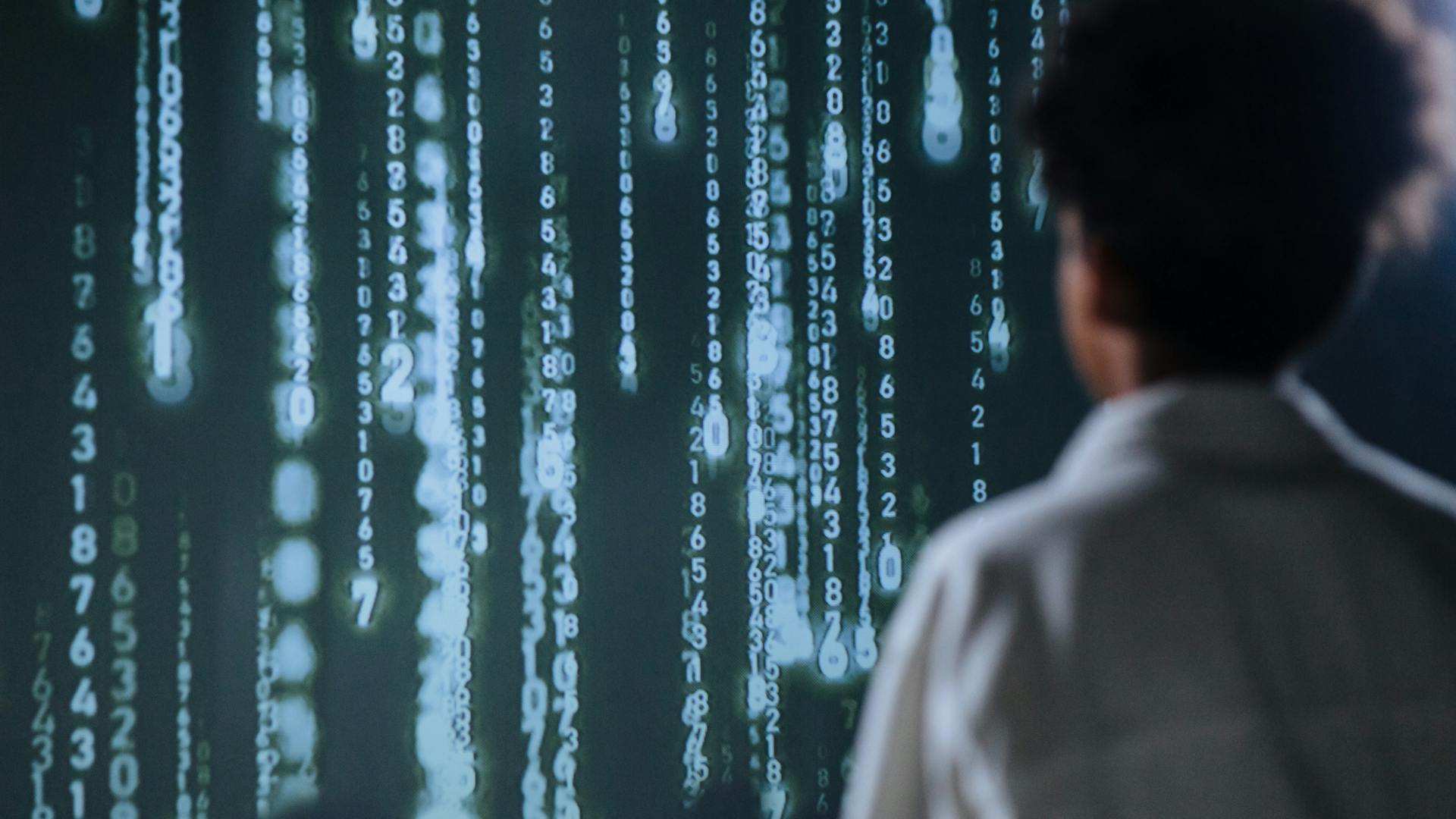
A sensitivity confusion matrix is a table used to evaluate the performance of a binary classification model. It's a simple yet powerful tool that helps you understand how well your model is doing.
