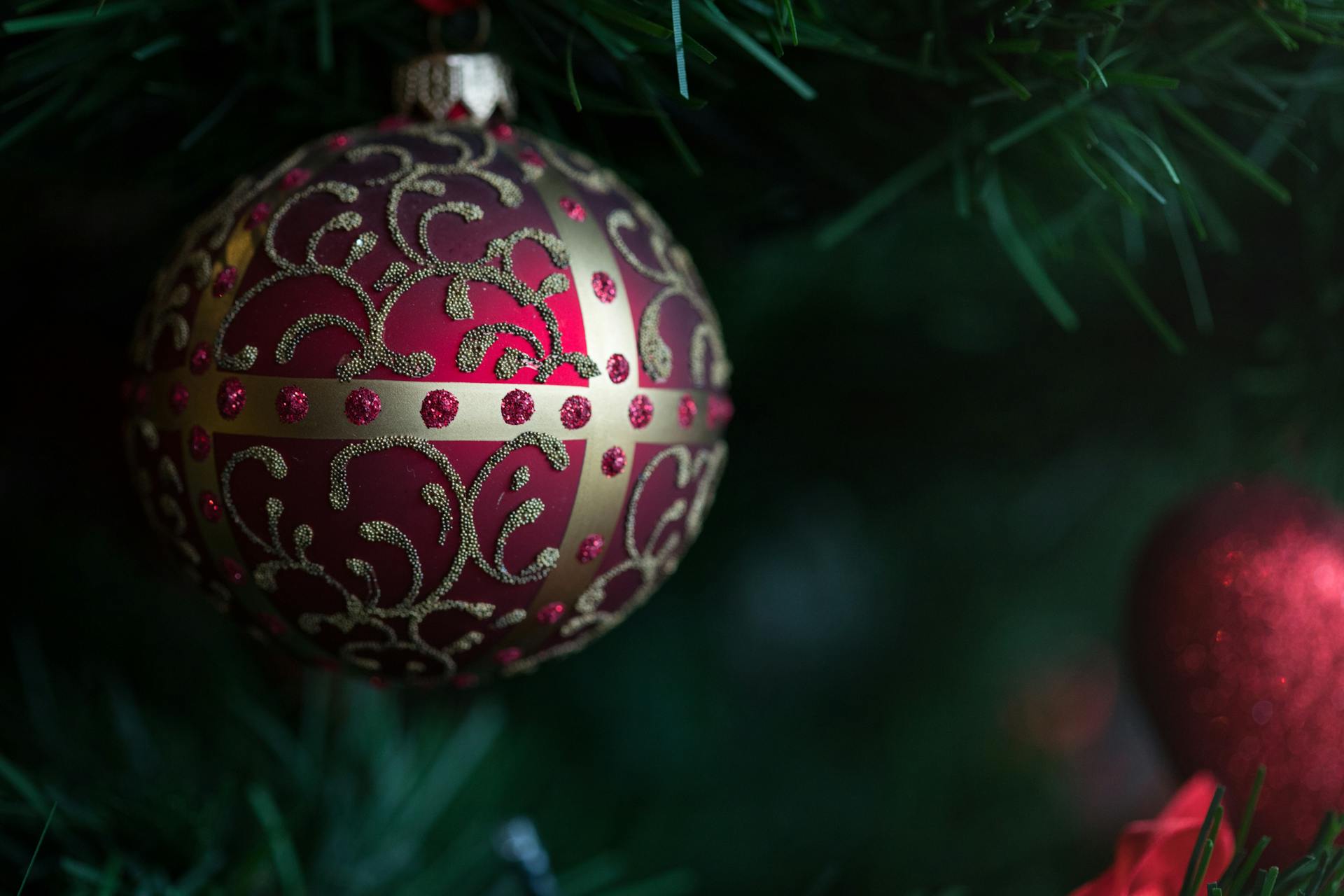
A ball tree is a data structure that's really useful for organizing and searching through large datasets. It's particularly effective when dealing with high-dimensional data.
