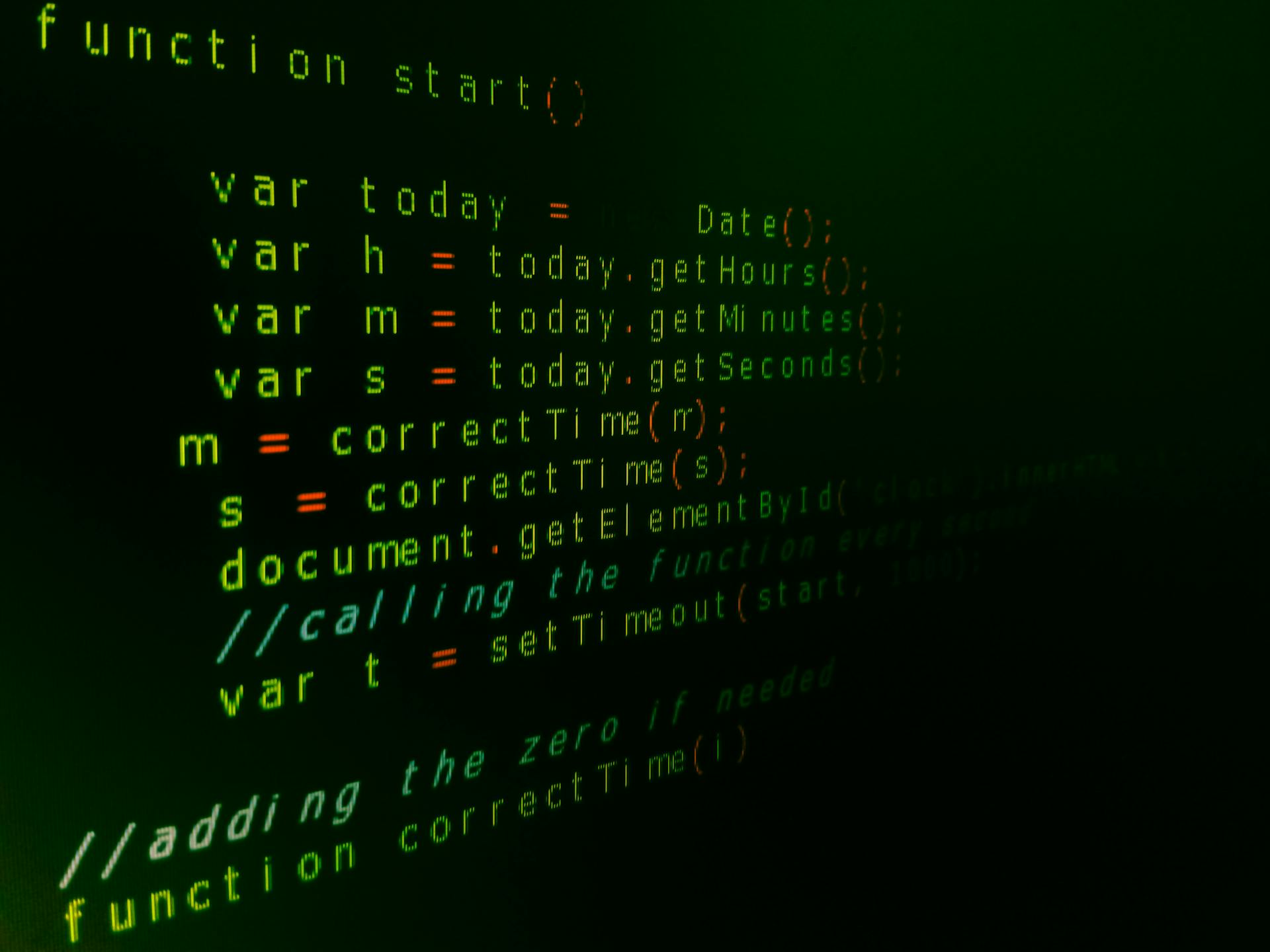
Start by understanding the basics of C++. C++ is a high-level, general-purpose programming language that was developed by Bjarne Stroustrup at Bell Labs in 1983.
C++ is an extension of the C programming language and adds object-oriented programming (OOP) features to it. This makes C++ a versatile language that can be used for a wide range of applications, from operating systems to web browsers.
To begin your C++ programming journey, you'll need to have a good grasp of the language's syntax and data types. C++ has a total of 32 data types, which can be classified into various categories such as numeric, character, and Boolean.
C++ syntax is relatively simple and easy to learn, with a focus on readability and maintainability. This makes it an ideal language for beginners to start with.
A fresh viewpoint: What Is the Hardest Code Language to Learn
Control Structures
Control Structures are a crucial part of programming, and in C++, they help you manage the flow of your code.
A Basic for Loop is a fundamental control structure that allows you to execute a block of code repeatedly for a specified number of times.
Loops can be used to perform repetitive tasks, such as finding the factorial of a number or generating a Fibonacci series.
You can also use Nested for Loops to tackle more complex problems, like finding the sum of digits in a number.
