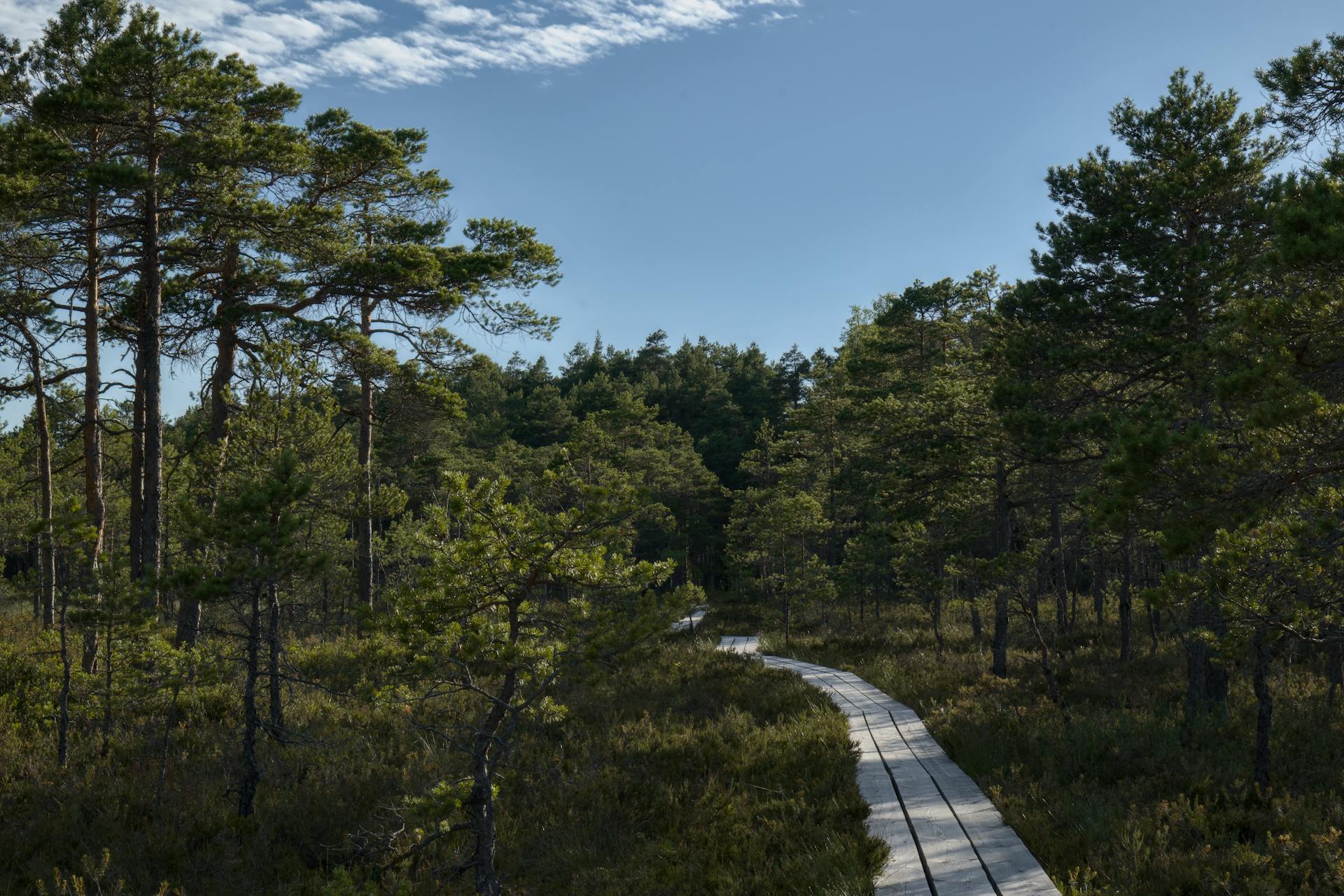
Grid search random forest is a powerful technique for optimizing the hyperparameters of a random forest model.