The ball tree data structure is based on the concept of a ball, which is essentially a sphere that encompasses a set of points in the data. This makes it easy to search for similar points within the data.
One of the key benefits of a ball tree is its ability to reduce the number of comparisons needed to find a match. By pruning branches that are unlikely to contain the desired point, the search time is significantly reduced.
Additional reading: Decision Tree Algorithm Machine Learning
What is a Ball Tree?
A ball tree is a binary tree where each node defines a D-dimensional ball containing a subset of the points to be searched. This tree is useful for organizing data points in a way that makes searching and querying more efficient.
Each internal node of the tree partitions the data points into two disjoint sets associated with different balls, which are defined by their distance from the ball's center. This property makes ball trees particularly effective for nearest-neighbor searches.
Take a look at this: Pruning in Decision Tree
The balls themselves may intersect, but each point is assigned to one or the other ball in the partition according to its distance from the ball's center. This ensures that each leaf node in the tree defines a ball and enumerates all data points inside that ball.
Each node in the tree defines the smallest ball that contains all data points in its subtree. This property is useful for quickly estimating the distance between a test point and any point in the tree.
The ball tree algorithm is related to the M-tree, but only supports binary splits, which results in a shallower tree structure and fewer distance computations. This usually yields faster queries, making the ball tree algorithm a popular choice for nearest-neighbor searches.
Construction and Properties
The k-d construction algorithm is a top-down process that builds the tree by recursively splitting the data points into two sets. It chooses splits along the dimension with the greatest spread of points, partitioning the sets by the median value.
This algorithm operates on the entire data set at once, making it an offline procedure. Finding the split for each internal node requires linear time in the number of samples contained in that node.
The time complexity of this algorithm is O(nlogn), where n is the number of data points, resulting in efficient construction of the tree.
Description
The ball tree nearest-neighbor algorithm is a powerful tool for searching through large datasets. It's a depth-first search that starts at the root and examines nodes in a specific order.
