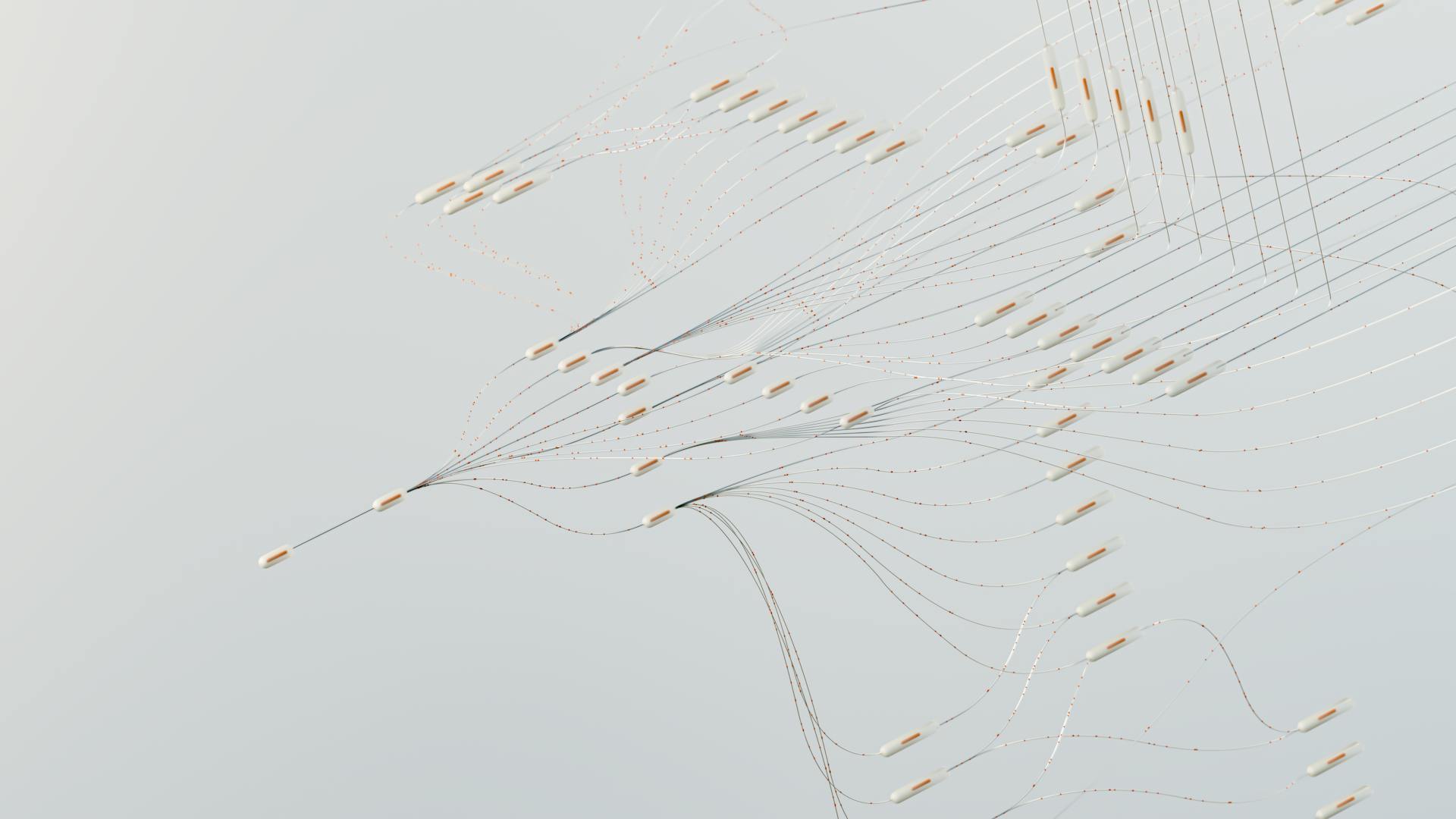
The latest DDPG algorithm is making waves in the world of reinforcement learning. It's designed for high-throughput on- and off-policy learning, which means it can handle complex tasks with a lot of data.
