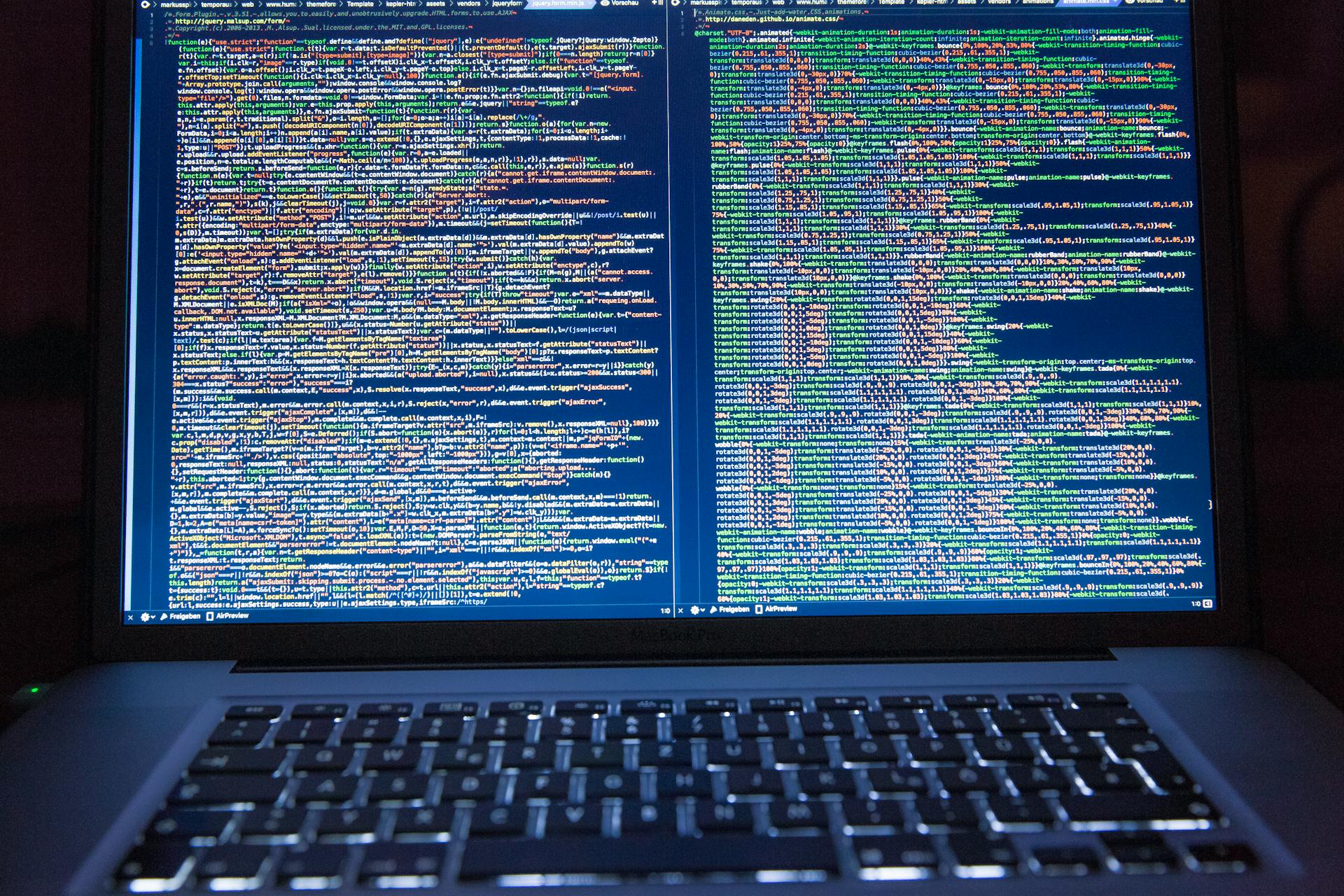
In Compsci 61a, you'll explore the basics of computer science, including algorithms, data structures, and software design.
