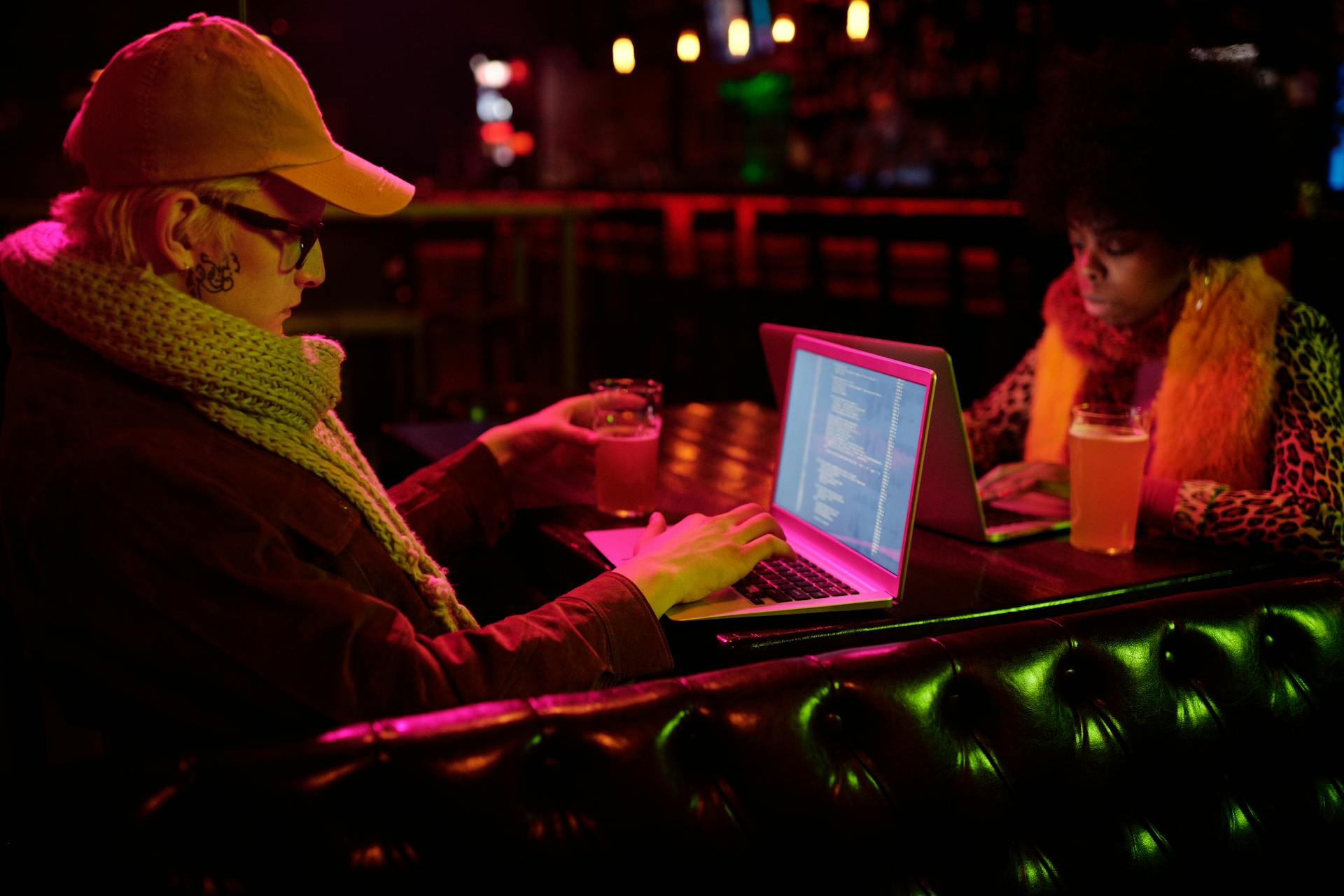
Learning to code can be a daunting task, but it's a valuable skill to have in today's digital age.