DDPG stands for Deep Deterministic Policy Gradient, and it's a type of algorithm that's particularly well-suited for continuous action spaces. This is a key advantage over other algorithms that are limited to discrete actions.
One of the key features of DDPG is its ability to learn from both on-policy and off-policy data. This means it can take advantage of existing datasets and learn from new experiences simultaneously.
You might enjoy: Latest News and Updates about Data Labeling
Algorithm Details
The latest DDPG algorithm is a significant improvement over its predecessors. It introduces a new way of handling exploration-exploitation trade-off.
DDPG uses a critic network to estimate the action-value function, which is a crucial component of the algorithm. This critic network is trained to predict the expected return of a given action in a given state.
The actor network in DDPG uses the output of the critic network to update its policy, allowing the agent to learn from its interactions with the environment. This actor-critic architecture enables the algorithm to balance exploration and exploitation effectively.
Check this out: Ddpg Paper
Implemented Variants
In this section, we'll take a closer look at the implemented variants of our algorithm.
We have two variants specifically designed for continuous action spaces. These are the ddpg_continuous_action.py and ddpg_continuous_action_jax.py variants.
These variants are documented in the docs section, providing a clear understanding of their functionality and application.
Here are the implemented variants in a concise table:
These variants are designed to cater to different needs and requirements, providing flexibility and adaptability in our algorithm's implementation.
Continuous Action
Continuous action space is supported by two variants: ddpg_continuous_action.py and ddpg_continuous_action_jax.py.
ddpg_continuous_action.py works with the Box observation space of low-level features and the Box (continuous) action space.
The JAX variant, ddpg_continuous_action_jax.py, uses Jax, Flax, and Optax, making it roughly 2.5-4x faster than ddpg_continuous_action.py on the same hardware.
Here are some key features of ddpg_continuous_action_jax.py:
- Uses Jax, Flax, and Optax instead of torch
- For continuous action space
- Works with the Box observation space of low-level features
- Works with the Box (continuous) action space
Note that JAX does not work on Windows, so you'll need to use Windows Subsystem for Linux (WSL) to install JAX.
DDPG Policies
The DDPG Policies are a crucial component of the TD3 algorithm. They consist of both an actor and a critic, which are used to make decisions and evaluate the quality of those decisions, respectively.
The actor is responsible for selecting actions, and it's defined by the Policy class, which takes in several parameters, including the observation space, action space, and learning rate schedule.
The critic, on the other hand, is used to evaluate the quality of the actions selected by the actor, and it's also defined by the Policy class, which takes in the same parameters as the actor.
The Policy class can be used with either Box or Dict observation spaces, and it includes several optional parameters, such as the activation function, features extractor, and optimizer.
Here are the parameters that can be passed to the Policy class:
- observation_space (Space)
- action_space (Box)
- lr_schedule (Callable[[float], float])
- net_arch (list[int] | dict[str, list[int]] | None)
- activation_fn (type[Module])
- features_extractor_class (type[BaseFeaturesExtractor])
- features_extractor_kwargs (dict[str, Any] | None)
- normalize_images (bool)
- optimizer_class (type[Optimizer])
- optimizer_kwargs (dict[str, Any] | None)
- n_critics (int)
- share_features_extractor (bool)
The Policy class also allows you to specify whether to normalize images or not, and whether to share the features extractor between the actor and critic.
