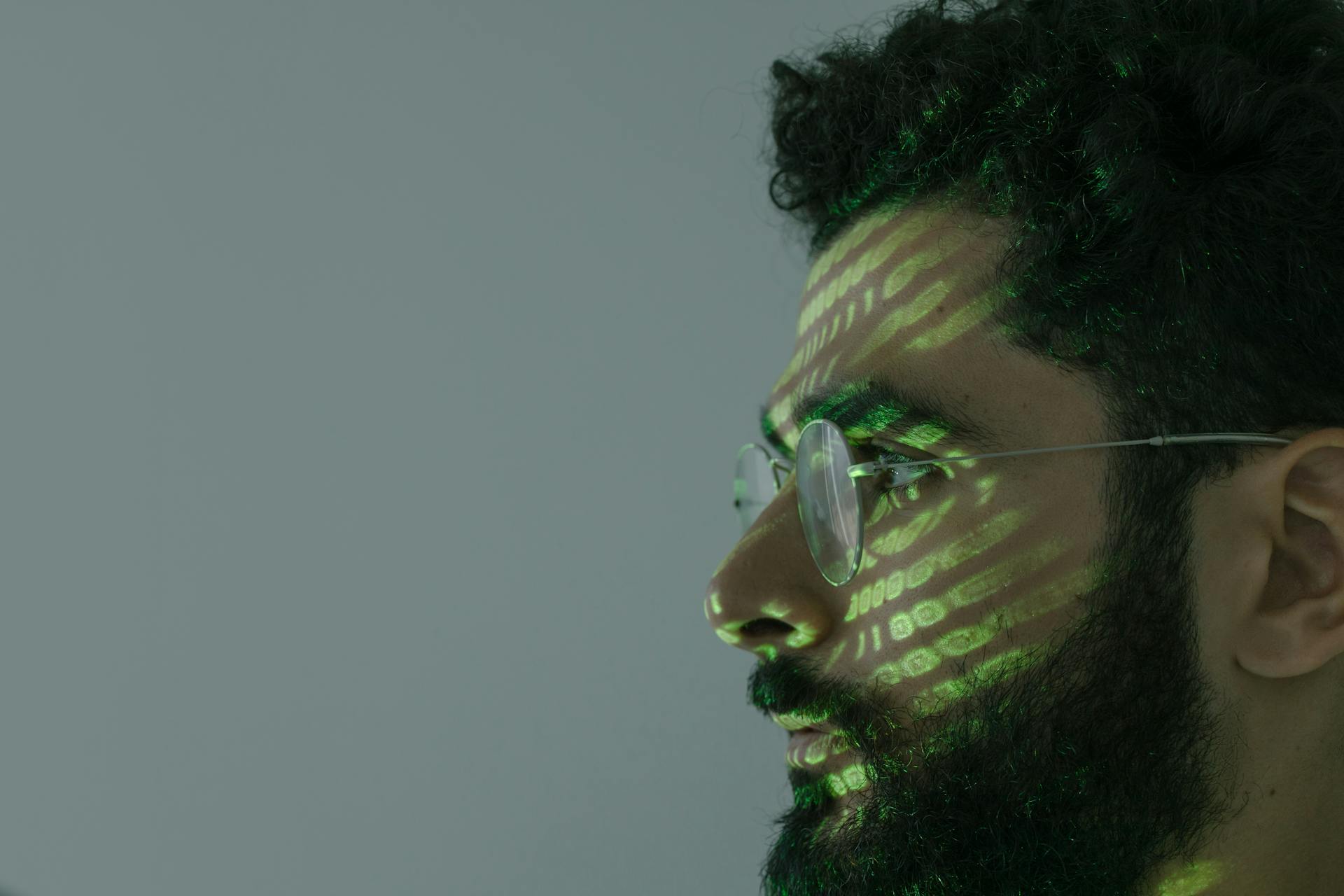
Learning binary code and understanding number systems can seem daunting, but it's actually quite straightforward.
Start by understanding the basics: binary code is a two-digit system that uses only two numbers, 0 and 1.
To get started, you'll need to learn about the binary number system, which consists of 0s and 1s.
Binary code is used in computers to represent information, and it's essential to understand how it works.
In the binary number system, each digit is called a bit, and it can be either 0 or 1.
Expand your knowledge: How to Code Binary Classifier in Python
Binary Fundamentals
Binary is a base two number system that uses only two symbols: 0 and 1. The radix of a binary number system is two, which is half the radix of our decimal system.
The binary system has its own way of counting, and it starts with 0 and 1. You can think of it as a binary version of our decimal counting system.
In binary, the radix determines how many different symbols are required, and in this case, it's just two. This simplicity makes binary a unique and fascinating number system.
You can create any number that a decimal system can using just two symbols. This is a fundamental concept to grasp when learning binary code.
The binary system has its own numeral representations, with 0 and 1 being the only two allowed. This limited set of symbols might seem restrictive, but it's actually quite powerful.
Binary Operations
Learning binary code can be a bit overwhelming at first, but understanding binary operations is a great place to start.
You can manipulate binary values using bitwise operators, which perform functions bit-by-bit on either one or two full binary numbers.
Bitwise operators are widely used in electronics and programming, making them a fundamental concept to grasp.
These operators can be performed in a programming language, allowing you to write code that works with binary values.
The OR bitwise operator is particularly useful for setting one or more bits in a number to be 1, and it's easy to confuse with the OR conditional operator - the double-pipe (||).
