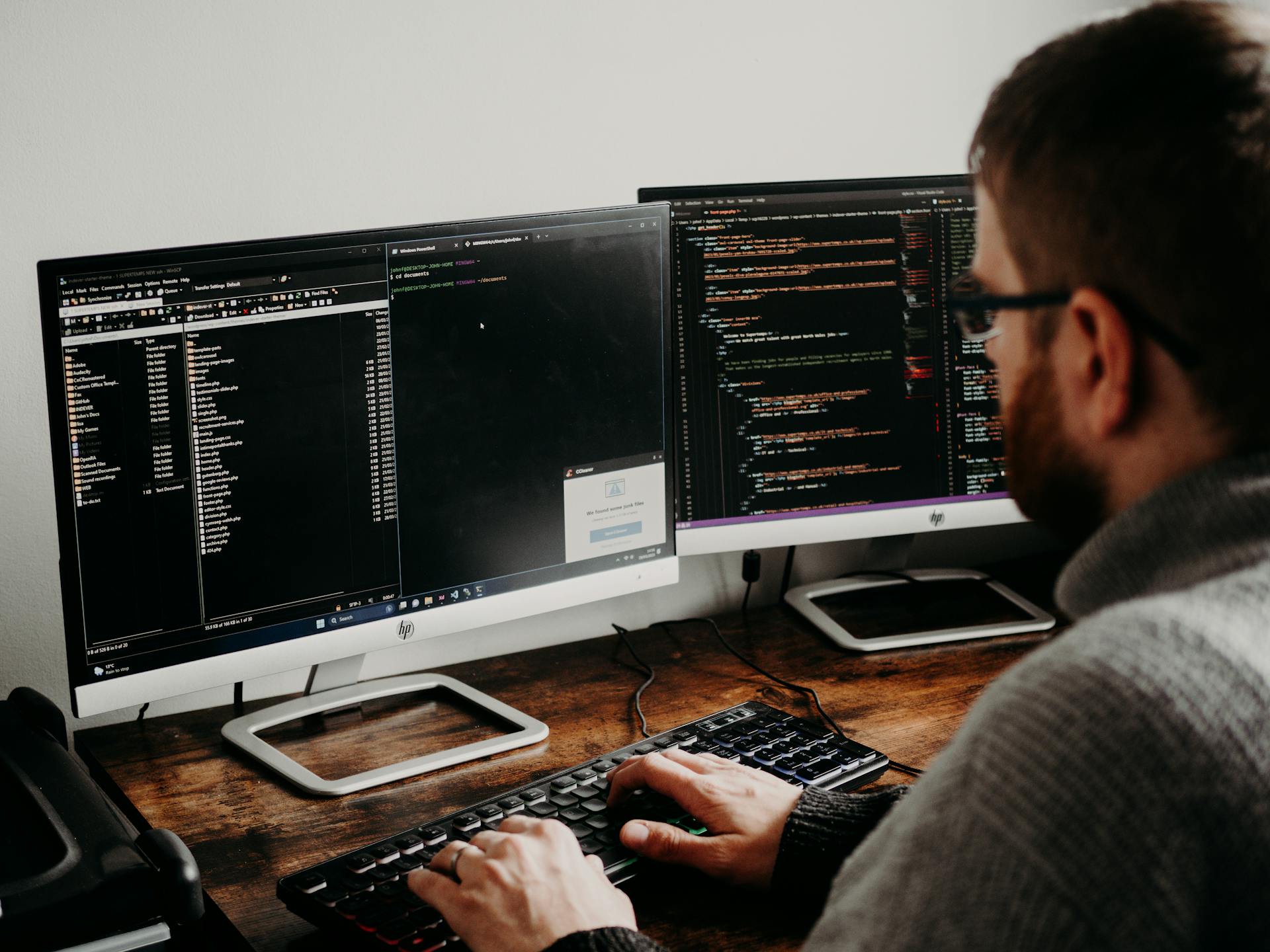
Learning to code Lua is an exciting journey, and with this comprehensive guide, you'll be well on your way to becoming proficient in this versatile scripting language.
Lua is a lightweight, high-performance language that's perfect for game development, embedded systems, and scripting.
One of the best things about Lua is its simplicity, making it an ideal language for beginners to learn.
Lua has a small but dedicated community, which means you'll find plenty of resources and libraries to help you along the way.
