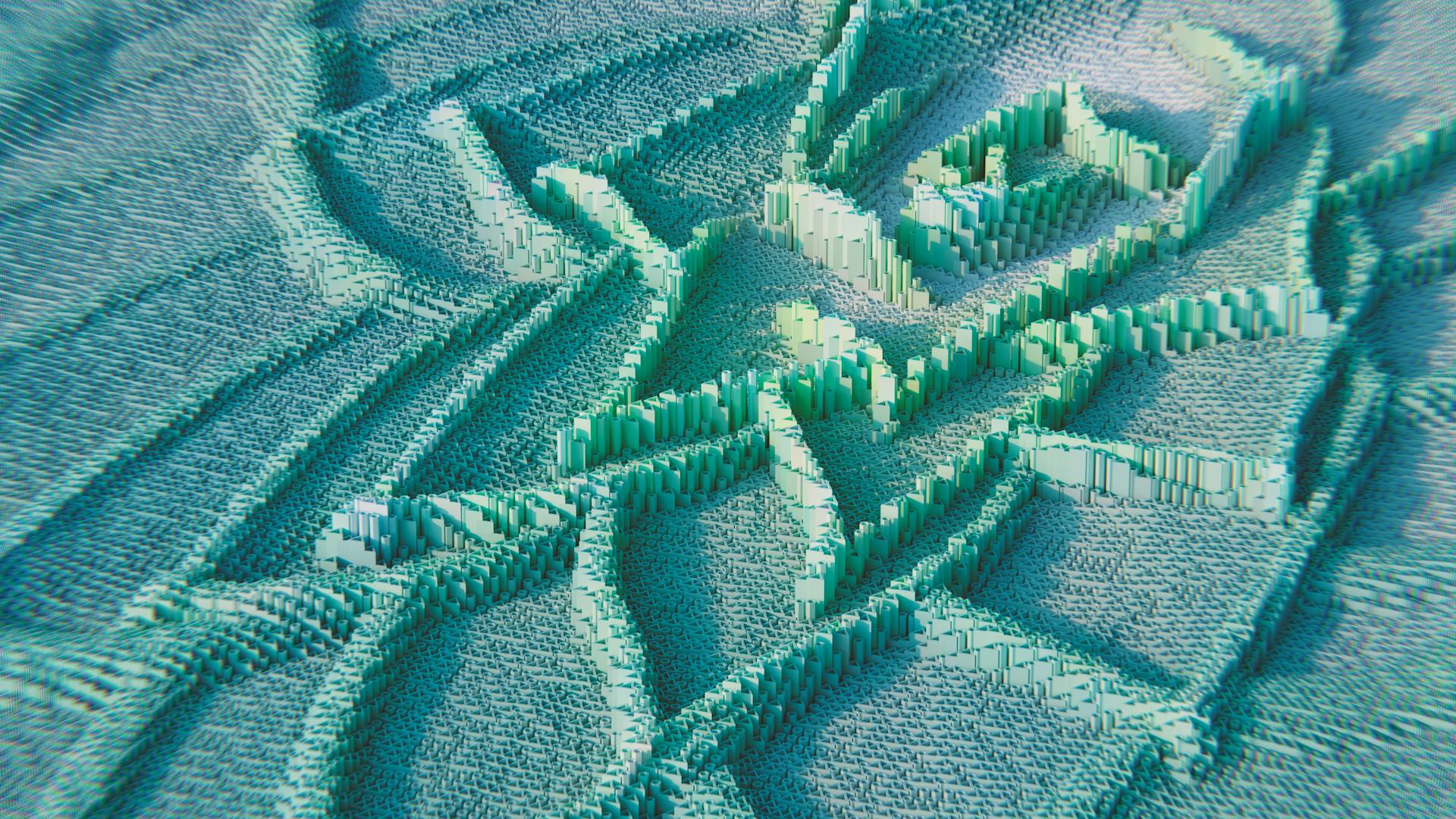
AP Comp Sci A is a challenging course that requires a solid foundation in programming concepts. Understanding data structures is crucial, as they are used to organize and store data in a way that makes it easily accessible and usable.
The course covers a range of data structures, including arrays, linked lists, stacks, and queues. Each of these data structures has its own strengths and weaknesses, and understanding when to use each one is key to writing efficient code.
To succeed in AP Comp Sci A, it's essential to have a good grasp of programming fundamentals, including variables, control structures, and functions. These concepts are the building blocks of more advanced topics, so make sure you have a solid understanding of them before moving on.
Practice is key to mastering these concepts, so be sure to work on plenty of problems and exercises to reinforce your understanding.
Data Types
Data Types are the foundation of any programming language, and in Java, they're no exception. Variables can hold different types of data, such as integers, floats, and characters.
In Java, primitive data types include integers, floats, and characters. These types are used to store and manipulate simple data.
Casting is a technique used to convert one data type to another, but it's not always possible. For example, you can't cast a string to an integer.
Understanding the ranges of variables is crucial to avoid errors. For instance, the range of an integer in Java is -2,147,483,648 to 2,147,483,647.