The grid search method involves creating a grid of possible hyperparameter combinations and evaluating the model's performance for each combination.
With a grid search, you can try different hyperparameters such as the number of trees, maximum depth, and number of features to consider, to see which combination yields the best results.
In practice, grid search can be computationally expensive, especially when dealing with large datasets.
Take a look at this: Random Forest Machine Learning Algorithm
Data Preparation
Data Preparation is a crucial step in any machine learning project. It involves cleaning and transforming the data to prepare it for modeling.
We start by cleaning the missing values in the data and replacing them with the mean. This is done to prevent any bias in the model caused by the missing values.
Before we can train a model, we also need to transform categorical features into numeric values. In the example, the categorical features Embarked and Sex are transformed into numeric values.
Suggestion: Grid Search Examples Python Model Selection
Some columns may be deleted to reduce model complexity, as seen in the example where some columns are deleted to simplify the dataset.
To get a better understanding of the data, we create paired plots for the columns of our data set. This helps us visualize the relationships between pairs of variables in the dataset.
Here are the steps involved in preparing and splitting the data:
- Split the data into input features (X) and the target column (y).
- Using train_test_split, split the data into training and testing sets with a 25:75 percent ratio.
Preprocessing and Exploring the Data
Preprocessing and Exploring the Data is a crucial step in the data preparation process. It involves cleaning and transforming the data to make it suitable for modeling.
Missing values can significantly impact the accuracy of our model, so we need to clean them up. In the example, we replace missing values with the mean, which is a common strategy.
Transforming categorical features into numeric values is also essential. In the example, we transform Embarked and Sex into numeric values, making it easier to work with.
We also need to reduce model complexity by deleting some columns. This is done to prevent overfitting and improve the model's generalizability.
Pair plots are a great way to visualize the relationships between variables in our dataset. By creating pair plots, we can quickly identify correlations and patterns in the data.
Here's a summary of the preprocessing steps:
- Cleaning missing values by replacing them with the mean
- Transforming categorical features into numeric values
- Deleting some columns to reduce model complexity
Prepare and Split Data
Preparing your data is a crucial step in any machine learning project. You need to clean and preprocess the data to ensure it's in a suitable format for modeling.
First, you'll want to remove missing values from your data. According to Example 1, you can replace missing values with the mean, which will help to prevent any skewing of your data.
To transform categorical features into numeric values, you can use one-hot encoding or label encoding. In Example 1, the Embarked and Sex features are transformed into numeric values.
Before training a model, it's essential to understand the relationships between variables in your dataset. You can create paired plots, as mentioned in Example 1, to visualize the relationships between pairs of variables.
To split your data into training and testing sets, you can use the train_test_split function, as shown in Example 3. This will allow you to evaluate the performance of your model on unseen data.
Here's a summary of the key steps involved in preparing and splitting your data:
By following these steps, you'll be able to prepare and split your data effectively, which is essential for building a robust machine learning model.
5. Max Sample
Max Sample plays a crucial role in determining how much of the dataset is given to each individual tree.
In our data preparation process, we have a large set of training datasets, and max_sample is a key factor in deciding how much data to allocate to each tree.
Having a good understanding of max_sample is essential in balancing the complexity and accuracy of our models.
By controlling the amount of data given to each tree, max_sample helps to prevent overfitting and underfitting, ensuring our models are well-balanced and accurate.
Building the Model
The first step in building a grid search random forest model is to train a single random forest model. This model uses a random forest algorithm, which has a large number of hyperparameters.
You can start by training a model with default Random Forest Classifier Hyperparameters. This is done by using the RandomForestClassifier() function, fitting the model to the training data with model.fit(X_train, y_train), and then predicting the mode with model.predict(X_test).
After training the model, you can evaluate its performance using metrics such as the classification report, which can be printed with print(classification_report(y_pred, y_test)).
To improve the model's performance, you can tune its hyperparameters using grid search. This involves defining a parameter grid with various values for the hyperparameters you want to configure. For example, you can define a range of values for n_estimators and max_depth, such as [25, 50, 100, 150] and [3, 6, 9], respectively.
