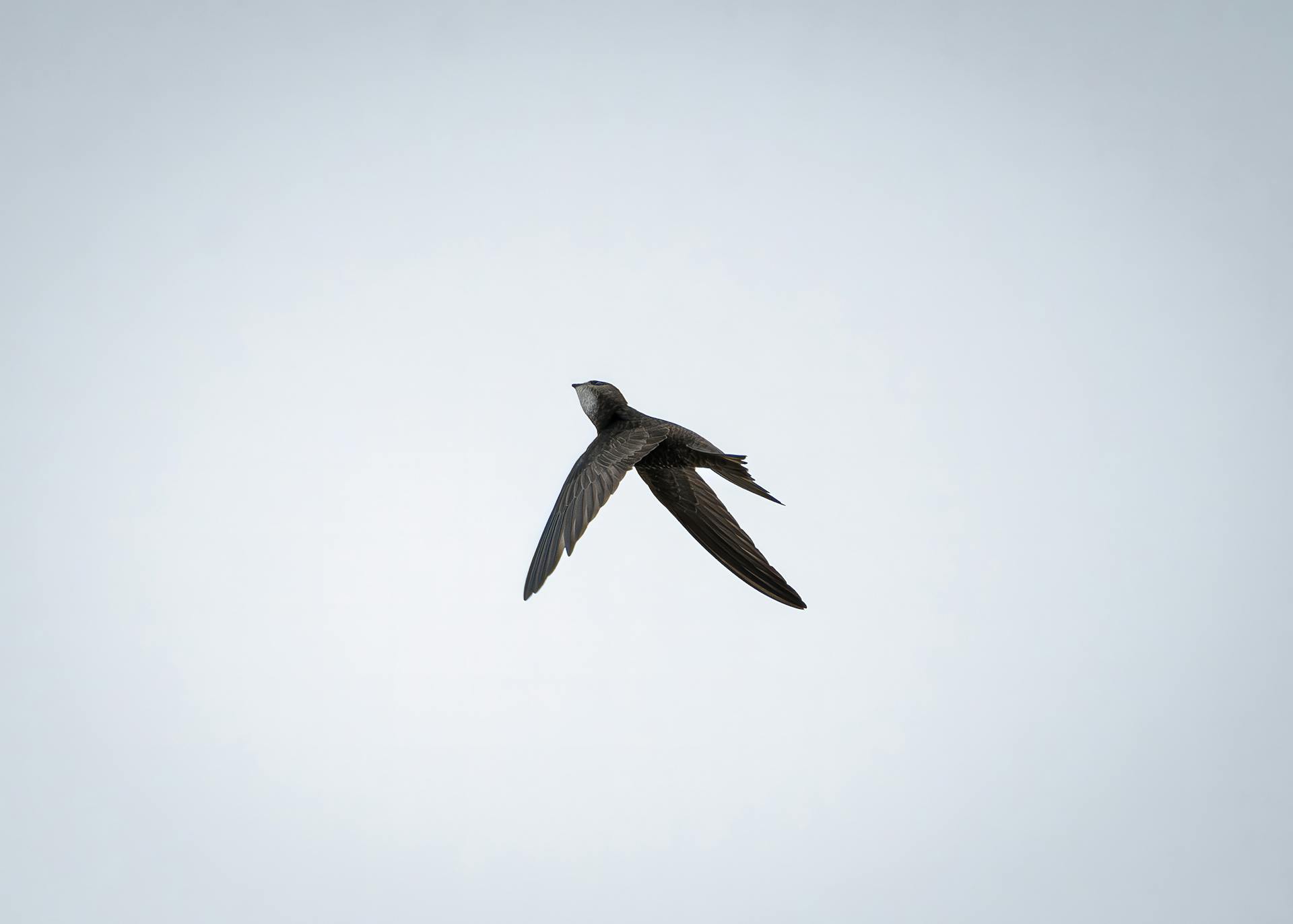
Learning to code Swift is an exciting journey, and it all starts with the basics. You'll need to understand the fundamentals of programming, including data types, variables, and control structures.
