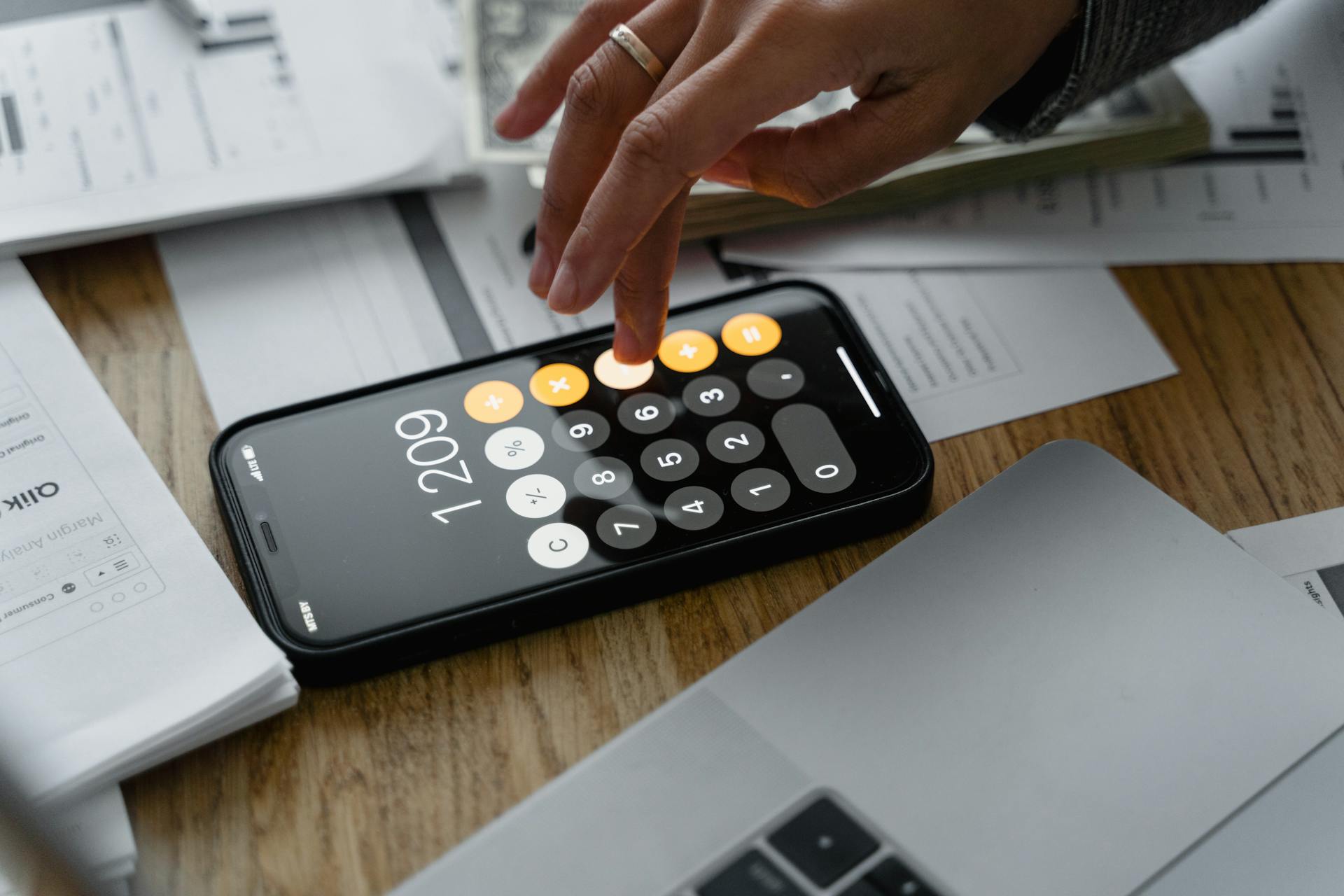
Coding an application can be a daunting task, but breaking it down into smaller steps makes it more manageable.
Start by defining your project's requirements and goals. Identify the problem you're trying to solve and the features your application will need to have.
A good place to begin is by choosing a programming language, such as Java or Python, which are popular choices for beginners.
Next, decide on the type of application you want to build, like a mobile app or a web application. This will help you determine the tools and technologies you'll need to use.
Setting Up
First, you need to ensure you have the necessary technologies installed in your coding environment.
To build for Android, you'll need to have Android Studio installed, along with some APIs and the Android SDK.
Choose the Android version you want React Native to support.
