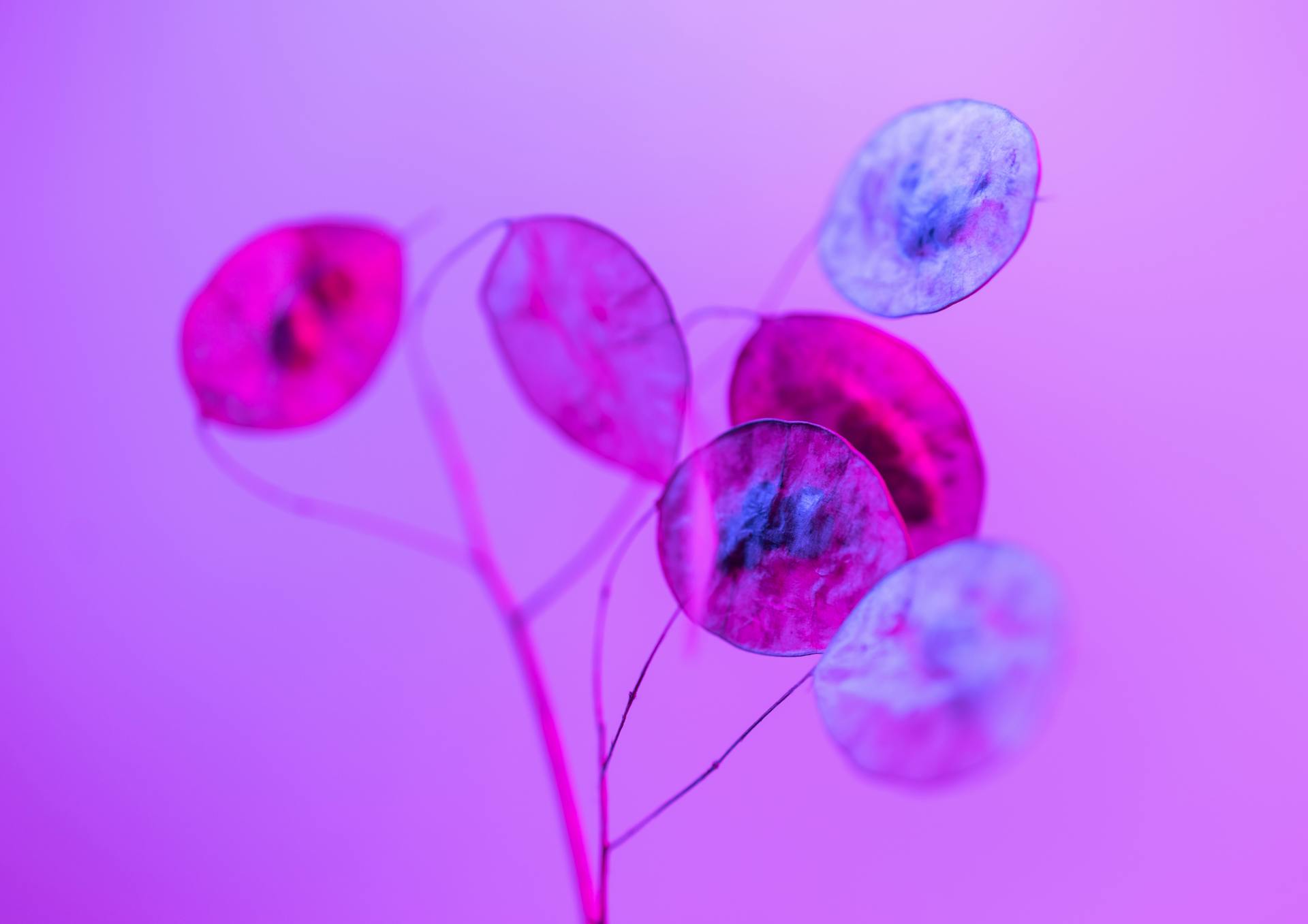
The Huggingface Tokenizer Pad is a crucial component of the Huggingface library, used to handle padding in tokenized text data.
It's a simple but essential tool that helps you work with sequences of different lengths.
The Tokenizer Pad is designed to work seamlessly with Huggingface's tokenizers, allowing you to easily pad your sequences to a consistent length.
This ensures that your models can process sequences of varying lengths without any issues.
The Tokenizer Pad is particularly useful when working with datasets that contain sequences of different lengths, such as text classification or language translation tasks.
On a similar theme: Long Text Summarization Huggingface
Tokenization
Tokenization is the process of converting text into a sequence of tokens, which can be used to represent the meaning and structure of the text. This process is crucial for many natural language processing tasks, including language modeling, text classification, and machine translation.
