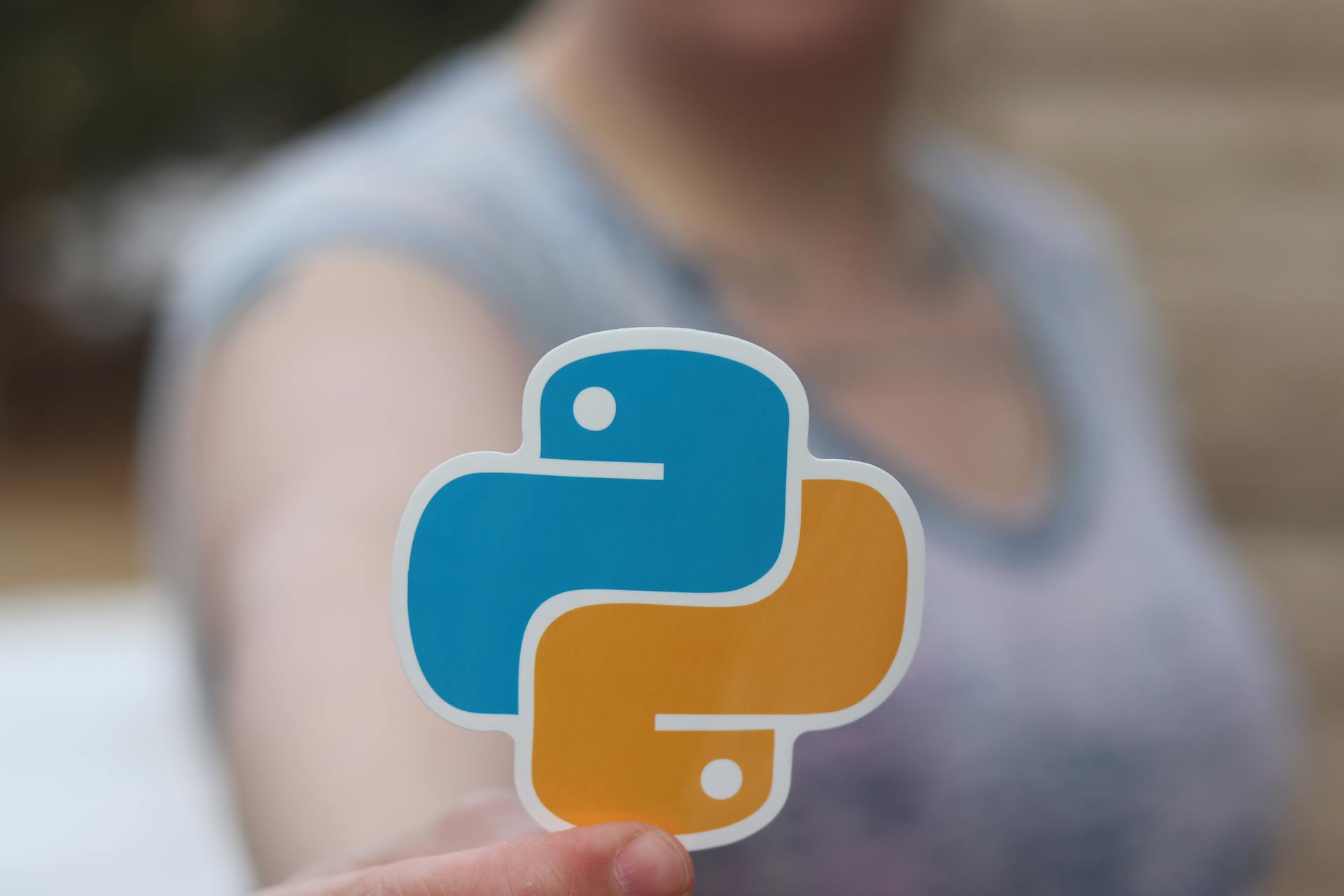
To code a binary classifier in Python, you'll need to use a machine learning algorithm that can distinguish between two classes. A simple approach is to use a logistic regression model, which is a type of supervised learning algorithm.
Logistic regression is a popular choice for binary classification because it's easy to implement and interpret. It works by learning the relationship between the input features and the target variable.
To get started, you'll need to import the necessary libraries, including scikit-learn and pandas. With these libraries, you can load your dataset, split it into training and testing sets, and then fit a logistic regression model to the data.
The scikit-learn library provides a range of tools and techniques for machine learning, including logistic regression. By using this library, you can quickly and easily implement a binary classifier in Python.
Intriguing read: Bayes Optimal Classifier
Choosing a Classifier
In a binary classification problem, you have two classes or labels to predict, such as spam or not spam emails.
