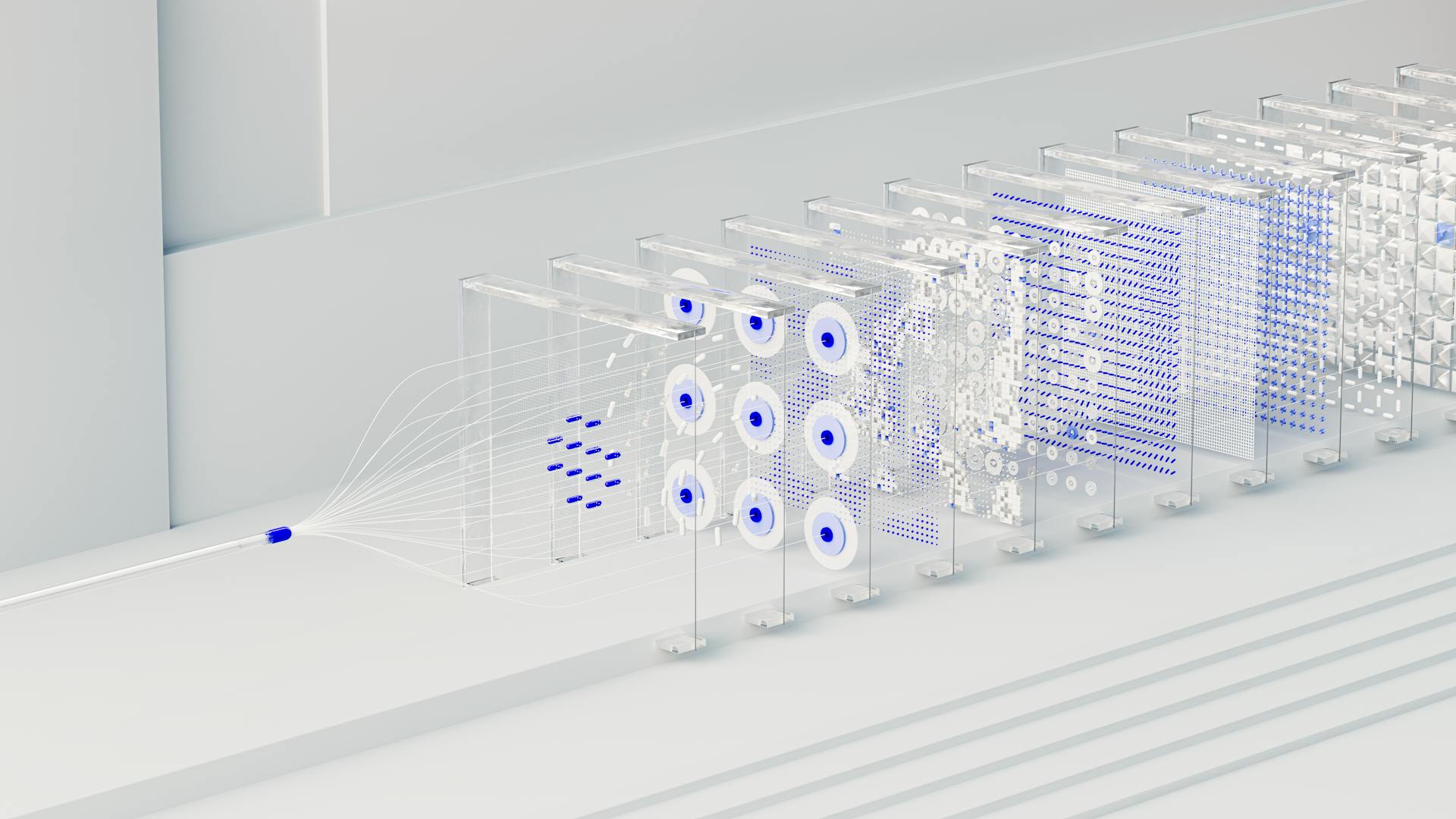
Tensor data preprocessing is a crucial step in machine learning. It involves transforming raw data into a format that can be used for training and testing models.
Data normalization is a common technique used to scale data to a common range. This is often done to prevent features with large ranges from dominating the model.
Reshaping data is another technique used to transform data into a suitable format. This can involve changing the dimensions of the data or converting it from one data type to another.
Data augmentation is a technique used to artificially increase the size of the training dataset. This can involve rotating, flipping, or zooming images to create new variations.
Expand your knowledge: Hugging Face Transformers Format
Importing Necessary Library
Importing the necessary library is the first step in tensor data preprocessing. You'll need to import the relevant modules from PyTorch, which includes transforms from torchvision.transforms.
The torchvision.transforms module provides a set of pre-defined transformations that can be applied to your data, such as resizing, normalizing, and converting images to tensors. These transformations are essential for preparing your data for training and testing.
