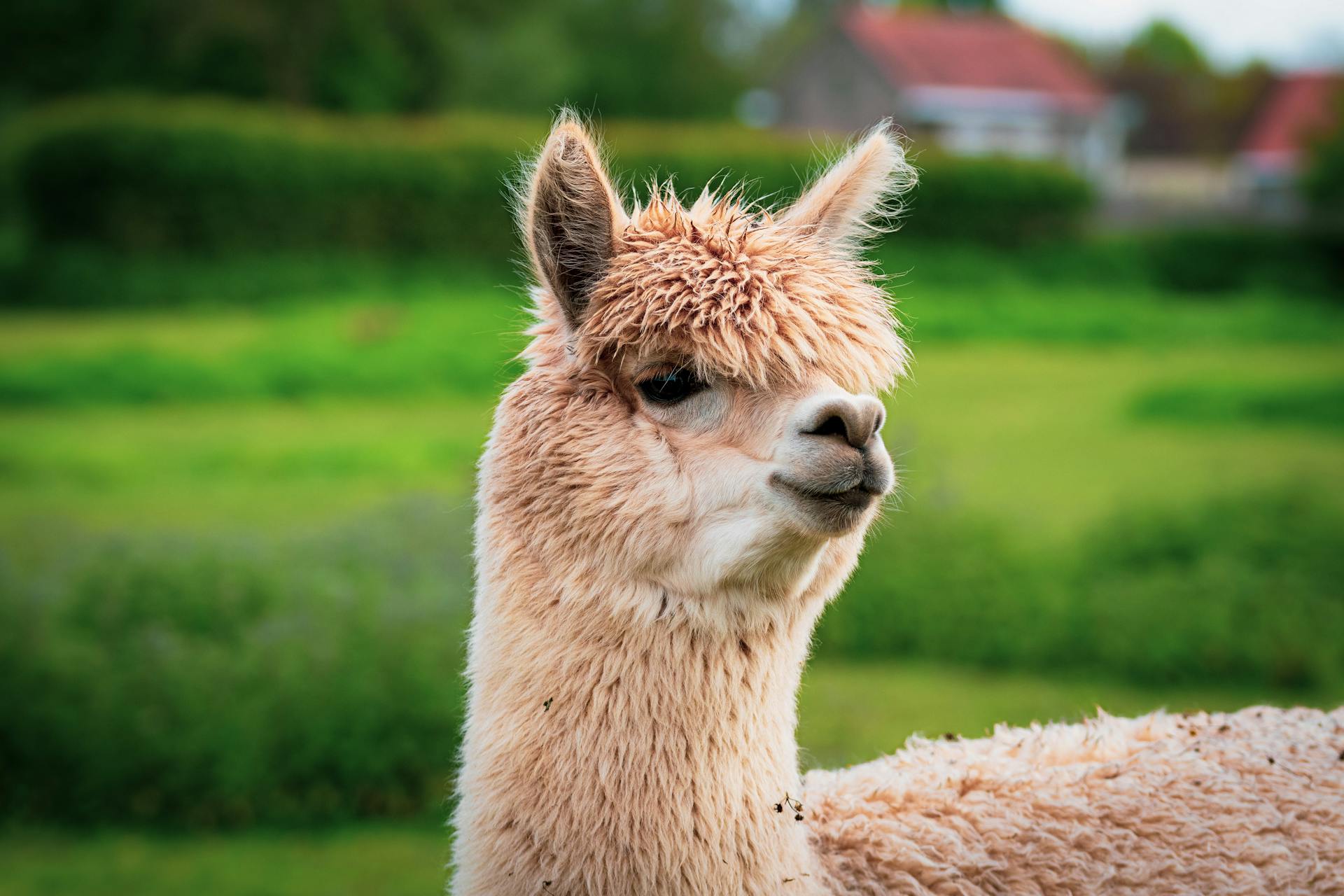
To get started with Llama 2 on Hugging Face, you'll first need to install the transformers library. This can be done using pip with the command `pip install transformers`.