Swift is a powerful language that's designed to work seamlessly with Apple's iOS operating system. In fact, it's the primary language used for developing iOS apps.
To get started, you'll want to set up a development environment, which includes installing Xcode, a free IDE provided by Apple. Xcode is an essential tool for any iOS developer.
Swift is a type-safe language, which means it helps prevent errors by ensuring that variables are declared with the correct data type. This is a huge advantage over other languages, where errors can be tricky to track down.
By learning Swift, you'll be able to create a wide range of apps, from simple games to complex productivity tools. The possibilities are endless, and the demand for skilled iOS developers is high.
Worth a look: Learn to Code an App
Getting Started
Getting Started with Swift is a breeze. You can install Xcode on your Mac via the App Store or download it from developer.apple.com.
Swift is a versatile language that can be used for a variety of projects. You can code Swift for iOS, macOS, tvOS, iPadOS, watchOS, and even server-side Swift.
One of the most straightforward ways to code Swift is with a Mac and Xcode. You can also try out the Swift Playgrounds app for iPad, which is a gamified way to learn Swift programming.
To get started with Swift, you'll need a Mac computer to install Xcode. If you don't have a Mac, you can try installing macOS on a PC.
Here are some key ways to get started with Swift:
- Install Xcode on your Mac via the App Store
- Try out the Swift Playgrounds app for iPad
- Use the Swift CLI for Linux for server-side Swift
- Check out Vapor or Kitura for server-side Swift
You can also use Xcode playgrounds to code Swift without building an entire app. This is a great way to try out some Swift code and get a feel for the language.
Getting started with Swift is as easy as installing Xcode on your Mac. You can also use the Swift Programming Language to code server-side apps, which don't live on an iPhone or iPad, but rather on a web server in the cloud.
You might enjoy: What Is the Hardest Code Language to Learn
Learning Swift
Learning Swift is a straightforward process that can be tailored to your learning style. You can start by reading books and following exercises to get a solid foundation in the language. This approach allows you to keep your own pace and make notes on the page.
Tutorials, articles, and guides are also a great way to learn Swift, with many resources available online. You can search for tutorials on Google or DuckDuckGo, or follow your favorite authors such as Swift By Sundell, AppCoda, Swift with Majid, or Hacking With Swift.
To get started with coding Swift, you'll need a Mac computer to install Xcode, or you can try to install macOS on a PC. You can also use the Swift Playgrounds app for iPad, which is a gamified and fun way to learn Swift programming.
You can also use the Swift Programming Language to code server-side apps, which live on a web server somewhere in the cloud. To get started with server-side Swift, you can use the Swift CLI for Linux, or through Vapor or Kitura.
Start with These Topics
Learning Swift can be a daunting task, but breaking it down into smaller topics can make it more manageable. Start with the basics, such as understanding the syntax and variables. You can explore topics like how to create a Swift program, print output to the console, write comments, declare a variable, and use arithmetic operators.
To get started with Swift, you can install Xcode on your Mac via the App Store or download it from developer.apple.com. Alternatively, you can use the Swift Playgrounds app for iPad, which is a gamified and fun way to learn Swift programming.
Swift is an open-source coding language used to build apps for iOS, Mac, Apple TV, and Apple Watch. It was created by Apple to give developers more freedom, control, and flexibility around their code. Swift uses a simple and expressive syntax that is easy to understand, even if you don't have any prior experience with coding.
Intriguing read: Generative Ai Coding
Some must-know topics for learning Swift include:
- How to create a Swift program
- How to print output to the console
- How to write comments
- How to declare a variable
- Arithmetic operators
- Variable data types
- Constants
You can also explore topics like conditionals, loops, and object-oriented programming (OOP) to take your Swift skills to the next level. With practice and dedication, you can become proficient in Swift and start building your own apps.
