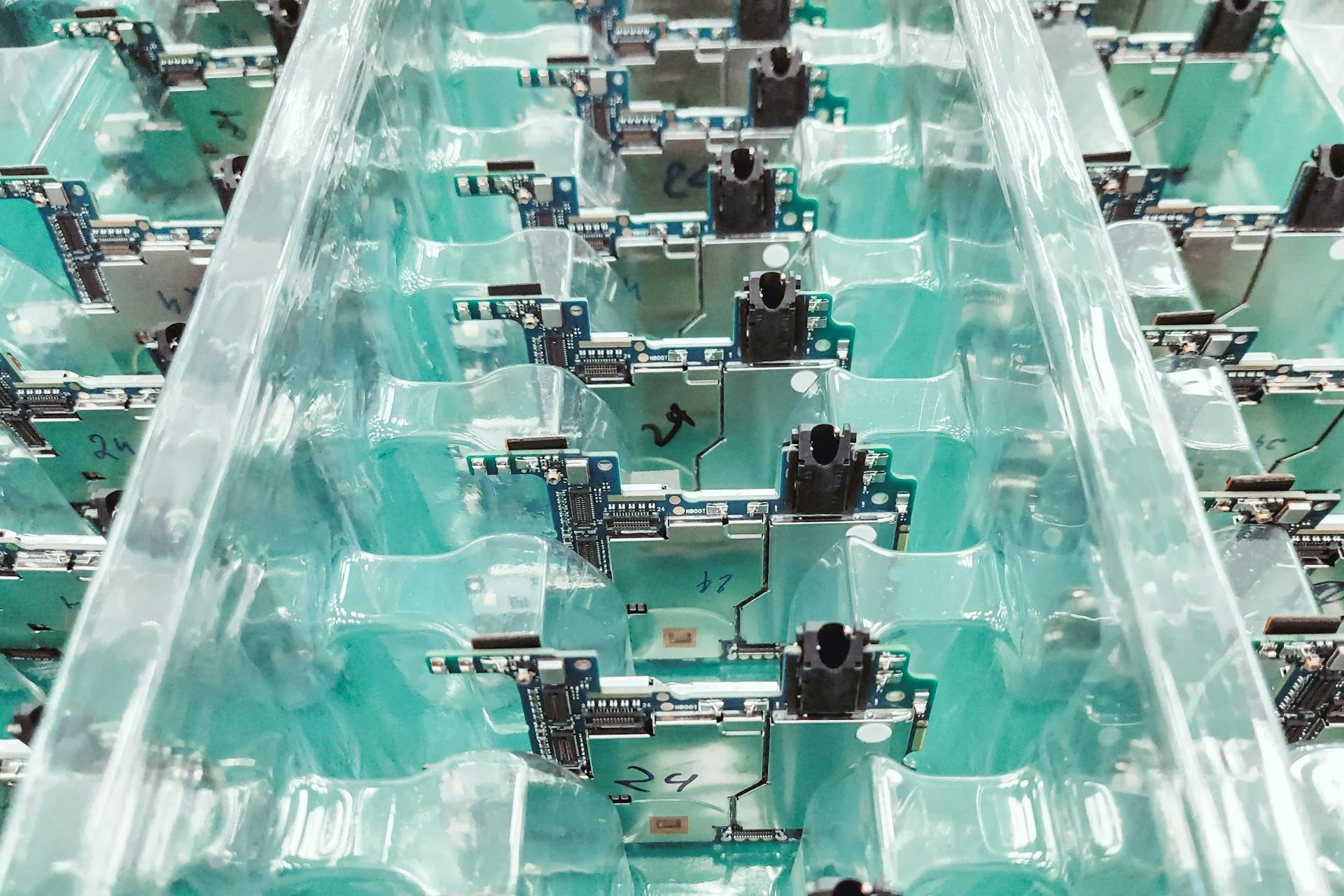
Computer Science 121 is a foundational course that sets the stage for a lifelong journey in tech. It's a course that covers the basics of computer science, including data structures, algorithms, and software engineering.
The course starts with an introduction to programming, where students learn the basics of Python, a popular and versatile language. The course also covers the concept of data structures, including arrays, linked lists, and stacks, which are essential for building efficient algorithms.
As a beginner, it's essential to understand the importance of data structures and algorithms in computer science. By mastering these concepts, you'll be able to write efficient code and solve complex problems with ease. With practice, you'll see the impact of good coding habits on your productivity and problem-solving skills.
Learning Resources
The official textbook for Compsci 121 is "Introduction to Computer Science" by David J. Malan, which covers the basics of programming and computer science concepts.
For online resources, the course website has a comprehensive collection of lecture notes, assignments, and study materials. These resources are updated regularly to match the pace of the course.
To supplement your learning, I recommend checking out the course's GitHub repository, which contains code examples and projects that you can work on to reinforce your understanding of the material.
Lecture Videos
Learning Resources are abundant online, but having a structured approach to learning can make all the difference. To help you stay on track, I've compiled a list of lecture videos covering various topics.
You can access the lecture videos for Expressions, starting from January 25th, with a new video uploaded every other day. By February 3rd, you'll have covered six videos on Expressions.
