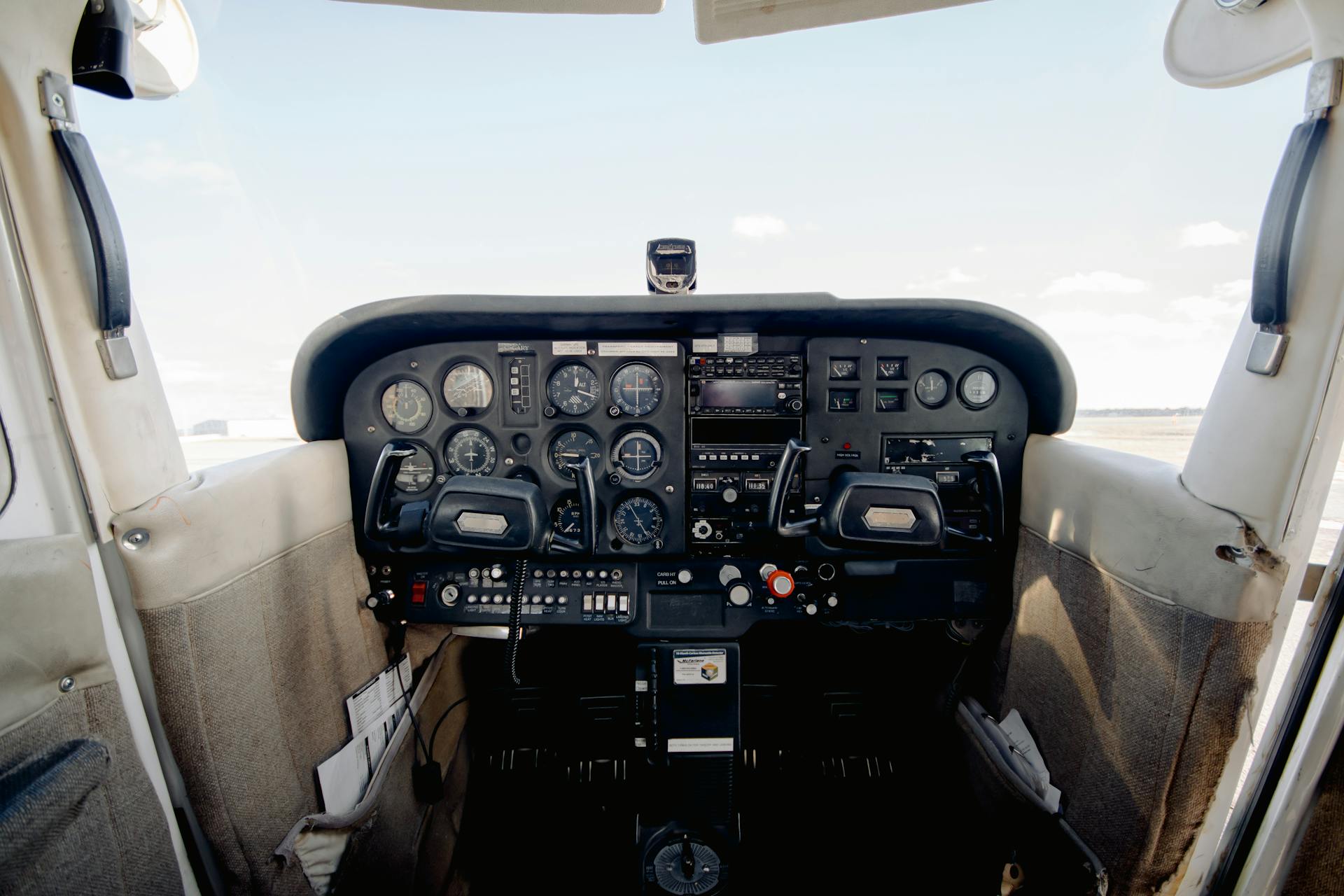
DDPG is a type of reinforcement learning algorithm that's particularly well-suited for continuous control tasks, like robotics or game playing.