The "Learn Code the Hard Way" approach emphasizes hands-on practice over theoretical knowledge. This method involves writing code for real-world projects and debugging your own mistakes.
You'll be writing code by the end of the first chapter, with a focus on building a simple calculator. This project helps you understand the basics of variables, functions, and control structures.
With each project, you'll be building on your existing knowledge and developing problem-solving skills.
Codecademy vs. Other Platforms
Codecademy is a popular online learning platform, but how does it stack up against other options? Codecademy has a massive library of courses, with over 48 million registered users and 25 million courses completed.
One notable difference is that Codecademy focuses on interactive coding exercises, which can be a major advantage for learners who prefer hands-on experience. In contrast, some platforms, like Coursera, focus more on video lectures and reading assignments.
Coursera, for example, offers a wide range of courses from top universities, but they often require a significant time commitment and may not be as engaging for some learners. Codecademy's interactive approach can be more effective for building practical coding skills.
Another option is FreeCodeCamp, which offers a comprehensive curriculum in web development and has a strong focus on community support. However, FreeCodeCamp's curriculum is more comprehensive and may be overwhelming for beginners.
Ultimately, the choice between Codecademy and other platforms depends on your individual learning style and goals.
Variables and Data Types
Variables and Data Types are the building blocks of any programming language. You can call a variable with a specific name.
In programming, variables can be of different types, such as strings, numbers, and more. The way you call a variable depends on its type. For strings, you must use the %s format, while for numbers, you can use %r or %d.
Here's a quick reference to help you keep track of the different variable types and their corresponding formats:
By understanding the different variable types and their formats, you'll be able to write more efficient and effective code.
Variables and Printing
Variables are a crucial part of any programming language, and understanding how to use them is essential for any programmer.
You can call a variable with a specific syntax, depending on its type. If the variable is a string (text), you'll use %s to call it.
When calling a variable, you should make the choice depending on the variable type. This ensures that the variable is treated correctly in your code.
There are different ways to call variables, and the correct method depends on the variable type. For example, numbers are called with %r or %d, while strings are called with %s.
Here's a quick reference to the different ways to call variables:
The use and effects of these different calls are explained in more detail in Exercises 6 and 21, so be sure to check those out for more information.
More Practice
In the world of Python, practice is key to mastering the basics. To help you solidify your understanding of variables and data types, let's dive into some more practice exercises.
You can use %r to help when concatenating strings, but keep in mind that it doesn't coerce the variable into a format, unlike %s or %d.
Here are some special characters to keep in mind when working with strings: \' for an apostrophe, \t for a tab, \\ for a backslash, and
for a new line.
In the next script, you can import an external module and use its functions as methods. This is useful when you want to simulate a script importing another script, even if the script was previously run in IPython.
Here's a list of special characters you should know:
- \' for an apostrophe
- \t for a tab
- \\ for a backslash
for a new line
File Operations
File operations are a crucial part of coding, and understanding how to work with files can be a game-changer for any project. You can open a file in read, write, or append mode using the 'r', 'w', or 'a' argument, respectively.
The 'w' argument is used for writing, but if you don't specify it, the default is 'r' mode, which means you can't write to the file. You can also use 'w+' or 'r+' for read-write mode, and 'a+' for read-append mode.
You might enjoy: Learn to Code in R
To open a file, you can use the open function, which takes two arguments: the filename and the mode. For example, open('file', 'w') opens a file called 'file' in write mode.
You can read the whole file at once using the readlines function, which returns a list of lines in the file. This is useful for reading big files, but be aware that it loads the whole file into memory at once.
Here's a summary of the different file operations:
The 'seek' function allows you to move the file pointer to a specific position in the file, kind of like rewinding a tape. This can be useful for reading or writing specific parts of a file.
You can also use the readline function to read one line at a time, which can be useful for reading big files or files with a lot of lines. The readline function returns a string containing the next line in the file.
When working with files, it's a good idea to close them when you're done with them to free up system resources. You can use the close function to close a file.
Functions
Functions are a crucial part of coding, and understanding how they work is essential to writing effective code. A function can have no, one, or several arguments.