Next Level Topics
Learning Swift is a lot of fun, and mastering it is even more rewarding. Now that you've learned the basics, it's time to take your skills to the next level.
To build your own iOS apps, you can start by following a beginner's Xcode tutorial. You can also create an iOS game with Swift in Xcode, or learn how to go from app idea to app in just a few steps.
Building a successful iOS app requires more than just coding skills. To increase your chances of success, consider learning how to beat the iOS coding interview, or how to get freelance iOS development projects.
For your interest: Learn Morse Code App
If you're looking to advance your career, you can aim to become a senior iOS developer or learn how to make your skills recession-proof.
Here are some specific next steps to consider:
You can also explore advanced topics in Swift, such as closures, generics, and error handling, to take your skills to the next level.
Functions
Functions are the building blocks of any program, allowing you to execute specific tasks in a program.
A function can be thought of as a reusable piece of code that performs a particular action. For example, a function could add two numbers together in a program.
To define a function, you need to know how to call it, which is a crucial part of programming. You should learn how to define and call a function.
Functions can also return a value, which is useful for storing the result of a calculation or operation. You should learn how to return a value from a function.
When working with functions, you'll come across parameters and arguments. Parameters are the inputs a function expects, while arguments are the actual values passed to the function. You should learn about parameters and arguments.
Default and in-out parameters can also be used in functions. Default parameters provide a default value if no argument is provided, while in-out parameters allow you to modify the original value passed to the function.
You can return multiple values from a function, which can be useful for returning multiple results from a calculation or operation.
Here are the main topics to learn about functions in Swift:
- How to define and call a function
- How to return a value from a function
- Parameters and arguments
- Default and in-out parameters
- Returning multiple values
Classes and Structures
Learning Swift involves understanding the fundamentals of classes and structures, which are key components of the language.
A class is a blueprint for creating objects in Swift, allowing you to define the structure of data in a program. Classes help make code more readable and reduce repetition.
Classes can be used to create instances, which are unique copies of the class. To create a class, you define its properties and methods.
Intriguing read: Visual Studio Code C# Console Application
Class inheritance is a feature that allows one class to inherit the properties and methods of another class. This enables code reuse and helps to create a hierarchy of classes.
A structure is a custom data type that can be used to store related values. Structures are similar to classes, but they don't support inheritance.
To create a structure, you define its properties and methods. You can also create an instance of a structure, which is a unique copy of the structure.
Structures can have mutating methods, which allow you to modify the properties of the structure. This is useful when you need to change the values stored in the structure.
Here are the main topics to focus on when learning about classes and structures:
- What is a class?
- How to create a class and an instance of a class
- Class inheritance and method overriding
- What is a structure?
- How to create a structure
- How to create an instance of a structure
- Structure and mutating methods with structures
Practice and Feedback
Practice and feedback are crucial components of learning to code Swift. To make progress, practice coding for one hour every day, for a year. This habit will help you build momentum and make learning a sustainable process.
Spaced repetition is a technique that can help you retain what you've learned. By learning on Mondays, Wednesdays, and Fridays, and giving your mind rest in between, you can increase comprehension and recall. This technique is backed up by science, making it a valuable tool in your learning arsenal.
To take your learning to the next level, share your project code to get feedback on code structure, technical decisions, and tradeoffs you've made. This will help you identify areas for improvement and refine your skills.
Expand your knowledge: Books to Help Learn Code in Java
Bootcamp
A bootcamp can be a great way to learn Swift programming and iOS development, with many having high success rates.
These immersive classroom/workshop environments usually have one or more tutors who teach you everything you need to know.
Some popular bootcamps include Big Nerd Ranch, DevMountain, and General Assembly, which offer job guarantees or help you find work as a professional Swift developer.
However, bootcamps can be expensive, so it's essential to consider your budget before signing up.
