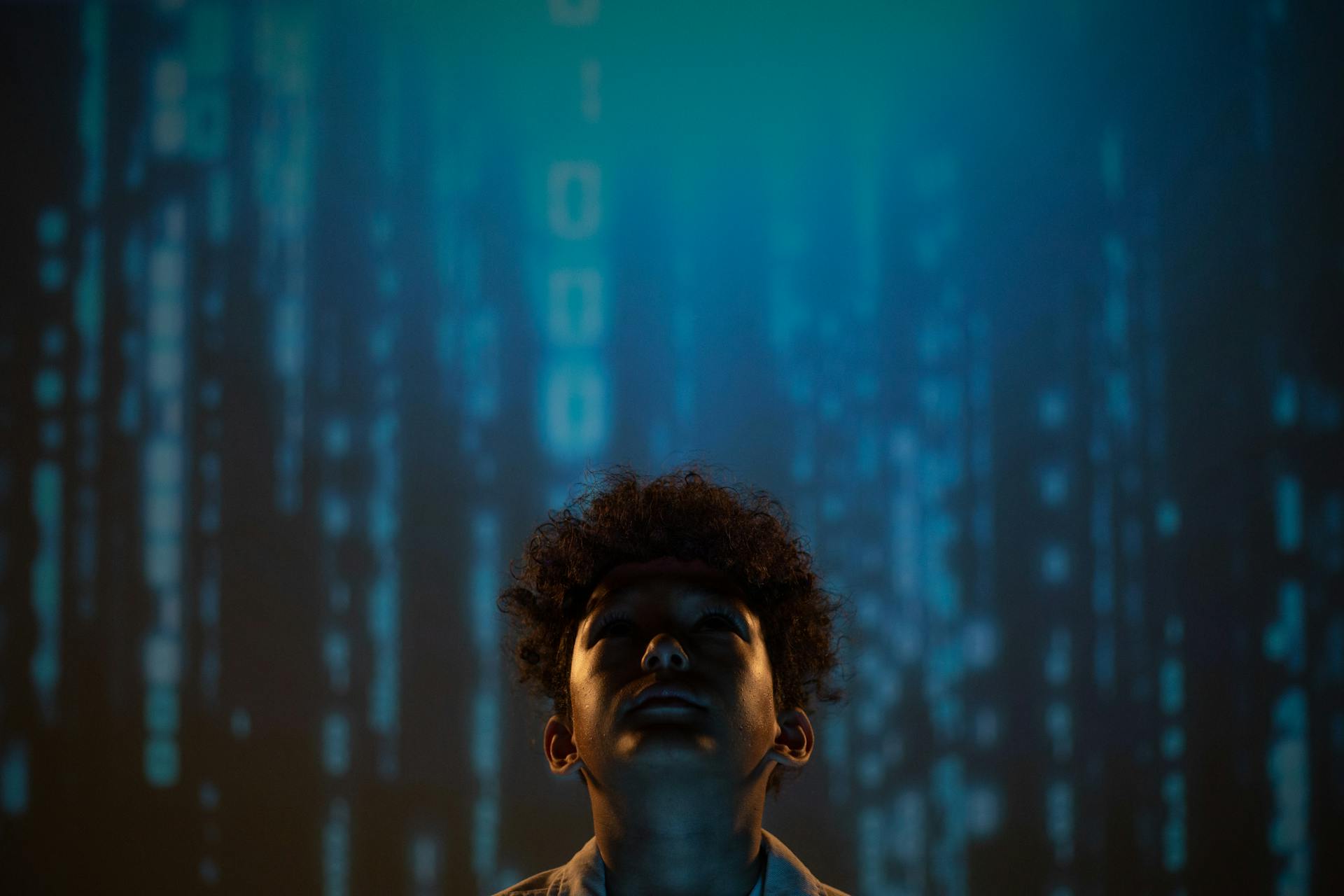
A confusion matrix is a table used to describe the performance of a classification model, and it's a crucial tool in machine learning. It's essentially a summary of how well our model is doing on a specific task.
