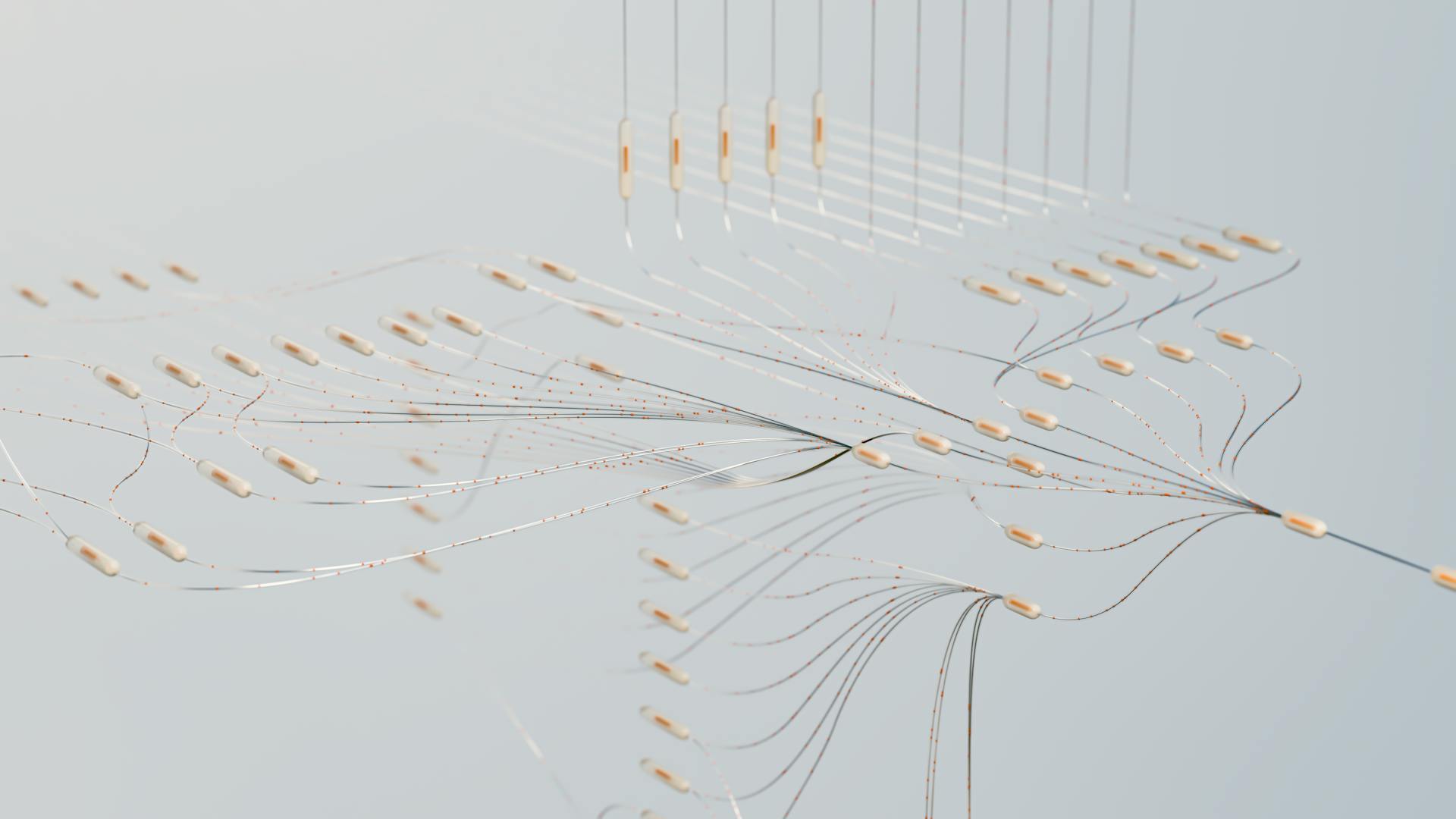
PyTorch Scaled Dot Product Attention is a game-changer for machine learning models, allowing them to focus on the most relevant information in a sequence.