On a similar theme: What Is the Hardest Code Language to Learn
Getting Started
To begin learning Lua, you'll need a code editor or IDE, such as Notepad++ or Visual Studio Code, to write and run your code.
Lua is a lightweight language, so you can get started with just a basic text editor if you don't have any other software installed.
You can download the Lua interpreter from the official Lua website, which includes a distribution package that includes the Lua executable and several libraries.
The Lua interpreter is a command-line tool that you can use to execute Lua scripts from the command line.
You can also use online platforms like Repl.it or Ideone to write and run Lua code without installing any software.
It's a good idea to have a basic understanding of programming concepts like variables, data types, and control structures before diving into Lua.
To get familiar with Lua syntax, start with simple scripts like the "Hello, World!" program, which is a great way to test your setup and get a feel for the language.
Basic Concepts
Lua's syntax is straightforward and easy to grasp. You can start writing Lua code as soon as you open a script page in Roblox Studio.
Local variables are limited to their chunk or block, while global variables are available throughout the namespace. This means you don't need to worry about explicit type declaration, and you can focus on writing your code.
Here's a quick rundown of the basic data types in Lua: strings, numbers, Booleans, and nil. You can use the type function to get the data type of any variable.
Why Use Lua
Lua is a lightweight language that's perfect for embedded systems and applications where performance is critical. It has a tiny memory footprint and fast execution speed.
Lua's design makes it easy to learn, even for beginners. Its straightforward syntax and minimalistic design allow you to pick up and start coding quickly.
One of the key benefits of Lua is its extensibility. It can be easily integrated with other programming languages, especially C and C++. This makes it a powerful tool for adding scripting capabilities to existing applications.
Lua supports multiple programming paradigms, including procedural, object-oriented, and functional programming. This versatility allows developers to use the style that best fits their needs.
Lua is widely used in the gaming industry for scripting game logic, thanks to its speed and flexibility. Popular game engines like Unity and Corona SDK use Lua for their scripting needs.
Here are some of the key reasons why Lua is a great choice:
- Lightweight and fast
- Easy to learn
- Extensible
- Versatile
- Lua scripting in game development
- Active community and resources
Variables and Data Types
Variables in Lua are pretty straightforward. They're like containers that hold data, and you don't need to declare a variable's type beforehand. You can think of them as labels that you give to a storage area where your programs can manipulate the data.
In Lua, variables can be either local or global. Local variables are limited to their block or chunk, while global variables are available throughout the namespace. This means that if you declare a variable inside a function, it's only accessible within that function, but if you declare it outside a function, it's accessible everywhere.
