use Keras methods like fit() and predict().
Loading individual samples and batches can be cumbersome and time-consuming. You could write a generator function that shuffles and loads batches from your dataset, but that sounds like a lot of unnecessary work.
Instead, we recommend converting your dataset to a tf.data.Dataset using the to_tf_dataset() method. This allows you to stream data from your dataset on-the-fly and use Keras methods like fit() and predict().
Here's an interesting read: Transfer Learning Keras
The tf.data.Dataset class is incredibly versatile and can be created from Tensors in memory or using a load function to read files on disc or external storage. It can also be transformed arbitrarily with the map() method, or methods like batch() and shuffle() can be used to create a dataset that's ready for training.
Massively parallel, asynchronous data loading and training are possible with tf.data.Dataset, but the requirement for graph compilation can be a limitation. This is particularly true for Hugging Face tokenizers, which are usually not (yet!) compilable as part of a TF graph.
Frequently Asked Questions
What is a dataset map?
A dataset map is a process that prepares input data for a model by applying tokenization functions, typically from the Transformers library. This step is crucial for effective model performance and accurate results.
Featured Images: pexels.com