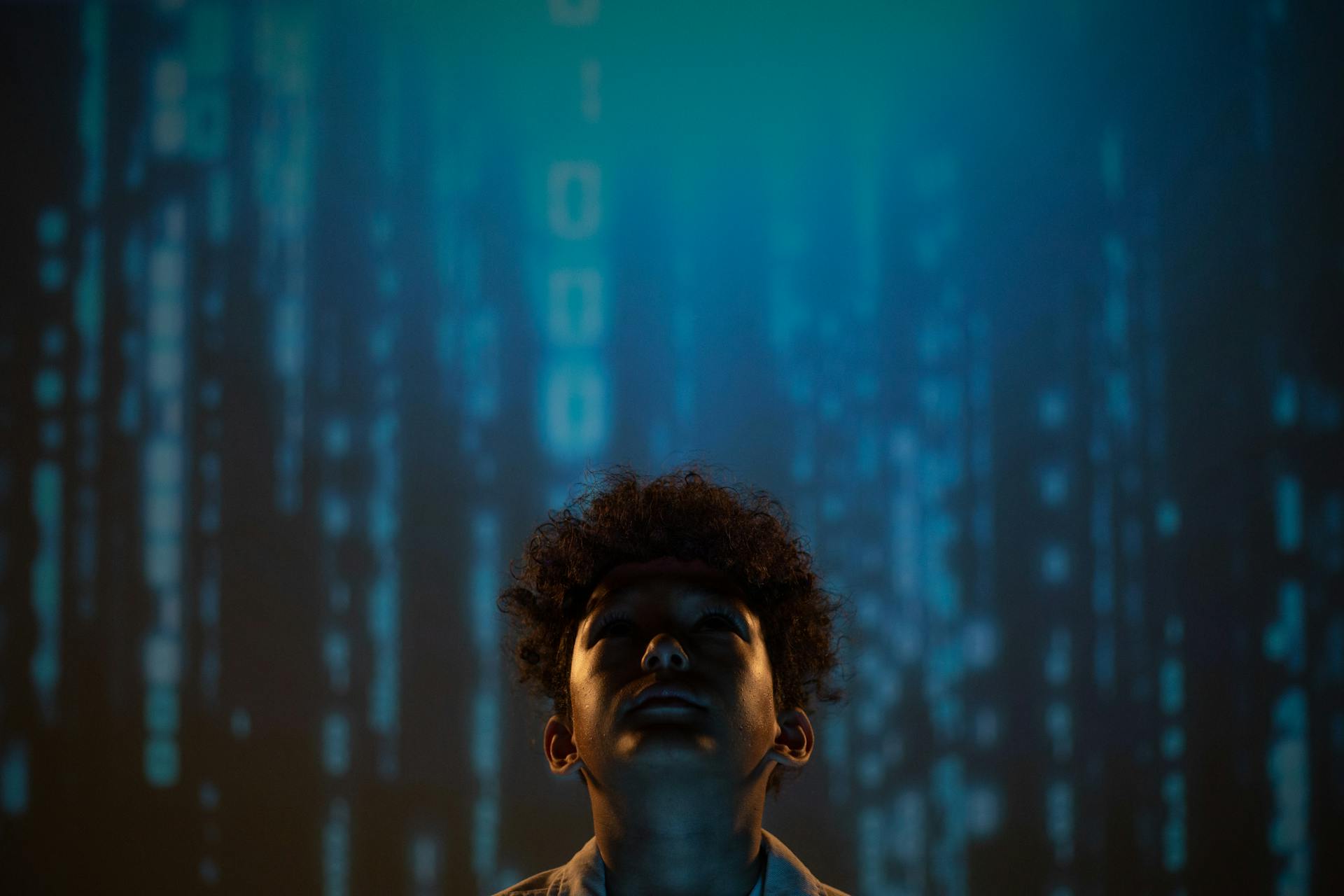
A confusion matrix is a powerful tool in machine learning that helps you evaluate the performance of your model. It's a table that summarizes the predictions made by your model and the actual outcomes, making it easy to visualize and understand the accuracy of your model.
You should use a confusion matrix when you're working with a classification problem, as it's most effective in this type of scenario. This is because classification problems involve predicting a categorical label or class, which is exactly what a confusion matrix is designed to help with.