The choice of classifier depends on the nature of the data and the problem you're trying to solve. For example, if you have a large dataset with many features, a Support Vector Machine (SVM) might be a good choice, as it can handle high-dimensional data.
However, if your data is linearly separable, a simple Perceptron might be sufficient.
Types of
Choosing the right classifier can be a bit overwhelming, but let's break it down into manageable types.
Binary Classifiers are useful for yes or no questions, as they can only output two possible labels.
The Logistic Regression classifier is a type of Binary Classifier that's great for handling linearly separable data.
Decision Trees are a popular choice for their simplicity and interpretability, but they can be prone to overfitting.
Random Forest Classifiers are an ensemble method that combines multiple Decision Trees to improve accuracy and reduce overfitting.
Support Vector Machines (SVMs) are powerful classifiers that can handle high-dimensional data and non-linear relationships.
K-Nearest Neighbors (KNN) Classifiers are a simple yet effective choice for datasets with a large number of features.
Worth a look: Pruning in Decision Tree
Loss Functions
Choosing a loss function is crucial when it comes to training a model, as it affects the model's training dynamics and convergence.
Binary Cross-Entropy Loss, also known as Log Loss, is a popular choice for logistic regression and neural network models. It's well-suited for binary classification problems where you want to estimate probabilities of the positive class.
Hinge Loss is commonly used in Support Vector Machines (SVMs), and it's effective for SVM-based binary classifiers. It encourages correct classification while penalizing instances within a certain margin of the decision boundary.
Gini impurity is used in decision tree-based models, such as random forests, to measure the disorder or impurity in a node's class distribution.
You might like: Decision Tree Algorithm Machine Learning
Implementing a Classifier
To implement a classifier, you'll need to choose a library such as PyTorch, Keras, or scikit-learn. PyTorch involves creating and training a neural network for binary classification tasks, while Keras requires compiling the model with a loss function, optimizer, and evaluation metric.
Recommended read: Confusion Matrix for Mnist Pytorch
You can also use scikit-learn, which provides a range of algorithms for binary classification, including logistic regression and support vector machines (SVMs). For example, you can use the SVC from scikit-learn to build an SVM classifier, which involves choosing a kernel function and building the classifier.
Here are some popular libraries for binary classification in Python:
Remember to choose a library that fits your needs and data type.
What Is a SVM?
A Support Vector Machine, or SVM for short, is a type of algorithm used for classification tasks. It's a powerful tool that can help us identify patterns in data and make predictions.
At its core, an SVM is designed to find the best line or decision boundary that separates two classes in a dataset. This line is often referred to as the "hyperplane." The goal is to maximize the distance between the classes, making it easier to distinguish between them.
In binary classification, which is a common application of SVMs, we're dealing with two distinct classes. These classes are typically labeled as "positive" and "negative", where the positive class represents the presence or occurrence of something, and the negative class represents its absence.
Here are the key characteristics of SVMs:
- They're designed to find the best hyperplane that separates two classes.
- They're particularly useful for high-dimensional data, where other algorithms may struggle to find meaningful patterns.
By understanding how SVMs work, we can better appreciate their strengths and weaknesses, and use them effectively in our classification tasks.
What is LightGBM?
LightGBM is an open-source, high-performance gradient boosting system that's designed for efficient and scalable machine learning applications. It's a popular choice for both structured and unstructured data in various fields.
It was created specifically for speed and accuracy, making it a well-liked alternative for many users. LightGBM supports parallel and distributed processing, which allows it to handle enormous datasets with millions of rows and columns.
A key feature of LightGBM is its ability to manage massive datasets with ease. This is due to its histogram-based techniques and leaf-wise tree growth, which enable it to grow deep and effective trees.
LightGBM builds trees according to the size of a leaf, choosing splits that minimize loss. This approach produces better results compared to other gradient-boosting frameworks.
It uses regularization techniques and early halting to prevent overfitting, which is a common problem in machine learning. By doing so, LightGBM ensures that its predictions are accurate and reliable.
Logistic Regression
Logistic Regression is a popular algorithm for binary classification. It models the relationship between input features and the probability of belonging to a specific class using a logistic function.
This activation function maps any input to a value between 0 and 1, interpreted as the probability of the positive class.
In binary classification, the goal is to predict the class label of new data points based on patterns learned from a labelled dataset. The primary objective is to predict which of the two classes a given data point belongs to.
Logistic regression is particularly useful for binary classification because it can handle non-linear relationships between features and the target variable. By using a logistic function, it can model complex relationships and make accurate predictions.
