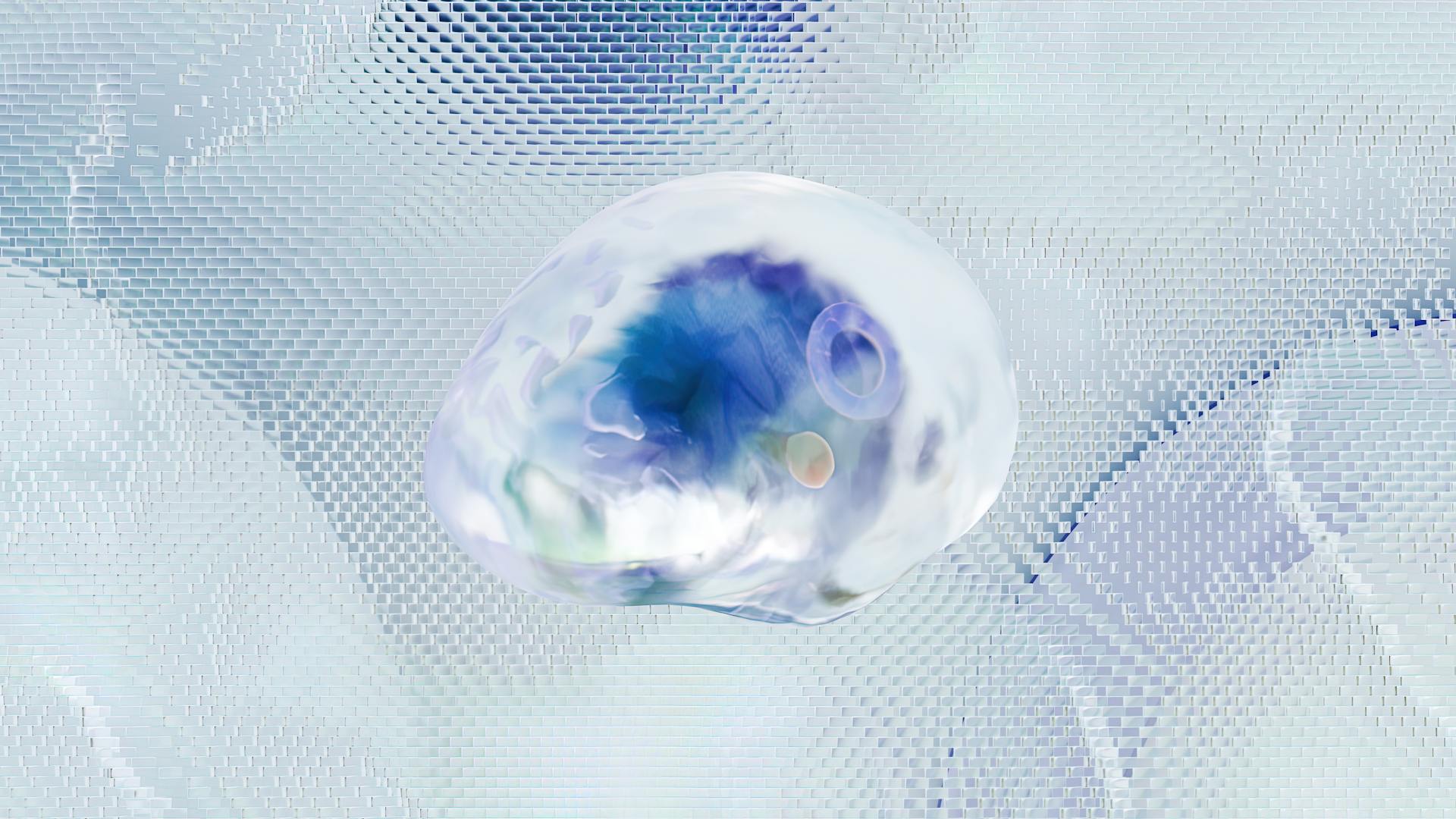
Flux is an open-source machine-learning framework that provides a simple and efficient way to build and train neural networks. It's designed for rapid prototyping and development.