To access the CIFAR10 dataset, you'll need to import it from torchvision.datasets. The CIFAR10 dataset is a popular choice for image classification tasks, consisting of 60,000 32x32 color images in 10 classes.
The DataLoader from torch.utils.data is also essential for importing, which helps to load and manage your dataset efficiently. With DataLoader, you can easily create data loaders that can handle large datasets and provide them to your model in batches.
A fresh viewpoint: Random Shuffle Dataset Python Huggingface
Data Preprocessing
Data preprocessing is a crucial step in machine learning, and it's essential to do it efficiently. You can use PyTorch's DataLoader class to load and preprocess data in batches, optimizing memory usage and training performance.
PyTorch supports a wide range of preprocessing operations, including transforming data, preprocessing structured data, and working with PyTorch tensors. You can also use Ray Data for preprocessing data, which supports a variety of operations, including caching the preprocessed dataset.
To optimize data preprocessing, you can implement custom transforms to handle specific preprocessing requirements, use GPU acceleration, and normalize data properly. It's also essential to handle missing data carefully and monitor data quality to ensure the best performance of your models.
Here's an interesting read: Confusion Matrix for Mnist Pytorch
PyTorch Best Practices
Using GPU acceleration can significantly speed up data preprocessing, especially when dealing with large datasets.
PyTorch's GPU-accelerated operations are designed to take advantage of the power of graphics cards, making them an essential tool for large-scale data preprocessing.
Data augmentation is a crucial step in data preprocessing, and PyTorch offers a variety of techniques to increase the diversity of your training data.
By applying data augmentation, you can improve the generalization of your models and make them more robust to different inputs.
Here are some common data augmentation techniques you can use in PyTorch:
- Rotation
- Flipping
- Scaling
- Color jittering
Custom transforms are also a powerful tool in data preprocessing, allowing you to create custom transformation functions to handle specific requirements unique to your dataset.
By implementing custom transforms, you can ensure that your data is properly preprocessed and that your models receive the best possible input.
Normalization is a critical step in data preprocessing, and PyTorch offers several normalization techniques to ensure that your data is properly scaled.
Here's an interesting read: How to Fine Tune Llm on Custom Data
By normalizing your data, you can prevent features with large scales from dominating the learning process and ensure that your models receive a balanced input.
Missing data can be a major issue in data preprocessing, but PyTorch offers several strategies to handle missing values, including mean imputation and interpolation.
By carefully handling missing data, you can ensure that your models receive the best possible input and that your results are accurate and reliable.
Optimizing your data preprocessing pipeline is essential to ensure consistency and reproducibility, and PyTorch offers several tools to help you streamline your pipeline.
By automating as much of your pipeline as possible, you can save time and reduce errors, ensuring that your models receive the best possible input.
Monitoring data quality is critical to ensure that your models receive the best possible input, and PyTorch offers several tools to help you monitor your data.
By continuously monitoring your data quality, you can identify and address any issues before they impact your models.
Documenting your preprocessing steps is essential to ensure that you can reproduce your results and understand the impact of each step on your final model.
By documenting your preprocessing steps, you can ensure that your results are reproducible and that you can identify any issues that may arise.
A unique perspective: Pytorch Transfer Learning
Image Preprocessing
Image Preprocessing is a crucial step in data preprocessing. It helps ensure that your image data is in a format that can be effectively used for machine learning models.
The code for performing data preprocessing on image datasets, such as the CIFAR-10 dataset, uses PyTorch's torchvision library. This library provides a convenient way to load and transform image data.
Converting images to tensors is a fundamental step in image preprocessing. It allows machine learning models to process the image data more efficiently.
Normalizing pixel values is another important step in image preprocessing. This involves scaling the pixel values to a common range, typically between 0 and 1.
DataLoader objects can be set up for training and testing data to facilitate efficient data loading and processing. This is particularly useful when working with large datasets.
Pad
Pad is a strategy used to ensure tensors have a uniform shape, which can be an issue when sentences of varying lengths are input into the model.
Tensors need to have a uniform shape, so padding is necessary to make them rectangular.
Padding is achieved by adding a special padding token to shorter sentences, which can be done by setting the padding parameter to True.
The first and third sentences are now padded with 0’s because they are shorter.
Random Shuffling
Random shuffling is a crucial step in data preprocessing, especially when training certain models. Randomly shuffling data for each epoch can be important for model quality depending on what model you are training.
Ray Data provides multiple options for random shuffling, which is a great feature for those who need it. You can find more details on shuffling data in the Ray Data documentation.
Shuffling data can help prevent overfitting by introducing randomness into the training process. This is particularly useful when working with complex models that can easily memorize the training data.
In some cases, shuffling data may not be necessary, and other preprocessing techniques may be more effective. However, for certain models, shuffling data can make a significant difference in model quality.
