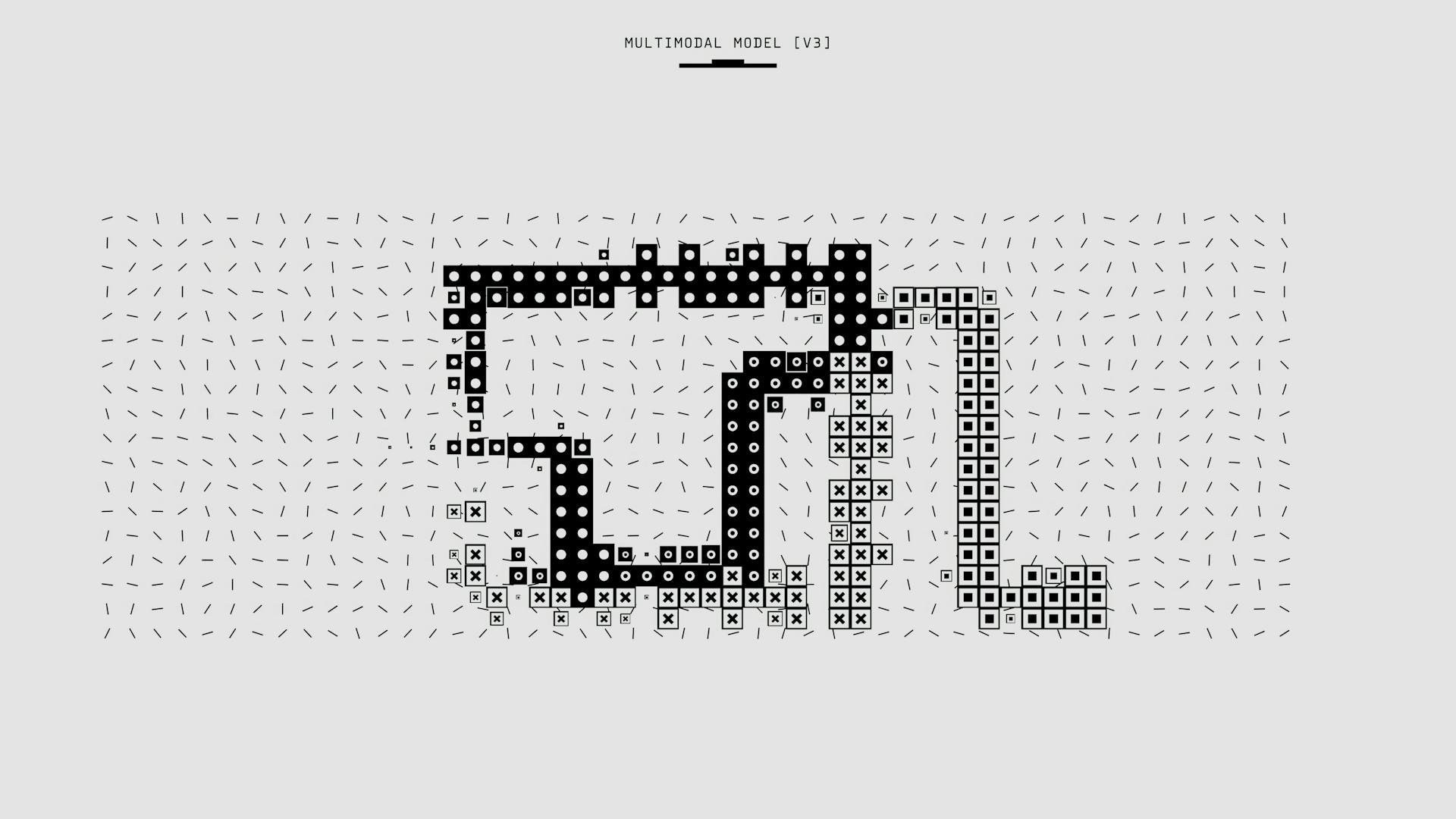
The KNN algorithm is a popular machine learning technique used for classification and regression tasks. It's a simple yet effective method that can be used with various types of data.
KNN stands for K-Nearest Neighbors, and it works by finding the closest neighbors to a new data point in the feature space. The algorithm then uses the characteristics of these neighbors to make predictions.
The KNN algorithm is often used in real-world applications, such as customer segmentation, image classification, and recommender systems.
You might like: Perceptron Learning Algorithm
What is KNN?