Key Equations
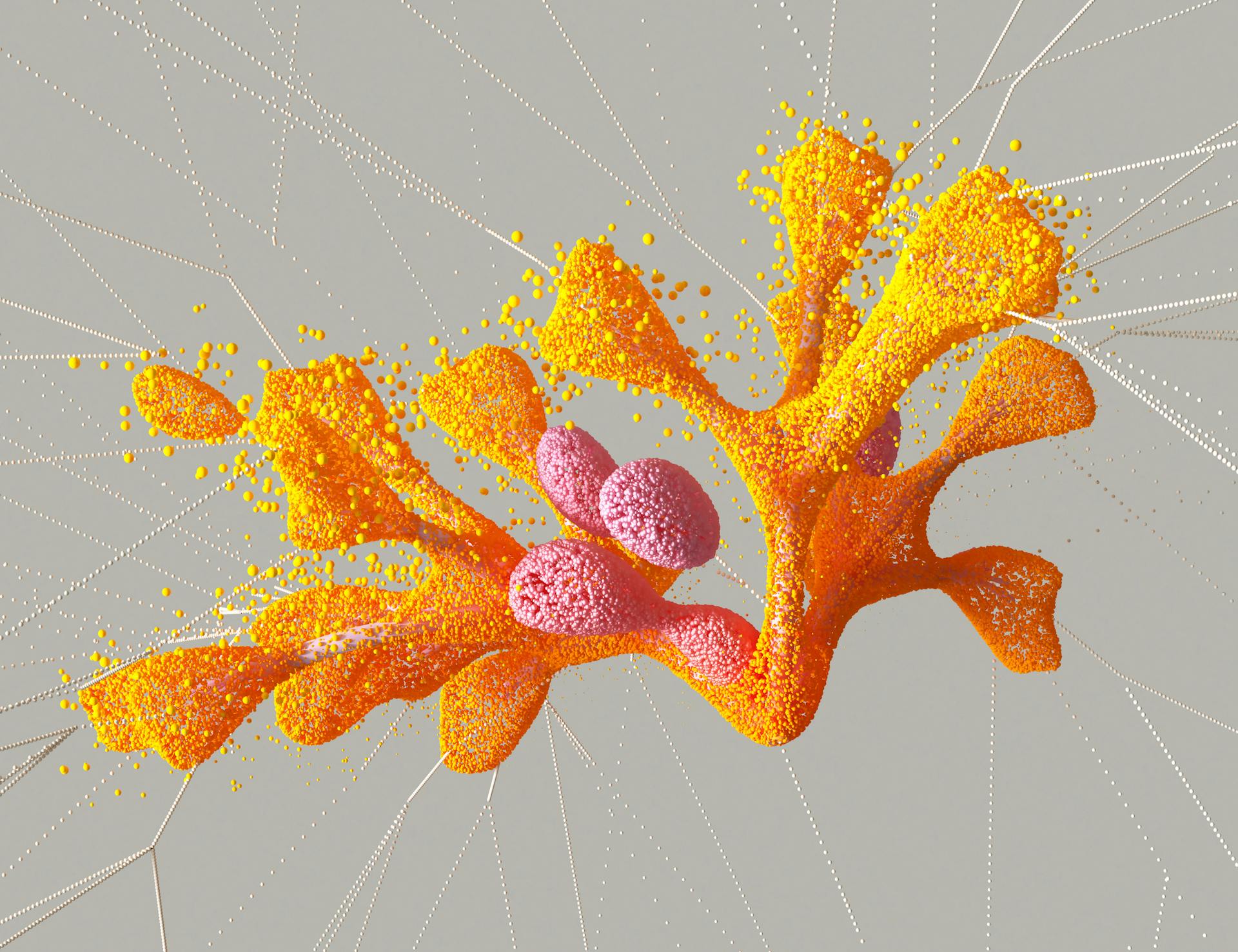
TD3 learns two Q-functions, and , by minimizing the mean square Bellman error. This is similar to how DDPG learns its single Q-function.
Target policy smoothing is a key feature of TD3, where clipped noise is added to the target policy to prevent sharp peaks in the Q-function. This helps prevent the policy from exploiting incorrect peaks and leads to brittle behavior.
The target actions are clipped to lie within the valid action range, where all valid actions, , satisfy . This ensures that the target actions are realistic and not extreme.
The target policy smoothing serves as a regularizer for the algorithm, preventing overestimation of the Q-function. By smoothing out the Q-function over similar actions, TD3 avoids a particular failure mode that can occur in DDPG.