Installing an emulator is recommended, and Genymotion is a good option.
On a similar theme: Android Application Code
If you're using a Macbook, you can install Android Studio as well as Genymotion.
You'll also need to install the react-native CLI and its dependencies, such as Node.js version 4.0 or later.
Consider installing Xcode to set up native iOS SDKs.
The expo-cli is highly recommended, especially if you're new to React Native, as it will make setting up easier and help you test your app.
If you're absolutely new to React Native, you can use Create React Native, which is similar to Create React App for web apps.
On a similar theme: Unity Learn Create with Code
Windows and Messages
Windows sends messages to your application to notify it of events. You can handle these messages in your WndProc function.
To handle messages, you implement a switch statement in your WndProc function. An important message to handle is WM_PAINT, which is sent when part of your window must be updated.
You can use the BeginPaint and EndPaint functions to prepare for and complete the drawing in the client area. BeginPaint returns a handle to the display device context used for drawing in the client area.
An application typically handles many other messages, such as WM_CREATE, which is sent when a window is first created, and WM_DESTROY, which is sent when the window is closed.
Here are some common messages and their purposes:
- WM_PAINT: Sent when part of the window must be updated.
- WM_CREATE: Sent when a window is first created.
- WM_DESTROY: Sent when the window is closed.
To handle a WM_PAINT message, you call BeginPaint, then handle all the logic to lay out the text, buttons, and other controls in the window. Finally, you call EndPaint.
In your WndProc function, you can use a switch statement to handle different messages. For example, you can use the WM_DESTROY message to post a quit message when the window is closed.
Building and Testing
Building and testing is an essential part of coding an application. To build a Windows C++ desktop application, you need to compile the code in Visual Studio.
You can compile the code by deleting all the code in the editor, copying the example code, and pasting it into the HelloWindowsDesktop.cpp file. Then, on the Build menu, choose Build Solution, and the results of the compilation will appear in the Output window.
To run the application, press F5, and a window with the text "Hello, Windows desktop!" should appear.
What Are We Building?
We're building a project called Colorify, which shows a colored circle on the web page. It has some buttons that let you change the colors by clicking on them.
The project involves basic DIV styling with border-radius and centering to create the colored circle. This styling technique is crucial for achieving the desired visual effect.
We'll also be laying out buttons to provide an interactive experience for the user. Three buttons labeled Red, Green, and Yellow will be used to change the circle's color.
Template Literals will be used to create dynamic text for the buttons. This feature allows us to insert values into strings, making the code more efficient and readable.