The K-Nearest Neighbors algorithm is one of the most basic yet essential classification algorithms in machine learning. It's non-parametric, meaning it doesn't make any assumptions about the distribution of data.
KNN is widely used in real-life scenarios, including pattern recognition, data mining, and intrusion detection. It's a supervised learning algorithm, which means it's trained on labeled data to make predictions.
The algorithm works by analyzing a training set to classify new data points into groups. It's easy to visualize, with unclassified points marked as 'White'.
KNN is a simple and popular algorithm, widely used for both classification and regression tasks. It's easy to understand, but its performance can be affected by the choice of K and the distance metric.
Related reading: What Is a Machine Learning Algorithm
Minkowski Distance
Minkowski Distance is a type of distance metric used in machine learning algorithms, including the k-nearest neighbors (knn) algorithm.
NCA, or Neighborhood Components Analysis, uses a squared Mahalanobis distance metric, which is calculated as the dot product of two matrices: M = L^T L, where M is a symmetric positive semi-definite matrix of size (n_features,n_features).
This distance metric is used to calculate the probability of a sample being correctly classified according to a stochastic nearest neighbors rule in the learned embedded space.
The probability of a sample being correctly classified is calculated using the softmax function over Euclidean distances in the embedded space.
In the context of NCA, the goal is to learn an optimal linear transformation matrix that maximizes the sum over all samples of the probability of being correctly classified.
Curious to learn more? Check out: Embedded Machine Learning
Training and Implementation
Training a KNN model involves storing a matrix of pairwise distances, which takes up n_samples**2 memory. This is a significant consideration for large datasets.
The time complexity of training a KNN model depends on the number of iterations done by the optimization algorithm, but you can set a maximum number of iterations with the argument max_iter.
The time complexity for each iteration is O(n_componentsxn_samplesxmin(n_samples,n_features)). This indicates that the algorithm's performance will degrade as the size of the dataset increases.
To implement a KNN model, you'll need to follow these steps:
- Load the data
- Initialise the value of k
- For getting the predicted class, iterate from 1 to total number of training data points
The default value of k when using the Scikit-Learn Library is 5, and the default distance metric used is Euclidean.
K-D Tree
The K-D Tree is a data structure that helps speed up nearest neighbors searches by efficiently encoding aggregate distance information. It's a binary tree structure that recursively partitions the parameter space along the data axes.
The K-D Tree is a generalization of two-dimensional Quad-trees and 3-dimensional Oct-trees to an arbitrary number of dimensions. It's constructed very fast, without needing to compute D-dimensional distances.
The K-D Tree approach is very fast for low-dimensional (D < 20) neighbors searches, but becomes inefficient as D grows very large. This is one manifestation of the "curse of dimensionality".
In scikit-learn, K-D Tree neighbors searches are specified using the keyword algorithm='kd_tree', and are computed using the class KDTree.
Python Implementation
Python Implementation is where the magic happens. You can implement the KNN model in Python using the following steps.
To load the data, you'll need to use a library like scikit-learn. The brute-force approach is a naive neighbor search implementation that involves computing distances between all pairs of points in the dataset, which scales as O[D N^2].
For the KNN model, you'll need to initialize the value of k, which determines the number of nearest neighbors to consider. In the classes within sklearn.neighbors, brute-force neighbors searches are specified using the keyword algorithm='brute'.
The KNN algorithm can be implemented using the following steps:
- Load the data
- Initialise the value of k
- For getting the predicted class, iterate from 1 to total number of training data points
The brute-force approach is not efficient for large datasets, but it can be very competitive for small data samples. In the classes within sklearn.neighbors, brute-force neighbors searches are computed using the routines available in sklearn.metrics.pairwise.
Choosing the Value of K
The value of K in the KNN algorithm is crucial and should be chosen based on the input data, as it defines the number of neighbors in the algorithm.
Readers also liked: Q Learning Algorithm
If the input data has more outliers or noise, a higher value of K would be better. It's recommended to choose an odd value for K to avoid ties in classification.
Cross-validation methods can help in selecting the best K value for the given dataset.
The decision surface becomes over jealous and tries to fit every datapoint correctly when K is too small, leading to overfitting.
The decision surface becomes smooth as K increases, but also becomes too general and may underfit the data.
To find the right value of K, you can use k-fold cross validation to assess the model's performance for different values of K.