Primitive Types
Primitive types are the fundamental building blocks of programming, and in Java, they're used to store and manipulate data.
Variables are used to store values in a program, and they can hold primitive data types such as numbers, characters, and booleans.
In programming, casting is the process of converting one data type to another, which can be useful when working with variables.
The range of a variable depends on its data type, with some types having a wider range than others.
String methods are used to manipulate and analyze strings, which are sequences of characters.
Java is a popular programming language that's widely used in many industries, and it's known for its simplicity and ease of use.
Variables can hold a range of values, from a single character to a large number, depending on their data type.
In Java, strings are a type of primitive data type that's used to store sequences of characters.
Array Basics
Arrays are a fundamental data type in programming, and understanding their basics is crucial for efficient coding.
Arrays are collections of elements of the same data type stored in contiguous memory locations, making them a vital tool for storing and manipulating large datasets.
In AP Computer Science A, Unit 6 focuses on array basics, where you'll learn about array creation and access. This involves understanding how to declare and initialize arrays, as well as how to access and modify their elements.
Arrays can be traversed using various methods, including the Enhanced For Loop, which simplifies the process of iterating over array elements.
Developing algorithms using arrays is a critical skill, as it enables you to create efficient and effective solutions to complex problems.
Boolean Logic
Boolean Logic is a fundamental concept in computer science that allows us to make decisions based on conditions.
Boolean expressions are the building blocks of Boolean Logic, consisting of variables, operators, and values.
In programming, we use Boolean expressions to evaluate conditions, and the result is either True or False.
If-Else statements are a type of control flow that allows us to execute different blocks of code based on a condition.
Else If statements are used when we need to check multiple conditions and execute different blocks of code accordingly.
Compound Boolean expressions allow us to combine multiple conditions using logical operators, making our code more efficient.
Equivalent Boolean expressions can be simplified to make our code more readable and maintainable.
For another approach, see: How Can Young Kids Learn How to Code
Control Structures
Control Structures are the backbone of programming, allowing you to control the flow of your code and make decisions based on conditions. They're essential for writing efficient and effective algorithms.
Loops are a type of control structure that enable you to repeat a set of instructions, making it ideal for tasks that require repetition. For example, a while loop can be used to iterate over a list of items until a certain condition is met.
For loops, on the other hand, allow you to iterate over a specific range or collection, making it perfect for tasks that require a fixed number of iterations. This can be particularly useful when working with strings or other data structures.
Nested iteration is also a key concept in control structures, allowing you to iterate over multiple levels of data. This can be useful when working with complex data structures, such as arrays or matrices.
Iteration
Iteration is a fundamental concept in programming that allows you to repeat a set of instructions. In the context of AP Computer Science A, iteration is covered in Unit 4 – Iteration in Programming.
Iteration is used to traverse arrays and 2D arrays, allowing you to access and manipulate their elements. You can use while loops, for loops, and nested iteration to develop algorithms that work with strings and other data structures.
The Enhanced For Loop is a special type of loop that is specifically designed for arrays, making it easier to traverse and access their elements. This loop is introduced in Unit 6 – Array Basics in AP Computer Science A.
Nested iteration is used to traverse 2D arrays, which are discussed in Unit 8 – 2D Arrays in AP Computer Science A. This type of iteration allows you to access and manipulate the elements of a 2D array in a more complex and flexible way.
While loops and for loops are also used for iteration, and are covered in Unit 4 – Iteration in Programming. These types of loops allow you to repeat a set of instructions until a certain condition is met, or to iterate over a collection of elements.
Recursion
Recursion is a fundamental concept in programming that allows a function to call itself repeatedly until it reaches a base case that stops the recursion.
It's a powerful technique used in various applications, including searching and sorting algorithms.
In Unit 10, we learn about Recursion and its importance in programming.
Recursive functions can be used to solve problems that have a recursive structure, meaning they can be broken down into smaller sub-problems of the same type.
This approach can be more efficient and elegant than using loops, especially for problems that have a recursive nature.
Recursion is used in Unit 10 – Recursion, where we explore its applications in searching and sorting.
The base case is crucial in recursion, as it provides a stopping point for the recursive function to prevent infinite loops.
A well-designed recursive function can make code easier to understand and maintain.
In the context of recursion, searching and sorting are two key areas where this technique is applied.
By understanding recursion, you can write more efficient and effective code that solves complex problems.
Recursion can be a bit tricky to grasp at first, but with practice and experience, it becomes second nature.
Explore further: Black Girls Code
Sources
- https://library.fiveable.me/ap-comp-sci-a
- https://knowt.com/exams/AP/AP-Computer-Science-A
- https://blog.collegevine.com/the-ultimate-guide-to-the-ap-computer-science-a-exam
- https://www.albert.io/blog/one-month-ap-computer-science-study-guide/
- https://www.crimsoneducation.org/us/blog/navigating-ap-computer-science-a-a-comprehensive-guide/
Featured Images: pexels.com