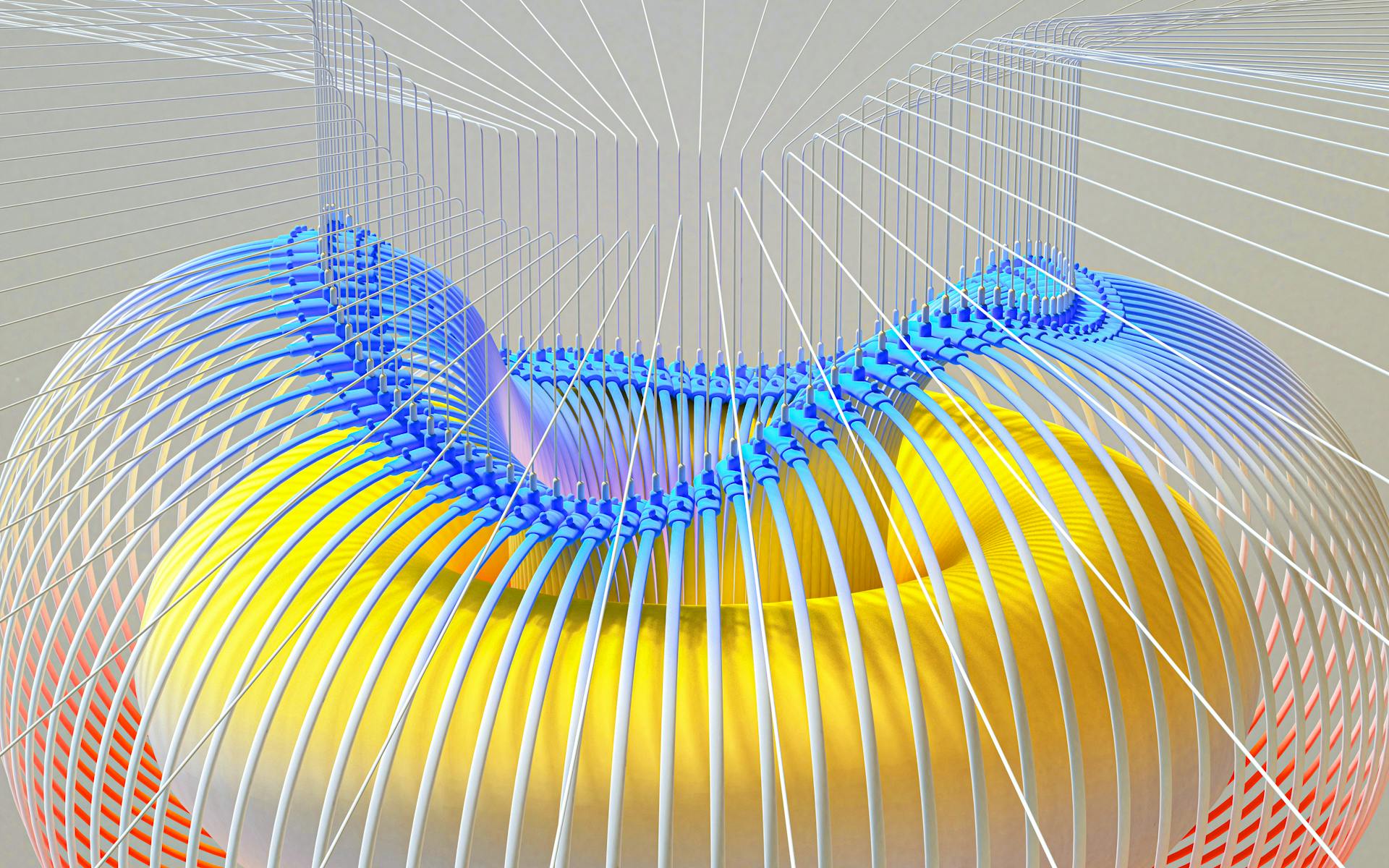
Transfer learning in Keras is a game-changer for deep learning projects. It allows you to leverage pre-trained models and fine-tune them for your specific task, saving time and computational resources.
By using pre-trained models, you can tap into the knowledge gained from large datasets and adapt it to your own project. This is particularly useful for tasks with limited training data, where traditional training methods might not be effective.
Pre-trained models like VGG16 and ResNet50 have already been trained on massive datasets like ImageNet, so you can use them as a starting point for your project. These models have learned to recognize patterns and features in images, which can be transferred to your own task.