Here are some common types of loops you'll encounter in C++:
- Basic for Loop
- Basic while Loop
- Basic do-while Loop
- Nested for Loops
Basic Programs
Control Structures are the building blocks of programming, and understanding them is essential for writing efficient and effective code.
The journey begins with basic programs that introduce you to the fundamentals of programming. You start with the classic "Hello World" program, which is a great way to get familiar with your programming environment.
The "Hello World" program is often the first program you write when learning a new language, and it's a great way to ensure that your environment is set up correctly.
A unique perspective: Learn How to Code Google's Go Programming Language
One of the next steps is to learn how to take input from the user, which is done using functions like `gets()`.
Here are some basic programs that you should familiarize yourself with:
- Hello World
- Taking Input from User
- Find ASCII Value of Character
- Using gets() function
- If-Else
- Switch Case
- Checking for Vowel
- Reversing Case of Character
- Swapping Two Numbers
- Largest and Smallest using Global Declaration
Loops
Loops are a fundamental concept in programming that allow you to execute a set of statements repeatedly. This can be done using different types of loops.
A basic for loop is a type of loop that allows you to iterate over a sequence of elements. It's commonly used when you know the number of iterations in advance. For example, you can use a for loop to print the numbers from 1 to 10.
A while loop is another type of loop that allows you to execute a set of statements as long as a certain condition is met. It's commonly used when you don't know the number of iterations in advance. For instance, you can use a while loop to print the numbers from 1 to 10, but this time, the loop will continue as long as the number is less than or equal to 10.
You might like: How Long Does It Take to Learn Morse Code
A do-while loop is similar to a while loop, but it executes the loop body at least once before checking the condition. This is useful when you want to ensure that the loop body is executed at least once. For example, you can use a do-while loop to print the numbers from 1 to 10, and the loop will continue as long as the number is less than or equal to 10.
Here are some common types of loops:
- Basic for Loop
- Basic while Loop
- Basic do-while Loop
- Nested for Loops
Loops can be used to solve a variety of problems, such as finding the factorial of a number. The factorial of a number is the product of all positive integers less than or equal to that number. For instance, the factorial of 5 is 5 * 4 * 3 * 2 * 1 = 120.
Pointers
Pointers are a fundamental concept in programming that can be a bit tricky to grasp at first, but don't worry, I'm here to help you understand them better.
Pointers are variables that store memory addresses as their values. They can be used to access and manipulate data in memory.
You can use pointers to create simple programs, which is a great way to get started with programming. For instance, you can use pointers to implement memory management, which is essential for any program that needs to allocate and deallocate memory dynamically.
Pointers can also be used to work with arrays of pointers, which is a powerful technique for handling complex data structures. This is especially useful when you need to store multiple pointers to different data types.
To navigate through memory using pointers, you can use the increment and decrement operators, which allow you to move the pointer to the next or previous memory location.
Pointers can also be compared to each other, which is useful for checking if two pointers point to the same memory location.
In some cases, you may need to work with pointers to pointers, which is a bit more advanced but still a useful technique to know.
Here are some common operations you can perform with pointers:
Pointers can also be used to concatenate strings, which is a common task in programming. And, if you need to reverse a string, pointers can help you do that too.
Finally, pointers can be used to swap two numbers, which is a simple but useful technique to know. They can also be used to point to functions, which is a more advanced topic but still worth exploring. And, of course, there's the null pointer, which is a special type of pointer that points to no memory location at all.
Concept of Recursion
Recursion is a fundamental concept in programming that can be a bit tricky to grasp at first, but it's actually quite simple once you understand it. Recursion is a way of solving problems by breaking them down into smaller versions of the same problem.
One of the most basic examples of recursion is adding two numbers. For instance, if you want to add 3 and 4, you can break it down into smaller problems like adding 3 and 1, and then adding 1 and 4, and finally adding 3 and 4.
Recursion is often used in mathematical problems like finding the factorial of a number. The factorial of a number is the product of all positive integers less than or equal to that number. For example, the factorial of 5 is 5 x 4 x 3 x 2 x 1.
The Fibonacci series is another classic example of recursion, where each number is the sum of the two preceding numbers. This sequence starts with 0 and 1, and each subsequent number is the sum of the previous two.
Recursion can also be used to find the sum of the first n numbers, where n is a given number. For instance, if you want to find the sum of the first 5 numbers, you can break it down into smaller problems like finding the sum of the first 4 numbers and adding 5 to it.
Here are some common examples of recursive problems:
- Adding Two Numbers
- Factorial
- Fibonacci Series
- Sum of First N Numbers
- Sum of Digits
- Palindrome
- Power of N
- Largest Array Element
- Prime or Composite
- LCM of Two Numbers
- GCD of Two Numbers
- Reverse a String
Recursion can be a powerful tool in programming, but it can also be tricky to understand and use correctly. With practice and experience, however, you can master the art of recursion and solve complex problems with ease.
Featured Images: pexels.com