Here is an example of a parameter grid:
The grid search algorithm will test all permutations of this parameter grid to find the optimal combination of hyperparameters.
How It Works
Grid search is an exhaustive technique that tests all permutations of a parameter grid to find the best model configuration.
The grid search algorithm requires us to provide the hyperparameters we want to configure, along with a range of values for each hyperparameter. For instance, we might have a range of [16, 32, and 64] for n_estimators and a range of [8, 16, and 32] for max_depth.
The number of model variants results from the parameter grid and the specified parameters. In the example given, the search grid tests 9 different parameter configurations.
A random forest is a robust predictive algorithm that can handle classification and regression tasks. As a so-called ensemble model, the random forest considers predictions from a group of several independent estimators.
We restrict the hyperparameters optimized by the grid search approach to the following two: n_estimators and max_depth. These two hyperparameters have the most significant influence on model performance.
Here are the key hyperparameters to focus on for grid search:
- n_estimators: determines the number of decision trees in the forest
- max_depth: defines the maximum number of branches in each decision tree
Step 4: Building a Single
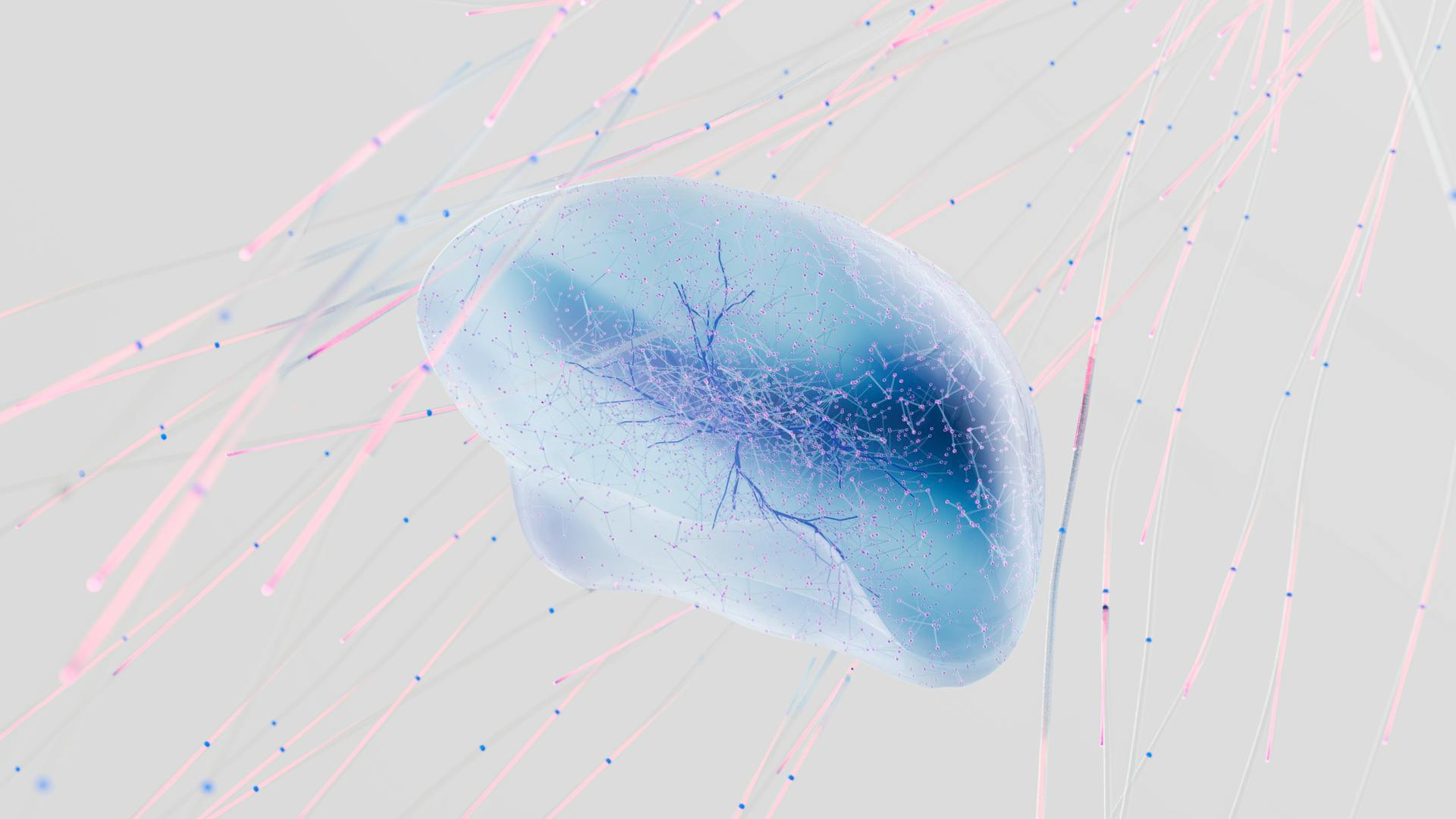
Building a Single Random Forest Model is a crucial step in our model-building process.
We use a random forest algorithm for this model, which has a large number of hyperparameters that need to be adjusted.