Hugging Face provides a simple way to integrate Llama 2 into your existing codebase. You can do this by importing the `pipeline` class from the `transformers` library and specifying the `Llama2ForConditionalGeneration` model. This model is the foundation for the Llama 2 pipeline on Hugging Face.
Once you have the pipeline set up, you can use it to generate text by calling the `generate` method. This method takes in a prompt and returns a generated response. You can also customize the generation process by specifying parameters such as the maximum length of the output and the number of generations to perform.
The Llama 2 pipeline on Hugging Face supports various tasks, including text classification, question answering, and text generation. To perform these tasks, you'll need to specify the relevant pipeline class and task. For example, to perform text classification, you would use the `Llama2ForSequenceClassification` model.
You might enjoy: Llama 2 Fine Tuning Huggingface
Getting Started
To use Llama 2 with Hugging Face, you need to raise a request on the model page, making sure you're using the same email IDs in both places.
The first step is to download the Llama 2 model, which can be done in several ways. One way is to use the from_pretrained() and save_pretrained() functions from the Hugging Face library.
Unlike the previous method, using snapshot_download() functions will download the entire content of the model's repository. This can be filtered using the allow_patterns and ignore_patterns parameters.
Here are the three ways to download the model from Hugging Face:
- Use from_pretrained() and save_pretrained() HF functions
- Use snapshot_download() HF functions
If you choose to use the snapshot_download() function, you'll need to specify the files you want to download using the allow_patterns and ignore_patterns parameters. This will help you filter the files extensions.
Model Overview
The Llama 2 model is a collection of foundation language models ranging from 7B to 70B parameters. It's a significant improvement over the original Llama model, with some architectural tweaks and pre-training on 2 trillion tokens.
Here's an interesting read: Fine Tune Llama Huggingface
The Llama 2 model is developed by a large team of researchers from Meta AI, including Hugo Touvron, Louis Martin, and Kevin Stone. They've also released some follow-up works, including Llama2, which is an improved version of Llama with some architectural tweaks.
Llama 2 is optimized for dialogue use cases and outperforms open-source chat models on most benchmarks. It's also been fine-tuned for safety improvements, making it a suitable substitute for closed-source models.
Here's a breakdown of the Llama 2 model's architecture:
The Llama 2 model uses the FlashAttention-2 and other optimizations to improve the speed and efficiency of inference and training. It's also been extended to handle a longer context, making it suitable for applications like multi-document QA and long text summarization.
Intriguing read: Claude 2 Ai
Usage and Tips
To download the weights for the LLaMA models, you'll need to fill out a form. After that, you can use the conversion script to convert them to the Hugging Face Transformers format.
The script requires enough CPU RAM to host the whole model in float16 precision, which can be a significant amount - for the 65B model, it's 130GB of RAM needed. You'll also need to save the vocabulary and special tokens file to a directory.
Here are some things to keep in mind when using the LLaMA tokenizer:
- The tokenizer is a BPE model based on sentencepiece.
- One quirk of sentencepiece is that when decoding a sequence, if the first token is the start of the word, the tokenizer does not prepend the prefix space to the string.
For the Llama2 models, the original inference uses float16, but the checkpoints uploaded on the Hub use torch_dtype = 'float16'. You can obtain the weights for the Llama2 models by filling out a form, and the architecture is very similar to the first LLaMA with the addition of Grouped Query Attention (GQA).
Check this out: Ollama Huggingface
Usage Tips
To get started with using LLaMA models, you'll first need to obtain the weights. This can be done by filling out a form, which will give you access to the model checkpoints.
You'll need to download the weights and convert them to the Hugging Face Transformers format using a conversion script. The script can be called with a command like `python conversion_script.py --input weights_path --output output_path`.