You can give different names to functions and arguments to avoid confusion. This is especially important when working with multiple functions and variables.
Functions can also return something or not, and you can use various formatting options to represent different data types, such as %r for raw, %d for digit, and %s for string.
A fresh viewpoint: Is Transfer Learning Different than Deep Learning
Why Your Series?
I've always wondered why some programming books are more effective than others, and I think I've found a clue. The Learn to Code The Hard Way series is a great example of this, and its name is a big part of its success.
The series was originally called "the hard way" because the author, Zed Shaw, considered it a more challenging approach to learning to code. People thought it was hard because they were actually typing in code and learning to write it.
See what others are reading: How Hard Is It to Learn to Code
Typing in code is how most programmers learn, and it's a hands-on approach that's effective for beginners. In fact, most programming books at the time were geared towards experienced programmers, not beginners.
The author had to put a warning at the beginning of the book: "if you're an expert, you're going to hate this book." But for beginners, it was a great resource.
If this caught your attention, see: Learn to Code Book
Functions Can Return Values
Functions can be used to perform operations and return values. This is useful for reusing code and making it more efficient.
You can use different types of functions, such as integer, float, and string functions, to perform various operations. For example, the integer function can be used to perform mathematical operations, while the string function can be used to manipulate text.
Here are some examples of how to use functions to return values:
These formats can be used to return different types of values from functions. For example, you can use the %r format to return a raw value, or the %s format to return a string value.
It's worth noting that some formats are safer to use than others. For example, using %r is generally safer than using %s, as it can help prevent errors caused by unexpected input.
Composition
Composition is a powerful tool for writing clean and efficient code. It solves the problem of reuse by giving you modules and the ability to call functions in other classes.
In composition, you're not using inheritance to create a parent-child relationship, but rather a has-a relationship, where one class has another class that it uses to get its work done.
This approach is especially useful when you have code that's used in many different unrelated places and situations. In fact, it's recommended to use composition to package code into modules that are reused throughout your software.
Here's a quick guide to help you decide when to use composition:
By using composition, you can avoid duplicated code and make your software more maintainable and efficient. It's a simple yet effective way to write clean code that's easy to understand and modify.
Override Explicitly
When you explicitly override a function, it can run multiple versions of that function.
This is because the variable you're using to call the function can be an instance of a class that overrides it.
In some cases, the function you're trying to override might not be defined in the class you're using, but it is defined in a parent class.
This means you'll need to make sure you're using the correct variable to call the function.
For example, if you have a Parent class with an override function and a Child class that extends Parent, using an instance of Child will run the override function defined in Child.
Control Structures
Control structures are the backbone of programming, and they're actually pretty straightforward once you grasp the basics. In the context of code, control structures determine the order in which instructions are executed.
Conditional statements, like if-else statements, are a type of control structure that allows your code to make decisions based on certain conditions. For example, as seen in the article section, you can use an if-else statement to check if a variable is greater than or equal to a certain value, and then execute different code based on that condition.
Loops, on the other hand, are used to repeat a set of instructions until a certain condition is met. For instance, as demonstrated in the article section, you can use a while loop to keep asking the user for input until they enter a valid number.
Loops and Lists
Loops and Lists are powerful tools in programming that allow us to automate repetitive tasks and work with collections of data. We can use loops to iterate through lists, which are like arrays that can hold different types of data.
A key thing to remember when working with mixed lists is that we need to use %r to print their contents, since we don't know what's inside them. For example, in Exercise 32, Loops and Lists, we see that when we try to print a mixed list, we get a string representation of it.
We can also use the range function to create lists with specific numbers of elements. The range function takes three arguments: start, stop, and step. For example, range(5) creates a list with the numbers 0 through 4, while range(1, 5) creates a list with the numbers 1 through 4.
Two-dimensional lists, also known as lists of lists, are lists that contain other lists as elements. These can be useful for representing tables or matrices of data. For example, in Exercise 32, we see a two-dimensional list with two rows and three columns.