The default Random Forest Classifier Hyperparameters are used to train the model.
We fit the model to the training data (X_train, y_train) and make predictions on the test data (X_test).
The model's performance is evaluated using the classification report.
Here are the default hyperparameters used for the Random Forest Model:
Note that hyperparameter tuning doesn't always work, and sometimes the default hyperparameters are the best estimators.
Building
Building a single random forest model is a great place to start. We can train the first model using a random forest algorithm, which has a large number of hyperparameters.
The model uses a random forest algorithm. The random forest algorithm has a large number of hyperparameters. This can make it challenging to tune the model for optimal performance.
You might enjoy: Tune Random Forest Grid Search R
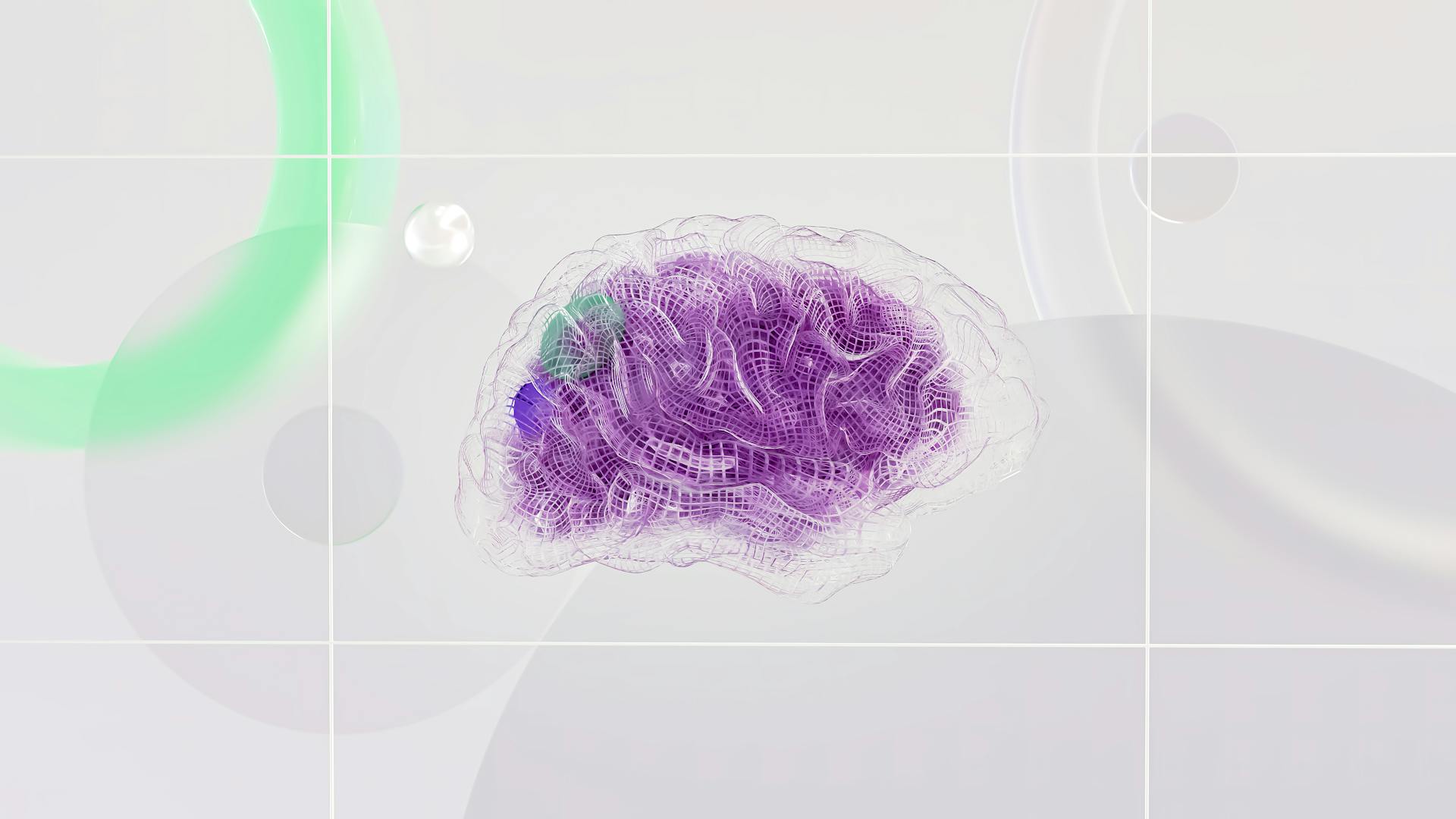
To train the model, we'll use a default Random Forest Classifier with its hyperparameters. The model will be trained on the X_train dataset and evaluated on the y_test dataset.
Here's a code snippet to get us started:
```
model = RandomForestClassifier()
model.fit(X_train, y_train)
y_pred = model.predict(X_test)
metrics.print(classification_report(y_pred, y_test))
```
We can also tune the hyperparameters of the model using grid search. This involves specifying a range of values for each hyperparameter and evaluating the model's performance for each combination.
For example, we might specify the following hyperparameter grid:
```
param_grid = {