The optimal value of K should be used for all predictions, as it determines the number of neighbors that will be checked to determine the K Nearest Neighbor classifier of a specific query point.
Lower K values can have high variance but low bias, while higher K values can have high bias but low variance.
The validation error curve can be used to find the optimal value of K, where the validation error is minimal.
The optimal value of K lies between the point where the validation error starts to increase again due to underfitting.
Advantages and Disadvantages
The KNN algorithm has some great advantages that make it a popular choice in machine learning. The algorithm is easy to implement due to its relatively low complexity.
One of the key benefits of KNN is its ability to adapt easily to new data. As the algorithm stores all data in memory storage, it can adjust itself as new examples are added, contributing to future predictions.
The KNN algorithm requires only a few hyperparameters to train, making it a convenient option for many use cases. These hyperparameters include the value of k and the choice of distance metric.
Here are the key advantages of the KNN algorithm at a glance:
- Easy to implement with low complexity.
- Adapts easily to new data.
- Requires only a few hyperparameters (k and distance metric).
Advantages of the
The KNN algorithm has some really cool advantages that make it a popular choice for many machine learning tasks. One of the main advantages is that it's easy to implement, thanks to its relatively low complexity.
The algorithm adapts easily to new data, which means that whenever a new example or data point is added, it adjusts itself to take that new information into account. This is because it stores all the data in memory, allowing it to make more accurate predictions.
There are only a few hyperparameters that need to be adjusted when training a KNN algorithm. These include the value of k and the choice of distance metric.
Disadvantages of the
The KNN algorithm, while having its advantages, also has some significant disadvantages that need to be considered.
One major disadvantage of the KNN algorithm is that it does not scale well. This is because it is considered a lazy algorithm, taking up a lot of computing power and data storage, making it both time-consuming and resource exhausting.
The algorithm is also affected by the curse of dimensionality, which makes it hard to classify data points properly when the dimensionality is too high.
This curse of dimensionality also makes the algorithm prone to overfitting, a problem that can be addressed by applying feature selection and dimensionality reduction techniques.
A higher value of k can help deal with outliers or noise in the input data, but it's generally recommended to choose an odd value for k to avoid ties in classification.
Applications and Use Cases
The KNN algorithm has a wide range of applications in machine learning, making it a versatile tool for various tasks.
Data Preprocessing is one area where KNN shines, particularly with the KNN Imputer method, which is effective in imputing missing values in datasets.
KNN algorithms excel in Pattern Recognition, as demonstrated by their high accuracy when trained on the MNIST dataset.
In Recommendation Engines, KNN is used to assign new query points to pre-existing groups based on a large corpus of datasets, providing personalized recommendations.
The KNN algorithm is widely used in classification problems, thanks to its ease of interpretation and low calculation time, making it a popular choice in the industry.
Here are some key applications of the KNN algorithm:
- Data Preprocessing: KNN Imputer for sophisticated imputation methodologies
- Pattern Recognition: High accuracy on the MNIST dataset
- Recommendation Engines: Assigning new query points to pre-existing groups
The KNN algorithm's time complexity is O(MNlog(k)), but with specialized data organization and preprocessing techniques, it can be made more efficient.
Performance and Complexity
The knn algorithm's performance and complexity are worth examining. The time complexity of the prediction phase is O(n*d), which can be a significant factor, especially for large datasets.
Sorting the distances or finding the k smallest distances can add an extra O(N log N) or O(Nk) step, making it even more computationally expensive. This is especially true for high-dimensional data.
The good news is that the majority voting step is O(1), so it doesn't add much to the overall time complexity. The space complexity of the algorithm is O(Nd), which is the space required to store the training dataset.
Time Complexity Breakdown:
The K Nearest Neighbor model is known for its time efficiency, thanks to the fact that it doesn't involve any training period.
The time complexity of the prediction phase is O(n*d), where n is the number of train points and d is the distance between the test data point and each data point in the train.
This means that the algorithm takes time proportional to the product of the number of train points and the distance.
In addition to the prediction phase, the sorting step can also be a factor in the overall time complexity, with a time complexity of O(N log N) or O(Nk) using algorithms like quicksort or partial sorting.
The good news is that the majority voting step, which is typically O(1), doesn't add much to the overall time complexity.
Overall, the time complexity of the algorithm is O(n*d), but the sorting step can be a bottleneck for large datasets or high-dimensional data.