To make the buttons functional, we'll add click handlers to listen for user interactions. This will trigger a response when a button is clicked, changing the circle's color accordingly.
DOM manipulation will be used to set the values of the buttons and the circle's color. This involves modifying the Document Object Model to reflect the changes made by the user.
Curious to learn more? Check out: Visual Studio Code C# Console Application
To Build This
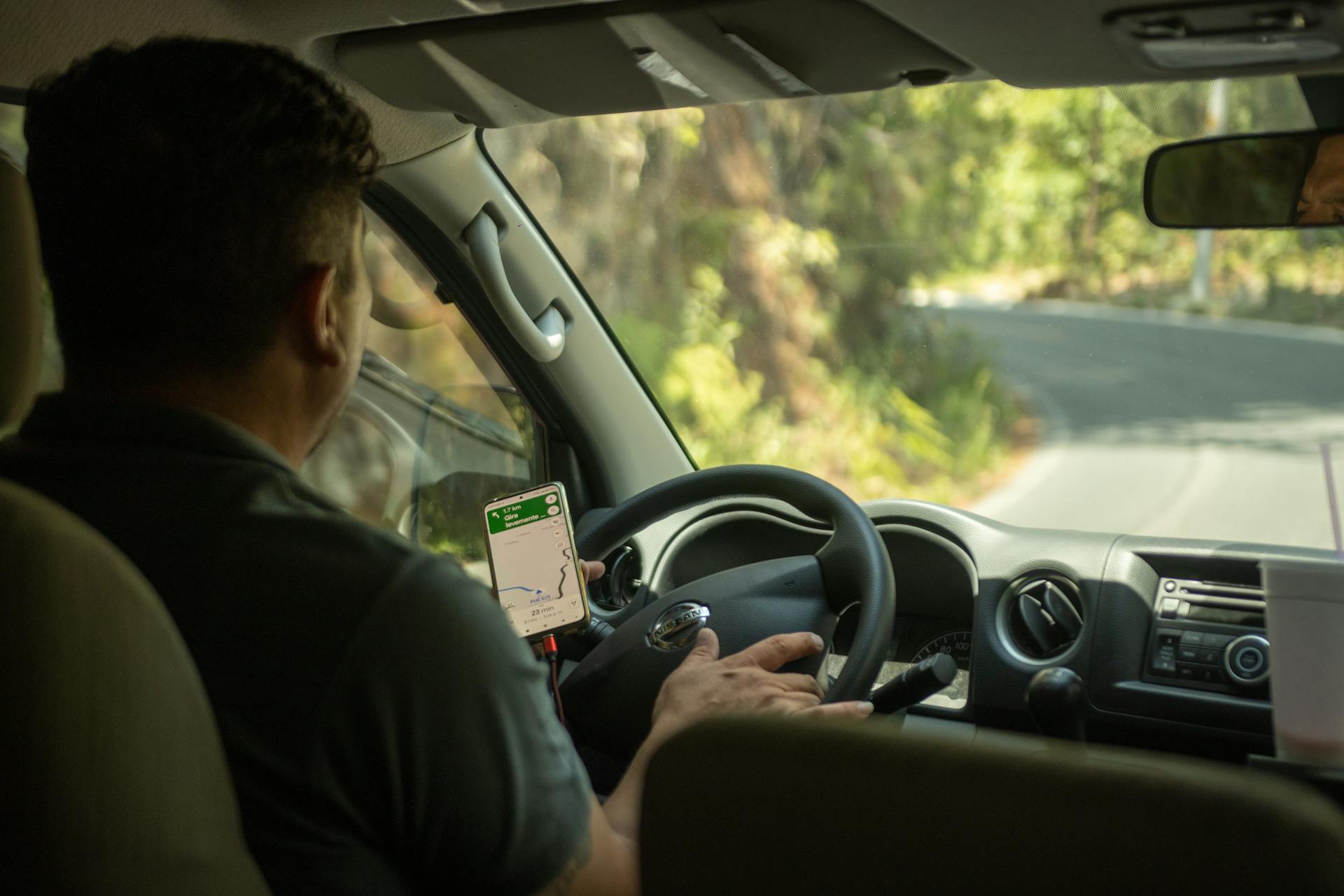
Delete all the code in HelloWindowsDesktop.cpp and copy the example code to paste it into the file. This will replace the existing code with the new example.
You'll need to compile the code with specific flags to ensure it works correctly: /D_UNICODE /DUNICODE /DWIN32 /D_WINDOWS /c. This tells the compiler to use Unicode and Windows-specific flags.
The code includes several global variables, such as szWindowClass and szTitle, which are used to store the main window class name and title bar text, respectively. These variables are used throughout the code.
To register the window class, the code uses the RegisterClassEx function, which takes a WNDCLASSEX structure as an argument. This structure contains information about the window class, such as its name, icon, and cursor.
The code also uses the CreateWindowEx function to create the main window, passing in various parameters such as the window class name, title, and initial position.
Here are the key flags to use when compiling the code:
- /D_UNICODE
- /DUNICODE
- /DWIN32
- /D_WINDOWS
- /c
Write Unit Tests
Writing unit tests is a great industry practice that will help you later on when you begin to work for a company or even take on freelance projects. It makes it easier to identify errors and bugs and fix them as early as possible.
Writing unit tests before coding your app is recommended, making it easier to catch problems early on.
Unit tests focus on testing small pieces of code, such as functions, objects, classes, or methods.
React Native comes with a testing framework called "Jest" preinstalled, making it easy to get started with unit testing.
You should only test a single thing in each test, and tests should be readable and maintainable.
You might enjoy: Test Automation for Ai and Ml Code
Integration Testing
Integration testing is a crucial step in building and testing your app. It ensures that different components work in sync.
You might find issues like an outdated dependency creating an error in another part of your app, as mentioned earlier. This can be frustrating, but it's a great opportunity to learn and improve your app.
Here's an interesting read: Learn Morse Code App
Integration testing helps you identify these problems early on, saving you time and effort in the long run. It's a win-win situation for both you and your users.
By testing your app's components together, you can catch bugs and fix them before they become major issues. This will give you peace of mind and confidence in your app's performance.
Component Testing
Component testing is a crucial step in building and testing your app. It involves checking whether your app does what the user intends it to.
You might find, for example, that if a user types in a job they're looking for and hits enter, they see the expected results. This is exactly what component testing is all about.