At each node, the algorithm performs one of three operations: it ignores the node if the distance from the test point to the node is greater than the furthest point in the priority queue, scans through every point in the node if it's a leaf node, or recursively calls itself on the node's two children if it's an internal node.
The algorithm maintains a max-first priority queue, often implemented with a heap, to keep track of the k nearest points encountered so far.
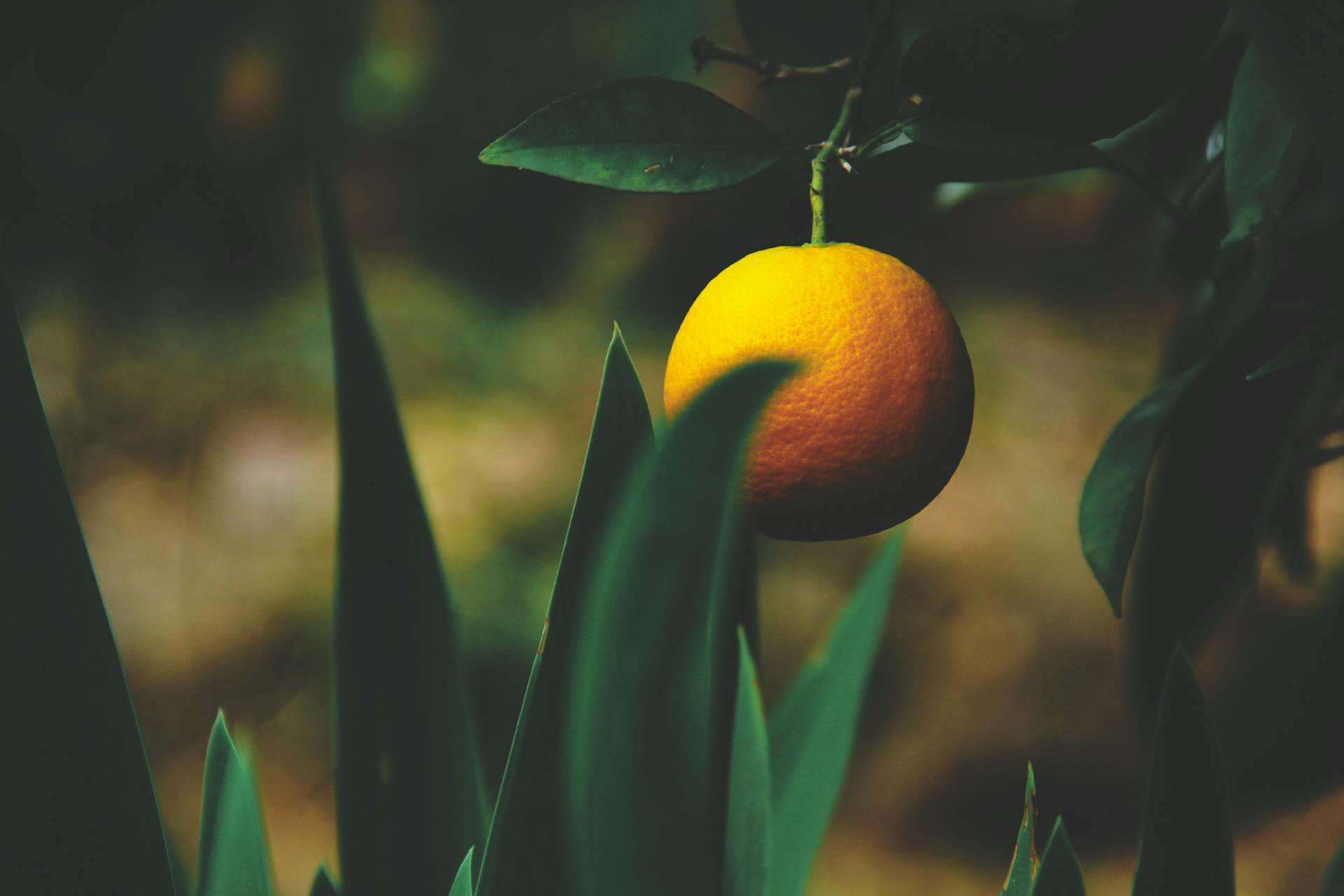
The order in which the algorithm searches the children of an internal node is crucial - searching the child whose center is closer to the test point first increases the likelihood that the further child will be pruned entirely during the search.
Here's a summary of the algorithm's operations:
- Ignore the node if the distance from the test point to the node is greater than the furthest point in the priority queue.
- Scan through every point in the node if it's a leaf node.
- Recursively call the algorithm on the node's two children if it's an internal node.
K-D Construction
The k-d construction algorithm is an offline procedure that operates on the entire data set at once.
This algorithm builds the tree top-down by recursively splitting the data points into two sets. Splits are chosen along the single dimension with the greatest spread of points.
The sets are partitioned by the median value of all points along that dimension. This process requires linear time in the number of samples contained in that node.
Finding the split for each internal node takes linear time. This yields an algorithm with a time complexity of O(n log n), where n is the number of data points.
Use Cases and Scenarios
The Ball Tree algorithm is a great choice for certain types of data. It's often preferred for sparse, high-dimensional data where hypersphere partitioning is effective.
In these scenarios, the Ball Tree algorithm excels in nearest neighbor searches. It's used in applications like this, where it can efficiently find the closest matches.
The Ball Tree algorithm is particularly well-suited for data with low to moderate dimensions. This makes it a great option for many real-world applications.
Expand your knowledge: Top Machine Learning Applications at Fin Tech Companies
Suitable Scenarios
The Ball Tree algorithm is a valuable tool for specific scenarios. It's more suitable for sparse, high-dimensional data where hypersphere partitioning is effective.
In such cases, the algorithm excels and is used in applications like nearest neighbor searches. This is because it can efficiently handle low to moderate dimensions.