It's based on the Transformer architecture, which revolutionized the field of natural language processing by abandoning the traditional recurrent neural network approach.
At its core, Scaled Dot Product Attention uses a scaled dot product to compute the attention weights, which are then used to compute the output of the attention mechanism.
This process involves three main components: query, key, and value vectors, which are all learned during training.
What Is Scaled Dot Product Attention?
Scaled Dot Product Attention is a type of attention mechanism used in transformer models, allowing the model to weigh the importance of different input elements.
This mechanism was first introduced in the paper "Attention Is All You Need" by Vaswani et al., which proposed the transformer architecture.
The scaled dot product attention mechanism calculates the attention weights by taking the dot product of the query and key vectors, dividing by the square root of the key vector's dimension, and then applying a softmax function.
This is done to prevent extremely large values from dominating the attention weights, which can cause the model to focus too much on a single input element.
The formula for scaled dot product attention is QK^T / sqrt(d) * V, where Q is the query vector, K is the key vector, V is the value vector, and d is the dimension of the key vector.
Transformer Encoder
The Transformer Encoder is a crucial component in the Transformer architecture. It's responsible for encoding the input sequence into a continuous representation that can be used by the decoder.
This encoding process is done through a series of self-attention mechanisms, which allow the model to weigh the importance of each input element relative to the others.
Calculating Scores and Weights
Calculating attention scores involves taking the dot-product of query and key vectors, which gives a measure of how much focus one token should have on another.
The dot-product values can become large, especially with high-dimensional embeddings. To avoid this, we scale the dot-products by dividing by the square root of the dimension of the key vectors.
Scaling is crucial to ensure model training stability and prevent computational issues such as vanishing or exploding gradients.
The scaling factor doesn't change the distribution of attention scores, but ensures they remain on a manageable scale.
If we assume query and key vectors are independent random variables with a mean of zero and a variance of one, their dot-product has a mean of zero but a variance equal to the number of dimensions.
We prefer these values to have a variance equal to one, which demands a scaling factor.
Here's a step-by-step summary of the scaled dot-product attention mechanism:
- Compute dot-product of query and key vectors
- Scale dot-products by dividing by the square root of the dimension of the key vectors
- Apply softmax function to the scaled scores to obtain the scaled weights
- Use the scaled weights to compute a weighted sum of the value vectors
Query, Key, and Value Matrices
To compute the query, key, and value vectors, we perform three matrix multiplications on each feature vector using three learnable weight matrices.
Each feature vector has size d, a row-vector of dimensionality 1 by d, and concatenating these features will form a matrix X with 11 rows and d columns.
The three weight matrices are learnable with a size of d×d, and they are shared among all elements of the input sequence X.
We can compute the query, key, and value vectors sequentially by going through each feature xᵢ from i=1 to i=11, or more efficiently by multiplying the entire feature sequence X by the weight matrices.
In practice, it's easier to make the query, key, and value vectors have the same dimensionality to simplify tracking their dimensionalities, so we use the same dimensionality d=dₖ=dᵥ for all three vectors.
This ensures that the dot product between the query and key vectors results in a matrix with appropriate dimensions to compute the attention weights, which are then used to compute the weighted sum of the value vectors.
Detailed Explanation
In the Scaled Dot-Product Attention mechanism, the query, key, and value matrices are crucial components.
The query matrix Q, key matrix K, and value matrix V are used in the simplest case, where the same embeddings are used for all three.
Matrix multiplication is used to compute the dot product of the query matrix Q and the transpose of the key matrix K.
The dot products are scaled by dividing by the square root of the dimension of the key vectors (dk = 3).
The softmax function is applied to the scaled dot products to obtain the attention weights.
Here's a breakdown of the softmax function:
- The softmax function is applied to each row of the scaled logits to get the attention weights.
- For the first row, the attention weights are computed as follows: attention_weights[0]=softmax([0.081,0.185,0.289,0.392,0.081,0.496])
- This process is repeated for each row in the scaled attention logits to get the full attention weight matrix.