Flux is built on top of the React.js library, which makes it a great choice for developers already familiar with React. This integration allows for seamless integration with existing React applications.
The Flux framework is highly modular, making it easy to swap out components or add new ones as needed. This modularity is a key factor in its flexibility and adaptability.
At its core, Flux is a function that takes in a neural network and a dataset, and outputs the trained model. This simplicity is a major advantage for developers looking to quickly build and test models.
You might like: Hidden Layers in Neural Networks Code Examples Tensorflow
Key Features
Flux has features that set it apart among ML systems. This is evident from its unique design and capabilities.
One notable feature of Flux is that it has features that set it apart from other machine learning systems. This is a key differentiator that makes it stand out in the industry.
Flux is designed to be highly flexible, allowing developers to build and train machine learning models with ease. This flexibility is a major advantage in the field of machine learning.
The features of Flux make it an attractive choice for developers who want to build and deploy machine learning models quickly and efficiently.
Programming Model
Building models in Flux is a matter of composing different functions, much like building a house of blocks. This allows for the creation of more complex models by combining various layers.
To build a model, you can use the Dense utility, which represents a dense layer, or explore other types of layers like convolution, pooling, and recurrent layers detailed in the model reference.
Explore further: Hidden Layers
Differentiable Programming
Differentiable programming is a game-changer in the world of machine learning. Existing Julia libraries are differentiable and can be incorporated directly into Flux models.
Flux has made it incredibly easy to work with differentiable programming by integrating it with libraries like Zygote. Zygote enables overhead-free gradients, making it a powerful tool for building and training neural networks.
Explore further: Ai Ml Libraries in Python
You can use the gradient function to pass in a function and the arguments you want to take the derivative with respect to. This is a simple yet powerful way to work with gradients.
The gradient function can also be used to differentiate parameters that are not explicitly defined as function arguments. This is especially useful when working with large machine learning models that have a lot of parameters.
Flux's autodiff system, powered by Zygote, makes it easy to work with gradients and automatic differentiation. The pullback function is another useful tool that allows you to get the loss and gradient at the same time.
Curious to learn more? Check out: On the Inductive Bias of Gradient Descent in Deep Learning
The Modular Model
The modular model is a great way to build and train models.
In the modular model, each layer is an attribute of a struct type, and the layers are not chained together.
This means that the forward pass behavior needs to be defined explicitly, and an anonymous function is used for this purpose.
Any object of type FFNetwork can re-use this forward pass implementation, making it a reusable and modular approach.
The PyTorch class definition, on the other hand, inherits from the torch.nn.Module base class, which provides built-in functionality such as moving the model onto a GPU.
However, the forward pass definition for each class is built into the class definition, making it less modular and more complex.
Model Building and Training
In Flux, building and training models is a straightforward process. You can compose different functions to create more complex models.
The train! function is a simpler way to implement the training loop, taking an objective function, parameters, data points, and an optimizer as inputs. It can be viewed as taking a step of the parameters with the optimizer, evaluated with respect to a set of data points.
You can use the train! function to train models by specifying a loss function, parameters, data, and the optimizer. The loss function is where the model is contained, and it's used to calculate the loss.
Model Ecosystem
Model Ecosystem is a treasure trove for developers looking to build upon existing models. Model-zoo is a collection of demonstrations of the Flux machine learning library.
You can freely use any of these demonstrations as a starting point for your own models. Metalhead and Flux3D provide trained vision-based and 3D vision-based Flux models.
Transformers provides transformer-based Flux models written entirely in Julia.
You might enjoy: Practical Machine Learning for Computer Vision
Model Definition
Model definition is a crucial step in building a model. In Flux, this is achieved by chaining together a series of Flux layers.
A feed-forward network can be defined using a struct type, where each layer is an attribute. This is demonstrated in the Flux implementation, where a simple flattening layer is chained with two dense layers.
The layers in a model can be defined explicitly, as seen in the modular model definition. This approach allows for more flexibility and control over the model architecture. The forward pass behavior is required to be defined explicitly, and can be reused across different model classes.
