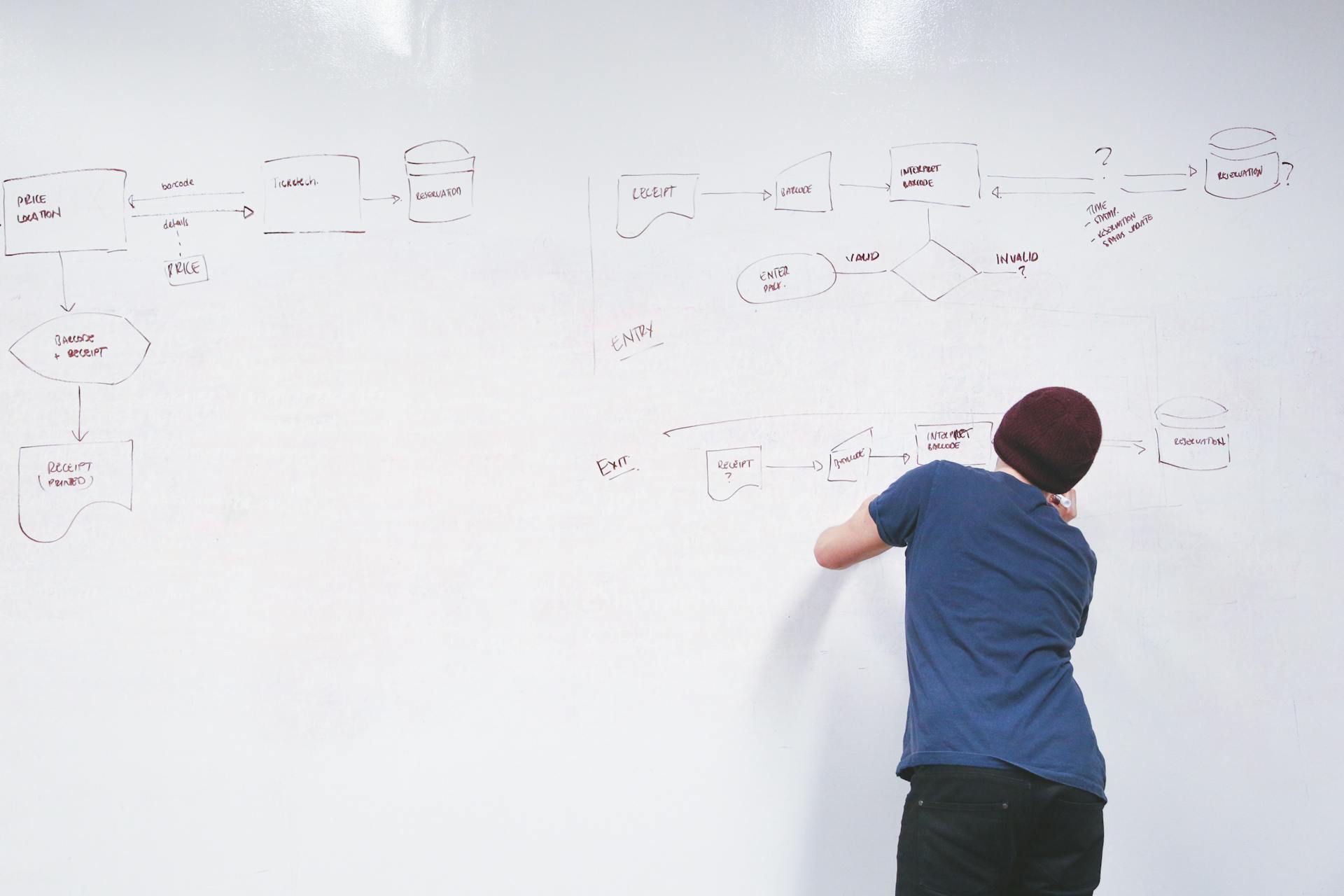
Compsci 188 is a course that delves into the world of search algorithms and problem-solving techniques. It's a crucial subject that helps you develop the skills to tackle complex problems.