You'll get hands-on experience and work on multiple projects with the help of tutors, which can be a fantastic way to learn and stay motivated.
Code Feedback
Practice coding Swift for one hour every day, for a year. This habit will help you build momentum towards reaching your goal of successfully learning Swift programming.
Getting good at Swift coding isn't a matter of smarts or intelligence, but a matter of exposure: how many hours, effort, and deliberate practice you're investing in exposing yourself to Swift code.
You can get feedback on your Swift code by sharing your project code to get feedback on code structure, technical decisions, tradeoffs you've made, and more.
Spaced repetition is a technique that can increase comprehension and recall. It involves exercising regularly, finding a balance between learning and relaxation, and spacing out the repetitions and exercises.
Here are some ways to get feedback on your Swift code:
- Share your project code with others
- Join online communities or forums where you can get feedback on your code
- Participate in coding challenges or hackathons where you can get feedback from peers and mentors
Remember, practice makes permanent – build a habit and make progress towards your goal. Miss a day or two, and that's alright, just pick it up again the next day.
Here's an interesting read: Learn Your Name in Morse Code Day
How Long Does It Take?
Learning a programming language like Swift takes time and dedication. It takes around one to two months to develop a basic understanding of Swift, assuming you study for about an hour a day.
With consistent effort, you can get to work on your first application with Swift pretty quickly. You can find detailed tutorials online to help you get started.
To become a proficient Swift developer, it's recommended to spend a few months practicing and building applications on your own. This will help you develop a deeper understanding of the language and its frameworks.
Becoming a professional iOS or Apple device developer takes even longer, with a minimum of six months to a year of intense learning and practice required.
Additional reading: Can I Develop Net Core Application in vs Code Mac
Online Courses
Online courses are a great way to learn Swift, and many of them are available for free or at a low cost. You can choose from a variety of formats, including videos, tutorials, and interactive coding exercises.
One popular option is to take a course on a platform like Coursera, where you can earn a shareable certificate of completion. The iOS App Development with Swift Specialization course, for example, takes about five months to complete and covers the fundamentals of iOS application development through Swift.
If you're looking for something more flexible, you can try a boot camp or a series of video tutorials. Many online courses are designed to be completed at your own pace, so you can learn Swift in the time and place that works best for you.
Some popular online courses for learning Swift include:
- Learn Swift by Codecademy
- Developing iOS 10 Apps with Swift by Stanford University
- iOS Development in Swift by Plymouth University
- Udacity: Swift for Beginners
These courses can be a great way to get started with Swift, and many of them are designed to help you build real-world projects and gain practical experience.
iOS Development
To learn iOS development, you can start with Apple's free SwiftUI Tutorials course, which takes approximately four to five learning hours to complete.
This course covers the basics of Swift development, even if you have no experience with iOS and Swift, and features videos and picture tutorials to help you learn as you follow the steps.
Swift is the 12th most searched for programming language, according to the TIOBE Index, which tracks the popularity of top programming languages using search engine traffic.
For more advanced developers, Apple has published a number of excellent free books and courses on the Apple Developer website, including Debugging UIKit Views with Reveal, a 36-minute free course that helps you find and fix rendering problems in UIKit views.
iOS App Development with Swift Specialization on Coursera is a five-month course that covers the fundamentals of iOS application development through Swift and includes dozens of tutorials to help you learn design patterns, protocol extensions, and more.
You can also learn how to build your first iOS app with the Swift: Build Your First App (2021) YouTube video tutorial, which features the latest versions of Xcode 12 and Swift 5.
Discover more: Learn to Code Python for Free
iOS App Development on Coursera
If you're looking to learn iOS app development on Coursera, you're in luck. The iOS App Development with Swift Specialization is a great place to start, and it's delivered by the University of Toronto. This specialization covers the fundamentals of iOS application development through Swift, and it takes about five months to complete.