In a confusion matrix, each row represents a true class, and each column represents a predicted class. This helps us visualize where our model is going wrong. For example, if we're building a model to classify images as either cats or dogs, the confusion matrix will have two rows (one for cats and one for dogs) and two columns (one for cats and one for dogs).
The accuracy of a model is calculated by dividing the number of correct predictions by the total number of predictions. But, as we'll see later, accuracy isn't the only metric we should be looking at.
Check this out: Calculate Accuracy from Confusion Matrix
What Is a Confusion Matrix
A confusion matrix is a table used to evaluate the performance of a classification model.
It compares the actual class labels with the predicted class labels and displays the number of true positives, false positives, true negatives, and false negatives.
The matrix is typically a square table with the actual class labels on one axis and the predicted class labels on the other.
In a binary classification problem, the matrix would have two rows and two columns, representing the positive and negative classes.
The diagonal elements of the matrix represent the number of correct predictions, while the off-diagonal elements represent the number of incorrect predictions.
The confusion matrix is a useful tool for understanding the strengths and weaknesses of a classification model.
Take a look at this: Elements of Statistical Learning Solutions
Type 1 and Type 2 Errors
Type 1 and Type 2 errors are essential concepts in machine learning, particularly when working with confusion matrices in TensorFlow.
A Type 1 error occurs when we incorrectly predict a positive outcome as negative, which is also known as a false negative from the confusion matrix.
Curious to learn more? Check out: When Should You Use a Confusion Matrix
To better understand the difference between Type 1 and Type 2 errors, let's take a look at the following definitions:
A Type 2 error is essentially the opposite of a Type 1 error, where we predict a negative outcome as positive, which can be a false positive in the context of the confusion matrix.
For another approach, see: Error Singular Matrix
Implementing in TensorFlow
Implementing in TensorFlow is a straightforward process. You can use the code provided for implementing a confusion matrix in sklearn and TensorFlow, along with visualization code.
To get started, you'll need to set up a Tensorflow callback to log the confusion matrix on epoch end. This will allow you to visualize the performance of your model over time.
Here's a breakdown of the components of a 2X2 confusion matrix:
- True Positive (TP)
- False Positive (FP)
- True Negative (TN)
- False Negative (FN)
Implementation with TensorFlow
Implementing a confusion matrix in TensorFlow is a straightforward process. The code for implementing a confusion matrix in TensorFlow is provided in the article section.
You can implement a confusion matrix using TensorFlow by following the code example provided. The code is designed to work with both sklearn and TensorFlow, making it a versatile tool for data analysis.
The confusion matrix is a fundamental tool in data analysis, used to evaluate the performance of machine learning models. It provides a clear and concise way to visualize the accuracy of a model.
Here's a quick rundown of what you can expect from the confusion matrix implementation in TensorFlow:
- Code availability: The code for implementing a confusion matrix in TensorFlow is available in the article section.
- Two implementation methods: The article section provides two different ways to implement a confusion matrix in TensorFlow, using sklearn and TensorFlow separately.
- Visualization code: The article section also includes visualization code to help you understand the results of your confusion matrix.
By following the code example and using the visualization code, you'll be able to effectively implement a confusion matrix in TensorFlow and gain valuable insights into your machine learning model's performance.
Take a look at this: How to Code Binary Classifier in Python
Implementing in TensorFlow
To implement a confusion matrix in TensorFlow, set up a tensorboard callback to log it on epoch end. This will help you visualize the performance of your model over time.
You can refer to the Github repo link provided in the example to see how it's done. The repository is novasush/Tensorboard-with-Fashion-MNIST.
To log a confusion matrix in tensorboard, you will need to use the tensorflow.keras.callbacks.TensorBoard class. This class can be used to log various metrics, including the confusion matrix.