A confusion matrix is particularly useful when you have an unbalanced dataset, where one class has a significantly larger number of instances than the others. This is because the matrix will highlight the classes that are being misclassified, allowing you to take corrective action to improve your model's performance.
Understanding Precision and Recall
Precision and Recall are two essential metrics that help you evaluate the performance of your classification model. Precision tells you how many of the correctly predicted cases actually turned out to be positive, which is crucial in cases where False Positive is a higher concern than False Negatives.
To calculate Precision, you divide the number of true positive predictions by the total number of positive predictions made by the model. In our example, Precision is 50%, which means that 50% of the correctly predicted cases turned out to be positive.
Precision is important in music or video recommendation systems, e-commerce websites, etc. where wrong results could lead to customer churn and be harmful to the business.
Recall, on the other hand, measures the effectiveness of a classification model in identifying all relevant instances from a dataset. It is the ratio of the number of true positive (TP) instances to the sum of true positive and false negative (FN) instances.
To calculate Recall, you divide the number of true positive predictions by the sum of true positive and false negative instances. In our example, Recall is 75%, which means that 75% of the actual positive cases were successfully predicted by our model.
Recall is a useful metric in cases where False Negative trumps False Positive, such as in medical cases where it doesn’t matter whether we raise a false alarm, but the actual positive cases should not go undetected.
Here's a summary of the key differences between Precision and Recall:
Note that Precision and Recall are not always mutually exclusive, and in some cases, you may want to combine them to get a more comprehensive understanding of your model's performance.
Types of Confusion Matrices
There are several types of confusion matrices, each serving a specific purpose. The most common type is the standard confusion matrix, which is used to evaluate the performance of a binary classifier.
A standard confusion matrix categorizes predictions into four quadrants: true positives, false positives, true negatives, and false negatives. This type is useful for evaluating models that predict a binary outcome.
A contingency table is a type of confusion matrix that's particularly useful for evaluating the performance of a model on a categorical target variable.
Binary
In binary classification, we have a 2x2 Confusion Matrix that helps us understand how well our model is performing. This matrix is shown in the example for image recognition, where we're trying to determine whether an image is of a Dog or Not Dog.
A True Positive (TP) is counted when both the predicted and actual values are Dog. This is the total count of correct predictions.
The False Positive (FP) count is the total number of instances where the prediction is Dog, but the actual value is Not Dog. This can be a sign that our model is overestimating the presence of Dogs.
A True Negative (TN) occurs when both the predicted and actual values are Not Dog. This is the total count of correct rejections.
A False Negative (FN) is counted when the prediction is Not Dog, but the actual value is Dog. This means our model is underestimating the presence of Dogs.
Here's a summary of the binary classification metrics:
Matrices with Multiple Categories
Confusion matrices are not limited to binary classification and can be used in multi-class classifiers as well. They can be used to summarize communication of a whistled language between two speakers, with zero values omitted for clarity.
A confusion matrix for a multi-class classification problem is a square matrix where the number of rows and columns is equal to the number of classes. For example, if we have to predict whether a person loves Facebook, Instagram, or Snapchat, the confusion matrix would be a 3 x 3 matrix.
The true positive, true negative, false positive, and false negative for each class are calculated by adding the cell values. This is done by looking at the cell values within the matrix.
Each cell within the matrix shows the count of instances where the model predicted a particular class when the actual class was another. The rows represent the actual classes (ground truth) in the dataset, while the columns represent the predicted classes by the model.
A 3X3 Confusion matrix is shown below, where each row represents the instances in the actual class, and each column represents the instances in a predicted class.
In this matrix, the diagonal cells show correctly classified samples, while the off-diagonal cells show model errors. To read the matrix, you can look at the cell values and understand how the model performed. For example, if you look at the top row, the model correctly labeled 700 (out of all 1000) negative reviews.
Error Types and Metrics
A Type 1 error occurs when a model predicts a positive instance, but it's actually negative, leading to false positives.
In a courtroom scenario, a Type 1 Error can result in the wrongful conviction of an innocent person.
Type 1 errors are directly related to precision, which is the ratio of true positives to the sum of true positives and false positives.
Precision emphasizes minimizing false positives.
Type 2 errors occur when a model fails to predict a positive instance, resulting in false negatives.
In medical testing, a Type 2 Error can lead to a delayed diagnosis and subsequent treatment.
