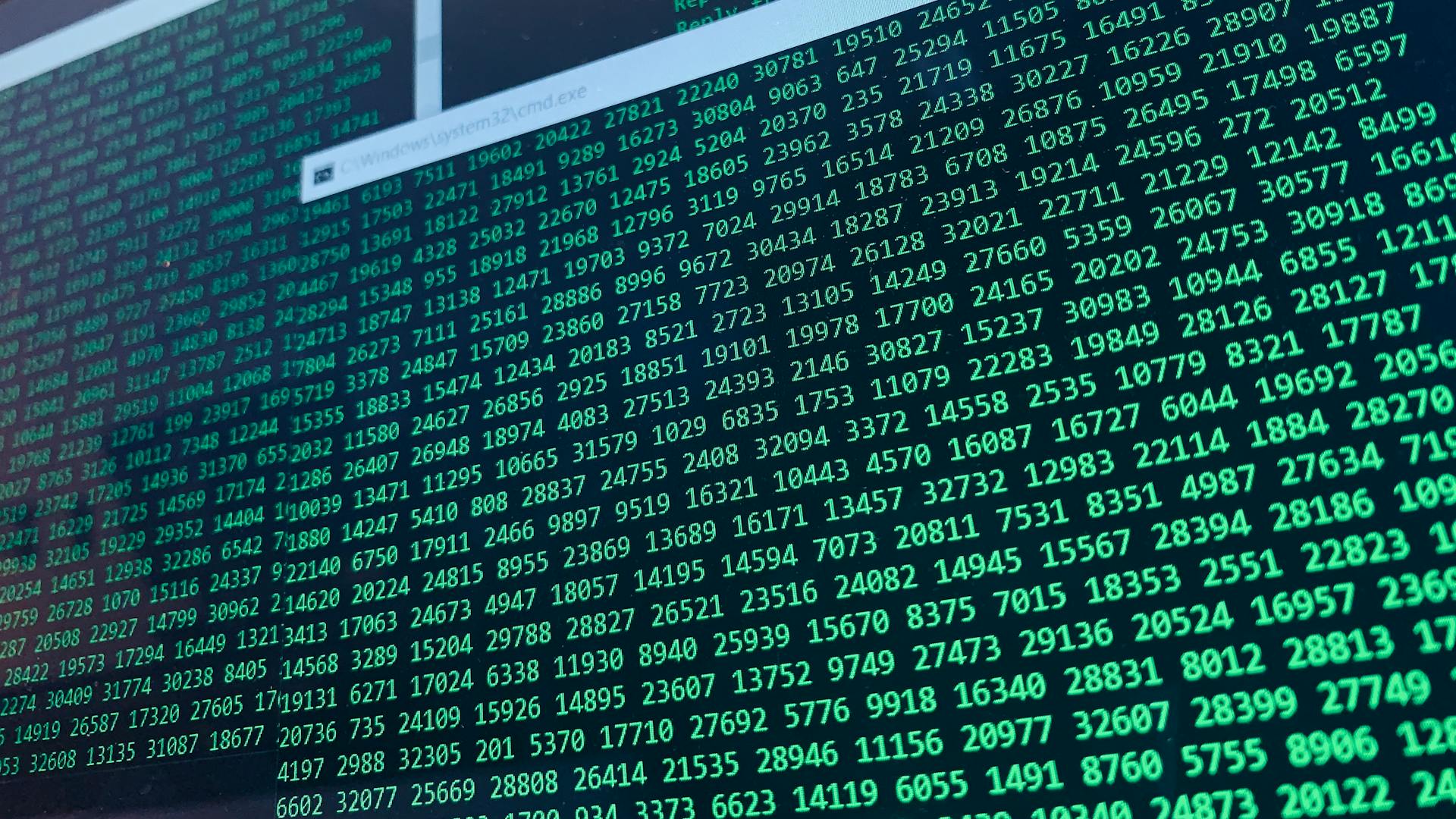
A confusion matrix is a critical tool for evaluating the performance of a classification model, and PyTorch provides a simple way to create and visualize one.
To use PyTorch's confusion matrix, you'll first need to import the necessary libraries, including `torch` and `torch.nn`.
You can then create a confusion matrix using the `torch.nn.functional` module's `confusion_matrix` function, passing in the true labels and predicted labels as arguments.
This function will return a square matrix where the rows represent the true labels and the columns represent the predicted labels.
What Is a Confusion Matrix?
A confusion matrix is a table used to describe the performance of a classification model. It's a simple yet powerful tool that helps us understand how well our model is doing.
The matrix itself is a square table with true positives, false positives, true negatives, and false negatives on its axes. This layout helps us visualize the relationships between these metrics.
In the context of binary classification, a confusion matrix can be used to calculate precision, recall, and F1 score, which are all important metrics for evaluating model performance.
Explore further: Confusion Matrix in Ai
What Is a Confusion Matrix?
A confusion matrix is a table used to evaluate the performance of a classification model. It helps us understand how well the model is doing in predicting the correct class labels.
A confusion matrix is typically a square table with the true class labels on one axis and the predicted class labels on the other. The table has four main sections: true positives, false positives, true negatives, and false negatives.
The true positives are the number of instances where the model correctly predicted the class label. In one example, a model predicted 85 out of 100 instances correctly, with 15 instances being incorrectly classified. This means the true positives were 85.
The false positives are the number of instances where the model incorrectly predicted a class label. In the same example, the model incorrectly classified 15 instances as belonging to the positive class.