In Compsci 188, you'll learn about various search algorithms such as Breadth-First Search (BFS) and Depth-First Search (DFS). These algorithms are used to find the shortest path between two nodes in a graph.
BFS is particularly useful for finding the shortest path, as it explores all the nodes at the current depth level before moving on to the next level. This approach ensures that the algorithm doesn't get stuck in an infinite loop.
One of the key takeaways from Compsci 188 is the importance of problem-solving techniques. By learning how to break down complex problems into smaller, manageable parts, you'll be able to tackle even the most daunting challenges.
Problem-solving techniques, such as divide and conquer, are essential for solving complex problems. By dividing a problem into smaller sub-problems, you can solve each one individually and then combine the solutions to get the final answer.
Recommended read: Grokking Algorithms Second Edition
Grading and Evaluation
Your overall grade in Compsci 188 will be determined by four main components: Programming Assignments (30%), Electronic Assignments (10%), Midterm Exam (25%), and Final Exam (35%).
The grading scale is fixed and based on a percentage system, with clear cutoffs for each letter grade.
Here's a breakdown of the grade scale:
Your grades can also be adjusted upward based on class participation and extra credit.
Introduction and Overview
This course, Compsci 188, is all about designing intelligent computer systems. You'll learn the basic ideas and techniques that underlie this field.
A specific emphasis will be placed on the statistical and decision-theoretic modeling paradigm, which is a powerful approach to building intelligent systems. By the end of the course, you'll have built autonomous agents that can efficiently make decisions in various settings.
Your agents will be able to draw inferences in uncertain environments and optimize actions for arbitrary reward structures.
Lecture: Tu/Th 2:00-3:30 PM, Wheeler 150
Lecture is held on Tuesdays and Thursdays from 2:00-3:30 pm in Wheeler 150.
You can find the lecture schedule, along with the syllabus that includes slides and deadlines, by checking the syllabus itself.
Introduction
This course is a great opportunity to learn about intelligent computer systems. The lectures will be held on Tuesdays and Thursdays from 2:00-3:30 pm in Wheeler 150.
You'll have access to a syllabus that outlines the slides, deadlines, and lecture schedule. This will help you stay organized and on track.
The course focuses on the statistical and decision-theoretic modeling paradigm. This is a key area of study in artificial intelligence.
By the end of the course, you'll have built autonomous agents that can make decisions in various settings. Your agents will be able to draw inferences in uncertain environments and optimize actions for different reward structures.
You'll also learn machine learning algorithms that can classify handwritten digits and photographs. This is a fundamental skill in artificial intelligence.
Discover more: Artificial Intelligence for Social Good
Search Algorithms
Search algorithms are a crucial part of artificial intelligence, and they play a significant role in solving complex problems. A* search, for example, is a popular algorithm that uses a heuristic function to guide the search towards the goal state.
The A* algorithm takes a heuristic function as an argument, which is a function that estimates the distance from a given state to the goal state. The Manhattan distance heuristic, implemented in searchAgents.py, is a good example of a heuristic function that estimates the distance between two points in a grid-based environment.
A* search has been implemented in the empty function aStarSearch in search.py, and it can be tested on the original problem of finding a path through a maze to a fixed position using the Manhattan distance heuristic. The test command is: python pacman.py -l bigMaze -z .5 -p SearchAgent -a fn=astar,heuristic=manhattanHeuristic.
The A* algorithm is faster than uniform cost search, expanding about 549 nodes compared to 620 nodes in uniform cost search. However, the actual numbers may differ slightly due to ties in priority.
Here's a comparison of the search strategies on openMaze:
Suboptimal search strategies, like the ClosestDotSearchAgent, can find a reasonably good path quickly, even if it's not the optimal path. The agent solves the maze in under a second with a path cost of 350.
Breadth First Search
Breadth First Search (BFS) is a graph search algorithm that explores all the nodes at a given depth level before moving on to the next level.