One key application of ball trees is expediting nearest neighbor search queries. These queries aim to find the k points in the tree that are closest to a given test point by some distance metric.
A simple search algorithm, KNS1, can be used to exploit the distance property of the ball tree. This algorithm ignores any subtree whose ball is further from the test point than the closest point encountered so far.
Works?
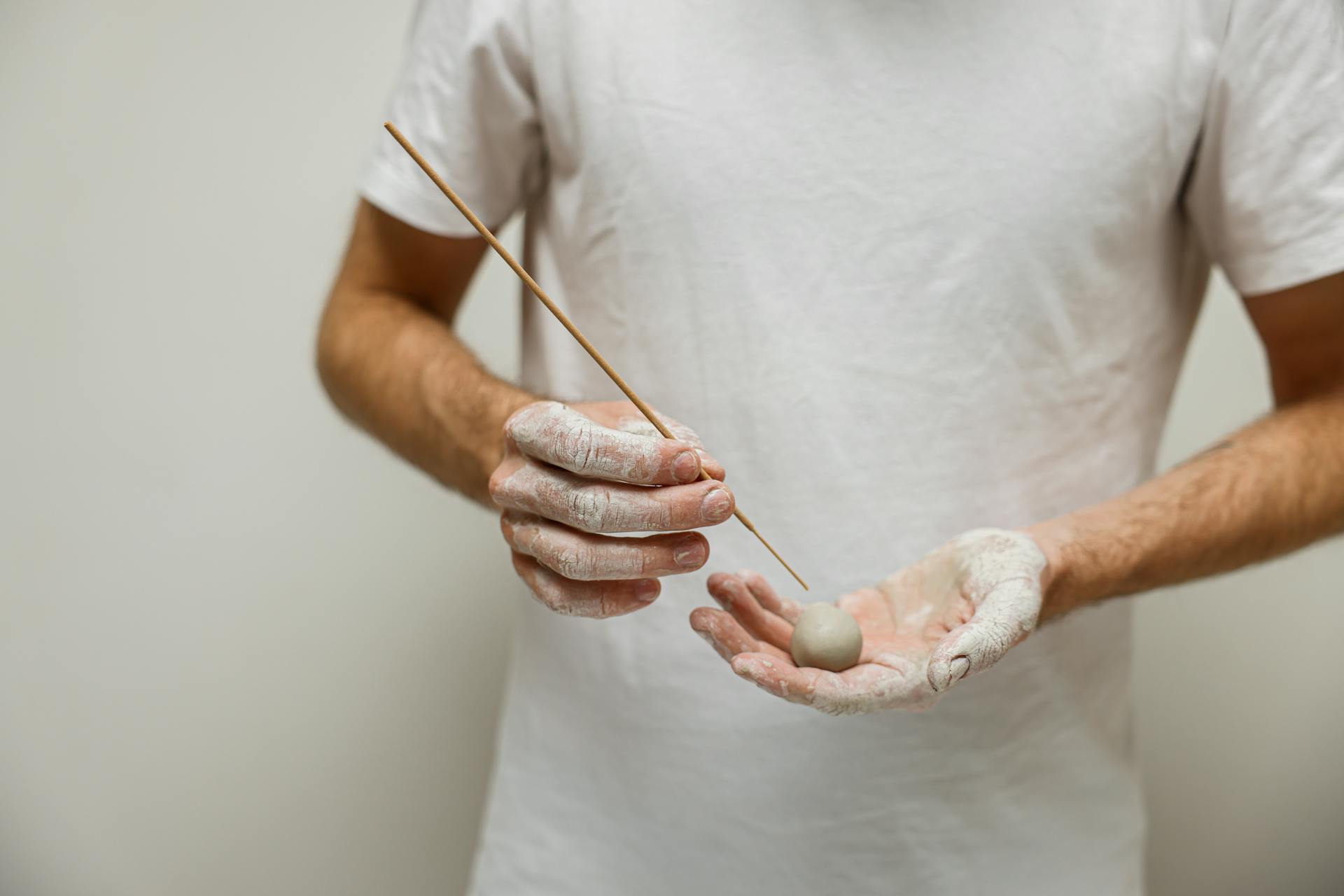
The Ball tree algorithm is specifically designed for nearest neighbor searches, which makes it particularly useful in situations where you need to quickly find the closest match in a large dataset.