The matrix is divided into four quadrants, each representing a different outcome. True Positives (TP) and True Negatives (TN) are the correct predictions, while False Positives (FP) and False Negatives (FN) are the incorrect ones.
The accuracy of a model is the total number of correct predictions (TP + TN) divided by the total number of predictions. A perfect model would have an accuracy of 1, but in reality, it's rare to achieve this.
What Is a Confusion Matrix?
A confusion matrix is a table that shows the actual classes of outcomes and the predicted classes. It's a simple yet powerful tool for evaluating the performance of a model.
The rows of the confusion matrix represent the actual classes, while the columns represent the predictions made by the model. This allows us to easily see which predictions are incorrect.
There are five key metrics that can be calculated from a confusion matrix: accuracy, misclassification, precision, sensitivity (also known as recall), and specificity. These metrics provide a clear picture of how well a model is performing.
Here's a breakdown of each metric:
These metrics are essential for evaluating the performance of a model and making informed decisions about its use.
Metrics and Definitions
Accuracy is a metric that calculates the proportion of correct predictions out of all predictions made by a model. It's calculated as (TP + TN) / (TP + FP + TN + FN), where TP is the number of true positives, TN is the number of true negatives, FP is the number of false positives, and FN is the number of false negatives.
Prevalence is another important metric that tells us the proportion of the positive class in the data. It's calculated as P / (P + N), where P is the number of actual positive cases and N is the number of actual negative cases.
The no information rate, on the other hand, is the proportion of observations that fall into the "majority" class, and it's calculated as max(P / (P + N), N / (P + N)). This is an important baseline for judging binary classifiers, as a classifier that doesn't achieve an accuracy above this rate is essentially performing worse than simply always guessing the majority class.
Here's a table summarizing the different metrics mentioned:
Sensitivity, also known as the true positive rate, is the proportion of actual positive cases that are correctly identified by the model. It's calculated as TP / (TP + FN). Specificity, on the other hand, is the proportion of actual negative cases that are correctly identified by the model, and it's calculated as TN / (TN + FP).
Accuracy
Accuracy measures how often the model is correct. It's calculated by dividing the number of correct predictions by the total number of observations.
Accuracy is a fundamental metric in evaluating a model's performance. In the context of binary classification, accuracy is the proportion of correctly predicted instances out of all instances.
To calculate accuracy, you can use the formula: Accuracy = (TP + TN) / (TP + FP + TN + FN), where TP is True Positives, TN is True Negatives, FP is False Positives, and FN is False Negatives.
For example, if a model predicts 80 out of 100 instances correctly, its accuracy would be 80%.
Created Metrics
The Created Metrics section of our article is where things get really interesting. We're going to dive into the different measures that help us evaluate our classification model, and I'm excited to share them with you.
Accuracy is a measure of how well our model is doing overall, calculated by dividing the number of correct predictions (TP + TN) by the total number of predictions (TP + TN + FP + FN).
We also have Precision, which is the ratio of true positives (TP) to the sum of true positives and false positives (TP + FP). This metric is especially useful when we want to know how accurate our model is at predicting the positive class.
Sensitivity, also known as Recall, is a measure of how well our model detects the positive class, calculated by dividing the number of true positives (TP) by the sum of true positives and false negatives (TP + FN).
Specificity is another important metric that measures how well our model can distinguish between the positive and negative classes, calculated by dividing the number of true negatives (TN) by the sum of true negatives and false positives (TN + FP).
Lastly, we have the F-score, which is a measure of how well our model is doing overall, taking into account both precision and recall. This metric is especially useful when we want to know how well our model is performing in terms of both accuracy and detection of the positive class.
Here are the Created Metrics in a concise table:
These metrics are essential in evaluating our classification model and making adjustments to improve its performance. By understanding what each metric represents, we can make informed decisions to optimize our model and achieve better results.