The confusion matrix will be logged on epoch end, allowing you to see how your model is performing as it trains. This can be a valuable tool for identifying areas where your model needs improvement.
Expand your knowledge: Log Trick Reparameterization Trick
Creating a 2x2
Creating a 2x2 confusion matrix involves identifying four key metrics: True Positive (TP), False Positive (FP), True Negative (TN), and False Negative (FN). These metrics are essential for evaluating the performance of a classification model.
A True Positive (TP) occurs when the model correctly predicts the presence of a positive class. Conversely, a False Positive (FP) occurs when the model incorrectly predicts the presence of a positive class when it's actually absent.
A True Negative (TN) occurs when the model correctly predicts the absence of a positive class. On the other hand, a False Negative (FN) occurs when the model incorrectly predicts the absence of a positive class when it's actually present.
Suggestion: Confusion Matrix Metrics
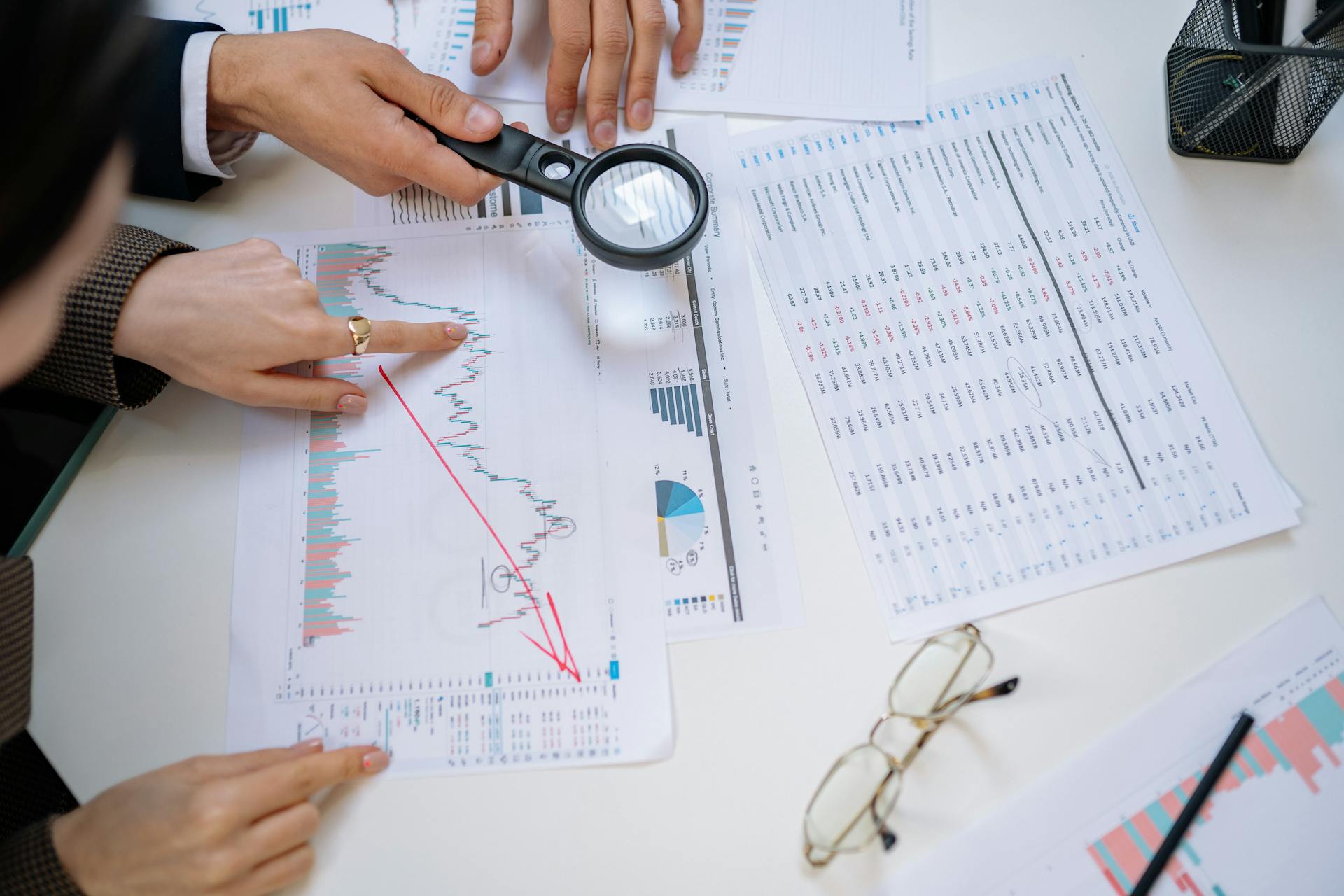
Here's a breakdown of these metrics:
By understanding these metrics, you can create a 2x2 confusion matrix and use it to evaluate the performance of your classification model.