It's implemented in the breadthFirstSearch function in search.py, which uses a Queue as its frontier.
The algorithm works by maintaining a set of visited nodes to avoid expanding already visited states.
The frontier is initialized with the start state of the problem, and then nodes are popped out and their successors are pushed back in.
BFS finds the shallowest node in the search tree first, which means it explores all the nodes at a given depth level before moving on to the next level.
Here's a step-by-step breakdown of the BFS algorithm:
- Initialize a frontier (Queue) and a set of visited nodes
- Push the start state of the problem into the frontier
- While the frontier is not empty:
- Pop a node from the frontier
- If the node is the goal state, return the path
- If the node has not been visited, mark it as visited and push its successors into the frontier
- If the frontier is empty and no goal state has been found, return an empty list
Note that BFS does not necessarily find a least cost solution, but it does find a solution that is guaranteed to be no worse than the solution found by DFS in terms of path length.
Depth First Search
Depth First Search is a linear search algorithm that explores a graph or tree by visiting a node and then exploring as far as possible along each of its edges before backtracking.
This algorithm is particularly useful for traversing large graphs or trees, as it only requires a small amount of extra memory to keep track of the current path.
Depth First Search can be implemented using recursion or iteration, and it's often used in applications such as web crawlers and social network analysis.
One key advantage of Depth First Search is that it can be used to detect cycles in a graph, which is a common problem in many real-world applications.
In a Depth First Search traversal, a node is visited and then its neighbors are visited, but the order in which the neighbors are visited can vary depending on the implementation.
The time complexity of Depth First Search is O(V + E), where V is the number of vertices and E is the number of edges in the graph.
This is because Depth First Search visits each vertex and edge exactly once, making it a very efficient algorithm for traversing graphs.
Expand your knowledge: Code First Girls
A* Search
A* Search is a type of graph search algorithm that uses a heuristic function to guide the search towards the goal. It's implemented in the empty function aStarSearch in search.py.
A* takes a heuristic function as an argument, which is used to estimate the distance from a given state to the goal. The nullHeuristic heuristic function in search.py is a trivial example.
The A* algorithm uses a priority queue to manage the nodes to be visited, where the priority of each node is determined by its combined cost and heuristic value. This is calculated using the formula f(n) = g(n) + h(n), where g(n) is the cost of reaching the node and h(n) is the heuristic value.
A* search can be tested on the original problem of finding a path through a maze to a fixed position using the Manhattan distance heuristic in searchAgents.py. This can be done using the command python pacman.py -l bigMaze -z .5 -p SearchAgent -a fn=astar,heuristic=manhattanHeuristic.
In this case, A* finds the optimal solution slightly faster than uniform cost search, expanding about 549 search nodes compared to 620 for uniform cost search. However, the numbers may differ slightly due to ties in priority.
The A* algorithm is particularly useful for solving problems where the goal is not directly reachable, but there are alternative paths that can be explored. It's a powerful tool for finding the shortest path in a graph or network.
Here's a comparison of the A* algorithm with other search strategies:
Note that the numbers may vary depending on the specific problem and implementation.
Suboptimal Search
In some cases, even with A* and a good heuristic, finding the optimal path through all the dots is hard. The ClosestDotSearchAgent solves this problem by greedily eating the closest dot.
The ClosestDotSearchAgent is implemented in searchAgents.py, but it's missing a key function that finds a path to the closest dot. This function is called findPathToClosestDot.
To solve this problem, we can fill in the AnyFoodSearchProblem, which is missing its goal test. Then, we can solve that problem with an appropriate search function.
The quickest way to complete findPathToClosestDot is to use an A* search function with a heuristic that estimates the distance to the closest dot. This approach allows the agent to find a reasonably good path quickly.
However, the ClosestDotSearchAgent won't always find the shortest possible path through the maze. This is because repeatedly going to the closest dot may not result in finding the shortest path for eating all the dots.
For example, imagine a maze with two dots, one close to the starting point and one far away. If the agent always goes to the closest dot, it may end up taking a longer path to reach the faraway dot.