Check this out: Binary Classifier
To implement logistic regression, you can use Python and scikit-learn, just like in our example where we generate a synthetic dataset and train a logistic regression model.
Here are some key aspects of logistic regression:
- Probability score: Logistic regression outputs a probability score between 0 and 1, indicating the likelihood of a data point belonging to the positive class.
- Binary decision: Based on the probability score, you can make a binary decision, such as "spam" or "not spam", to classify the data point.
Implement with Scikit-Learn
Implementing a classifier with Scikit-Learn is a straightforward process. You can start by generating a dataset using Scikit's make_blobs function, which creates a linearly separable dataset with 2 features.
To build the SVM classifier, you'll need to choose a kernel function and construct the classifier using Scikit-learn's SVC function. This involves selecting a kernel that makes nonlinear data linearly separable, if necessary.
Building the classifier involves initializing the SVM classifier and fitting the training data to it. The training process starts with the fit method, which optimizes the model's parameters to minimize prediction errors.
Once the classifier is trained, you can use it to predict new data samples and evaluate its performance using metrics like accuracy, precision, recall, and F1 score.
Suggestion: Ensemble Classifier
Here's a step-by-step guide to implementing a binary SVM classifier with Scikit-Learn:
1. Import the necessary libraries, including Scikit-learn and Matplotlib.
2. Generate a dataset using Scikit's make_blobs function.
3. Choose a kernel function and construct the SVM classifier using Scikit-learn's SVC function.
4. Initialize the classifier and fit the training data to it using the fit method.
5. Use the trained classifier to predict new data samples and evaluate its performance.
Some key aspects to consider when implementing a binary SVM classifier with Scikit-Learn include:
- Choosing the right kernel function for your data
- Selecting the optimal parameters for the classifier
- Evaluating the classifier's performance using relevant metrics
- Visualizing the decision boundary using tools like Mlxtend
Implement with PyTorch
To implement a classifier with PyTorch, you'll need to have the library installed, which you can do using pip.
PyTorch is a popular deep learning library that makes it easy to create and train neural networks. You can start by importing the necessary libraries, preparing your data, and splitting it into training and testing sets.
To create a neural network model for binary classification, you can use a simple feedforward neural network. This involves defining the model architecture, including the number of inputs, hidden layers, and outputs.
Take a look at this: Hidden Layers in Neural Networks Code Examples Tensorflow
Here's a high-level overview of the steps involved in implementing a classifier with PyTorch:
- Import libraries
- Prepare data
- Create a neural network model
- Define the model architecture
- Train the model on the training data
By following these steps, you can create a classifier that can accurately classify input data into one of two classes.
Implement with Keras
Implementing a classifier with Keras involves creating and training a neural network for binary classification tasks. You can customize the model architecture, hyperparameters, and data preprocessing based on your specific task and dataset.
To perform binary classification with Keras, you need to compile the model by specifying the loss function, optimizer, and evaluation metric. This is typically done using the model.compile() method.
You can use various loss functions, such as binary cross-entropy or categorical cross-entropy, depending on the type of data you're working with. The optimizer choice also depends on the specific task and dataset.
Some common optimizers used in Keras include Adam, RMSprop, and stochastic gradient descent.
Here's a list of common loss functions used in Keras for binary classification:
- Binary Cross-Entropy (BCE)
- Categorical Cross-Entropy (CCE)
Remember to add callbacks for early stopping or model checkpointing to improve model performance and prevent overfitting.
Training and Evaluation
Training a binary classifier in Python requires a solid understanding of model training and evaluation. Model training is a crucial step where the selected machine learning algorithm learns from the labelled training data to make predictions about new, unseen data.
The heart of model training is utilizing the training dataset, which typically includes feature vectors and corresponding class labels. The model learns to recognize patterns and relationships within this dataset, enabling it to make predictions on unseen data.
To assess the performance of a binary classification model, you need to use appropriate evaluation metrics. Common evaluation metrics for binary classification include accuracy, precision, recall, F1-score, and ROC-AUC.
The choice of evaluation metric depends on the specific goals of your binary classification problem. For example, in a medical diagnosis scenario, recall may be more critical than precision to ensure that all disease cases are correctly identified.
Training
Training is a crucial step in the machine learning process where the model learns from labelled training data to make predictions about new, unseen data. This process involves various aspects such as training data, loss functions, optimization techniques, and evaluation metrics.
See what others are reading: Ai and Machine Learning Training
Model training typically starts with defining the model, as seen in the LightGBM Classifier example where a LightGBM Classifier is initialized with a specified evaluation metric.