Calculating Metrics
Calculating metrics from a confusion matrix is a crucial step in evaluating the performance of a machine learning model.
To calculate the True Positive (TP) value, you need to look for the cell where the actual value and predicted value are the same, which is cell 1 in the 3×3 confusion matrix for the IRIS dataset.
The False Positive (FP) value is calculated by summing the values of the corresponding column except for the TP value, which means excluding the value in cell 1.
The True Negative (TN) value is calculated by summing the values of all columns and rows except the values of the class being calculated, which is why you need to exclude the values in cells 1, 4, and 7 in the example.
Consider reading: Claude 3 Context Window
Calculating FN, FP, TN, and TP Values
Calculating FN, FP, TN, and TP Values is a crucial step in understanding how well a model is performing.
The False-negative value for a class, FN, is the sum of values of corresponding rows except for the TP value.
You can calculate the True-positive value, TP, by looking for where the actual value and predicted value are the same.
For the class Setosa using the 3×3 confusion matrix, the TP value is the value of cell 1.
The False-positive value, FP, is the sum of values of the corresponding column except for the TP value.
The sum of values of the corresponding column except for the TP value is 3 for the class Setosa.
The True-negative value, TN, is the sum of the values of all columns and rows except the values of that class that we are calculating the values for.
For the class Setosa, TN is the sum of values of all columns and rows except the values of that class, which is (cell 5 + cell 6 + cell 8 + cell 9).
This means you need to add up the values in cells 5, 6, 8, and 9 to get the TN value for the class Setosa.
TN equals 9 for the class Setosa.
Accuracy Metrics
Accuracy Metrics are used for measuring the performance of binary or multi-class classification functions. They provide a way to evaluate how well a model is doing in terms of correct predictions.
Categorical Accuracy measures the percentage of correct predictions when the true labels are one-hot encoded. It compares the index of the highest predicted probability with the index of the true label.
SparseCategoricalAccuracy is similar to Categorical Accuracy but works with integer-encoded labels instead of one-hot encoded labels. This is useful when dealing with a large number of classes to save memory.
TopKCategoricalAccuracy calculates how often the true label is in the top K predictions, where K is a specified number. For example, top 5. It's useful for multi-class problems with many classes.
SparseTopKCategoricalAccuracy is the sparse version of TopKCategoricalAccuracy, working with integer-encoded labels instead of one-hot encoded labels. This makes it more efficient for large-scale problems.
Accuracy metrics give a more complete picture of a model's performance than the F1-score alone, especially when dealing with uneven class distributions.
Expand your knowledge: Top Machine Learning Applications at Fin Tech Companies
Binary Classification
Binary classification is a fundamental concept in machine learning, and it's essential to understand how to work with it. In binary classification, the model predicts one of two classes or outputs.
A confusion matrix is a crucial tool for evaluating the performance of a binary classification model. It's a 2x2 table that summarizes the predictions made by the model against the actual outcomes.