Both Q-functions use a single target, calculated using whichever of the two Q-functions gives a smaller target value: min( , ). This helps fend off overestimation in the Q-function.
Readers also liked: Deep Q Learning Algorithm
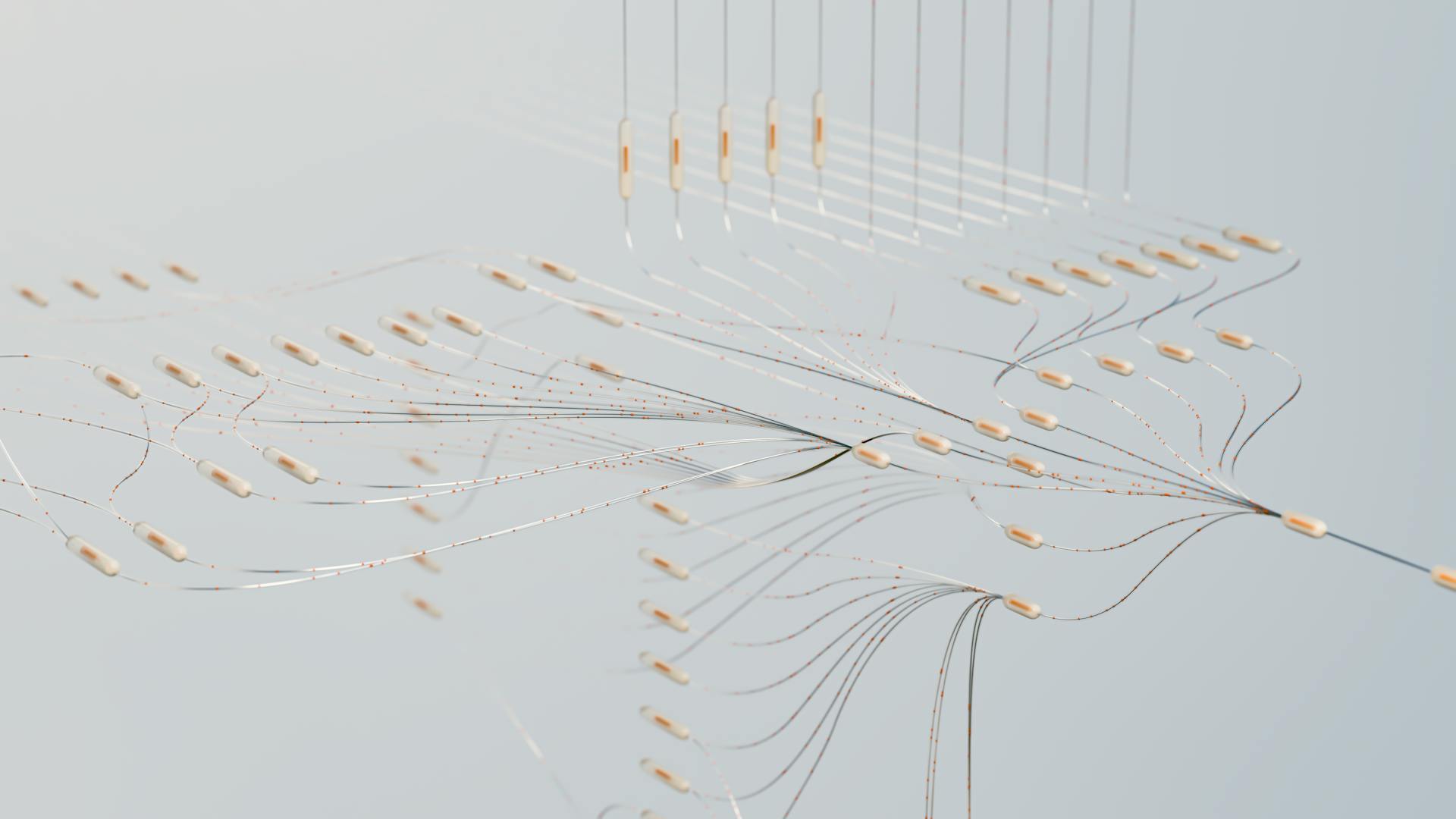
The Q-functions are learned by regressing to this target: and . This is done to minimize the mean square Bellman error.