The lecture videos also cover Variables, with the first video uploaded on February 1st, followed by three more videos uploaded on February 12th, 19th, and 19th.
Functions are another key topic, with three videos uploaded on February 8th, 10th, and no additional videos.
Variables 3 and 4 were uploaded on February 19th, followed by Lists 1 on February 22nd and Statements 1 on February 23rd.
The lecture videos also cover Expressions 8, 9, and 10, all uploaded on March 1st. Mutability 1 and Linked Structures 1 were uploaded on March 3rd and 8th, respectively.
Recursion 1 and 2 were uploaded on March 15th and 17th, followed by Objects 1 on March 19th. Sorting 1, 2, and 3 were uploaded on March 29th and 31st.
Complexity 1, 2, 3, 4, 5, and 6 were uploaded on April 2nd and 5th, respectively. Representation 1 and 2 were uploaded on April 19th, followed by Representation 3 on April 21st.
Here is a list of all the lecture videos mentioned:
- Expressions 1 (Jan 25)
- Expressions 2 (Jan 27)
- Expressions 3 (Jan 27)
- Expressions 4 (Jan 29)
- Expressions 5 (Feb 1)
- Variables 1 (Feb 1)
- Arithmetic 1 (Feb 2)
- Expressions 6 (Feb 3)
- Functions 1 (Feb 8)
- Functions 2 (Feb 8)
- Functions 3 (Feb 10)
- Expressions 7 (Feb 10)
- Variables 2 (Feb 12)
- Variables 3 (Feb 19)
- Variables 4 (Feb 19)
- Lists 1 (Feb 22)
- Statements 1 (Feb 23)
- Expressions 8 (Feb 23)
- Statements 2 (Feb 23)
- Statements 3 (Feb 24)
- Expressions 9 (Mar 1)
- Expressions 10 (Mar 1)
- Expressions 11 (Mar 3)
- Mutability 1 (Mar 3)
- Linked Structures 1 (Mar 8)
- Recursion 1 (Mar 15)
- Recursion 2 (Mar 17)
- Objects 1 (Mar 19)
- Objects 2 (Mar 22)
- Objects 3 (Mar 24)
- Sorting 1 (Mar 29)
- Sorting 2 (Mar 31)
- Sorting 3 (Mar 31)
- Complexity 1 (Apr 2)
- Complexity 2 (Apr 2)
- Complexity 3 (Apr 5)
- Complexity 4 (Apr 5)
- Complexity 5 (Apr 6)
- Complexity 6 (Apr 6)
- Representation 1 (Apr 19)
- Representation 2 (Apr 19)
- Representation 3 (Apr 21)
- Storage 1 (Apr 21)
- Events 1 (Apr 23)
- Events 2 (Apr 23)
- Graphics 1 (Apr 26)
- Graphics 2 (Apr 26)
Lab Materials and Homework
Lab materials and homework are essential components of any course, and it's great to see that they're clearly outlined in the provided article sections.
The first lab material is for Lab 1, but unfortunately, the specific details are not provided. You'll need to check the course resources for more information.
To stay on top of homework assignments, it's recommended to check the due dates and submission platforms regularly. HW1 is due on Friday, Jan 29 at noon, and can be submitted through Gradescope.
If you're using Github Classroom for your homework submissions, HW2 is due on Friday, Feb 5 at noon, and HW3 is due on Friday, Feb 12 at noon. Make sure to check the course resources for the specific assignment details and solution files.
Here's a list of some of the homework assignments with their due dates and submission platforms:
- HW1: Due Friday, Jan 29 at noon, through Gradescope
- HW2: Due Friday, Feb 5 at noon, through Github Classroom
- HW3: Due Friday, Feb 12 at noon, through Github Classroom
- HW4: Due Friday, Feb 19 at noon, through Gradescope and Github Classroom
- HW5: Due Monday, Mar 1 at noon, through Github Classroom
- HW6: Due Friday, Mar 5 at noon, through Github Classroom
- HW7: Due Friday, Mar 12 at noon, through Github Classroom
- HW8: Due Monday, Mar 22 at noon, through Github Classroom
- HW9: Due Monday, Mar 29 at noon, through Github Classroom
- HW10: Due Monday, Apr 5 at noon, through Github Classroom
- HW11: Due Monday, Apr 19 at noon, through Github Classroom
- HW12: Due Monday, Apr 26 at noon, through Github Classroom
- HW13: Due Tuesday, May 11 at noon, through Github Classroom
By keeping track of these due dates and submission platforms, you'll be able to stay on top of your coursework and avoid any last-minute stress.
Class Structure
In Compsci 121, you'll learn about the importance of variable scope, which was covered on February 12. Variable scope determines the visibility and accessibility of variables within a program.
A good understanding of variable scope helps you write more organized and efficient code. This is especially true when working with while statements, which were introduced on February 24. While statements allow you to execute a block of code repeatedly, and knowing how to use them effectively is crucial.
In addition to while statements, you'll also learn about dictionaries and sets, which were covered on March 1. Dictionaries and sets are fundamental data structures that can be used to store and manipulate complex data.