Let's break down the components of a confusion matrix. TP (True Positives) represents the number of actual positive cases that the model predicted correctly. In the example from Example 1, TP is 6 out of 8 actual positive cases.
The number of actual positive cases that the model predicted as negative is represented by FN (False Negatives). In the same example, FN is 2 out of 8 actual positive cases.
FP (False Positives) represents the number of actual negative cases that the model predicted as positive. In the example, FP is 2 out of 7 actual negative cases.
TN (True Negatives) represents the number of actual negative cases that the model predicted correctly. In the example, TN is 1 out of 7 actual negative cases.
Here's a summary of the components of a confusion matrix:
Multi-Class Classification
In multi-class classification, the outputs are greater than 2 classes, which means we have more than two possible outcomes.
The confusion matrix for multi-class classification is a bit more complex than the binary classification matrix, but it still helps us evaluate the performance of our model.
For each class, we need to calculate the true positives (TP), false negatives (FN), false positives (FP), and true negatives (TN) separately. This is because we won't get these values directly as in binary classification.
The sum of values in the corresponding row except for the TP value gives us the FN value. This is how we calculate FN for each class.
The IRIS DATASET is a popular example of a multi-class classification problem, with 3 classes: Versicolor, Virginia, and Setosa. We can use a decision tree classifier to classify the given instance as one of these three flowers.
Precision and recall are performance metrics used to retrieve data from training data in pattern recognition, object detection, and classification tasks.
For your interest: Binary Classification
Evaluation
Evaluating the performance of a machine learning model is crucial, and one of the most commonly used tools for this is the confusion matrix.
A confusion matrix typically looks like this, with rows representing actual classes and columns representing predicted classes.
In a binary classification problem, the confusion matrix will have two rows and two columns, with the following labels: Predicted Negative (0), Predicted Positive (1), Actual Negative (0), and Actual Positive (1).
True Negative and False Positive are the labels for the intersection of Actual Negative (0) and Predicted Positive (1) and Predicted Negative (0) and Actual Positive (1) respectively.
True Positive and False Negative are the labels for the intersection of Actual Positive (1) and Predicted Positive (1) and Predicted Negative (0) and Actual Positive (1) respectively.
The code to plot the confusion matrix from the predicted data of our model is also provided.
Approximately 84% of the data appears to be correct, which is a significant progress toward obtaining the required output.
However, it's worth noting that we can further optimize this model by leveraging a larger dataset and fine-tuning the hyper-parameters.
Here are some key factors to consider when working with complex datasets:
- Complex Data Preprocessing
- Advanced Data Encoding
- Understanding Data Correlation
- Multiple Neural Network Layers
- Feature Engineering
- Regularization
Why We Need
We need a confusion matrix to accurately measure the performance of classification models, especially when dealing with imbalanced target class data.
Accuracy alone can be misleading in such cases, as it may give a false sense of the model's performance.
Accuracy is not enough to quantify the performance of a classification model, especially when the target classes are not properly balanced.
A binary classification model with imbalanced target class data is a perfect example of this issue.
The confusion matrix allows us to measure Recall and Precision, which are essential metrics for evaluating the performance of ML models.
These metrics, along with Accuracy and the AUC-ROC curve, provide a more comprehensive understanding of a model's performance.
In fact, the confusion matrix is a crucial tool for measuring the performance of ML models, especially in cases of imbalanced target class data.
Sources
- https://dataaspirant.com/confusion-matrix-sklearn-python/
- https://www.freecodecamp.org/news/binary-classification-made-simple-with-tensorflow/
- https://www.analyticsvidhya.com/blog/2021/06/confusion-matrix-for-multi-class-classification/
- https://towardsdatascience.com/exploring-confusion-matrix-evolution-on-tensorboard-e66b39f4ac12
- https://neptune.ai/blog/keras-metrics
Featured Images: pexels.com