You'll have access to dozens of tutorials that will help you learn design patterns, protocol extensions, and more. By the end of the program, you'll receive a shareable certificate of completion, which is a great addition to your portfolio.
As part of this specialization, you'll learn how to design and develop apps for iOS, use XCode, and other development tools. You'll even get to work on projects, such as building your own iOS app or game. This hands-on approach will help you gain practical experience and build a portfolio of work to showcase your skills.
Debugging UIKit Views
Debugging UIKit Views is a crucial part of iOS development. Debugging UIKit Views with Reveal is a 36-minute free course that's perfect for advanced developers.
This course is ideal for those with programming experience in UIKit views and Xcode. You should consider this course if you need a comprehensive tool to find and fix rendering problems in UIKit views.
Reveal is a powerful tool that helps you find auto layout constraint problems, modify layout and rendering even as your app is running, and fix other debugging issues that may arise.
Programming Fundamentals
To learn Swift programming, you need to acquire two essential skills, which we'll discuss next.
You can acquire the basic skills needed for Swift development through online courses and tutorials. This is how I learned to code, and it's a great way to get started.
To learn the fundamentals of Swift, consider taking the "Swift Fundamentals" course on Pluralsight. This course has a high rating from hundreds of students and is a great learning path for beginners.
The "Swift Fundamentals" course teaches you the essential steps in the app development process through tutorials and covers topics like working with data and Swift playgrounds. It takes about three to four learning hours to complete.
Discover more: No Code Application Development Platform
If you prefer video tutorials, check out the "Swift Programming Tutorial for Beginners" by CodeWithChris on YouTube. This free tutorial has over four million views and covers topics like variables, data types, and switch statements.
This tutorial will give you a solid foundation in Swift programming and help you become a professional developer.
Recommended read: Learn How to Code Google's Go Programming Language
Frequently Asked Questions
Is swift code easy to learn?
Swift is designed to be easy and fun to learn, making it a great choice for developers of all levels. Its simplicity and safety features make it an ideal language for beginners and experienced programmers alike.
How long will it take to learn Swift?
Learning Swift typically takes 1-2 months with daily study, or less time with part-time or full-time dedication. Get started and discover the fundamentals of Swift in a shorter period than you think.
Sources
- Swift (apple.com)
- Swiftui (apple.com)
- Learn Swift with online courses and programs (edx.org)
- Swift documentation site (swift.org)
- Xcode (apple.com)
- SwiftUI Tutorials – Apple Developer (apple.com)
- iOS 12 Swift 4.2 – The Complete iOS Development with Swift Bootcamp – Udemy (udemy.com)
- Swift Tutorial Full Course for Beginners – freeCodeCamp (youtube.com)
- Swift Fundamentals – Pluralsight (pluralsight.com)
- Free Swift Tutorial – Code Conquest (codeconquest.com)
- AppSync Setup with Amplify – Ray Wenderlich (raywenderlich.com)
- iOS App Development with Swift Specialization – Coursera (coursera.org)
- Debugging UIKit Views with Reveal (raywenderlich.com)
- Learning Functional Programming with Swift – LinkedIn (linkedin.com)
- SwiftUI by Tutorials (First Edition): Declarative App Development on the Apple Ecosystem – Ray Wenderlich Tutorial Team (amazon.com)
- Swift Tutorial – Tutorialspoint (tutorialspoint.com)
- Swift Programming Tutorial for Beginners – CodeWithChris (youtube.com)
- Free Swift and iOS Tutorials – Hacking with Swift (hackingwithswift.com)
- Learn Swift Fast (2020) Full Course For Beginners – Code With Chris (youtube.com)
- StackOverflow (stackoverflow.com)
- Dev.to (dev.to)
- Apple Developer Forums (apple.com)
- r/iOSProgramming (reddit.com)
- Swift projects to learn programming - DevProjects (codementor.io)
- Learning to code as a 30-year-old kid with Apple's Swift ... (arstechnica.com)
Featured Images: pexels.com