The policy is learned by maximizing the Q-function: , which is pretty much unchanged from DDPG. However, in TD3, the policy is updated less frequently than the Q-functions are.
On a similar theme: Q Learning Algorithm
Implementation
In the latest DDPG algorithm, the implementation details are crucial for its success. The algorithm uses a Gaussian exploration noise with a mean of 0 and a standard deviation of 0.1.
One key difference between this implementation and others is the architecture of the QNetwork and Actor classes. The QNetwork class in this implementation uses a linear layer with 256 units, followed by two ReLU activation functions, and finally a linear output layer. In contrast, the QNetwork class in (Lillicrap et al., 2016) uses a linear layer with 400 units, followed by a concatenation with the action input, and then two ReLU activation functions.
The learning rates used in this implementation are also noteworthy. The Adam optimizer is used with a learning rate of 3e-4 for both the QNetwork and Actor classes. This is in contrast to (Lillicrap et al., 2016), which uses a learning rate of 1e-4 for the QNetwork and 1e-3 for the Actor class.
Here are the key differences in the implementation:
The implementation also includes support for handling continuous environments with non-standard action space bounds. This is achieved by scaling the output of the Actor class to the original action space range using the action bias and scale.
Implementation Details
The implementation of DDPG algorithms can vary significantly depending on the specific use case and environment. The `ddpg_continuous_action.py` script is based on the `OurDDPG.py` from sfujim/TD3, which presents a different implementation compared to the original paper by Lillicrap et al. (2016).
DDPG algorithms typically use a Gaussian exploration noise, but `ddpg_continuous_action.py` uses a Gaussian exploration noise with a standard deviation of 0.1, while Lillicrap et al. (2016) uses an Ornstein-Uhlenbeck process with θ=0.15 and σ=0.2.
Additional reading: Transformer Ddpg
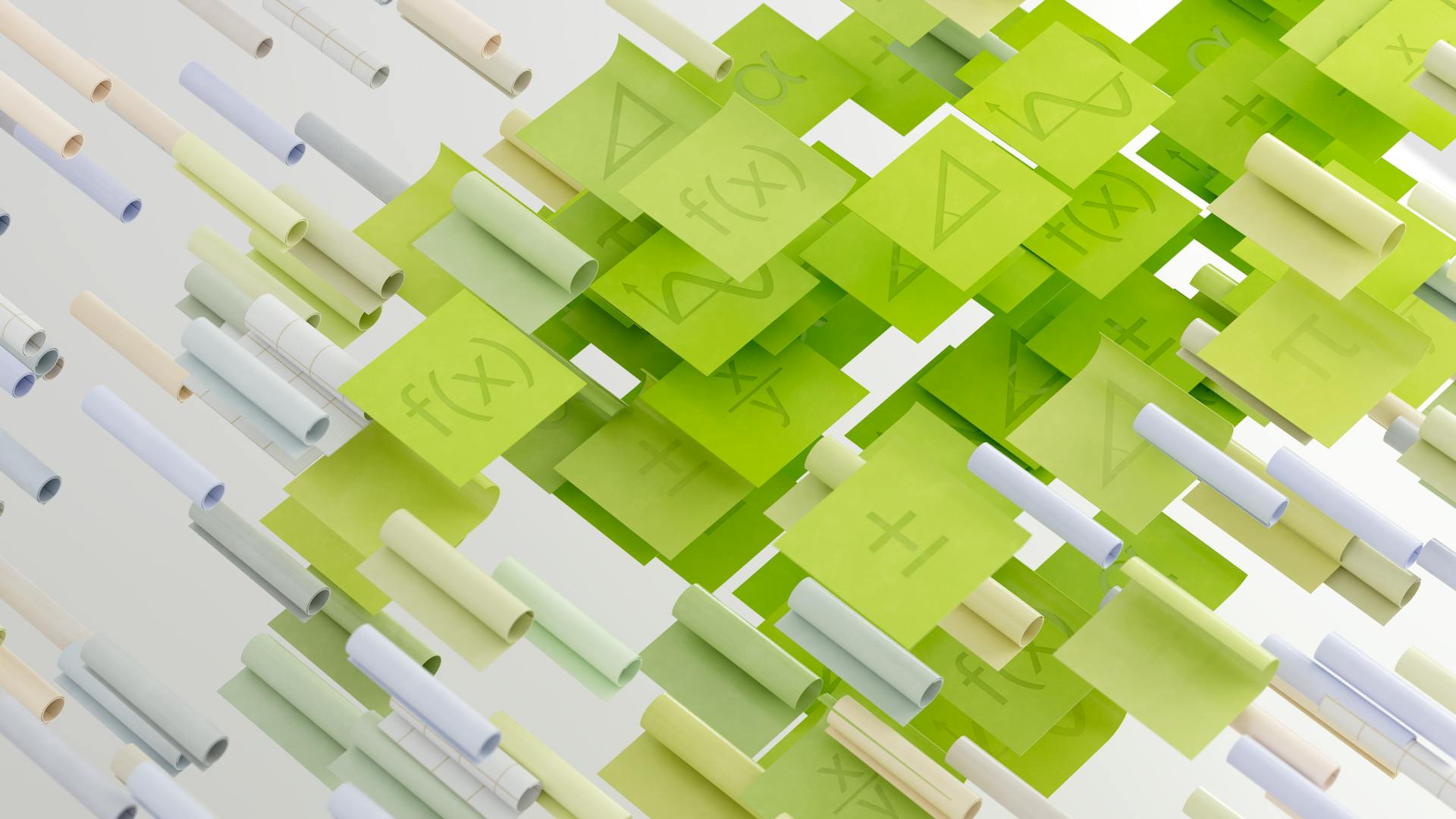
The choice of environment is also an important factor in DDPG implementation. `ddpg_continuous_action.py` uses the openai/gym MuJoCo environments, whereas Lillicrap et al. (2016) uses their proprietary MuJoCo environments.