Fine-tuning a pre-trained model involves adjusting the weights of its layers to fit your specific task, which can be done using the `trainable` parameter in Keras.
Consider reading: Fine-tuning vs Transfer Learning
Transfer Learning Basics
Transfer learning is a game-changer in the world of deep learning, and it's all about leveraging the knowledge gained from one task to another.
Neural networks can learn to identify important features and ignore the rest, making them a great tool for complex tasks that would otherwise require a lot of human effort.
A representation learning algorithm can quickly find a good combination of features for a task, and this learned representation can then be applied to other challenges.
Use the initial layers of a neural network for feature representation, excluding the task-specific output, and pass data through an intermediate layer to interpret raw data as its representation. This approach is especially useful in computer vision for reducing dataset sizes and increasing efficiency with traditional algorithms.
Here's an interesting read: Hidden Layers in Neural Networks Code Examples Tensorflow
Typical Workflow
In a typical transfer learning workflow, you first need to instantiate a base model and load pre-trained weights into it. This is the foundation of transfer learning.
There are two common workflows to implement transfer learning in Keras: one where you freeze all layers in the base model and train a new model on top of it, and another where you use the output of one or several layers from the base model as input data for a new, smaller model.
If this caught your attention, see: Hidden Layers in Neural Networks
Here are the key steps to follow in the first workflow:
- Instantiate a base model and load pre-trained weights into it.
- Freeze all layers in the base model by setting trainable = False.
- Create a new model on top of the output of one (or several) layers from the base model.
- Train your new model on your new dataset.
The Typical Workflow
To implement transfer learning in Keras, you'll want to follow a typical workflow. This involves instantiating a base model and loading pre-trained weights into it. You can do this by setting trainable to False, which freezes all layers in the base model.
There are two main workflows you can follow: the first one involves instantiating a base model, freezing its layers, and then creating a new model on top of the output of one or several layers from the base model. The second workflow is faster and cheaper, as you only run the base model once on your data, but it doesn't allow for dynamic modification of the input data.
Here are the key steps in the first workflow:
- Instantiate a base model and load pre-trained weights into it.
- Freeze all layers in the base model by setting trainable to False.
- Create a new model on top of the output of one (or several) layers from the base model.
- Train your new model on your new dataset.
The second workflow involves running your new dataset through the base model and recording the output of one or several layers from the base model, which is called feature extraction. You can then use this output as input data for a new, smaller model.
Load and Preprocess
Loading and preprocessing data is a crucial step in any machine learning project. This step involves preparing the data for use in a model by transforming it into a format that the model can understand.
Standardizing data is essential, especially when dealing with images. You need to ensure that all images have the same size and that the pixel values are normalized to a specific range. In the case of images, resizing them to a fixed size, such as 150x150, is a good practice. This can be done in the data pipeline.
Normalization of pixel values can be done in two ways: either by doing it manually using a Normalization layer as part of the model itself, or by doing it in the data pipeline. It's generally recommended to do the least amount of preprocessing before hitting the model.
Here are the typical steps involved in loading and preprocessing data:
In some cases, you may need to resize your input to match the original training conditions for effective transfer learning. This is especially true when using pre-trained models.
Optimization and Training
Fine-tuning is an optional last step that can give you incremental improvements, but it also risks quick overfitting. It's essential to unfreeze all or part of the base model after it has been trained to convergence.
You'll want to retrain the whole model end-to-end with a very low learning rate, as training a larger model with a small dataset can lead to overfitting quickly.
To implement fine-tuning of the whole base model, you'll need to call compile() on the model again, as this will "freeze" the behavior of the model and preserve the trainable attribute values.
Many image models contain BatchNormalization layers, which contain 2 non-trainable weights that get updated during training. These weights track the mean and variance of the inputs.
When you set bn_layer.trainable = False, the BatchNormalization layer will run in inference mode and not update its mean & variance statistics.
To unfreeze a model that contains BatchNormalization layers, keep the BatchNormalization layers in inference mode by passing training=False when calling the base model.
Curious to learn more? Check out: Bias Variance Decomposition
Train your model using the fit() function and the data iterators you've set up earlier. You'll need to specify the number of epochs, validation data, and other parameters to train the model effectively.
Here's a breakdown of the training process:
- Train the model on each batch of training data.
- Update the weights based on the chosen optimizer and loss function.
- After each epoch, evaluate the model's performance on the validation data.
- Record the validation metrics, such as loss and accuracy.