To perform component testing, you need to see your app in action. This can be done using an emulator or a real mobile device. It's worth noting that with emulators, you might miss some details about the app's performance on a mobile device or even UI interactions when a user takes an action.
React Native recommends Genymotion, and you can also use the free Android Studio emulator or simulator on XCode. To be safe, you can use both an emulator or simulator and a real mobile device.
Here are some key points to keep in mind when performing component testing:
- Check if the UI renders correctly, including button sizes and placements.
- Verify that user actions result in the expected outcomes.
- Use an emulator or real mobile device to test your app.
- Consider using Genymotion or the free Android Studio emulator or simulator on XCode.
Publishing
To publish your app, you need to create a Google Play Store account, which requires a Google account. You can use your existing Gmail account if you have one.
To digitally sign your app, you'll need to create a release key and use Play App Signing to manage your signing key. This will help keep your key safe and allow Google to sign your app's APK.
You'll also need to generate an upload key, which you can use keytool to create. Then, update your Gradle files to include keystore information, which will allow your app to be signed with the release key. Next, generate the release build, which will package your app in a format that the app store can work with.
You might like: Can I Generate Code Using Generative Ai Models
Here are the necessary app information details you'll need to publish your app on the Play Store:
- App name
- App description
- App screenshots
- App version
- Bundle ID
- Developer or publisher profile
To publish on the Apple App Store, you'll need to create an Apple Developer Account and enroll in the Apple Developer Program, which costs $99 per year. You'll also need to sign up for an Expo account and install Expo Application Services to make the process easier.
Publish
Publishing your app on the app store requires some setup and configuration. You'll need to create an Apple Developer Account and enroll in the Apple Developer Program, which comes with an annual fee of $99.
To make the process easier, you can use Expo to publish your app on the app store. You'll need to sign up for an Expo account and install Expo Application Services.
You'll need to configure your project using Expo in your terminal, create your app's build, and add a bundle identifier that will be used to uniquely identify your app. This will help you set up your app on App Store Connect.
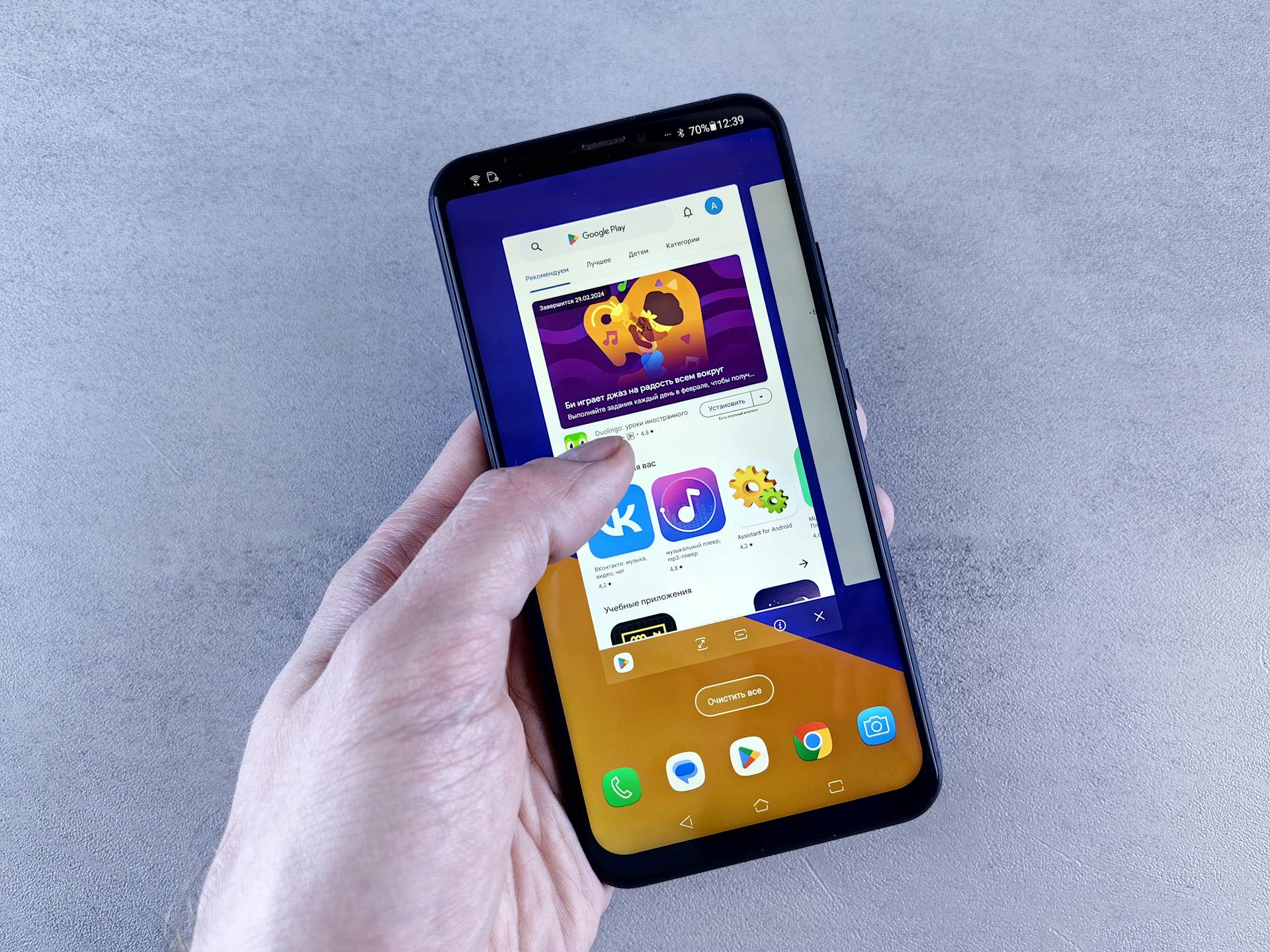
To set up your app on App Store Connect, you'll need to create an account and add app information, including screenshots, app promotional text, app description, keywords, support URL, app version, and copyright. You'll also need to provide app review information and specify pricing, even if your app is free.
Here are the necessary app details you'll need to fill in:
- App promotional text
- App description
- Keywords
- Support URL
- App version
- Copyright