A unique perspective: Conditional Random Fields
Quickstart
To begin with data preprocessing, you'll need to install Ray Data and Ray Train. This involves four basic steps that we'll outline below.
First, create a Ray Dataset from your input data. This is the foundation of your preprocessing workflow.
Next, you'll apply preprocessing operations to your Ray Dataset. This might involve cleaning, transforming, or aggregating your data.
Now, input the preprocessed Dataset into the Ray Train Trainer, which internally splits the dataset equally in a streaming way across the distributed training workers. This ensures that your data is properly prepared for training.
Finally, consume the Ray Dataset in your training function. This is where the magic happens, and your model starts to learn from the data.
Here's a summary of the steps:
- Create a Ray Dataset from your input data.
- Apply preprocessing operations to your Ray Dataset.
- Input the preprocessed Dataset into the Ray Train Trainer.
- Consume the Ray Dataset in your training function.
Caching the
Caching the preprocessed dataset can be a huge performance booster. If your preprocessed dataset is small enough to fit in Ray object store memory, materialize it in Ray's built-in object store by calling materialize() on the preprocessed dataset.
This method tells Ray Data to compute the entire preprocessed dataset and pin it in the Ray object store memory, so when iterating over the dataset repeatedly, the preprocessing operations don't need to be re-run.
However, if the preprocessed data is too large to fit into Ray object store memory, this approach will greatly decrease performance as data needs to be spilled to and read back from disk. Transformations that you want to run per-epoch, such as randomization, should go after the materialize call.
Another trick to increase pipeline performance is caching data in memory or local storage to avoid repeating reading and extraction. Using the caching function from tf.data, you can store data points in memory to avoid re-processing them.
Just be careful not to overload the cache with too much data, as this can decrease performance. It's usually better to do complex transformations offline rather than executing them on a training job and caching the results.
Readers also liked: Hugging Face Run Locally
Transformations and Loading
Transformations are a crucial step in tensor data preprocessing, and the code uses transforms.Compose() to define a series of transformations.
To convert images to tensors, the code uses transforms.ToTensor(), which is a common step in many machine learning pipelines.
The code also normalizes the pixel values, which is essential for many deep learning models.
The CIFAR-10 dataset is loaded for both training and testing, applying the defined transformations during loading.
The dataset is downloaded to the specified root directory if it's not already available, which ensures that the data is consistent across different runs.
Data is loaded using DataLoader objects for both the training and testing datasets, specifying the batch size and whether to shuffle the data.
The code creates DataLoader objects for both the training and testing datasets, which enables efficient batch processing during training.
By using a DataLoader, the code can easily switch between different batch sizes and shuffling strategies, which can be useful for hyperparameter tuning.
The CustomDataset class is used to load data from a CSV file named 'phishing_data.csv', which demonstrates how to work with custom datasets.
Data is then wrapped in a DataLoader object for efficient batch processing during training, which is a common pattern in many machine learning applications.
Suggestion: How to Code Binary Classifier in Python
Custom Dataset and Loader
You can create a custom dataset class to load and preprocess your data for machine learning tasks. The CustomDataset class inherits from PyTorch's Dataset and loads data from a CSV file.
The __init__ method initializes the dataset by loading the CSV file specified by csv_file, and you can also apply additional transformations using the transform argument. This method is essential for loading and preprocessing your data.
Data preprocessing is a crucial step in machine learning, and the CustomDataset class handles missing values using SimpleImputer, categorical variables using LabelEncoder, and numerical features using StandardScaler.
The __len__ method returns the total number of samples in the dataset, which is useful for determining the number of epochs or iterations in your training process.
You can access individual samples from the dataset using the __getitem__ method, which returns a tuple containing the sample (features) and its corresponding target (label).
Here are the key methods of the CustomDataset class:
- __init__ Method: Initializes the dataset by loading the CSV file.
- Data Preprocessing: Handles missing values, categorical variables, and numerical features.
- __len__ Method: Returns the total number of samples in the dataset.
- __getitem__ Method: Returns a tuple containing the sample and its target.
Once you have created your custom dataset, you can wrap it in a DataLoader object for efficient batch processing during training. This is demonstrated in the code that creates a DataLoader using the CustomDataset class to load data from a CSV file named 'phishing_data.csv'.
Frequently Asked Questions
What is a tensor in data science?
A tensor is a multidimensional array that stores numerical data, representing various types such as text, audio, images, and numerical values in deep learning models. It's a fundamental data structure in data science that enables efficient manipulation of complex data.
Sources
- https://www.geeksforgeeks.org/data-preprocessing-in-pytorch/
- https://huggingface.co/docs/transformers/en/preprocessing
- https://docs.ray.io/en/latest/train/user-guides/data-loading-preprocessing.html
- https://theaisummer.com/data-processing-optimization/
- https://www.javatpoint.com/data-preprocessing-machine-learning
Featured Images: pexels.com