Why Do We Need a Confusion Matrix?
A confusion matrix is essential for assessing a classification model's performance. It provides a thorough analysis of true positive, true negative, false positive, and false negative predictions.
A model's recall, accuracy, precision, and overall effectiveness in class distinction can be better understood with a confusion matrix. This is particularly helpful when there is an uneven class distribution in a dataset.
In such cases, the confusion matrix helps evaluate a model's performance beyond basic accuracy metrics.
Check this out: Confusion Matrix Accuracy Formula
Interpreting Confusion Matrix Results
In a courtroom scenario, a Type 1 Error occurs when the court mistakenly convicts an individual as guilty when they are actually innocent.
Precision is affected by false positives, and it's the ratio of true positives to the sum of true positives and false positives.
A Type 1 Error can have profound consequences, leading to the wrongful punishment of an innocent person who did not commit the offense.
In the context of medical testing, a Type 2 Error occurs when a diagnostic test fails to detect the presence of a disease in a patient who genuinely has it.
Recall is directly affected by false negatives, and it's the ratio of true positives to the sum of true positives and false negatives.
Minimizing false positives is crucial for precision, while minimizing false negatives is key for recall.
A false positive can be thought of as a wrongful conviction, while a false negative can be seen as a delayed diagnosis.
Discover more: Matrix Error Codes
Using Confusion Matrices in PyTorch
Using Confusion Matrices in PyTorch is a crucial step in evaluating the performance of a machine learning model.
A confusion matrix is a table used to evaluate the performance of a classification model. It is a square table with the true class labels on one axis and the predicted class labels on the other.
The diagonal elements of a confusion matrix represent the number of correct predictions, while the off-diagonal elements represent the number of incorrect predictions.
By analyzing the confusion matrix, we can identify the types of errors the model is making and improve its performance.
Binary Classification
In binary classification, we're dealing with just two classes: 0 and 1, yes and no, dog and not dog. The confusion matrix for binary tasks is a 2x2 matrix that helps us understand how well our model is performing.
The confusion matrix is constructed such that the row indices correspond to the true class labels and the column indices correspond to the predicted class labels. For binary tasks, the matrix has the following structure:
True Positives (TP) are the instances where the model correctly predicted the positive class, while False Positives (FP) are the instances where the model incorrectly predicted the positive class. True Negatives (TN) are the instances where the model correctly predicted the negative class, while False Negatives (FN) are the instances where the model incorrectly predicted the negative class.
For example, let's say we have a dog image recognition model, and the confusion matrix looks like this:
In this example, the model correctly predicted 5 out of 6 dog images as dogs (TP = 5), and incorrectly predicted 1 out of 4 not dog images as dogs (FP = 1).
Multi-Class Classification
In multi-class classification, you have more than two possible classes for your model to predict. The confusion matrix expands to accommodate these additional classes.
Each cell within the matrix shows the count of instances where the model predicted a particular class when the actual class was another. For example, in a 3X3 Confusion matrix, each cell represents the count of instances where the model predicted a particular class (column) when the actual class was another (row).
A 3X3 Confusion matrix is shown below for the image having three classes. The actual classes (ground truth) are represented by the rows, and the predicted classes are represented by the columns.
You might like: 3x3 Confusion Matrix
Here's a breakdown of what each cell in the matrix represents:
- True Positive (TP): The image was of a particular animal, and the model correctly predicted that animal.
- True Negative (TN): The image was not of a particular animal, and the model correctly predicted it as not that animal.
- False Positive (FP): The image was not of a particular animal, but the model incorrectly predicted it as that animal.
- False Negative (FN): The image was of a particular animal, but the model incorrectly predicted it as a different animal.
Here's an example of a 3X3 Confusion matrix with numbers:
This matrix shows the count of instances where the model predicted a particular class when the actual class was another. For example, 8 cats were correctly identified, 1 was misidentified as a dog, and 1 was misidentified as a horse.
Multilabel
In multilabel tasks, the confusion matrix is a valuable tool for evaluating model performance. It's a Nx2x2 tensor, where each 2x2 matrix corresponds to the confusion for that label.
Each 2x2 matrix has a specific structure, which is crucial to understand when working with multilabel tasks. The confusion matrix accepts the following input: predictions (preds) and true labels (target).
To update the metric, you can pass in the predictions and true labels as tensors. The preds tensor can be an integer or float tensor, and if it's a floating point tensor with values outside the [0,1] range, it will be auto-applied with sigmoid per element. Additionally, it will be converted to an int tensor with thresholding using the value in threshold.
Consider reading: Transfer Learning Enables Predictions in Network Biology
The confusion matrix has several parameters that can be adjusted, including num_classes, threshold, ignore_index, normalize, and validate_args. These parameters allow you to customize the calculation of the confusion matrix to suit your specific needs.
Here are the possible values for the normalize parameter:
- true
- pred
- all
- none
By adjusting these parameters, you can get a more accurate picture of your model's performance on multilabel tasks.
Sources
- https://pytorch.org/ignite/generated/ignite.metrics.confusion_matrix.ConfusionMatrix.html
- https://lightning.ai/docs/torchmetrics/stable/classification/confusion_matrix.html
- https://www.geeksforgeeks.org/confusion-matrix-machine-learning/
- https://www.v7labs.com/blog/confusion-matrix-guide
- https://stackoverflow.com/questions/74020233/how-to-plot-confusion-matrix-in-pytorch
Featured Images: pexels.com