One key aspect of DDPG is its use of a critic network to estimate the value function, which helps the agent learn to make better decisions over time.
This approach is especially useful in complex environments where the agent needs to learn from trial and error.
Suggestion: Latest Ddpg Algorithm
Theory
The theory behind DDPG is rooted in deterministic policy gradients, which was first established in Silver 2014. This laid the groundwork for the development of DDPG.
The DDPG algorithm adapts this theory to the deep RL setting, as seen in Lillicrap 2016. This adaptation gave rise to the DDPG algorithm we use today.
Expand your knowledge: Transformer Ddpg
Overview
DDPG is a popular Deep Reinforcement Learning (DRL) algorithm for continuous control. It extends the Deep Q-Network (DQN) algorithm to work with continuous action spaces.
DDPG introduces a deterministic actor that directly outputs continuous actions. This is a key difference from DQN, which uses a discrete action space.
Additional reading: Alpha Beta Pruning Algorithm
The DDPG algorithm combines techniques from DQN, such as the use of a replay buffer and a target network. These techniques help improve the stability and efficiency of the algorithm.
Here are some key papers that have contributed to the development of DDPG:
- Deterministic Policy Gradient Algorithms, Silver et al. 2014
- Continuous Control With Deep Reinforcement Learning, Lillicrap et al. 2016
Key Equations
In DDPG, learning a Q function and learning a policy are two distinct parts, and we'll explore the math behind them.
The Q function is a crucial component of DDPG, and it's learned through a specific equation that updates the function's parameters based on the agent's experiences.
DDPG's policy is also learned through a separate equation that updates the policy's parameters based on the agent's experiences, which is essential for making informed decisions in the environment.
DDPG's learning process involves updating the Q function's parameters using a combination of a critic and an actor, which are two essential components of the algorithm.
The critic's role is to evaluate the Q function's output, and it does so using a specific loss function that calculates the difference between the predicted and actual values.
A unique perspective: Soft Actor Critic
The actor, on the other hand, uses the Q function's output to make decisions in the environment, and it does so by selecting actions that maximize the Q function's output.
DDPG's policy updates are based on the actor's output, which is used to select actions that maximize the Q function's output, resulting in improved performance over time.
The Q function's updates are based on the critic's output, which is used to evaluate the Q function's output and calculate the loss function, leading to improved performance over time.
In practice, DDPG's learning process involves iterating between updating the Q function and the policy, which leads to improved performance over time.
Q-Learning
Q-Learning is a type of reinforcement learning that involves learning from trial and error.
In Q-Learning, an agent learns to predict the expected return or reward for a given state-action pair. This is done by updating the Q-value, which is a measure of the expected return, based on the current Q-value and the reward received for a particular action.
Check this out: Deep Q Learning Algorithm
Q-Learning is a model-free learning method, meaning it doesn't require a model of the environment. Instead, it relies on trial and error to learn the optimal policy.
The Q-value is updated using the Q-Learning update rule, which is Q(s, a) ← Q(s, a) + α[r + γmaxa′Q(s′, a′) - Q(s, a)], where α is the learning rate, r is the reward, γ is the discount factor, and a′ is the next action.
Q-Learning has been used in a variety of applications, including robotics and game playing.
Broaden your view: Llama 3 8b Best Finetune Model
Implementation
To implement DDPG, you'll need to define the actor and critic networks. These networks are typically implemented using neural networks, with the actor network predicting the optimal action and the critic network estimating the value of the current state-action pair.
The actor network is trained to maximize the expected return, which is calculated by the critic network. The critic network is trained to predict the expected return, using the actor's policy to generate actions.
In practice, this means that the actor and critic networks are trained simultaneously, with the actor's policy being updated based on the critic's value estimates. This process is repeated for a specified number of iterations, with the networks being updated at each iteration.
On a similar theme: Hidden Layers in Neural Networks Code Examples Tensorflow
Introduction
DDPG is a state-of-the-art algorithm in the field of reinforcement learning. It's specifically designed for environments with continuous action spaces.