Type 2 errors are directly related to recall, which is the ratio of true positives to the sum of true positives and false negatives.
Recall focuses on minimizing false negatives.
Example
A confusion matrix is a simple yet powerful tool for evaluating the performance of a classification model. It's a table that summarizes the predictions made by your model and compares them to the actual outcomes.
You can think of a confusion matrix as a scoreboard that keeps track of the number of correct predictions, called true positives (TP) and true negatives (TN), and the number of incorrect predictions, called false positives (FP) and false negatives (FN).
Let's take a look at an example from the world of image classification. Imagine a model that's trying to identify cats, dogs, and horses in images. A confusion matrix for this model might look like this:
In this example, the model correctly identified 8 cats, 10 dogs, and 8 horses. It also made some mistakes, misidentifying some cats as dogs, some dogs as cats, and some horses as dogs.
Here's a breakdown of what each of these numbers means:
- True Positive (TP): The image was of a particular animal, and the model correctly predicted that animal. For example, a picture of a cat correctly identified as a cat.
- True Negative (TN): The image was not of a particular animal, and the model correctly predicted it as not that animal. For example, a picture of a car correctly identified as not a cat, not a dog, and not a horse.
- False Positive (FP): The image was not of a particular animal, but the model incorrectly predicted it as that animal. For example, a picture of a horse mistakenly identified as a dog.
- False Negative (FN): The image was of a particular animal, but the model incorrectly predicted it as a different animal. For example, a picture of a dog mistakenly identified as a cat.
By looking at this confusion matrix, you can get a sense of how well your model is performing and identify areas where it needs improvement.
Implementation and Tools
To implement a confusion matrix, you'll need to import the necessary libraries, which in this case includes Python. The libraries used are not explicitly stated in the section, but it's likely that libraries such as NumPy and seaborn are required.
In order to compute the confusion matrix, you'll need to create a NumPy array for the actual and predicted labels. This array will be used to calculate the accuracy of your model.
The implementation of the confusion matrix is further enhanced by plotting it with the help of seaborn's heatmap. This visual representation can help identify any discrepancies between the actual and predicted labels.
To get a better understanding of your model's performance, you can also compute a classifications report based on confusion metrics.
Implementation Using Python
