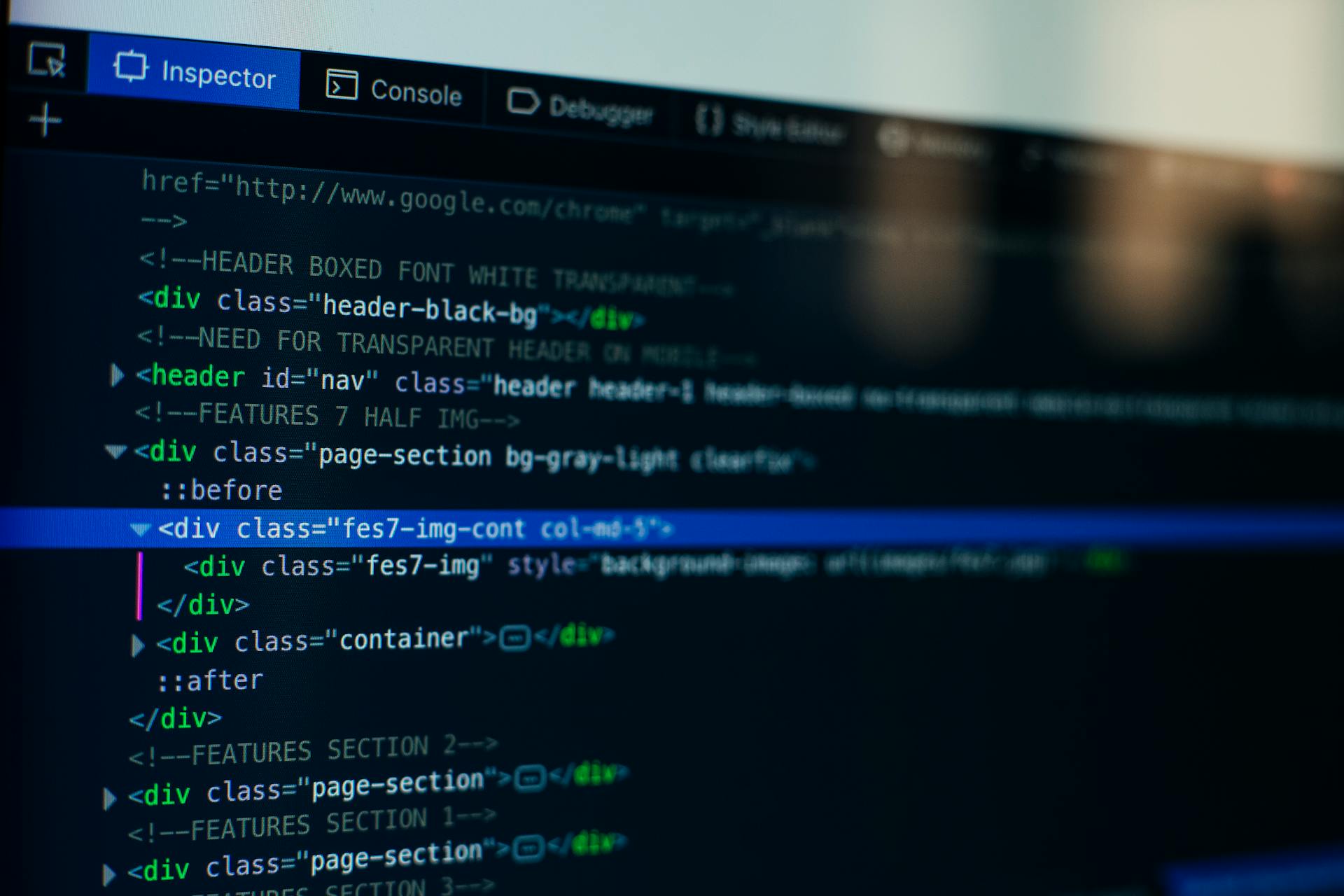
Developing a .NET Core application in Visual Studio Code (VS Code) on a Mac is entirely possible, thanks to the .NET Core SDK.
The .NET Core SDK is a cross-platform development kit that allows you to create and run .NET Core applications on Windows, macOS, and Linux.
You can install the .NET Core SDK on your Mac by running a simple command in the terminal, which will download and install the required components.
This includes the .NET Core runtime, the .NET Core SDK, and the .NET Core CLI, which you'll use to create and manage your .NET Core projects.
See what others are reading: Create with Code Unity Learn
Prerequisites
To develop a .NET Core application in VS Code on a Mac, you'll need to meet some prerequisites.
You'll need Visual Studio Code with the C# Dev Kit installed. For more information on how to install extensions on VS Code, check out the VS Code Extension Marketplace.