Space Complexity
Space Complexity is a critical aspect of an algorithm's performance. The algorithm's space requirements can have a significant impact on its overall efficiency.
During the training phase, the space complexity is directly related to the size of the training dataset. It's O(N * d), where N is the number of data points and d is the number of features or dimensions.
This means that as the dataset grows, the space requirements will increase exponentially. I've seen this happen with large datasets, where the algorithm's memory usage becomes a bottleneck.
In the prediction phase, the algorithm needs to store distances between input data points and all training points, which requires O(N) space. This is a significant consideration for algorithms that need to handle large volumes of data.
Overall, the space complexity of the algorithm is O(Nd), which is a key factor to consider when evaluating its performance.
Handling Special Cases
Handling Special Cases can be tricky, especially when dealing with high-dimensional data. The knn algorithm's efficiency can be severely impacted by the curse of dimensionality.
In such cases, it's essential to consider using a reduced dimensionality technique, like PCA, to transform the data into a lower-dimensional space. This can significantly improve the algorithm's performance.
However, it's worth noting that the choice of distance metric can also have a substantial impact on the algorithm's accuracy, especially in cases where the data is not Euclidean.
Unsupervised
Unsupervised learning can be a bit tricky, but let's break it down.
The NearestNeighbors algorithm in scikit-learn implements unsupervised nearest neighbors learning. It provides a uniform interface to three different nearest neighbors algorithms: BallTree, KDTree, and a brute-force algorithm.
These algorithms can be controlled through the keyword 'algorithm', which must be one of ['auto','ball_tree','kd_tree','brute']. If 'auto' is passed, the algorithm attempts to determine the best approach from the training data.
Choosing the right algorithm is crucial, as each option has its strengths and weaknesses. For example, the brute-force algorithm based on routines in sklearn.metrics.pairwise can be slow for large datasets.
The choice of algorithm can also affect the result when two neighbors have identical distances but different labels. The result will depend on the ordering of the training data.
Check this out: Machine Learning Supervised vs Unsupervised Learning
Sensitive or Missing Data
Handling sensitive or missing data can be a challenge, especially when working with KNN, a method that doesn't perform well with noisy data.
KNN is particularly vulnerable to missing data, which can lead to inaccurate results and poor model performance.
If your data is sensitive to noise, you may want to consider using a different algorithm altogether, as KNN is not equipped to handle this type of data effectively.
For instance, if you're working with a dataset that's prone to missing values, you may need to explore other methods, such as imputation or data augmentation, to compensate for the missing data.
Unbalanced Data
Handling unbalanced data can be a challenge. K Nearest Neighbors, in particular, does not perform well with unbalanced data.
It's essential to recognize the issue early on to avoid poor model performance. With unbalanced data, the model may favor the majority class.
In such cases, it's not uncommon to see a high accuracy rate for the majority class, but a low accuracy rate for the minority class. This can lead to biased results.
To mitigate this issue, consider using techniques like oversampling the minority class or undersampling the majority class.
High-Dimensional Data
High-Dimensional Data can be a challenge for machine learning algorithms. Calculating distances between each data instance would be prohibitively expensive, making it difficult to work with large or high-dimensional data.
KNN is particularly affected by this issue, as it relies heavily on calculating distances between data points. In fact, KNN does not work well with high-dimensional data.
Frequently Asked Questions
Is KNN supervised or unsupervised?
The K-Nearest Neighbors (KNN) algorithm is a supervised machine learning algorithm. It relies on labeled data to classify new, unknown data points.
What is a real life example of KNN?
KNN is used in facial recognition systems to identify individuals in images, and in medical diagnosis to help doctors analyze gene expression and diagnose diseases
Why is KNN called lazy learner?
KNN is called a "lazy learner" because it doesn't build a model from training data, instead storing the entire dataset until a new data point is classified or predicted. This approach saves computation time, but requires more memory to store the entire dataset.
Sources
- https://scikit-learn.org/1.5/modules/neighbors.html
- https://www.geeksforgeeks.org/k-nearest-neighbours/
- https://www.analyticsvidhya.com/articles/knn-algorithm/
- https://zilliz.com/blog/k-nearest-neighbor-algorithm-for-machine-learning
- https://medium.com/@pingsubhak/machine-learning-basics-k-nearest-neighbors-9e8e2d46db75
Featured Images: pexels.com