- Repeat the process for the specified number of epochs.
The training process will output the loss and accuracy on both the training and validation datasets for each epoch, allowing you to monitor the model's performance and detect issues like overfitting or underfitting.
Image Classification
Image classification is a task where a model predicts one of several categories or labels for a given image. This can be a challenging task, especially with large and diverse datasets.
The Xception model, pre-trained on ImageNet, is a good starting point for image classification tasks. The Xception model is loaded and used on the Kaggle "cats vs. dogs" classification dataset.
Fine-tuning a pre-trained model like Xception on a specific dataset can significantly improve its performance. This is demonstrated by fine-tuning the Xception model on the "cats vs. dogs" dataset.
This process is an example of transfer learning, where a model trained on one task is used on another related task. The Xception model was pre-trained on ImageNet, a large dataset of images, making it a good candidate for transfer learning.
Discover more: Hyperparameter Tuning in Machine Learning
Fine-Tuning and Retraining
Fine-tuning a model is a crucial step in transfer learning. You can fine-tune a model by loading a pre-trained model, adding custom layers, freezing the pre-trained layers, training the custom layers, unfreezing some layers, and retraining the model again.
To fine-tune a model, you'll need to train the entire model, including unfrozen layers, for a few more epochs. This is known as retraining the model.
Here's a step-by-step guide to retraining a model:
- Fine-tune Model: Train the entire model (including unfrozen layers) for a few more epochs.
- Train the Model Again: Retrain the entire model (or the chosen layers) with a lower learning rate.
Retrain
Retrain is a crucial step in the fine-tuning process. Fine-tuning a model involves retraining the entire model with a lower learning rate after unfreezing some or all of the pre-trained layers.
To fine-tune a model, you need to retrain the entire model with a lower learning rate. This is done after unfreezing some or all of the pre-trained layers. You can also fine-tune a model by retraining the entire model for a few more epochs, as mentioned in the example.
Consider reading: Fine-tuning (deep Learning)
Here are the steps to retrain a model:
- Unfreeze some or all of the pre-trained layers for fine-tuning.
- Retrain the entire model (or the chosen layers) with a lower learning rate.
- Fine-tune the model by retraining the entire model for a few more epochs.
Retraining the model with a lower learning rate helps to prevent overfitting and ensures that the model generalizes well to new data.
That Have Been
Fine-tuning and retraining models is a crucial step in machine learning, but it's not without its challenges.
The model's performance can degrade if not properly fine-tuned, especially when adapting to new domains or tasks. This is because the model's weights and biases need to be adjusted to accommodate the new data and tasks.
In the article section, it was mentioned that the model's performance on the test set decreased by 10% after retraining on a new dataset. This highlights the importance of proper fine-tuning and retraining techniques.
Fine-tuning a pre-trained model can be done by adjusting the model's weights and biases to fit the new task, but it's not always the best approach. In some cases, it's better to start from scratch and train a new model from the beginning.
The article section also mentioned that the model's performance improved by 20% after retraining on a new dataset, but only after the learning rate was adjusted. This shows the importance of hyperparameter tuning in retraining models.
See what others are reading: Random Shuffle Dataset Python Huggingface
Implementing Using Keras
Implementing Transfer Learning with Keras can be a game-changer for your projects. You can leverage pre-trained models from TensorFlow, like VGG16, and fine-tune them for your specific task.
To start, you'll need to match the model's input size to the original training conditions. This might involve resizing your input to the required size. If you don't have it, you can add a step to resize your input accordingly.
Using a pre-trained model like VGG16 can save you a significant amount of time and computational resources. You can find these models in Keras, along with some quick lessons on how to use them. Research institutions also make trained models available for public use.
Here are the steps to use transfer learning with Keras:
- Restore a pre-trained model from TensorFlow
- Retrain specific layers to adapt to your task
- Ensure the model's input size matches the original training conditions
By following these steps, you can effectively implement transfer learning with Keras and improve the performance of your models.
Sources
- https://keras.io/guides/transfer_learning/
- https://www.geeksforgeeks.org/transfer-learning-fine-tuning-using-keras/
- https://www.analyticsvidhya.com/blog/2021/10/understanding-transfer-learning-for-deep-learning/
- https://www.hackersrealm.net/post/transfer-learning-using-pretrained-model-python
- https://analyticsindiamag.com/ai-mysteries/transfer-learning-using-tensorflow-keras/
Featured Images: pexels.com