Types of Errors and Metrics
There are two types of errors in a confusion matrix: Type I and Type II. Type I errors are also known as False Positives, which occur when a model predicts a positive outcome when it's actually negative. Type II errors are also known as False Negatives, which occur when a model predicts a negative outcome when it's actually positive.
A simple way to remember the difference between Type I and Type II errors is to rewrite the terms. False Positive has only one negative word, making it a Type I error, while False Negative has two negative words, making it a Type II error.
Here are the different metrics that can be calculated from a confusion matrix:
- Accuracy (all correct / all) = TP + TN / TP + TN + FP + FN
- Misclassification (all incorrect / all) = FP + FN / TP + TN + FP + FN
- Precision (true positives / predicted positives) = TP / TP + FP
- Sensitivity aka Recall (true positives / all actual positives) = TP / TP + FN
- Specificity (true negatives / all actual negatives) = TN / TN + FP
These metrics can help you evaluate the performance of your model and identify areas for improvement.
Creating a Matrix
A confusion matrix is a table used to evaluate the performance of a binary classifier. It's created by comparing the actual and predicted values of a dataset.
To generate a confusion matrix, you need actual and predicted values. These can be created using NumPy, as shown in the code snippet: `actual = numpy.random.binomial(1, 0.9, size = 1000)` and `predicted = numpy.random.binomial(1, 0.9, size = 1000)`.
Once you have the actual and predicted values, you can use the `metrics.confusion_matrix` function from the sklearn module to create the confusion matrix. This function takes the actual and predicted values as input and returns a confusion matrix.
The confusion matrix is a table that displays the number of true positives, true negatives, false positives, and false negatives. It's a useful tool for evaluating the performance of a binary classifier.
Here's a breakdown of what each cell in the confusion matrix represents:
This table helps you understand the types of errors that can occur in a binary classification problem. For example, if you have a high number of false positives, it may indicate that your classifier is too sensitive and is classifying too many negative cases as positive.
Precision
Precision is a crucial metric in evaluating the performance of a binary classifier. It's a measure of how often we correctly classify an observation as positive when we predict positive.
To calculate precision, we divide the number of true positives by the sum of true positives and false positives. This means that precision focuses on the positive class, specifically how well we're doing at identifying actual positives.
Precision is also known as the positive predictive value. It tells us the proportion of positive predictions that are actually correct.
The false discovery rate, which is the proportion of false positives among all positive predictions, is closely related to precision. In fact, adding precision and the false discovery rate together will give us a total of 1, since they're complementary measures.
Here's a formula for precision: True Positive / (True Positive + False Positive).
Types of Errors
A confusion matrix can help you understand the types of errors your model is making. There are two types of errors: Type I and Type II.
Type I errors are also known as False Positives. This occurs when you predict an actual negative observation to be true. The false positive rate, also known as the miss rate, indicates how often this happens.
Type II errors are also known as False Negatives. This occurs when you predict an actual positive observation to be false. The false negative rate indicates how often this happens.
Here's a simple way to keep Type I and Type II errors straight: consider the meanings of these words. False Positive contains one negative word (False), so it's a Type I error. False Negative has two negative words (False + Negative), so it's a Type II error.
Here's a summary of the two types of errors:
Understanding the types of errors your model is making can help you identify areas for improvement and optimize your model's performance.
Frequently Asked Questions
What is confusion matrix sensitivity?
Confusion matrix sensitivity measures a model's ability to correctly identify true positives, or instances that actually belong to a specific category. It's a key metric for evaluating a model's performance in recognizing true positives.
Are recall and sensitivity the same?
Yes, recall and sensitivity are interchangeable terms, referring to the proportion of actual positive instances correctly identified by a model. They are often used interchangeably in the context of evaluating model performance.
Sources
- https://benhay.es/posts/demystifying-confusion-matrix/
- https://wiki.eigenvector.com/index.php?title=Confusionmatrix
- https://statisticallearning.org/binary-classification.html
- https://www.w3schools.com/python/python_ml_confusion_matrix.asp
- https://towardsdatascience.com/taking-the-confusion-out-of-confusion-matrices-c1ce054b3d3e
Featured Images: pexels.com