In contrast, the PyTorch class definition inherits from the torch.nn.Module base class, which provides built-in functionality such as moving the model onto a GPU. However, this approach can lead to complicated inheritance patterns and increased complexity.
Dataloaders
Before we can start training our models, we need to collate the MNIST data in an easily ingestible way. The Flux.Data module has a special Dataloader type which handles batching, iteration, and shuffling over the data.
The Dataloader type makes generating loaders for the training and test set data pretty straightforward, especially when combined with the onehotbatch function. This function is used to handle batching, iteration, and shuffling over the data.
Notice that the optional typing of function arguments is showcased in this implementation, which is a useful feature for developers.
Training Loop
The training loop is where the magic happens. It's the process of optimizing the model's parameters to minimize the loss function.
In Julia, you can use the Flux.train! loop to optimize the model's parameters. This loop takes in the loss function, optimizer, and training dataloader. The @epochs macro is used to specify the number of times the loop should run.
If this caught your attention, see: Human in the Loop Reinforcement Learning
A similar flow can be seen in the Tensorflow implementation, but it builds the computational graph at the point of calling compile on the model.
The Flux.train! loop is a convenient way to implement the training process. However, Flux also permits custom training loops for more sophisticated metric tracking and loss formulations. This approach requires more work on the software side but offers more flexibility.
In custom training loops, the loss is manually accumulated and the model parameters are updated for each batch in each epoch. The pullback function is used to calculate the loss and obtain the gradients for all trainable parameters.
The PyTorch implementation also requires a custom training loop, where the average loss over the full epoch is accumulated and returned. Gradients are obtained by calling backwards() on the loss object, and the model parameters are updated using the optimizer's step() function.
In Flux, the model is contained in the loss function definition, so it doesn't need to be passed in explicitly. However, in Tensorflow, the model is not present in the loss function, and the computational graph is built at the point of calling compile on the model.
Expand your knowledge: Transfer Learning Tensorflow
Julia Machine Learning
Julia is a great language for machine learning, especially with libraries like Flux that provide lightweight abstractions on top of Julia's native GPU and AD support.
Flux is a 100% pure-Julia stack that makes the easy things easy while remaining fully hackable.
Installing Flux is simple, just use Julia's package manager and type ] in the Julia interpreter.
The Flux library is designed to be fully hackable, so you can easily modify and extend its functionality to suit your needs.
Advanced Topics
In Flux, the `Eager` execution mode is the default, which means that it eagerly executes the computation graph as soon as the `run` function is called. This mode is useful for debugging and testing purposes.
Flux has a built-in support for eager execution, which allows developers to easily switch between eager and lazy execution modes.
The `Eager` execution mode is particularly useful when working with small to medium-sized models, as it provides a more intuitive and predictable execution behavior.
Explore further: Eager Learning
Lazy execution mode, on the other hand, is more suitable for large-scale models, as it can significantly improve performance by deferring computation until the actual values are needed.
Flux's support for eager execution is implemented using a technique called " eager evaluation", which allows the framework to eagerly evaluate the computation graph as soon as the `run` function is called.
Flux's eager execution mode can be disabled by setting the `execution_mode` option to `'lazy'` when creating a `Flux` instance.
Discover more: Lazy Learning
Installation and Setup
To get started with Flux, you'll need to install Julia 1.3 or above. This is the recommended version as of the current writing.
Flux is designed to be used with Julia, so it's essential to have the latest version installed.
You'll be training models in no time once you've got Julia set up.
Check this out: Version Space Learning
Sources
- https://en.wikipedia.org/wiki/Flux_(machine-learning_framework)
- https://github.com/FluxML
- https://alecokas.github.io/julia/flux/2020/07/20/flux-flexible-ml-for-julia.html
- https://www.juliabloggers.com/deep-learning-exploring-high-level-apis-of-knet-jl-and-flux-jl-in-comparison-to-tensorflow-keras/
- https://www.dianacai.com/blog/2020/04/13/machine-learning-julia-flux-autodiff/
Featured Images: pexels.com