It creates a data structure that enables efficient multidimensional search operations by dividing the data into subsets and choosing a dimension to create a binary tree between them.
This binary tree structure is especially helpful in high-dimensional spaces, where conventional distance computations can be computationally costly.
The Ball tree algorithm allows for the prompt removal of distant points during nearest neighbor searches, which significantly speeds up the process.
It does this by surrounding each subset of data points with a hypersphere, characterized by a radius and a centroid.
This efficient data structure is exactly what you need when dealing with large datasets and complex search operations.
Related reading: How to Code Binary Classifier in Python
Implementation and Comparison
The ball tree is a versatile data structure that can be used for efficient nearest neighbor search. It's particularly useful for high-dimensional data.
To implement a ball tree, you need to define the maximum number of children for each internal node, which is typically set to 10. This value can be adjusted based on the specific requirements of your application.
One key advantage of ball tree over other data structures like KD-trees is its ability to reduce the number of nodes to be traversed during search. This results in faster query times, especially for large datasets.
Difference Between
The KD-Tree and Ball Tree algorithms are two popular spatial indexing methods used for organizing and efficiently querying multidimensional data. KD-Trees are particularly effective for low to high dimensions, while Ball Trees excel in scenarios with high-dimensional data.
The main difference between these algorithms lies in their splitting strategies and tree structures. KD-Trees employ axis-aligned hyperplanes with a binary tree structure, whereas Ball Trees divide data into hyperspheres using a non-binary structure.
KD-Trees are generally faster to construct due to their simpler structure, but Ball Trees offer improved efficiency in nearest neighbor searches for sparse high-dimensional data. Both algorithms exhibit logarithmic query time complexity in average cases, making them efficient for search operations.
Suggestion: Binary Classifier
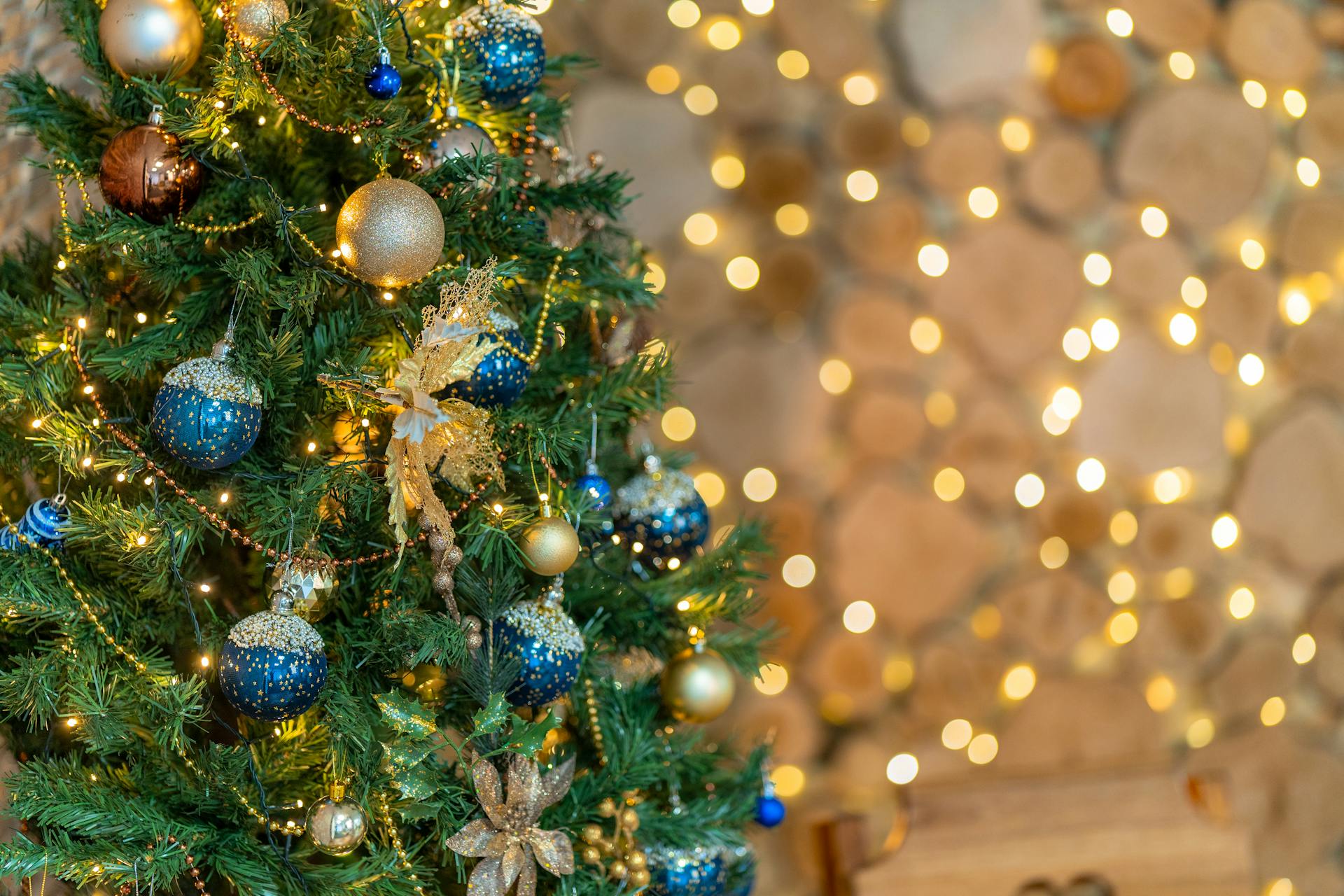
Here's a comparison of the two algorithms in a table:
Python
In the Python implementation of the BallTree algorithm, we can see how it's used to find the closest neighbor to a query point.
The BallTree structure is constructed using the sklearn.neighbors.BallTree class, taking in a dataset as input.
A sample 2D dataset is created using numpy, consisting of four points: [2, 3], [5, 4], [9, 6], and [8, 1].
To perform a BallTree query, we define a query point, which is an array of coordinates: [3, 5].
The k parameter is set to 1, meaning we're looking for the closest neighbor.
The output of the code includes the original dataset, the BallTree structure, the query point, and information about the closest neighbor, including its index, coordinates, and distance from the query point.
For another approach, see: Claude 3 Context Window
Q4: Construction Time Differences
Ball Tree construction is generally slower due to complex geometric computations.
In contrast, KD-Tree construction is significantly faster, thanks to its simpler binary structure.
This speed difference is a crucial consideration for projects with large datasets, where every second counts.