Lua allows multi-variable assignment in one line, which can be pretty handy. For example, you can assign multiple values to multiple variables at the same time. Here's a table summarizing the basic data types in Lua:
You can use the type function to determine the type of a value, which returns the name of the type as a string. For example, if you have a variable x = 42, the type(x) function would return the string "number".
Relational
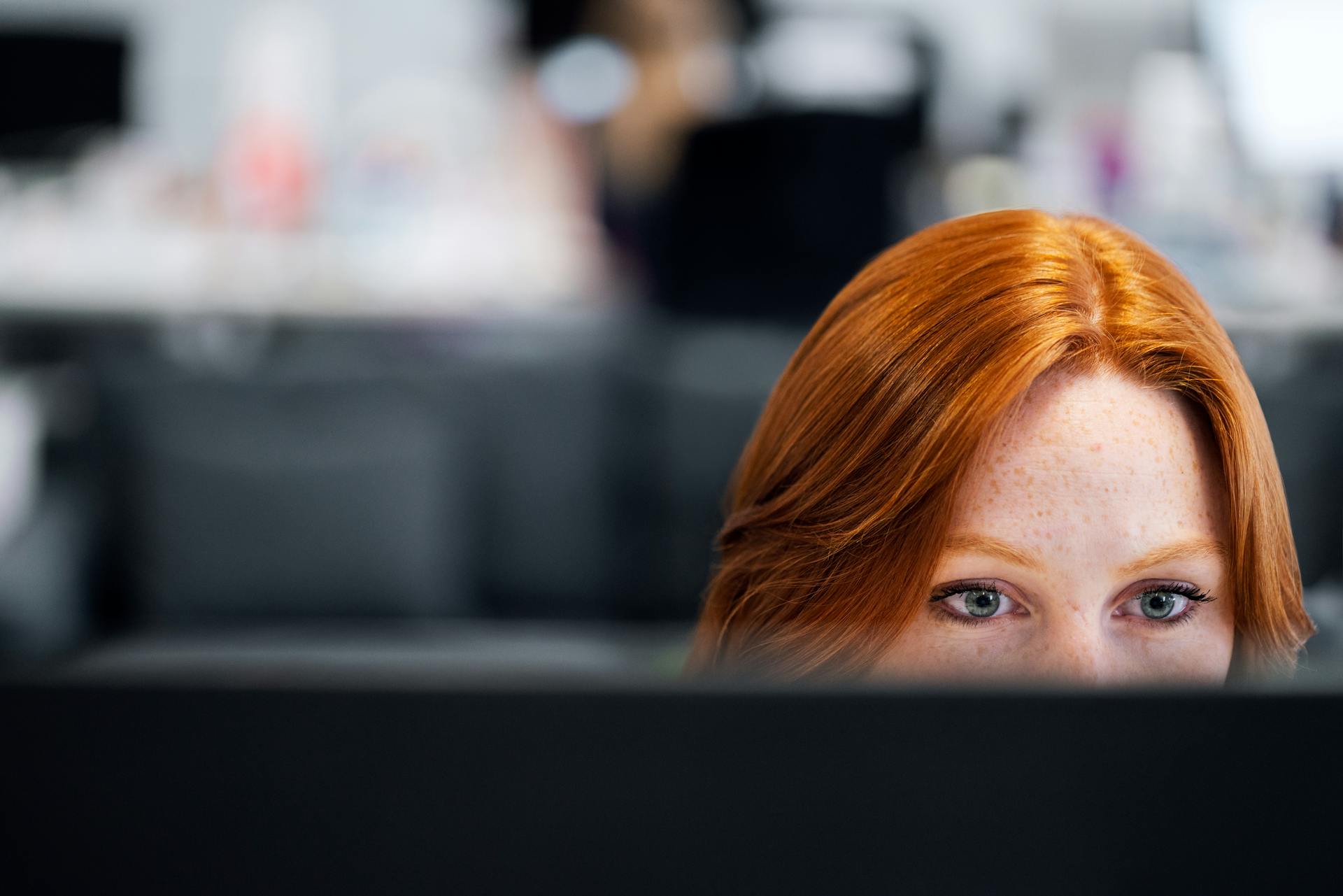
Relational operators are the ones we'll use in conditionals and loops because they return a Boolean value that evaluates to true or false.
There are six relational operators in total.
The equal operator is ==, which checks if two values are the same.
The not equal operator is ~=, which checks if two values are different.
The less than operator is <, which checks if one value is smaller than another.
The greater than operator is >, which checks if one value is larger than another.
The less than or equal to operator is <=, which checks if one value is smaller than or the same as another.
The greater than or equal to operator is >=, which checks if one value is larger than or the same as another.
Metatables and Metamethods
Metatables and metamethods are Lua's way of customizing the behavior of tables.
They can be a secret ingredient that add a unique flavor to your programs. Metatables are used in Lua to change the behavior of tables. Metamethods are a way of overloading the behavior of Lua tables and they are stored in the Metatables. Lua offers a variety of metamethods to handle different operations.
Metamethods are stored in the Metatables. This allows for custom behavior in tables. Metatables can be used to define custom addition for tables. This can be achieved by using metamethods.
Operators and Expressions
Operators and Expressions are the building blocks of any programming language, and Lua is no exception. In Lua, operators are used to perform operations on variables.
Lua has several types of operators, including arithmetic, relational, logical, and miscellaneous operators. These operators can be used to perform operations such as addition, subtraction, multiplication, and division.
Here are some examples of arithmetic operators in Lua:
You can use these operators to perform calculations on variables, like this: `local sum = 10 + 5`. The result of this expression would be stored in the variable `sum`.
Expressions in Lua are evaluated by the interpreter and return a value. They can be combined using operators, and can also include literals, variables, and function calls. Expressions can be grouped with parentheses to clarify the order of operations.
For example, the expression `(10 == 5)` would evaluate to `false`, because 10 is not equal to 5.
Control Structures
Control structures guide the flow of your program, and Lua has several types to help you achieve this. In Lua, you can use conditionals, which are similar to the classic if-else block found in other languages, with a few differences in formatting.