'n_estimators': [25, 50, 100, 150],
'max_features': ['sqrt', 'log2', None],
'max_depth': [3, 6, 9],
'max_leaf_nodes': [3, 6, 9],
}
```
Note that hyperparameter tuning doesn't always work, and sometimes the default hyperparameters are the best choice.
If this caught your attention, see: Random Shuffle Dataset Python Huggingface
Frequently Asked Questions
How is grid search different from randomized search?
Grid search tries every possible combination of hyperparameter values exactly once, whereas random search selects combinations randomly from the given domain. This fundamental difference affects the exploration-exploitation trade-off and overall efficiency of the search process.
What does the GridSearchCV() method do?
GridSearchCV() method searches for the best model parameters by cross-validating a grid of possible values, then uses the optimal parameters to make predictions. This technique helps find the most effective model settings for accurate predictions.
What is a grid search?
Grid search is a machine learning algorithm that tries every possible combination of hyperparameter values to find the best model. It's a thorough approach to hyperparameter tuning, but can be computationally expensive.
Sources
- https://www.relataly.com/hyperparameter-tuning-with-grid-search/2261/
- https://scikit-learn.org/1.5/modules/grid_search.html
- https://www.projectpro.io/recipes/tune-hyper-parameters-grid-search-r
- https://www.projectpro.io/recipes/do-optimal-parameters-for-random-forest-r
- https://www.geeksforgeeks.org/random-forest-hyperparameter-tuning-in-python/
Featured Images: pexels.com