The Hugging Face tokenizer provides several methods for tokenization, including the `PreTrainedTokenizer` method, which can convert a single index or a sequence of indices into a sequence of tokens.
You might like: Huggingface Interview Process
The `convert_ids_to_tokens` method is used to convert a single index or a sequence of indices into a sequence of tokens, using the vocabulary and added tokens. This method takes two arguments: `ids` and `skip_special_tokens`. The `ids` argument can be an integer or a list of integers, and the `skip_special_tokens` argument is a boolean that defaults to `False`.
The `tokenize` method is used to convert a string into a sequence of tokens, using the tokenizer. This method takes two arguments: `text` and `**kwargs`. The `text` argument is the sequence to be encoded, and the `**kwargs` argument is passed along to the model-specific prepare_for_tokenization preprocessing method.
Here's a summary of the tokenization methods provided by the Hugging Face tokenizer:
Pre-Trained Tokenizer
The Pre-Trained Tokenizer is a powerful tool in the Hugging Face library. It allows you to instantiate a new Tokenizer from an existing file on the Hugging Face Hub.
To do this, you'll need to use the `from_pretrained` method, which requires the identifier of a Model on the Hugging Face Hub that contains a tokenizer.json file. This can be a branch or commit id, and you can also specify an optional auth token to access private repositories.
Here are the parameters you can pass to the `from_pretrained` method:
- identifier (str) - The identifier of a Model on the Hugging Face Hub
- revision (str, defaults to main) - A branch or commit id
- token (str, optional, defaults to None) - An optional auth token used to access private repositories
Once you've instantiated a new Tokenizer, you can use it to tokenize text and perform other tasks. But what if you want to customize the tokenizer or add new tokens to the vocabulary? That's where the `PreTrainedTokenizerFast` class comes in.
The `PreTrainedTokenizerFast` class is a base class for all fast tokenizers, and it handles all the shared methods for tokenization and special tokens. It also includes methods for downloading and caching pretrained tokenizers, as well as adding tokens to the vocabulary. One of the benefits of using `PreTrainedTokenizerFast` is that it provides a unified way to add tokens to the vocabulary, so you don't have to worry about the specific vocabulary augmentation methods of the underlying dictionary structure.
Consider reading: Open Webui Add Tools from Huggingface
Token Processing
Token processing is a crucial step in any NLP pipeline, and the Hugging Face tokenizer makes it a breeze. You can convert IDs to tokens using the `convert_ids_to_tokens` method, which takes in a single index or a sequence of indices and returns the corresponding tokens.
Check this out: Hugging Face Api Token
The `convert_ids_to_tokens` method can also handle lists of IDs, making it a great tool for working with large datasets. By default, it will not remove special tokens in the decoding process, but you can change this behavior by setting the `skip_special_tokens` parameter to `True`.
Here are the methods you can use to convert IDs to tokens:
- `convert_ids_to_tokens`: Converts a single index or a sequence of indices to tokens.
- `skip_special_tokens`: Whether to remove special tokens in the decoding process (default is `False`).
If you're working with raw text, you can use the `tokenize` method to convert it into a sequence of tokens. This method takes in a string and returns a list of tokens, which can then be used for further processing.
Batch Decode
Batch decode is a powerful feature of tokenizers that allows you to convert a list of tokenized input ids into a list of strings. This is particularly useful when working with large datasets or complex models.
To use batch decode, you'll need to pass a list of lists of token ids to the `batch_decode` method. This can be obtained using the `__call__` method of the tokenizer.
You can also specify whether to remove special tokens in the decoding by setting the `skip_special_tokens` parameter to `True`. This can be useful if you want to get a more straightforward output.
When using batch decode, you can also choose to clean up tokenization spaces by setting the `clean_up_tokenization_spaces` parameter to `True`. If you don't specify this parameter, it will default to the value of the `clean_up_tokenization_spaces` attribute of the tokenizer.
Here are the parameters you can pass to the `batch_decode` method:
- `token_ids`: A list of lists of token ids
- `skip_special_tokens`: A boolean indicating whether to remove special tokens in the decoding
- `clean_up_tokenization_spaces`: A boolean indicating whether to clean up tokenization spaces
- `kwargs`: Additional keyword arguments to be passed to the underlying model's decode method
By using batch decode, you can efficiently convert large lists of token ids into human-readable strings.
Batch Encode
Batch encoding is a crucial step in token processing, allowing you to encode multiple inputs at once. This can significantly speed up the processing time, especially when dealing with large datasets.