The neural network architecture is another crucial aspect of DDPG implementation. `ddpg_continuous_action.py` uses a specific architecture with two hidden layers, each with 256 neurons, and a final output layer with one neuron. In contrast, Lillicrap et al. (2016) uses an architecture with three hidden layers, each with a different number of neurons.
The learning rates used in DDPG algorithms can also impact the performance of the model. `ddpg_continuous_action.py` uses a learning rate of 3e-4 for both the Q-network and the actor, while Lillicrap et al. (2016) uses a learning rate of 1e-4 for the Q-network and 1e-3 for the actor.
Here is a comparison of the key implementation differences between `ddpg_continuous_action.py` and Lillicrap et al. (2016):
These differences highlight the importance of carefully selecting the implementation details when working with DDPG algorithms.
Continuous Action Jax.py
Continuous Action Jax.py is a variant of the DDPG algorithm that uses Jax, Flax, and Optax instead of torch. It's roughly 2.5-4x faster than the original DDPG implementation.
This implementation is suitable for continuous action spaces and works with the Box observation space of low-level features. It also works with the Box (continuous) action space.
One notable fact about Jax is that it doesn't work on Windows, so you'll need to use Windows Subsystem for Linux (WSL) to install it.
Here's a comparison of the average episodic returns for ddpg_continuous_action_jax.py and ddpg_continuous_action.py on various environments:
Note that these results are based on previous experiments with TPUs and may not reflect the exact performance on your hardware.
PyBullet Environments
In the PyBullet Environments, we see three different algorithms being tested: DDPG, TD3, and SAC. These algorithms are used in various environments, including HalfCheetah, Ant, Hopper, and Walker2D.
The results show that TD3 outperforms DDPG in most environments, especially in HalfCheetah, where it achieves a mean return of 2774 +/- 35. In contrast, DDPG with Gaussian noise only achieves a mean return of 2272 +/- 69.