Additionally, you'll need the .NET 8 SDK.
You can install the .NET 8 SDK from the official .NET website.
Here are the specific tools you'll need:
- Visual Studio Code with C# Dev Kit installed
- .NET 8 SDK
Installation
To install .NET on your Mac, you can use the install-dotnet.sh script, which is an easy way of installing .NET.
You can also download the dotnet-install script from https://dot.net/v1/dotnet-install.sh and follow the steps outlined in the script. This includes opening a terminal, navigating to a folder where you want to download the script, downloading the script using wget, giving the script execute permissions, and running the script to install .NET.
The script defaults to installing the latest long term support (LTS) version, which is .NET 8. You can choose a specific release by specifying the channel switch, and include the runtime switch to install a runtime.
To install .NET for Visual Studio Code, you can use the C# Dev Kit extension, which will install .NET for you if it's not already installed. Alternatively, you can download and install the .NET SDK on your Mac and then open up Visual Studio Code to install the C# Dev Kit extension.
The necessary tools for .NET development on a Mac include Visual Studio Code, the C# Dev Kit extension, and the .NET SDK.
On a similar theme: Install Windows on Mac
Install .NET via Script
To install .NET via script, you can use the dotnet-install script, which is available for download from https://dot.net/v1/dotnet-install.sh. This script is perfect for automation and unelevated installs of the runtime.
First, open a terminal and navigate to a folder where you want to download the script, such as ~/Downloads. If you don't have the wget command, install it with Brew by running the command "brew install wget".
The script defaults to installing the latest long term support (LTS) version, which is .NET 8. You can choose a specific release by specifying the channel switch. To download the script, run the command "wget https://dot.net/v1/dotnet-install.sh".
To give the script execute permissions, run the command "chmod +x dotnet-install.sh". Then, run the script to install .NET by navigating to the script's location and running "./dotnet-install.sh".
Here's a step-by-step guide to installing .NET via script:
- Download the dotnet-install script from https://dot.net/v1/dotnet-install.sh.
- Give the script execute permissions by running the command "chmod +x dotnet-install.sh".
- Run the script to install .NET by navigating to the script's location and running "./dotnet-install.sh".
Note that the script defaults to installing the latest SDK to the ~/.dotnet directory. If you want to install a runtime, include the runtime switch in the script.
Install the Environment
To install the environment, you'll want to start by installing .NET. You can do this using the install-dotnet.sh script, which can be downloaded from the dot.net website.
For macOS users, the script is a convenient way to install .NET. To use it, open a terminal and navigate to a folder where you want to download the script. You can then run the script to install .NET.
The script defaults to installing the latest long term support (LTS) version of .NET, which is currently .NET 8. However, you can choose a specific release by specifying the channel switch.
To install .NET with the script, you'll need to download it using the wget command. If you don't have wget installed, you can install it using Brew.
Here are the steps to install .NET using the script:
1. Open a terminal.
2. Navigate to a folder where you want to download the script.
Suggestion: Can I Generate Code Using Generative Ai Models
3. Install wget if you don't have it.
4. Download the script using wget.
5. Give the script execute permissions.
6. Run the script to install .NET.
Alternatively, you can install .NET using a script downloaded from the dot.net website.
To make .NET available system-wide, you may need to add the directory where .NET was installed to the PATH variable. This is especially important if you used the manual installation method or the .NET install script.
Here are the variables you'll need to set:
- DOTNET_ROOT: set to the folder where .NET was installed
- PATH: include both the DOTNET_ROOT folder and the DOTNET_ROOT/tools folder
You can set these variables using the export command in your terminal. For example:
export DOTNET_ROOT=$HOME/.dotnet
export PATH=$PATH:$DOTNET_ROOT:$DOTNET_ROOT/tools
Setting Up VS Code
To set up VS Code for .NET Core development on a Mac, you'll need to install the .NET SDK and the C# Dev Kit extension. This extension provides intellisense, debugging, and unit test management capabilities.
First, install VS Code on your Mac, as it comes in a macOS edition. Then, open VS Code and install the C# Dev Kit extension from the Microsoft Marketplace. This will also install the C# extension and the .NET install tool.
A fresh viewpoint: Learn to Code on Mac
Here are the steps to follow:
- Install the .NET SDK on your Mac.
- Install the C# Dev Kit extension from the Visual Studio Marketplace.
- Open the Terminal in VS Code to access the command line.
By following these steps, you'll be able to develop .NET Core applications in VS Code on your Mac.
Install Essential Plugins
Installing Essential Plugins is a crucial step in setting up VS Code for ASP.NET Core development. You'll want to install the C# extensions needed for creating ASP.NET Core apps by clicking their icons in the left menu or pressing Ctrl Shift+X to open their list.