Here's a simple example of how this can happen:
In this example, the agent starts at the top-left corner and has two dots to eat. If it always goes to the closest dot, it will first eat the top-right dot and then the bottom-right dot. However, a shorter path would be to eat the bottom-right dot first and then the top-right dot.
This highlights the importance of using an optimal search algorithm, like A*, when possible.
Problem-Specific Solutions
In Compsci 188, you'll learn that problem-specific solutions often involve adapting algorithms to fit the unique characteristics of a particular problem. This approach can lead to significant performance improvements.
For example, if you're dealing with a problem that requires frequent updates to a data structure, using a data structure like a hash table can be a game-changer. Hash tables are designed to handle fast lookups and insertions, making them ideal for applications where data is constantly changing.
By understanding the specific requirements of a problem, you can choose the right algorithm and data structures to solve it efficiently. This is a crucial skill to develop in Compsci 188, as it will serve you well in your future programming endeavors.
If this caught your attention, see: Compsci 61b
Finding Corners
Finding corners is a crucial step in problem-solving. Corners are often the key to unlocking a solution.
In the case of the Tower of Hanoi problem, the corner is the final move that transfers the largest disk to the target peg. This move is the culmination of a series of precise steps that require careful planning and execution.
Here's an interesting read: Dining Philosophers Solution
The corner is also a turning point in the sliding puzzle problem, where it's essential to identify the correct orientation of the remaining tiles to reach the solution. This involves rotating and sliding tiles to create a path to the solution.
The corner is a critical element in the Rubik's Cube problem, where it's necessary to solve the corner pieces to unlock the rest of the puzzle. Solving the corner pieces requires a combination of rotation and permutation moves.
By finding the corners, you can gain a deeper understanding of the problem and develop a more effective solution.
Eating Dots
A consistent heuristic is crucial for solving the FoodSearchProblem efficiently. This is evident from the fact that A* with a null heuristic, equivalent to uniform-cost search, quickly finds an optimal solution to the testSearch with no code change.
The FoodSearchProblem requires a new search problem definition, which formalizes the food-clearing problem. This definition is implemented in searchAgents.py.
To find an optimal solution, you should use AStarFoodSearchAgent, which is a shortcut for -p SearchAgent -a fn=astar,prob=FoodSearchProblem,heuristic=foodHeuristic.
Our implementation takes 2.5 seconds to find a path of length 27 after expanding 5057 search nodes.
A non-trivial non-negative consistent heuristic will receive 1 point. Make sure that your heuristic returns 0 at every goal state and never returns a negative value.
Here's a breakdown of the additional points you'll receive based on the number of nodes expanded:
Answer
Using a Stack as a frontier means that the node pushed into it first will be dealt with last. This can be a deliberate design choice to ensure that certain nodes are processed in a specific order.
If you're finding that Pacman is moving too slowly, you can try adjusting the frame time using the option –frameTime 0. This can help speed up the game.
Make sure to complete Question 2 before moving on to Question 5, as Question 5 builds upon your answer for Question 2. This will ensure that you have a solid foundation for tackling the next question.
Frequently Asked Questions
Is CS 188 easy?
CS 188 is considered a fairly easy course, but its relevance to the data industry is limited. If you're interested in AI principles and applications, this course provides a solid introduction.
Is Compsci a math class?
Computer science is not a math class, but it does heavily rely on mathematical concepts to understand computation and develop software systems. While math is a fundamental tool in computer science, it's not the only focus of the field.
Sources
- https://berkeleyai.github.io/cs188-website/
- https://slideplayer.com/slide/15799776/
- https://edge.edx.org/courses/course-v1:Berkeley+CS188+SP17/f5722e0cf4234e4284764db6414b685c/
- https://www.coursehero.com/sitemap/schools/234-University-of-California-Berkeley/courses/486309-COMPSCI188/
- https://www.misaka-9982.com/2022/12/16/CS188-Proj-1/
Featured Images: pexels.com