Here are some examples of how to create different types of ranges using the range function:
Loops are also useful for iterating through lists and performing actions on each element. In Exercise 34, Accessing Elements of Lists, we see an example of using a for-loop to iterate through a list of strings.
While Loops
While Loops are a type of control structure used to execute a block of code repeatedly until a certain condition is met.
They require an initialization, a condition, and an increment or decrement to control the loop.
In Exercise 33, a While Loop is used to generate numbers from 0 to 6 with an increment of 2.
The user is prompted to enter an integer, a maximum, and an increment, and the loop runs until the maximum value is reached.
The loop starts with i = 0, and at each iteration, the current value of i is added to the list of numbers.
The loop continues until i exceeds the maximum value, in this case, 6.
Here's a simple example of how the While Loop works:
At each iteration, the current value of i is added to the list of numbers, and the loop continues until i exceeds the maximum value.
Even More Practice
In this section, we'll dive deeper into control structures with some hands-on practice.
To start, consult the manual for guidance on Exercise 25, Even More Practice. This exercise involves running a Python script called ex25.py to identify any errors.
First, run python ex25.py to find out any errors. This step is crucial in identifying potential issues with the code.
Next, use the Python engine to run pieces of code from this file, rather than triggering the whole code from this file.
Check out file 25_1.txt for further clarification on the exercise.
In the next script, you import the above script (ex25.py) as an external module and use its function as methods. This simulates a script importing another script.
Consider reading: Learn How to Code Tetris Game Python
Here's a step-by-step guide to completing this exercise:
- Run python ex25.py to find out any errors.
- Use the Python engine to run pieces of code from this file.
- Check out file 25_1.txt for further clarification.
By following these steps, you'll be able to complete Exercise 25, Even More Practice and gain a better understanding of control structures in Python.
Repetition
Repetition is a crucial aspect of learning any programming language, including Python. It's a well-known fact that programmers don't really have to remember every fine detail - they only need to remember the source where they can look a given detail up.
In the context of Python, repetition can be seen in exercises like Loops and Lists, where you're expected to manually type code to reinforce your understanding of concepts like mixed lists and range functions. For example, you're asked to build lists using the range function, which can be done in various ways, such as range(5): [0, 1, 2, 3, 4] or range(0, 10, 2): [0, 2, 4, 6, 8].
Zed, the author of LPTHW, warns against copy-and-pasting code snippets, instead encouraging you to type all code manually to help you remember Python constructs. This approach may work for some people, but it's not self-evident that it's efficient for every novice programmer.
A different take: How to Learn to Code in Python
In exercises like While Loops, you're presented with examples of how to use loops to generate numbers within a certain range. For instance, using a while loop, you can generate numbers from 0 to 6 with an increment of 2, as shown in the example: range(0, 10, 2): [0, 2, 4, 6, 8].
Data Structures
Data Structures are a fundamental part of coding, and understanding them is crucial for any aspiring programmer. You can work with mixed lists, which contain a mix of different data types, like numbers and strings.
In Python, you can use the %r format specifier to print the contents of a mixed list, as shown in the example: I got 'pennies'. This is useful when you don't know what's in the list.
You can also build lists from scratch using the range function, which generates a sequence of numbers. For example, range(5) generates the list [0, 1, 2, 3, 4]. This is a powerful tool for creating lists of numbers.
Here are some examples of using the range function:
Dictionaries, on the other hand, are a type of data structure that stores key-value pairs. You can use the .get() method to retrieve a value from a dictionary, even if the key doesn't exist. For example: print "%r" % stuff.get('color', None) will print None if the key 'color' doesn't exist in the dictionary.
Accessing List Elements
You can access elements of a list using a for-loop, which is generally better than a while-loop. Most of the time, a for-loop is a more elegant solution.
A for-loop is particularly useful when you need to iterate over a list and perform an action on each element. For example, if you have a list of words, you can use a for-loop to print each word.
You can also use the index of an element to access it, but be careful not to go out of range. If you try to access an element that doesn't exist, you'll get an error.