To implement a confusion matrix for binary classification using Python, you need to import the necessary libraries.
NumPy is one of the essential libraries for creating arrays and performing numerical computations, which is used in this implementation.
To create the confusion matrix, you need to compute it using the actual and predicted labels, which can be represented as a NumPy array.
The seaborn library is used to plot the confusion matrix as a heatmap, providing a visual representation of the classification results.
For a binary classification problem, the confusion matrix can be computed and plotted to evaluate the performance of the model.
The classification report based on confusion metrics provides a summary of the model's performance, including precision, recall, and F1-score.
Table
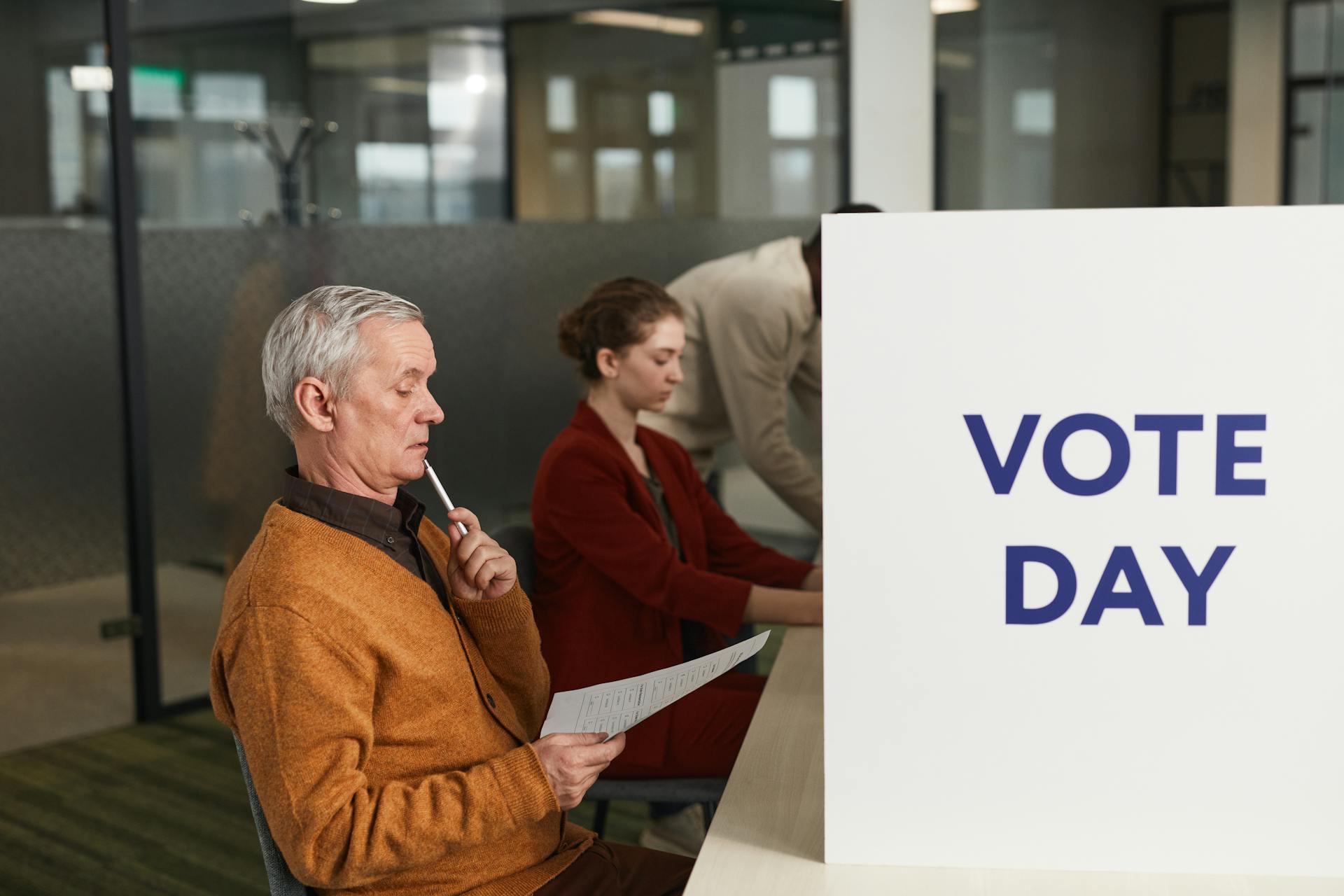
A table of confusion, also known as a confusion matrix, is a table with two rows and two columns that reports the number of true positives, false negatives, false positives, and true negatives. This is essential for detailed analysis, especially when dealing with unbalanced data.
The most informative metric to evaluate a confusion matrix is the Matthews correlation coefficient (MCC). This is according to experts Davide Chicco and Giuseppe Jurman.
In a confusion matrix, the number of real positive cases is represented by P, and the number of real negative cases is represented by N. These numbers are crucial for understanding the performance of a classifier.
A true positive (TP) is a test result that correctly indicates the presence of a condition or characteristic. This is a key metric to track in a confusion matrix.
Here are some key metrics included in a confusion matrix:
Accuracy (ACC) is calculated as TP + TN/P + N, but it can be misleading in unbalanced data.
Frequently Asked Questions
Why is confusion matrix better than accuracy?
A confusion matrix provides more detailed insights than accuracy alone, offering a deeper understanding of a model's performance through metrics like precision and recall. This makes it a more comprehensive tool for evaluating classification models.
Sources
- classification_report() (scikit-learn.org)
- Understanding the Confusion Matrix in Machine Learning (geeksforgeeks.org)
- 10.1017/S0952675705000552 (doi.org)
- 10.1186/s13040-021-00244-z (doi.org)
- "Evaluation: From Precision, Recall and F-Measure to ROC, Informedness, Markedness & Correlation" (researchgate.net)
- 10.1016/j.patrec.2005.10.010 (doi.org)
- "An Introduction to ROC Analysis" (elte.hu)
- 10.1162/tacl_a_00675 (doi.org)
- "A Closer Look at Classification Evaluation Metrics and a Critical Reflection of Common Evaluation Practice" (doi.org)
- 10.1016/S0034-4257(97)00083-7 (doi.org)
- How to interpret a confusion matrix for a machine learning ... (evidentlyai.com)
- Understanding Confusion Matrix | by Sarang Narkhede (towardsdatascience.com)
Featured Images: pexels.com