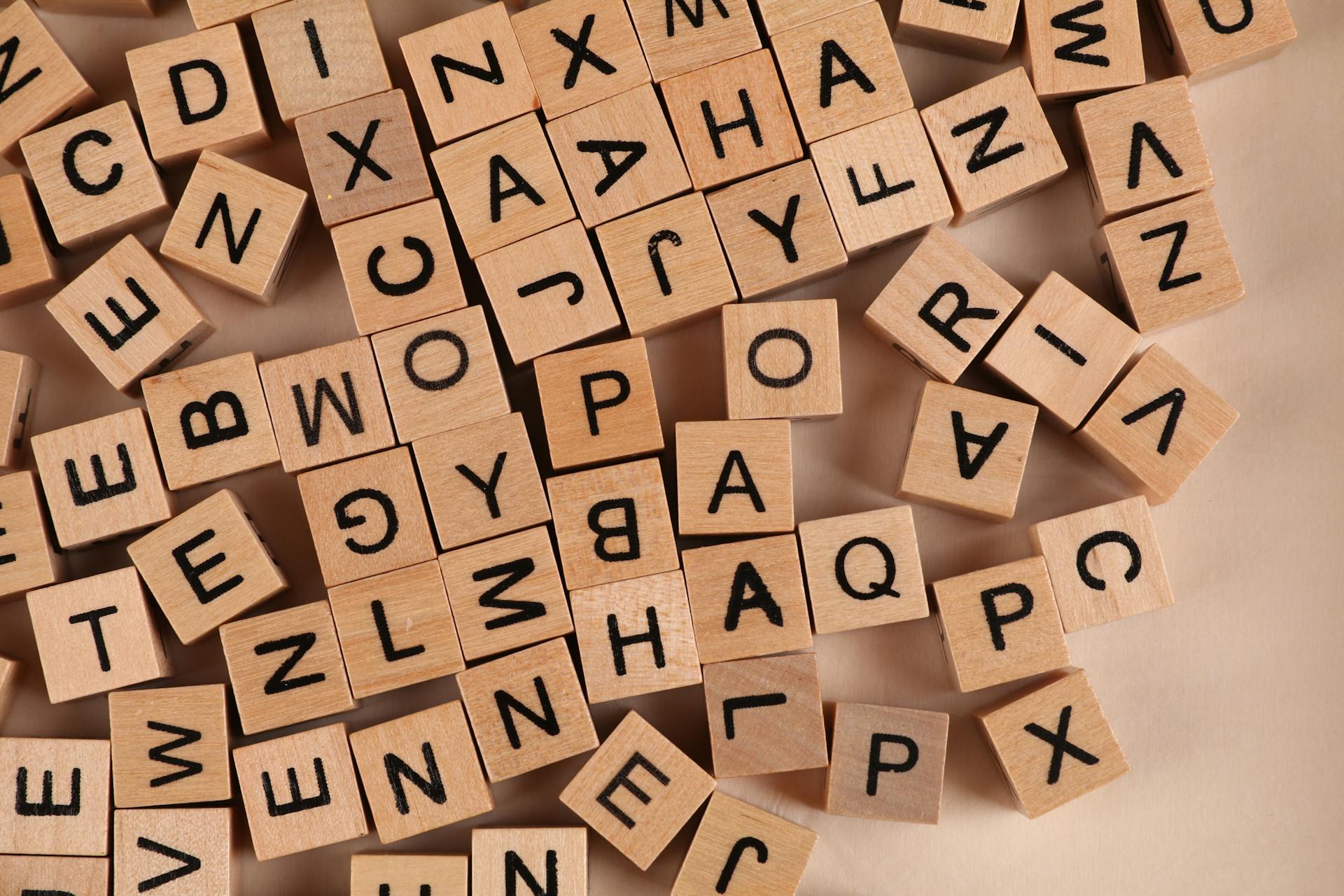
Building a suffix array is a fundamental step in many text processing algorithms. It's a data structure that stores all the suffixes of a given text in a sorted order.
The suffix array construction process starts by sorting the suffixes of the text, which can be done in O(n log n) time using a comparison-based sorting algorithm. This is because there are n suffixes to sort.
A good example of a comparison-based sorting algorithm for suffix array construction is the merge sort algorithm. It works by recursively dividing the suffixes into smaller chunks, sorting each chunk, and then merging the sorted chunks back together.
The choice of sorting algorithm can significantly impact the performance of the suffix array construction process. For instance, using a sorting algorithm like quicksort can lead to a worst-case time complexity of O(n^2).
Suffix Array Construction
Building a Suffix Array can be a straightforward process. There are more advanced algorithms available, but a simple and naive method exists.
This method, although not the most efficient, is a good starting point for understanding the basics of Suffix Array construction. It can be used as a foundation for further learning and improvement.
The naive method is a good option for small datasets or educational purposes, as it can help illustrate the concept of Suffix Arrays in a clear and easy-to-understand way.
Naive Method
The Naive Method is a simple approach to building a Suffix Array, but it's not the most efficient way to do it.
This method involves creating a Suffix Array by sorting all the suffixes of a given string. Although there are more advanced algorithms available, this naive method can still be a good starting point for understanding the basics of Suffix Array construction.
The time complexity of this method is O(n^2 log n), which is relatively slow compared to other algorithms. However, it's a great way to see how the Suffix Array is built step by step.