The `encode_batch` method takes a list of single sequences or pair sequences to encode, which can be either raw text or pre-tokenized. Whether the input is pre-tokenized is determined by the `is_pretokenized` argument, which defaults to False.
You can also specify whether to add special tokens using the `add_special_tokens` argument, which defaults to True. This is useful if you want to include special tokens like [CLS] and [SEP] in your encoded sequences.
The `encode_batch_fast` method is even faster than `encode_batch`, but it doesn't keep track of offsets, so they will all be zeros.
Here are the arguments for the `encode_batch` method:
- input: A list of single sequences or pair sequences to encode
- is_pretokenized: Whether the input is already pre-tokenized (default: False)
- add_special_tokens: Whether to add special tokens (default: True)
- json: A valid JSON string representing a previously serialized Tokenizer
Note that the `json` argument is only used for encoding a previously serialized Tokenizer, which is not typically the case when encoding raw text inputs.
Padding and Truncation
Padding and Truncation are essential strategies when working with tokenizers.
The set_truncation_and_padding function allows you to define these strategies for fast tokenizers provided by the HuggingFace tokenizers library.
You can specify the kind of padding that will be applied to the input, which can be one of the PaddingStrategy types.
Padding strategies determine how the input will be padded to reach the desired length.
The truncation strategy, on the other hand, determines how the input will be truncated when it exceeds the maximum allowed length.
Truncation strategies can be one of the TruncationStrategy types.
The max_length parameter specifies the maximum size of a sequence.
This is crucial when working with long inputs that need to be truncated.
The stride parameter determines the stride to use when handling overflow.
This is particularly important when dealing with large inputs that need to be processed in chunks.
If you want to pad the sequence to a multiple of a specific value, you can use the pad_to_multiple_of parameter.
This is especially useful when working with NVIDIA hardware with compute capability >= 7.5 (Volta).
Here's a summary of the parameters you can use when calling set_truncation_and_padding:
Training and Iteration
You can train a Hugging Face Tokenizer using any Python Iterator, which is a broad term that encompasses various types of sequences.
A list of sequences is a valid input, where each sequence is a list of strings.
To provide meaningful progress tracking, you need to specify the total number of sequences in the iterator.
You can use a generator that yields str or List[str] as an iterator.
A Numpy array of strings is also a valid input.
To train the Tokenizer, you can pass the iterator along with an optional trainer and the total number of sequences in the iterator.
You can use the following types of iterators:
- A list of sequences
- A generator that yields str or List[str]
- A Numpy array of strings
By specifying the total number of sequences, you can get meaningful progress tracking during the training process.
Post Processing
Post Processing is a crucial step in the Hugging Face Tokenizer Pad process. It involves three main steps: truncation, applying the PostProcessor, and padding.
Truncation is the first step, where the output is truncated according to the set truncation parameters provided with enable_truncation(). This ensures that the output is within the specified limits.
The PostProcessor is then applied to the truncated output. The PostProcessor is a critical component that can modify the output in various ways, such as removing special tokens or applying custom rules.
Padding is the final step, where the output is padded according to the set padding parameters provided with enable_padding(). This ensures that the output has a consistent length, which is often necessary for downstream tasks.
Here's a summary of the post-processing steps in a concise format:
- Truncate according to the set truncation params (provided with enable_truncation())
- Apply the PostProcessor
- Pad according to the set padding params (provided with enable_padding())
Class and Transformers
The Hugging Face tokenizer has a class called PreTrainedTokenizerFast, which is a base class for all fast tokenizers.
This class handles shared methods for tokenization and special tokens, making it easier to work with different underlying dictionary structures like BPE and sentencepiece.
PreTrainedTokenizerFast also contains methods for downloading and caching pretrained tokenizers, as well as adding tokens to the vocabulary in a unified way.
The added tokens are returned as a dictionary of token to index, making it easy to access and use the new tokens.
One of the benefits of using PreTrainedTokenizerFast is that it eliminates the need to handle specific vocabulary augmentation methods of the various underlying dictionary structures.
By using this class, developers can focus on the task at hand without getting bogged down in the details of tokenization and vocabulary management.
A fresh viewpoint: Dataset Huggingface Modify Class Label
Sources
- https://huggingface.co/docs/tokenizers/en/api/tokenizer
- https://huggingface.co/docs/transformers/main/en/pad_truncation
- https://huggingface.co/docs/transformers/v4.36.0/main_classes/tokenizer
- https://huggingface.co/docs/transformers/en/pad_truncation
- https://huggingface.co/transformers/v2.9.1/main_classes/tokenizer.html
Featured Images: pexels.com