You may also need to provide a privacy policy, which you can generate using online tools. You can choose to release your app manually after it's been approved or automatically, specifying the release date if you choose the latter.
Before submitting your build to App Store Connect, you'll need to update details like ascAppId, which is your application ID under your app information. You can then run a command to submit your build to App Store Connect and add compliance to your app.
Publishing on Google Play
Publishing on Google Play involves creating a Google Play Store account, which you can do using your existing Google account. This is likely the same account you use for Gmail or other Google services.
Intriguing read: Google Summer of Code Application
You'll also need a Google account to digitally sign your app using a release key. This can be done using Play App Signing, which allows Google to manage your signing key and keep it safe.
Google distributes your app as a .apk file, which is the format they use for installation.
To upload your app to the Play Store, you'll need to generate an upload key using keytool. This key is specific to each app and is used by Google to upload your app.
You'll also need to update your Gradle files to include keystore information, which stores your keys. This allows your app to be signed with the correct key.
Your app will be generated in the Android App Bundles (AAB) format, which helps Google generate optimized APKs for different device specifications.
Before uploading your app, make sure to test it thoroughly. This will ensure that your app is ready for users to install.
You can also use tools like Proguard to reduce the size of your APK, making it easier to upload and install.
To publish your app on the Play Store, you'll need to have the following information ready:
- App name
- App description
- App screenshots
- App version
- Bundle ID (a unique identifier in the format "com.company.appname")
- Developer or publisher profile
Finally, make sure to adjust your app's settings, such as the app name and slug, before uploading it to the Play Store.
Frequently Asked Questions
Is it difficult to code an app?
Learning to code an app can take a few months, but creating a flawless one can take years. Consider using a no-code platform for a faster and more efficient app-building experience.
Sources
- Create a traditional Windows Desktop application (C++) (microsoft.com)
- this GitHub repository (github.com)
- GitHub (github.com)
- Flutter (flutter.dev)
- Apache Cordova (apache.org)
- React Native (reactnative.dev)
- or from an API (amazon.com)
- Android Studio (android.com)
- expo-cli (expo.dev)
- Create React App (create-react-app.dev)
- Create React Native (reactnative.dev)
- Mocha (mochajs.org)
- React Native’s documentation (reactnative.dev)
- Git (git-scm.com)
- Chrome DevTools (chrome.com)
- Play App Signing (android.com)
- React Native’s official documentation page (reactnative.dev)
- update the Gradle files (reactnative.dev)
- test it (reactnative.dev)
- Apple Developer Account (apple.com)
- What is Low-Code? A Quick Guide ... (quickbase.com)
- Low Code (oracle.com)
Featured Images: pexels.com