Here are some examples of accessing elements of lists:
For example, if you have a list of fruits, you can use a for-loop to print each fruit, or you can use the index to access a specific fruit.
Dictionaries
Dictionaries are a fundamental data structure in programming, and they're incredibly useful for storing and retrieving data.
A dictionary has keys associated with values, and order doesn't matter. If you supply the key, you will get the value.
If the value doesn't exist when you call it, it will turn up an error. To avoid this, you can use the `.get()` method, which allows you to specify a default value to return if the key doesn't exist.
Here's an example of how to create a mapping of state to abbreviation:
```html
Dictionary 'cities': {'FL': 'Jacksonville', 'CA': 'San Francisco', 'MI': 'Detroit', 'OR': 'Portland', 'NY': 'New York'}
```
This dictionary maps state abbreviations to city names. You can access the city name for a given state by using the state abbreviation as the key.
If you try to access a key that doesn't exist, you'll get a `KeyError`. To avoid this, you can use the `.get()` method, like this: `print "%r" % stuff.get('color', None)`. This will return `None` if the key doesn't exist.
Here's a list of key concepts to keep in mind when working with dictionaries:
- A dictionary has keys associated with values.
- Order does not matter.
- You can use the `.get()` method to access a key's value, even if it doesn't exist.
- If a key doesn't exist, `.get()` will return `None` or a default value you specify.
Lack of
The lack of hands-on experience in some exercises can hinder learning. In the book Learn Python the Hard Way, the "Study Drills" often don't provide real exercises, unlike the ones in Think Python.
A good exercise should have a precise end goal, as seen in the exercise from Think Python, where the student is asked to write a faster, simpler version of a function using a dictionary. This type of exercise unleashes creativity by forcing the student to find the solution.
The Study Drills in Learn Python the Hard Way sometimes lack hands-on experience, as seen in Chapter 47: Automated Testing. The drills in this chapter don't provide the same level of challenge as a good exercise should.
To truly learn, exercises should provide a clear goal and not hold the student's hand too much. This is evident in the Think Python exercise, where the student is given a specific task to complete using a dictionary.
Related reading: Is Morse Code Hard to Learn
Object-Oriented Programming Fundamentals
You can import a file with functions and variables from another module, but both files must be in the same directory, unless you specify the path.
To access functions and variables from another module, you can use a class instead of importing the module. This is because a class method is essentially a class function.
Instance variables are accessed using the dot notation, like instance.class_function() or instance.class_method(). This is also known as dot notation syntax.
Multiple inheritance is generally avoided due to its complexity, but it can be useful when you need to reuse code from multiple sources.
Composition is a technique used to solve the problem of reusable code by allowing you to call functions in other classes. This is in contrast to inheritance, which creates a mechanism for implied features in base classes.
Here are some key differences between inheritance and composition:
Implicit inheritance allows child classes to inherit behavior from parent classes by default. This can be useful for repetitive code that needs to be used in many classes. However, actions on the child can also override or alter actions on the parent.
To create an empty block in a class, you can use the pass statement. This tells Python that there's nothing new to define in the class, and it will inherit all its behavior from the parent class.
Frequently Asked Questions
Is LearnCodeTheHardway good?
LearnCodeTheHardway is a valuable resource for learning Python, but it doesn't offer instruction, mentorship, or job assistance
What is the hardest way to code?
The hardest way to code is often considered to be INTERCAL, a programming language designed to be intentionally difficult to use, with a unique syntax and semantics that can be challenging even for experienced programmers. Its complexity and unconventional design make it a notorious choice for those seeking a coding challenge.
Sources
- https://learn.onemonth.com/learn-code-the-hard-way/
- https://www.vice.com/en/article/in-defense-of-learning-code-the-hard-way/
- https://ugoproto.github.io/ugo_py_doc/learn_python_the_hard_way/
- https://en.wikipedia.org/wiki/Zed_Shaw
- https://soshace.com/2019/09/17/learn-python-the-hard-way-a-detailed-book-review/
Featured Images: pexels.com