Complement (Not)
The complement of a binary value is like finding the exact opposite of everything about it. This is achieved by turning every 1 into a 0 and every 0 becomes a 1.
The complement operator is also called NOT, and it's the only bitwise operator that only operates on a single binary value. The tilde (~) key, which is reached by pressing shift+`, is used to represent the NOT operator in programming.
To find the complement of a binary number, you simply need to flip all the bits. For example, to find the complement of 10110101, you would turn every 1 into a 0 and every 0 into a 1.
The NOT operator is a fundamental concept in binary operations, and understanding how it works is essential for working with binary values.
Or
The OR bitwise operator is a simple but powerful tool in binary operations. It's used to combine two binary values, resulting in a new binary value where each bit is 1 if either of the original bits is 1.
To OR two different binary values, use the pipe, |, operator. For example, 5 | 3 equals 7.
OR'ing a binary value is useful if you need to apply a bit-mask to a value, or check if a specific bit in a binary number is 1, just like the AND operator.
Or Operator
The OR bitwise operator is the pipe | (shift+\, the key below backspace). It's used to set one or more bits in a number to be 1.
OR'ing a binary value is useful if you want to set one or more bits in a number to be 1. This is different from the OR conditional operator, which uses the double-pipe (||) and produces a true or false based on the input of multiple logic statements.
The OR bitwise operator is denoted by the pipe | symbol, which is often confused with the OR conditional operator. Make sure you don't switch them up, as it can lead to incorrect results.
Bitwise Operations
Bitwise operations are a powerful tool in binary code, allowing you to manipulate individual bits of a binary value.
You can perform bitwise operations using boolean logic operating on a group of binary symbols. Bitwise operators are widely used throughout both electronics and programming.
To AND two different binary values, use the ampersand (&) operator. This operation is useful for applying a bit-mask to a value or checking if a specific bit in a binary number is 1.
The four possible XOR combinations and their outcomes are:
- 0 XOR 0 = 0
- 0 XOR 1 = 1
- 1 XOR 0 = 1
- 1 XOR 1 = 0
Shifting left and right is an especially efficient way to multiply or divide by powers of two.
And
The AND operator is a fundamental part of bitwise operations. It's used to perform a binary AND operation between two values.
To AND two different binary values, you use the ampersand (&) operator. This is useful for applying a bit-mask to a value or checking if a specific bit in a binary number is 1.
The AND bitwise operator should not be confused with the AND conditional operation, which uses the double-ampersand (&&) and produces a true or false based on the input of multiple logic statements.
Xor
XOR is a powerful bitwise operator that can be used to check if bits are different. It's denoted by the caret symbol (^) between two values.
The XOR operator will only produce a 1 if either one or the other numbers has a 1 in that bit-position. This makes it useful for checking differences between binary values.
Here are the four possible XOR combinations and their outcomes:
- 0 XOR 0 = 0
- 0 XOR 1 = 1
- 1 XOR 0 = 1
- 1 XOR 1 = 0
For example, to find the result of 10011010 XOR 01000110, notice the 2 bit, which results from two 1's XOR'd together.
Programming and Representation
At their very lowest level, binary is what drives all electronics, making it inevitable to encounter binary in computer programming.
Binary code doesn't just represent ASCII letters; it's used to store all types of information on your computer, including images, audio, and operating systems.
In fact, all of the information on your computer is stored in binary code, making it essential to learn how to interpret other types of data beyond text.
Representing Values
In programming, representing values is a fundamental concept that's essential to understand. Binary is the base of all electronics and computer programming, and it's what drives all electronics.
A binary number can be represented by a 0b preceding the binary number, which is a convention used in programming languages like Arduino. This is a shorthand way of telling the computer that the number is binary, rather than decimal.
In binary, we only have two symbols: 0 and 1. But using these two symbols, we can create any number that a decimal system can. The radix of a binary number is two, which determines how many different symbols are required to represent a number.
To represent a binary number in a program, you can use the 0b notation. For example, 0b1010 is a binary number that represents the decimal value 10.
Here's a table showing some examples of binary numbers and their corresponding decimal values:
In programming, it's essential to understand how to represent values in different formats, including binary. By using the 0b notation, you can easily represent binary numbers in your code.
Operators in Programming
Binary is what drives all electronics, making it inevitable to encounter it in computer programming.
Binary values can be manipulated using bitwise operators, which perform functions bit-by-bit on either one or two full binary numbers.
In programming, bitwise operators make use of boolean logic operating on a group of binary symbols.
These operators are widely used throughout both electronics and programming, making them a fundamental part of any programming language.
You can perform standard mathematical operations like addition, subtraction, multiplication, and division on binary values, but bitwise operators allow for more precise manipulation of individual bits.
File Header Information
File header information is crucial for understanding what's stored in a file and how to interpret the data. It's like the table of contents for a book, giving you a roadmap of what to expect.
Header information tells you what is stored in a file and how the data should be interpreted. This is important for understanding the next step.
Learning how to read file header information is essential for programming and representation. You can't just dive into the data without knowing what it means.
Frequently Asked Questions
What is hello in binary?
Hello in binary is represented as 01001000 01000101 01001100 01001100 01001111. This binary code translates the individual letters of the word 'hello' into a series of 0s and 1s
Is learning binary code hard?
Learning binary code is simpler than you think, and understanding it can be a valuable skill for anyone interested in computer science or programming. With a little practice, you can grasp the basics of binary code and unlock its benefits.
Featured Images: pexels.com