The attention weights show how much attention the first token "the" should pay to each token in the sequence, including itself.
The token "mat" has the highest weight, indicating it is the most relevant for "the" in this context.
To compute the output, the attention weights are multiplied by the value matrix V.
For the first token "the", the output is computed as follows: the output is the result of multiplying the attention weights by the value matrix V.
Fused Implementations and Optimizations
PyTorch's scaled dot product attention has several fused implementations that optimize its performance.
For CUDA tensor inputs, the function will dispatch into one of the following implementations:
- FlashAttention: Fast and Memory-Efficient Exact Attention with IO-Awareness
- Memory-Efficient Attention
- A PyTorch implementation defined in C++
These implementations are designed to improve the efficiency of the scaled dot product attention mechanism, making it more suitable for large-scale applications.
Fused Implementations
Fused implementations are a key feature of the function in question. They allow for faster and more memory-efficient processing of CUDA tensor inputs.
There are three main implementations to consider: FlashAttention, Memory-Efficient Attention, and a PyTorch implementation defined in C++. Each has its own strengths and use cases.
FlashAttention, for example, is known for its fast and memory-efficient exact attention with IO-awareness. This makes it particularly well-suited for large-scale applications.
Memory-Efficient Attention, on the other hand, is optimized for memory usage and is a good choice when working with limited resources.
A PyTorch implementation defined in C++ is also available, offering a more traditional approach to attention mechanisms.
Here are the three implementations listed out for easy reference:
- FlashAttention: Fast and Memory-Efficient Exact Attention with IO-Awareness
- Memory-Efficient Attention
- A PyTorch implementation defined in C++
Hardware Dependence
Hardware dependence can greatly impact the performance of your code.
If you're running on a CPU without a GPU, your results might be different because the context manager will have no effect, and all three runs should return similar timings.
The compute capability of your graphics card also matters. Depending on what it supports, some implementations like flash attention or memory efficient might fail.
Running on different hardware can lead to varying results, so it's essential to consider your machine's capabilities when testing and optimizing your code.
Visualizing and Understanding Weights
To visualize the attention weights of a model, you can use a heatmap. This allows you to better understand the model's attention mechanism.
Using Matplotlib, you can create a heatmap to display the attention weights. This is a simple and effective way to gain insight into how the model is paying attention to different parts of the input.
The output of this visualization can be quite revealing, showing you exactly where the model is focusing its attention. It's a great tool for debugging and fine-tuning your model.
By examining the heatmap, you can get a sense of which parts of the input are most important to the model. This can help you identify areas where the model might be struggling or where you can make improvements.
IV. Conclusion
The torch.nn.functional.scaled_dot_product_attention function is a powerful tool in PyTorch that helps us find correlations and similarities between input elements.
We've seen how it can be used in the context of the Transformer architecture, which is a very important and recent architecture that can be applied to many tasks and datasets.
The Multi-Head Attention layer, a key component of the Transformer, uses a scaled dot product between queries and keys to achieve this.
This architecture has been successfully applied to sequence-to-sequence tasks and set anomaly detection, showcasing its versatility.
It's also worth noting that the Transformer architecture is permutation-equivariant if no positional encodings are provided, allowing it to generalize to many settings.
To get the most out of the Transformer architecture, it's essential to be aware of potential issues, such as the gradient problem during the first iterations, which can be solved by learning rate warm-up.
Sources
- https://medium.com/@saraswatp/understanding-scaled-dot-product-attention-in-transformer-models-5fe02b0f150c
- https://www.i32n.com/docs/pytorch/tutorials/intermediate/scaled_dot_product_attention_tutorial.html
- https://medium.com/@vmirly/tutorial-on-scaled-dot-product-attention-with-pytorch-implementation-from-scratch-66ed898bf817
- https://uvadlc-notebooks.readthedocs.io/en/latest/tutorial_notebooks/tutorial6/Transformers_and_MHAttention.html
- https://pub.aimind.so/scaled-dot-product-self-attention-mechanism-in-transformers-870855d65475
Featured Images: pexels.com