DDPG allows agents to learn optimal policies in tasks that require fine-grained control. This is a huge advantage in many applications.
Deep Deterministic Policy Gradient is particularly useful in situations where precise control is necessary, such as robotics or game development.
Continuous Action Py
Continuous action spaces are a key aspect of many reinforcement learning environments, and implementing them effectively can be a challenge. In the context of DDPG, continuous action spaces are handled through the use of a parametrized deterministic policy.
The actor network in DDPG takes the current observation as input and returns an action as output. This action is a deterministic function of the observation, and is learned by the actor through the minimization of a loss function.
To model the parametrized policy within the actor, a neural network with one input layer and one output layer is used. The output layer returns the action to the environment action channel, and is typically a tanh activation function.
In the case of the ddpg_continuous_action.py implementation, the actor network is defined as follows:
```python
class Actor(nn.Module):
def __init__(self, env):
super(Actor, self).__init__()
self.fc1 = nn.Linear(np.array(env.single_observation_space.shape).prod(), 256)
self.fc2 = nn.Linear(256, 256)
self.fc_mu = nn.Linear(256, np.prod(env.single_action_space.shape))
self.register_buffer("action_scale", torch.tensor((env.action_space.high-env.action_space.low)/2.0, dtype=torch.float32))
self.register_buffer("action_bias", torch.tensor((env.action_space.high+env.action_space.low)/2.0, dtype=torch.float32))
def forward(self, x):
x = F.relu(self.fc1(x))
x = F.relu(self.fc2(x))
x = torch.tanh(self.fc_mu(x))
return x * self.action_scale + self.action_bias
```
This implementation uses a tanh activation function to produce an action that is scaled to the original action space range.
In contrast, the implementation in the original DDPG paper uses a different architecture, with a larger number of layers and a different activation function:
```python
class Actor(nn.Module):
def __init__(self, env):
super(Actor, self).__init__()
self.fc1 = nn.Linear(np.array(env.single_observation_space.shape).prod(), 400)
self.fc2 = nn.Linear(400, 300)
self.fc_mu = nn.Linear(300, np.prod(env.single_action_space.shape))
def forward(self, x):
x = F.relu(self.fc1(x))
x = F.relu(self.fc2(x))
x = torch.tanh(self.fc_mu(x))
return x
```
This implementation uses a larger number of layers and a different activation function, which may affect the performance of the actor network.
In terms of hyperparameters, the ddpg_continuous_action.py implementation uses a batch size of 256 and a learning rate of 3e-4 for the actor optimizer. In contrast, the original DDPG paper uses a batch size of 64 and a learning rate of 1e-3 for the actor optimizer.
Here is a summary of the key differences between the two implementations:
Documentation
Documentation plays a crucial role in any implementation, especially in complex algorithms like DDPG. The PyTorch and Tensorflow implementations of DDPG in Spinning Up have nearly identical function calls and docstrings.
The function calls are so similar that they can be used interchangeably in many cases. However, there are some details relating to model construction that need to be taken into account.
In the documentation for DDPG, you'll find that the focus is on the Deep Deterministic Policy Gradient algorithm. This algorithm is a type of reinforcement learning algorithm that's particularly well-suited for continuous action spaces.
The docstrings in the PyTorch and Tensorflow implementations of DDPG are designed to be comprehensive and easy to understand. They provide a clear explanation of the algorithm and its components.
Worth a look: Pytorch Finetune
PyTorch Model Contents
In PyTorch, you can load a saved model using the `torch.load()` function, which yields an actor-critic object with properties described in the docstring for DDPG-PyTorch.
To get actions from this model, you can simply use the actor-critic object, which is returned by `torch.load()`.
Take a look at this: Fine Tune Embedding Models
Tensorflow Model Contents
The saved Tensorflow model can be accessed in two ways: by running the trained policy with the test_policy.py tool, or by loading the whole saved graph into a program with restore_tf_graph.
To run the trained policy, you can simply use the test_policy.py tool, which is a convenient option for quick testing.