Algorithms are the heart of computer science, and in this course, you'll learn about different types of algorithms, such as sorting and searching algorithms.
Sorting algorithms, like bubble sort and merge sort, are essential for organizing data in a specific order.
Broaden your view: Grokking Algorithms Second Edition
Course Information
Compsci 61a is a challenging course, but with the right information, you can succeed. The course covers the basics of computer science, including data structures, algorithms, and software design.
The course is designed for students with little to no prior experience in computer science, so don't worry if you're new to the field. You'll learn the fundamental concepts and skills needed to succeed in computer science.
The course meets three times a week, with two lectures and one discussion section.
Overview
The CS 61 series is an introduction to computer science, with a focus on software and machines from a programmer's point of view. This course is designed to teach you about programming, not a specific programming language.
The course is structured into three parts: CS 61A, CS 61B, and CS 61C. Here's a brief overview of what each part covers:
- CS 61A: Abstraction, allowing programmers to think in terms of the problem rather than low-level computer operations.
- CS 61B: Advanced engineering aspects of software, such as constructing and analyzing large programs.
- CS 61C: Machines and how they execute programs.
CS 61A is particularly interested in teaching you about programming techniques, like functional programming and object-oriented programming.
Info 206A
Info 206A is a 2-unit introduction to programming that overlaps with many topics in the first eight weeks of CS 61A. Professor Hany Farid has made all the videos and exercises for this course available online, which can be a great resource to supplement CS 61A.
The course Info 206A is designed to provide a solid introduction to programming and computation.
Suggestion: Cs50x Introduction to Computer Science
Teaching Essentials
Teaching 61A can be a unique opportunity for Berkeley students to gain experience and improve their understanding of the material. Being an undergrad student at Berkeley gives you the chance to teach an official course for thousands of students.
Having a good grasp of the material is essential to teaching 61A successfully. If you're sufficiently motivated and have a solid understanding of the concepts, you have a great shot at trying it out.
Prerequisites
Math prerequisites for CS 61A are flexible, and it's possible to take the course without knowing calculus, although taking calculus can be beneficial in practicing arithmetic and algebra.
Math 1A is listed as a corequisite, meaning it can be taken concurrently, and Math 10A or Math 16A are also acceptable alternatives.
There is no formal programming prerequisite, but prior programming experience is recommended, and most students have had at least one course equivalent to CS 10 or scored a 3 or above on an AP Computer Science exam.
Taking the course without prior programming experience can be challenging, and students may need to spend more time on assignments, potentially receiving lower final grades.
If this caught your attention, see: Comp Sci Math
CS 10
CS 10 is an introductory computer science course that moves at a more moderate pace than CS 61A. It covers topics such as variables, functions, recursion, and object-oriented programming, with an overlap of about 50% with CS 61A.
Expand your knowledge: Cs Theory
The course starts the semester in Snap!, a block-based programming language that allows students to focus on conceptual understanding without worrying about unfamiliar syntax.
CS 10 transitions into Python after the midterm, which is the primary language used in CS 61A. Big ideas and social implications are also covered, showing the beauty and joy of computing.
Data C88C (CS 88)
Data C88C, also known as CS 88, is a 3-unit course that covers approximately 70% of the content of CS 61A.
It has the same instructional team and order of topics as CS 61A, so you can expect a similar experience in both courses.
Data C88C is offered with 2 lectures per week and 2 hours of section per week, compared to 3 lectures and 3 hours of section per week in CS 61A.
The course has fewer total topics and assignments than CS 61A.
For students interested in Data Science, the content in Data C88C is sufficient.
Curious to learn more? Check out: Compsci 61b Data Structures
However, for students majoring or minoring in Computer Science, the additional content in CS 61A is important.