Q5: Memory Usage Differences
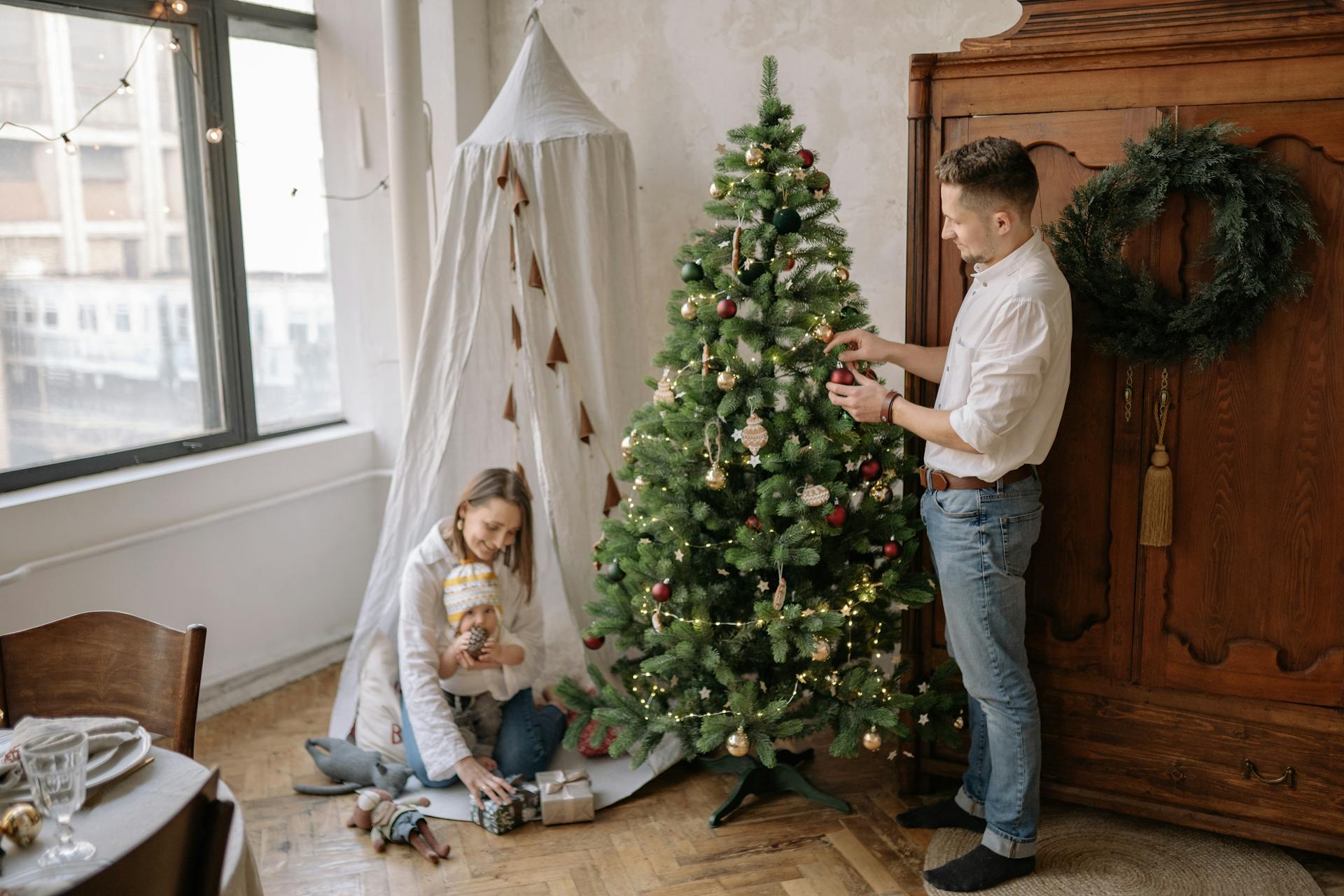
When implementing and comparing algorithms, it's essential to consider their memory usage. Ball Tree typically has higher memory usage compared to KD-Tree, making KD-Tree more memory-efficient.
In general, KD-Tree's memory efficiency is a significant advantage. Ball Tree's increased memory usage can be a drawback in certain applications.
Sources
- https://en.wikipedia.org/wiki/Ball_tree
- https://www.geeksforgeeks.org/ball-tree-and-kd-tree-algorithms/
- https://scikit-learn.org/1.5/modules/generated/sklearn.neighbors.BallTree.html
- https://medium.com/@geethasreemattaparthi/ball-tree-and-kd-tree-algorithms-a03cdc9f0af9
- https://www.activeloop.ai/resources/glossary/ball-tree/
Featured Images: pexels.com