The choice of evaluation metric is important, as it affects the model's performance. In the LightGBM Classifier example, the 'auc' (Area Under the ROC Curve) metric is used as the evaluation metric.
The LightGBM Classifier example also shows how to fit the model on the training data using the fit method, and make predictions on both the training and validation sets.
Here's a summary of the key steps involved in training a LightGBM Classifier:
The Gini Impurity metric is also an important aspect of model training, but it's not directly related to the LightGBM Classifier example.
Splitting
Splitting is a crucial step in the training process, where we divide our data into training and validation sets. This is typically done with an 80-20 ratio, where 80% of the data is used for training and 20% for validation.
The goal of splitting data is to ensure our model is not overfitting or underfitting. By splitting the data, we can evaluate our model's performance on unseen data and make adjustments as needed.
To split the data, we can use a library like scikit-learn, which provides a function called train_test_split. This function takes in the features and target variable, and splits them into training and validation sets.
Here's an example of how to use train_test_split: features = df.drop('Outcome', axis=1) target = df['Outcome'] X_train, X_val, Y_train, Y_val = train_test_split(features, target, random_state=2023, test_size=0.20)
The shapes of the training and validation sets can be displayed using X_train.shape, X_val.shape.
Preprocessing and Feature Engineering
Preprocessing and Feature Engineering is a crucial step in building a reliable binary classifier. To evaluate the performance of our model, we split the dataset in an 80:20 ratio.
This split allows us to train our model on a large portion of the data while still having a significant amount of data for validation. We can then use the validation set to fine-tune our model and prevent overfitting.
To ensure our features are on the same scale, we use standard scaling, which calculates the mean and standard deviation from the training data and applies the same transformation to both the training and validation sets. This is done using the StandardScaler from Scikit-Learn.
Here's a quick summary of the preprocessing steps:
Preprocessing
Data preprocessing is a crucial step in machine learning that can significantly impact model performance. It involves splitting the dataset into training and validation sets, typically in an 80:20 ratio.
To evaluate the model's performance during the training process, you can use this ratio to split the data. This allows you to see how well the model is doing as it learns from the data.
Feature scaling is another important aspect of preprocessing. It helps to ensure that all features are on the same scale, which can improve model performance. StandardScaler from Scikit-Learn is commonly used for this purpose.
Here's an interesting read: Pre Processing Data
The code to apply standard scaling to features is as follows:
By applying standard scaling, you can ensure that features have a mean of 0 and a standard deviation of 1, which can lead to improved model performance.
Dealing with Imbalance
Imbalanced data can lead to biased models that perform poorly on the minority class. This is because machine learning models trained on imbalanced data tend to be biased towards the majority class, aiming to minimize the overall error.
To address this issue, you can try resampling the data. Oversampling the minority class by duplicating existing samples or generating synthetic samples can help. Techniques like the Synthetic Minority Over-sampling Technique (SMOTE) can be particularly useful.
Undersampling the majority class by randomly removing samples is another option, but be cautious about information loss when using this method.
Algorithm-level solutions can also be effective. Cost-sensitive learning, for example, modifies the learning algorithm to consider class imbalance by assigning different misclassification costs to different classes. Many algorithms in Scikit-learn support this.
Ensemble methods like Balanced Random Forests or EasyEnsemble can also help address class imbalance by creating multiple models and combining their predictions.
Treat the minority class as an anomaly detection problem, where the goal is to identify rare instances. Techniques like Isolation Forest or One-Class SVMs can be employed.
Alternatively, you can collect more data for the minority class if possible. A larger dataset can help models better understand the minority class.
To evaluate the performance of your model, consider using metrics less sensitive to class imbalance, such as precision, recall, F1-score, the area under the Precision-Recall curve (AUC-PR), or the Matthews correlation coefficient (MCC).
Here are some strategies for dealing with imbalanced data:
- Resampling (oversampling or undersampling)
- Algorithm-level solutions (cost-sensitive learning, ensemble methods)
- Anomaly detection (treating the minority class as an anomaly)
- Data-level solutions (collecting more data, engineering features)
Sources
- https://spotintelligence.com/2023/10/09/binary-classification/
- https://graphite-note.com/binary-classification-model-in-ml/
- https://github.com/christianversloot/machine-learning-articles/blob/main/creating-a-simple-binary-svm-classifier-with-python-and-scikit-learn.md
- https://www.digitalocean.com/community/tutorials/how-to-build-a-machine-learning-classifier-in-python-with-scikit-learn
- https://www.geeksforgeeks.org/binary-classification-using-lightgbm/
Featured Images: pexels.com