The whole saved graph can be loaded into a program using the restore_tf_graph function, giving you more flexibility in how you work with the model.
This function is a powerful tool for loading and working with the saved model, and it's a great option when you need more control over the model's behavior.
Custom Neural Networks
Custom Neural Networks are a crucial part of creating a DDPG agent. To model the parametrized Q-value function within the critic, use a recurrent neural network with two input layers and one output layer.
A recurrent neural network must have an lstmLayer as one of its network layers. This is necessary to handle sequential data.
On a similar theme: Hidden Layers in Neural Networks
To create a recurrent neural network, use sequenceInputLayer as the input layer. This layer is essential for processing sequences of observations.
The network should be defined as an array of layer objects. This allows you to easily add and connect layers as needed.
To connect the layers, use the addLayers and connectLayers functions. This step is critical in building the neural network architecture.
The number of weights in the network can be displayed using the initialize function. This is a useful step in understanding the complexity of the network.
DDPG agents also require a recurrent network for the actor. This is because the critic has a recurrent network, and the actor must be able to handle sequential data as well.
The actor network can be converted to a dlnetwork object and initialized using the initialize function. This step is necessary to prepare the network for training.
The number of weights in the actor network can also be displayed using the initialize function. This is a useful step in understanding the complexity of the network.
To use a DDPG agent with recurrent neural networks, you must specify a SequenceLength greater than 1. This is a critical step in setting up the agent for training.
Readers also liked: Gpu for Ai Training
Model Checkpoint
Saving model weights on every improvement over the current best score gives you the flexibility to pause training and continue later by loading the saved model.
This approach also allows you to use the saved model as a starting point in some trials, rather than training everything from scratch every time.
You can save model weights on every improvement over the current best score to take advantage of this flexibility.
Worth a look: Distributed Training Huggingface
Multi Environment
In a multi agent environment, twenty agents are presented with a challenge that requires solving over 100 episodes. The environment is considered solved when the average score of all twenty agents is +30 or above.
The multi agent reacher environment is a specific example of this type of setup. It took 28 hours of training to solve this environment, not including failed trials.
The use of twenty actor-critic networks with a shared replay buffer was key to solving this environment. This allowed the experience tuple experienced by each agent to be useful to others.
Intriguing read: Multi Head Attention Math
The network architecture and hyperparameters largely remain the same as in the single agent environment. However, it took a lot more trials to get the agent to solve the environment due to slow learning.
Improvement from 22.36 to 22.37 took 20 minutes, which led to a "stop the training, tune hyperparameters and try again" loop. Model checkpointing was a lifesaver in this situation, allowing me to start training from where the last training process was stopped.
The trained agents in action can be seen in the following video.
Frequently Asked Questions
Is DDPG on or off policy?
DDPG is an off-policy algorithm, meaning it can learn from past experiences without directly interacting with the environment. This allows for more efficient learning in complex environments with continuous action spaces.
Is TD3 better than DDPG?
TD3 improves upon DDPG by addressing its limitations, resulting in better performance in continuous control tasks. This is achieved through key additions such as twin Critic networks and delayed policy updates.
What is the objective of DDPG?
DDPG aims to train a policy that outputs actions maximizing the value predicted by a value network, enabling effective control tasks. This strategy focuses on maximizing value to achieve optimal results.
What is the Ddpg agent in keras?
The DDPG agent in Keras is an off-policy algorithm that learns a policy (actor) and a Q-function (critic) for continuous action spaces, similar to DQN but for continuous actions. It's a powerful tool for training agents to make decisions in complex, real-world scenarios.
Sources
- https://spinningup.openai.com/en/latest/algorithms/ddpg.html
- https://docs.cleanrl.dev/rl-algorithms/ddpg/
- https://www.katnoria.com/ddpg-reacher/
- https://medium.com/@shruti.dhumne/deep-deterministic-policy-gradient-ddpg-dfb1f9c19115
- https://www.mathworks.com/help/reinforcement-learning/ref/rl.agent.rlddpgagent.html
Featured Images: pexels.com