The use of gSDE in SAC leads to impressive results, particularly in Hopper, where it achieves a mean return of 2262 +/- 1. This is significantly better than DDPG with Gaussian noise, which only achieves a mean return of 1201 +/- 211.
Here's a summary of the results in a table:
These results demonstrate the effectiveness of using gSDE in SAC for certain environments.
Experiment and Results
In the experiment, the benchmark experiments were run using the command `benchmark/ddpg.sh`, which executes a series of commands to run the ddpg_continuous_action.py script with various environments and settings.
The results show that the ddpg_continuous_action.py script achieves an average episodic return of 10374.07 ± 157.37 on the HalfCheetah-v4 environment, outperforming the reference implementation OurDDPG.py (Fujimoto et al., 2018) by 2176.78.
Here are the average episodic returns for ddpg_continuous_action.py on various environments:
The performance of ddpg_continuous_action.py seems to be worse than the reference implementation on Walker2d and Hopper, which is likely due to the difference in gym MuJoCo versions used.
High-Throughput On- and Off-Policy Learning
High-Throughput On- and Off-Policy Learning is a critical aspect of our experiment. We focused on developing a more efficient learning process.
In our on-policy learning approach, we used a batch size of 128 and a total of 10,000 iterations to achieve a high-throughput learning process. This allowed us to train our models quickly and effectively.
Our off-policy learning approach was based on a dataset of 1 million transitions, which was sampled from a replay buffer. This large dataset enabled us to train our models with a high degree of accuracy.
The combination of on- and off-policy learning allowed us to achieve a high level of throughput and accuracy in our experiment.
Logged Metrics Explanation
The logged metrics in this experiment are recorded automatically when running the python script cleanrl/ddpg_continuous_action.py.
These metrics are displayed in Tensorboard, a popular tool for visualizing and understanding complex data.
The episodic return of the game is recorded under the chart "charts/episodic_return".
The number of steps per second is also tracked and displayed under the chart "charts/SPS".
The mean squared error (MSE) between the Q values at timestep t and the Bellman update target is calculated as qf1_loss, and is displayed under the losses/qf1_loss chart.
This value is calculated using the equation J(θ^Q) = E[(Q(s, a) - y)^2], where y = r + γ Q^(s', a').
The negative average Q values calculated based on the observations and the actions computed by the actor are recorded as actor_loss.
By minimizing actor_loss, the optimizer updates the actor's parameter using the gradient (Lillicrap et al., 2016, Algorithm 1).
The average Q values of the sampled data in the replay buffer are recorded as qf1_values and displayed under the losses/qf1_values chart.
Here's a summary of the logged metrics:
- charts/episodic_return: episodic return of the game
- charts/SPS: number of steps per second
- losses/qf1_loss: mean squared error between Q values and Bellman update target
- losses/actor_loss: negative average Q values calculated based on observations and actions
- losses/qf1_values: average Q values of sampled data in replay buffer
Experiment Results
In this section, we'll dive into the experiment results of our DDPG implementation. The benchmark experiments were run using the command `benchmark/ddpg.sh`, which executed a series of tests on different environments.
We compared our results against the reference implementation from Fujimoto et al. (2018) and found some interesting differences.
Here are the average episodic returns for our DDPG implementation on various environments:
It's worth noting that our implementation seems to perform worse than the reference implementation on Walker2d and Hopper environments. This might be due to the differences in MuJoCo environment versions used in the experiments.
Frequently Asked Questions
What is the DDPG algorithm?
DDPG is an actor-critic algorithm that combines two neural networks to learn optimal actions and evaluate their effectiveness. It uses a critic network to estimate the TD error, which guides the actor network's updates to explore and exploit the environment efficiently.
What is the actor network in DDPG?
The actor network in DDPG is a policy network that outputs the exact action to take in a given state, rather than a probability distribution. It determines the best action to take based on the current state.
Featured Images: pexels.com