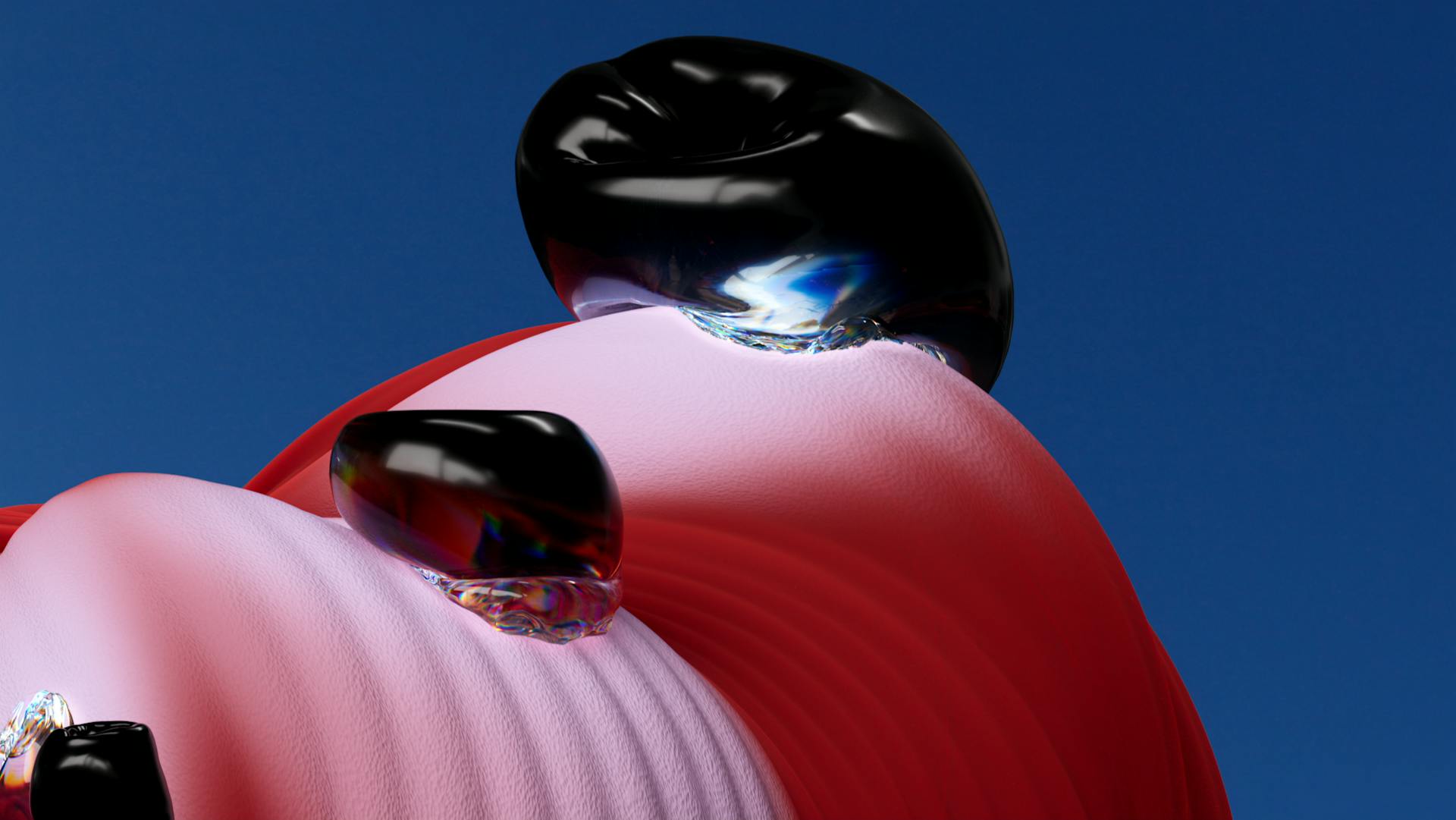
After conversion, you can load the model and tokenizer using the `AutoModelForCausalLM.from_pretrained()` method. This requires enough CPU RAM to host the whole model in float16 precision, which can be a significant amount of memory - for example, the 65B model requires 130GB of RAM.