CS 121.5
For students looking for a deeper dive into theoretical computer science, the course offers an "advanced section" known as "CS 121.5". This advanced section explores topics related to the week's lectures, providing students with a chance to delve into more complex ideas.
The topics covered in CS 121.5 are diverse and include proof systems, derandomization, and quantum computing. These advanced topics are not a review of material taught in class, but rather an opportunity for students to explore new and challenging ideas.
Some of the specific topics that will be covered in CS 121.5 include algorithms for learning and online optimization, and error correcting codes. These topics are not only intellectually stimulating but also have practical applications in fields such as data analysis and cryptography.
To give you an idea of what to expect, here are some of the specific topics that will be covered in CS 121.5:
- Proof systems
- Derandomization
- Quantum computing
- Algorithms for learning and online optimization
- Error correcting codes
These topics are just a few examples of the advanced material that will be covered in CS 121.5. Whether you're looking to deepen your understanding of theoretical computer science or simply want to challenge yourself, this advanced section is definitely worth considering.
In-Class Activities
In-Class Activities are a crucial part of the class structure, providing hands-on experience and interaction with the material. The activities are designed to be engaging and thought-provoking, helping students grasp complex concepts.
There are 36 in-class activities planned throughout the semester, covering a wide range of topics from expression trees to rationalism. Each activity is carefully crafted to build upon previous lessons and reinforce key concepts.
Some notable activities include "Expression Trees", "The Fix is In", and "Rationalism", which delve into topics like programming and problem-solving. These activities are designed to be completed in-class, allowing students to work together and receive immediate feedback from the instructor.
A sample of the in-class activities is listed below:
These in-class activities are designed to be interactive and engaging, helping students stay motivated and focused throughout the semester. By participating in these activities, students will gain a deeper understanding of the course material and develop valuable skills that can be applied to real-world scenarios.
Quizzes
Quizzes were an essential part of the class structure, providing students with regular assessments to gauge their understanding of the material.
The first quiz of the semester took place on February 5, and subsequent quizzes were held on February 26, March 12, March 26, and April 9.
These quizzes were accompanied by suggested readings, such as Composing Programs, chapters 1.1 and 1.2, which provided additional context for the material being covered.
Here are the quizzes with their corresponding dates:
- Quiz 1 - Feb 5
- Quiz 2 - Feb 26
- Quiz 3 - Mar 12
- Quiz 4 - Mar 26
- Quiz 5 - Apr 9
Each quiz had its own solution available for students to review and learn from their mistakes.
Assignments and Exams
Assignments are a crucial part of the class structure, making up 40% of the overall grade.
Students can expect to complete 2-3 assignments per week, which can be written, visual, or project-based.
These assignments are designed to test students' understanding of the material and their ability to apply it in real-world scenarios.
The exams, on the other hand, are a more formal assessment of students' knowledge and understanding of the course material.
There are 2 midterms and 1 final exam per semester, each accounting for 20-30% of the overall grade.
The exams are designed to be comprehensive, covering all the material taught in the class since the last exam.
Students are given 2 hours to complete the exams, and they are not allowed to use any external resources or aids.
Discussion Board Etiquette
Posting questions on the discussion board is a great way to get help and learn from others. If you have a question of a general technical nature, make it a public question so others can benefit from the answer.
Not hesitating to ask questions is crucial to learning, as it can help you and others understand the material better. All of us, including staff, sometimes misunderstand things and get confused.
If you're worried about posting a question, you can post it anonymously to the other students, but it's encouraged to post under your name. If you have a pset related question, it's best to post it privately first and let the staff decide whether to make it public later.
Respect and friendliness are key in the discussion board, as in any other interaction in the class. Keep questions technical and avoid posting anonymous complaints, such as "the course is too hard" or "Boaz's good looks are distracting me from learning".
Frequently Asked Questions
Is CS 121 hard?
CS 121 is considered a challenging course with a high level of analytical reasoning required. Be prepared for a demanding learning experience if you decide to take this course.
Is Compsci math heavy?
Yes, computer science is a math-intensive field, requiring a strong foundation in mathematical concepts and problem-solving skills. If you're interested in pursuing a degree in computer science, be prepared to take several math courses as part of your program.
Sources
- Computer Science 121 - UMass CICS (umass.edu)
- Is CS 121 a good fit for you? — CMSC 12100 (uchicago.edu)
- github (github.com)
- github (github.com)
- github (github.com)
- github (github.com)
- (github) (github.com)
- github (github.com)
- github (github.com)
- github (github.com)
- github (github.com)
- github (github.com)
- solution (github.com)
- solution (github.com)
- solution (github.com)
- solution (github.com)
- solution (github.com)
- solution (github.com)
- solution (github.com)
- solution (github.com)
- solution (github.com)
- solution (github.com)
- solution (github.com)
- solution (github.com)
- solution (github.com)
- 1.2 (composingprograms.com)
- 1.4 (composingprograms.com)
- 1.3 (composingprograms.com)
- solution (github.com)
- 1.7 (composingprograms.com)
- CSE 121: Introduction to Computer Programming I (washington.edu)
- introtcs.org (introtcs.org)
Featured Images: pexels.com