Students who complete Data C88C can proceed directly to CS 61B or take CS 61A subsequently, which offers a substantial amount of review due to the high topic overlap between the courses.
You cannot take Data C88C for credit after having taken CS 61A.
Lectures & Videos
Lectures & Videos can be a great way to learn, but it's essential to know what to expect.
There are three 50-minute live lectures per week, so you'll have plenty of opportunities to ask questions and engage with the material.
Before attending a live lecture, make sure to watch the video playlist for that lecture, as it covers all the required course material.
Live lectures will not cover all course material, but will instead focus on examples, so it's crucial to watch the videos to get a solid understanding of the subject.
Watching the video playlist before attending a live lecture can save you time and help you stay on top of your coursework.
Choosing a Section Format
Choosing a Section Format can be a bit daunting, but it's actually quite straightforward. You have two options: Mega section or regular section.
If you're a student with considerable prior programming experience, Mega section might be the way to go. This format is designed for students who learn well from watching videos, working independently, and coming to drop-in office hours when they need help.
On the other hand, regular sections have clear advantages for students who want to work with others and get to know the course staff. Most students choose regular section, and for good reason.
Here's a brief comparison of the two formats:
Ultimately, the choice between Mega section and regular section depends on your learning style and preferences.
Textbook
The online textbook for the course is Composing Programs, specifically created for this course based on Structure and Interpretation of Computer Programs.
We recommend completing readings before attending lecture to get the most out of the course.
The textbook Composing Programs is a valuable resource for learning course material, and it's great that it's tailored to the specific needs of this course.
Discover more: Best Comp Sci Masters Programs
Conditional Statements
Conditional statements are a fundamental part of programming, and understanding how they work can make a big difference in your coding journey.
You can have any number of elif clauses in a conditional statement, making it easy to check multiple conditions.
The elif clause is optional, but it's a game-changer when you need to check multiple conditions. I've seen many beginners struggle with this, but once you get the hang of it, it's a breeze.
A conditional expression evaluates to either a truthy value or a falsy value. Truthy values are things like True, non-zero integers, and strings with content, while falsy values are things like False, 0, and empty strings.
Here are the possible values a conditional expression can evaluate to:
- Truthy values: True, non-zero integers, strings with content
- Falsy values: False, 0, None, empty strings, empty lists
Only the first if/elif expression that evaluates to a truthy value will have its corresponding indented suite executed. This means you should prioritize your conditions and put the most important ones first.
If none of the conditional expressions evaluate to a true value, then the else suite is executed. Just remember, there can only be one else clause in a conditional statement.
Check this out: Code First Girls
Guesstimate and/or Pseudocode
At this point, you should have a solid understanding of the problem, even if you're not 100% sure how to implement it.
You can usually do one of two things: guesstimate what goes in the blanks based on template code, tip 1c, and multiple choice options, or try to pseudocode your own algorithm.
Guesstimating is a good option when you find the template code distracting, and it can help you fill in the blanks based on previous knowledge and options.
Pseudocoding, on the other hand, allows you to create a high-level description of your algorithm without worrying about the implementation details.
By pseudocoding, you can get a clear idea of the steps involved in solving the problem, and then try to make the template code fit your algorithm.
Broaden your view: Dining Philosophers Problem
Frequently Asked Questions
Is Compsci better than it?
Computer science graduates often earn higher salaries and are in high demand, but an IT degree focuses on maintaining and improving the technology built by computer science professionals. If you want to build the tech, computer science might be the better fit, but if you want to keep it running smoothly, IT is the way to go.
How many units is cs61a?
CS61A is a 4-unit course. This introductory computer science course covers the fundamental concepts of programming.
What is the course title cs61a?
CS61A is a computer science course that focuses on the fundamental principles of programming, emphasizing problem-solving, abstraction, and design. This course, also known as "Structure and Interpretation of Computer Programs," is a comprehensive introduction to programming concepts and techniques.
Featured Images: pexels.com