To take your development to the next level, install the NuGet gallery extension in VS Code to update and add NuGet packages. This will give you access to a vast library of packages that can help you build and deploy your ASP.NET Core apps.
Visual Studio Code does not offer built-in tools for creating an ASP.NET Core project, so you'll need to install some essential plugins to get started. You can do this by following the steps outlined below:
- Install C# extensions needed for creating ASP.NET Core apps.
- Install the NuGet gallery extension in VS Code.
Remember, having the right plugins installed will make a huge difference in your development experience. So take the time to install these essential plugins and get started with your ASP.NET Core projects!
Integrated Terminal
The Integrated Terminal in VS Code is a game-changer for developers. It allows you to run commands directly from within the editor.
You can use it to run and debug ASP.NET Core applications with ease. This feature is particularly useful for developers who work with ASP.NET Core.
The Integrated Terminal is also ideal for running .net commands, such as migrations or updating dependencies. This saves you time and effort by keeping all your development tools in one place.
With the Integrated Terminal, you can facilitate run-time debugging for mobile development projects. This is a huge advantage for mobile app developers who need to troubleshoot their code quickly.
See what others are reading: Ai for Software Developers
Developing the App
To start developing your .NET Core app in VS Code, you'll need to install the C# Dev Kit extension. This will allow you to create .NET apps from within VS Code.
You can install the C# Dev Kit extension by going to the Extensions Marketplace in VS Code and searching for "C# Dev Kit". Once installed, you'll be able to use it to create and manage your .NET projects.
To create an ASP.NET Core Web API, you'll need to use the VS Code terminal to run .NET command lines. This can be added by going to View and Terminal from the top menu.
Take a look at this: Create Feature for Dataset Huggingface
Use Source Control
As you start developing your app, using source control is a crucial step to ensure your code is organized and easily manageable. Visual Studio Code offers excellent support for C# and ASP.NET Core.
Having multiple versions of your code can get confusing, but with source control, you can track all changes and collaborate with your team seamlessly. Visual Studio Code simplifies writing code with code completions and parameter suggestions.
Good source control practices help you avoid losing your work in case of any issues or errors. This is especially important when working on complex projects, where small mistakes can have significant consequences.
You might enjoy: Android Application Open Source Code
Hello World App
To create a Hello World app, start by going to the Explorer view and selecting Create .NET Project. Alternatively, you can bring up the Command Palette using ⇧⌘P (Windows, Linux Ctrl+Shift+P) and then type ".NET" and find and select the .NET: New Project command.
To get started, you'll need to choose the project template. Choose Console app.
For your interest: Artificial Intelligence App Development
Select Run > Run without Debugging in the upper menu, or use the ⌃F5 (Windows, Linux Ctrl+F5) keyboard shortcut to run your app.
If you're using Visual Studio Code, the C# Dev Kit extension will install .NET for you if it's not already installed.
To learn more about debugging your C# project, read the debugging documentation.
Here's a step-by-step guide to creating a Hello World app:
- Choose the project template: Console app.
- Run your app: Select Run > Run without Debugging or use the ⌃F5 keyboard shortcut.
C# Coding Challenges
C# coding challenges can be a bit tricky, especially for macOS users. For .NET 8 and beyond, Visual Studio is not an option for them.
If you're a Linux user, you've never had the option of using Visual Studio for C# coding challenges.
That's because Visual Studio is not available for Linux users.
Benefits
Visual Studio Code has the same tools as Visual Studio for debugging and running source code: error window and output window.
We can enjoy a faster experience with VS Code than with Visual Studio, thanks to its efficient design.
Downloading Visual Studio Code is free of charge, as it's open-source software.
Several extensions are available that give VS Code a feel of native software, similar to using Visual Studio.
ASP.NET Core Web API
You can create an ASP.NET Core Web API in Visual Studio Code using the .NET command line. To do this, change directory to the folder where you want to create the application and run `dotnet new webapi -o RoundTheCode.VsCode.WebApi`. This will create a new Web API project.
The project will be set up to use Minimal APIs by default, but you can add controllers to the project by adding the `-controllers` option. To create a Web API controller, change directory to the Controllers folder and run `dotnet new controller -o HttpController`.
Here's an interesting read: Artificial Intelligence in Web Development
ASP.NET Core Web API
To create an ASP.NET Core Web API, you can use the .NET command lines in the VS Code terminal. Run `dotnet new webapi -o RoundTheCode.VsCode.WebApi` to create a new Web API project.
This will create a new project in the specified folder, which will be set up to use Minimal APIs by default. However, you can add controllers to the project by running `dotnet new webapi -o RoundTheCode.VsCode.WebApi -controllers`.