The LLaMA tokenizer is a BPE model based on sentencepiece, which has a quirk: when decoding a sequence, it doesn't prepend a prefix space to the string if the first token is the start of a word.
To use the LLaMA models, you'll also need to save the vocabulary and special tokens file to a directory.
Here are some key things to keep in mind when working with LLaMA models:
Note that you should also be aware of the dtype of the online weights, which can affect how the model is initialized and used. For example, the Llama2 models were trained using bfloat16, but the original inference uses float16.
Training and Fine-Tuning
Training and Fine-Tuning is a crucial step in getting the most out of your LLaMA-2-7B-32K model. The model has been trained using a mixture of pre-training and instruction tuning data.
The training data consists of 25% RedPajama Book, 25% RedPajama ArXiv (including abstracts), 25% other data from RedPajama, and 25% from the UL2 Oscar Data. This data is used to train the model to fill in missing chunks or complete the text. To enhance the long-context ability, data shorter than 2K word is excluded.
The inclusion of UL2 Oscar Data is effective in compelling the model to read and utilize long-range context. This is a key feature for tasks that require the model to understand complex, long-form text.
To fine-tune the model for specific applications, you can use the OpenChatKit. This allows you to fine-tune your own 32K model over LLaMA-2-7B-32K. The example datasets are placed in togethercomputer/Long-Data-Collections.
Here are some examples of how to fine-tune the model for specific applications:
These commands will fine-tune the model for the specific task and dataset.
From Transformer to
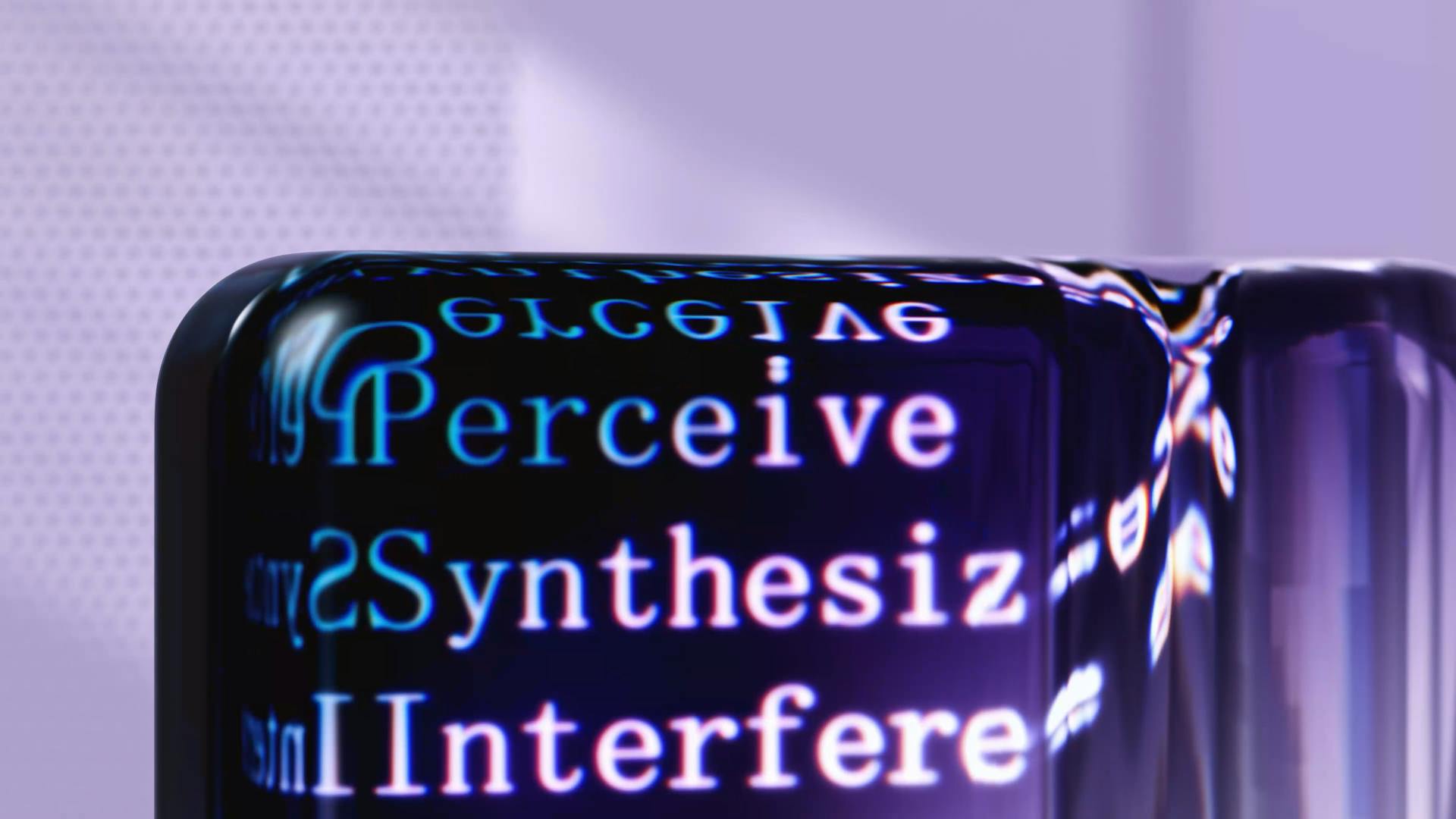
From Transformer to Llama, the evolution of language models has been rapid. The "Attention Is All You Need" paper introduced the pioneering "Transformer" architecture in 2017, changing the NLP field forever.
In the years that followed, the GPT model series emerged, showcasing the effectiveness of causal decoder architectures for pretraining, few-shot, and zero-shot learning. This led to the development of larger and more complex models.
The introduction of Llama 2 models by Meta in February 2023 marked a significant milestone, with key differences from the conventional transformer decoder architecture including a decoder-only model and the use of RMSNorm instead of LayerNorm.