One of the key advantages of the Naive Method is that it's easy to understand and implement. However, it's not suitable for large datasets due to its high time complexity.
To use the Naive Method, you'll need to start by creating a list of all the suffixes of the input string. Then, you'll need to sort this list in lexicographic order to create the Suffix Array.
The Naive Method is a good way to learn about Suffix Arrays and how they're used in string matching and searching algorithms.
Output
The output of the suffix array construction algorithm represents the sorted suffixes of the original string in lexicographical order.
Each number in the output array corresponds to the starting index of a suffix in the original string.
The suffix array is 0-based, meaning the indices start from 0.
The output for the string banana is [5, 3, 1, 0, 4, 2], which is a list of starting indices for the sorted suffixes.
The sorted suffixes corresponding to this output are the suffixes of the string banana in lexicographical order.
Note that the indices in the output array are not the actual suffixes themselves, but rather the starting positions of the suffixes in the original string.
Arrays
Arrays are a fundamental data structure used in suffix array construction. They allow us to store and manipulate large amounts of data efficiently.
A suffix array is essentially a sorted array of all suffixes of a given string. This means that each element in the array represents a suffix of the original string.
Suffix arrays are typically implemented as a 1D array, where each element is a pair of indices and a value. The value is the actual suffix, and the indices are used to keep track of its position in the original string.
The size of the suffix array is directly proportional to the size of the input string. For example, if the input string has 10 characters, the suffix array will have 10 elements, each representing a suffix of the string.
Suffix arrays can be used for various applications, including string matching and pattern searching. They are particularly useful when dealing with large datasets, as they allow for efficient searching and manipulation of data.
The time complexity of constructing a suffix array is typically O(n log n), where n is the size of the input string. This is because the sorting operation dominates the construction process.
Python 3
In Python 3, building a suffix array is a bit more involved, but the basic idea remains the same. The algorithm starts by creating a structure to store information about each suffix, including its original index and rank.
The rank is calculated based on the first two characters of the suffix, with each character's ASCII value subtracted by the ASCII value of 'a'. This gives a unique rank for each suffix, which is used for sorting.
To sort the suffixes, the algorithm uses a custom comparison function that compares the ranks and next ranks of two suffixes. If the ranks are different, the suffix with the lower rank comes first. If the ranks are the same, the suffix with the lower next rank comes first.
The sorting process is repeated multiple times, with the length of the sorting key increasing by a factor of 2 each time. This is done to ensure that the suffixes are sorted correctly based on the first 4, 8, 16, and so on, characters.
Here's a simplified table showing the steps involved in building a suffix array in Python 3:
The final step is to store the indexes of the sorted suffixes in the suffix array, which is then returned as the result. The time complexity of this algorithm is O(n Log(n) Log(n)), but can be improved to O(n Log n) using Radix Sort.
Applications and Properties
Suffix arrays are incredibly versatile, and their applications are numerous. They're used in pattern searching algorithms, such as the longest common prefix (LCP) algorithm and the enhanced version of the Knuth-Morris-Pratt (KMP) algorithm.
These algorithms use suffix arrays to quickly locate occurrences of a pattern within a text string, making them super efficient.
Text compression is another area where suffix arrays shine, particularly with the Burrows-Wheeler Transform (BWT). This algorithm rearranges the characters of a text string to enhance the compression ratio, and suffix arrays play a crucial role in constructing the BWT.
In bioinformatics, suffix arrays are extensively used in genome assembly algorithms. These algorithms reconstruct the complete DNA sequence of an organism by aligning and merging smaller DNA fragments, and suffix arrays enable efficient matching and comparison of DNA sequences.
Suffix arrays are also employed in various data mining applications, including substring matching, similarity searches, and sequence alignment. They're particularly useful in bioinformatics for tasks like DNA sequence analysis, gene identification, and protein motif discovery.
Here are some of the key applications of suffix arrays:
- Pattern Searching: Suffix arrays are used in algorithms like LCP and KMP to quickly locate occurrences of a pattern within a text string.
- Text Compression: Suffix arrays are employed in the Burrows-Wheeler Transform (BWT) to enhance the compression ratio.
- Genome Assembly: Suffix arrays enable efficient matching and comparison of DNA sequences in genome assembly algorithms.
- Data Mining and Bioinformatics: Suffix arrays are used in tasks like substring matching, similarity searches, and sequence alignment.
- Text Indexing: Suffix arrays are useful for creating text indexes, enabling rapid searches and retrieval of specific information within a large text corpus.
- Data Compression: Suffix arrays help in efficiently encoding and decoding sequences of symbols, resulting in reduced storage requirements and improved compression ratios.
Suffix arrays can also be utilized to find the longest common substring among multiple strings, making them a valuable tool in bioinformatics and data analysis.
Sources
- https://www.scholarhat.com/tutorial/datastructures/suffix-array-and-suffix-tree-in-data-structures
- https://www.hackerearth.com/practice/data-structures/advanced-data-structures/suffix-arrays/tutorial/
- https://www.geeksforgeeks.org/suffix-array-set-1-introduction/
- https://visualgo.net/en/suffixarray
- https://www.geeksforgeeks.org/suffix-array-set-2-a-nlognlogn-algorithm/
Featured Images: pexels.com