The while loop in Lua takes a condition and loops through a set of given statements until the condition is true. It's worth noting that the statements are initially only executed if the condition is true; if it's false then they won't be executed at all.
Lua also offers a for loop, which takes a series of conditions and executes a group of statements a known number of times. The conditions work like this: in the first argument, you pass a variable that will be manipulated by the loop structure. The second argument is a cap that ends the loop when the first initial variable gets to it.
Decision-Making
Decision-making is a crucial aspect of programming, and Lua offers a range of decision-making structures to help you make informed choices in your code.
Lua offers decision-making structures such as if-else blocks, which are similar to those found in other programming languages. These blocks can be used to create conditionals that check for specific conditions and execute different blocks of code based on the outcome.
For another approach, see: Learn How to Code Google's Go Golang Programming Language
The if-else block is a fundamental structure in Lua, and it's used to make decisions based on conditions. You can create as many elseif conditions as you need to provide more depth to your decision-making process.
In a game like Roblox, you might use decision-making structures to determine whether a character should collect a food item or not. This is where Lua's decision-making structures come into play, helping you create complex logic and make informed decisions in your code.
Lua's decision-making structures are designed to be easy to use and understand, making it a great language for beginners and experienced programmers alike.
Explore further: Create with Code Unity Learn
Higher-Order Closures
Higher-Order Closures are a powerful tool in programming that allows functions to be treated as variables.
They enable the creation of complex behaviors by enabling functions to take other functions as arguments or return functions as output.
A simple example of a Higher-Order Closure is the "makeAdder" function, which returns a new function that adds a fixed number to its input.
This is demonstrated in the example where "makeAdder(5)" returns a new function "addFive" that adds 5 to its input, resulting in "addFive(10)" outputting 15.
Higher-Order Closures can be used to create reusable code and simplify complex logic by breaking it down into smaller, more manageable functions.
Coroutines
Coroutines are a powerful feature in Lua that allows the suspension and resumption of functions. They can be used to create more advanced control operations, such as asymmetrical multiprocessing, non-preemptive multitasking, etc.
Coroutines can be used to create more complex control operations. They allow for the suspension and resumption of functions, which is useful for tasks that need to wait for external events.
A coroutine can be suspended using the coroutine.yield() function. This suspends the function, allowing other tasks to run while the coroutine is paused.
Coroutines can be resumed using the coroutine.resume() function. This continues the function where it left off, allowing the coroutine to pick up where it was suspended.
Using coroutines can make your code more efficient and easier to manage. They allow you to write more complex control operations and handle tasks that need to wait for external events.
Making a Script Executable
You can make a Lua script directly executable on Linux, UNIX, or macOS by adding a hashbang to the first line. This line contains the storage location of the Lua binary file, such as #!/usr/local/bin/lua.
To find the correct storage location of the Lua binary file, use the "which" command in the command line. This command will give you the exact path to the Lua interpreter on your system.
After adding the hashbang, mark the file as executable for the user by using the command "chmod +x filename.lua".
Using Comments in Scripts
Using comments in your scripts is a great way to make your code more understandable and maintainable. Comments are an essential part of any programming language.
They help you outline code components, document code features, and even activate or deactivate code lines. Comments are a lifesaver when you need to explain complex logic or remember why you made a particular choice.
A single-line comment in Lua starts with a double hyphen (--). It's that simple.
You can use multi-line comments to document larger sections of code. To write a multi-line comment, use double square brackets [[ and ]]. These comments can be especially helpful when you need to explain a series of related code lines.
Here's a quick rundown of comment purposes:
- Outlining code components
- Documenting code features
- Activating/deactivating code lines
Frequently Asked Questions
Is Codecademy good for Lua?
Codecademy is a great platform for learning Lua, with easy-to-follow steps and clear instructions that make coding a fun and executable experience. Many learners have reported a blast learning Lua with Codecademy's comprehensive course.
Sources
- https://www.ninjaone.com/blog/lua-programming-language-a-beginners-guide/
- https://dev.to/jd2r/the-lua-tutorial-544b
- https://gamedevacademy.org/lua-programming-tutorial-complete-guide/
- https://www.codingal.com/coding-for-kids/blog/what-is-lua-programming-in-roblox/
- https://www.ionos.com/digitalguide/websites/web-development/lua-tutorial/
Featured Images: pexels.com