Suggestion: Web Programming Education
To create a Web API controller, change directory to the Controllers folder in the VS Code terminal and run `dotnet new controller -o HttpController`. Then, inject the `IHttpService` interface and set up an endpoint that will call the `ReadAsync` method and return it as part of the response.
You can run the Web API project by using the `dotnet run` command, but for best results, use the built-in debugger in VS Code. To set up the debugger, add a file called `tasks.json` that provides information needed to run scripts and build sources.
Here are some popular extensions that can be integrated into ASP.NET Core development:
- Razor Syntax Highlighting: Enhances productivity through Razor Views' syntax (.cshtml files).
- REST client: Visual Studio Code's REST client allows for sending HTTP requests directly, providing an efficient means for debugging and testing APIs.
To launch the ASP.NET Core application in your browser, select the .NET Core Launch (web configuration) in the Run menu, update the root project's DLL in the program field, and then select the configuration to run it.
A Service
To create a service in ASP.NET Core Web API, start by making a new directory called Services in the terminal and then change directory to it.
We'll want to set up an interface and a class for our service, which can be done using the terminal by running the following commands:
The command will create a new interface called IHttpService, and a new class called HttpService.
Check this out: Visual Studio Code C# Console Application
Debugging and Testing
Debugging and testing are crucial steps in developing a robust ASP.NET Core application. You can debug an application in Visual Studio Code by setting up breakpoints and using the Debug View for effortless step-through code debugging and variable inspection.
To run unit test cases, you can use the command line to run all tests in your project from the VS Code or command prompt terminal. This allows you to view a listing of test cases within your project, run them one at a time, or debug individual ones as necessary.
Visual Studio Code offers many extensions designed to optimize the ASP.NET Core experience, such as Razor Syntax Highlighting to enhance productivity through Razor Views' syntax, and the REST client to send HTTP requests directly and provide an efficient means for debugging and testing APIs.
Debug an Application
Debugging an application can be a daunting task, but with the right tools, it can be a breeze. You can debug an application in Visual Studio Code, which offers an unparalleled experience for debugging ASP.NET Core apps.
To set up debugging in Visual Studio Code, you can watch a video that shows you how to add a breakpoint and use it to debug your application. This will also give you a chance to see how to create an ASP.NET Core Web API and add the relevant services and controllers.
One of the best features of Visual Studio Code is its ability to set breakpoints by pressing F9 or clicking the gutter. This makes it easy to debug projects and switch to Debug View for effortless step-through code debugging and variable inspection.
You can also use the REST client in Visual Studio Code to send HTTP requests directly, providing an efficient means for debugging and testing APIs.
Here are some popular extensions designed to optimize the ASP.NET Core experience:
- Razor Syntax Highlighting: Enhances productivity through Razor Views’ syntax (.cshtml files).
- REST client: Allows for sending HTTP requests directly, providing an efficient means for debugging and testing APIs.
Tasks in Visual Studio Code can be set up to run scripts or initiate processes automatically without writing code or entering commands manually. This can save you a lot of time and effort when debugging your application.
Run Tests
Running tests is an essential part of the debugging process. You can run all unit tests in your project from the VS Code or command prompt terminal.
The command line allows you to run all unit tests in one go, just like Visual Studio. This command can be especially useful when you have a large number of test cases to run.
VS Code features its own Test Explorer extension, similar to Visual Studio's .NET Core Test Explorer. This extension allows you to view a listing of test cases within your project.
With the Test Explorer, you can run test cases one at a time or debug individual ones as needed. This level of control can be a big help when you're trying to track down a specific issue.
Frequently Asked Questions
How to install C# in Visual Studio Code for Mac?
To install C# in Visual Studio Code on Mac, search for 'C# Dev Kit' in the Extensions view (Cmd+Shift+X) or let VS Code prompt you to install it when opening a C# file.
How do I create a .NET Core application in Visual Studio?
To create a .NET Core application in Visual Studio, start by selecting "Create a new project" and choose C# as the language. Then, follow the prompts to configure your project, entering "MyCoreApp" as the project name and verifying additional information before clicking "Create
Sources
- https://learn.microsoft.com/en-us/dotnet/core/install/macos
- https://www.roundthecode.com/dotnet-tutorials/how-to-use-vs-code-develop-build-dotnet-application
- https://learn.microsoft.com/en-us/dotnet/core/tutorials/with-visual-studio-code
- https://medium.com/@leadnatic/tips-to-develop-an-asp-net-core-application-using-visual-studio-code-84a19520e7fd
- https://code.visualstudio.com/docs/csharp/get-started
Featured Images: pexels.com