Some notable features of Llama 2 include the use of SwiGLU activation function and RoPE as positional embeddings. Grouped Query Attention was also used in the 70B model, and the model was trained on a 4K context length.
Here's a comparison of Llama 2 and Llama 3:
The release of Llama 3 in April 2024 brought even more improvements, including a bigger tokenizer, the use of Grouped Query Attention in the smaller 8B model, and an increased context length of 8K for all models.
Class Transformers
Class Transformers are a crucial part of the LLaMA model, and they're used for a variety of tasks such as token classification. The LLaMA tokenizer is based on byte-level Byte-Pair-Encoding, which uses ByteFallback and no normalization.
The LLaMA tokenizer has several parameters, including the vocabulary file path, unknown token, and special tokens like the beginning of sequence token and the end of sequence token. These special tokens are used for tasks like sequence classification and can be added to the start or end of sequences. The tokenizer also has a legacy mode that's used for handling tokens that appear after special tokens.
Here are some key parameters of the LLaMA tokenizer:
- Vocabulary file path: The path to the vocabulary file that contains the necessary vocabulary for the tokenizer.
- Unknown token: The token that's used when a token is not found in the vocabulary.
- Beginning of sequence token: The token that's used to indicate the start of a sequence.
- End of sequence token: The token that's used to indicate the end of a sequence.
- Legacy mode: A mode that's used to handle tokens that appear after special tokens.
The LLaMA model itself is a transformer decoder that consists of multiple layers, and it's used for tasks like token classification. The model has a token classification head that's used for tasks like named entity recognition.
ClassTransformers
ClassTransformers is a library that provides a range of pre-trained transformer models for natural language processing tasks. These models are based on the transformer architecture, which is particularly well-suited for tasks like language translation, text summarization, and question answering.
For another approach, see: How to Use Huggingface Model in Python
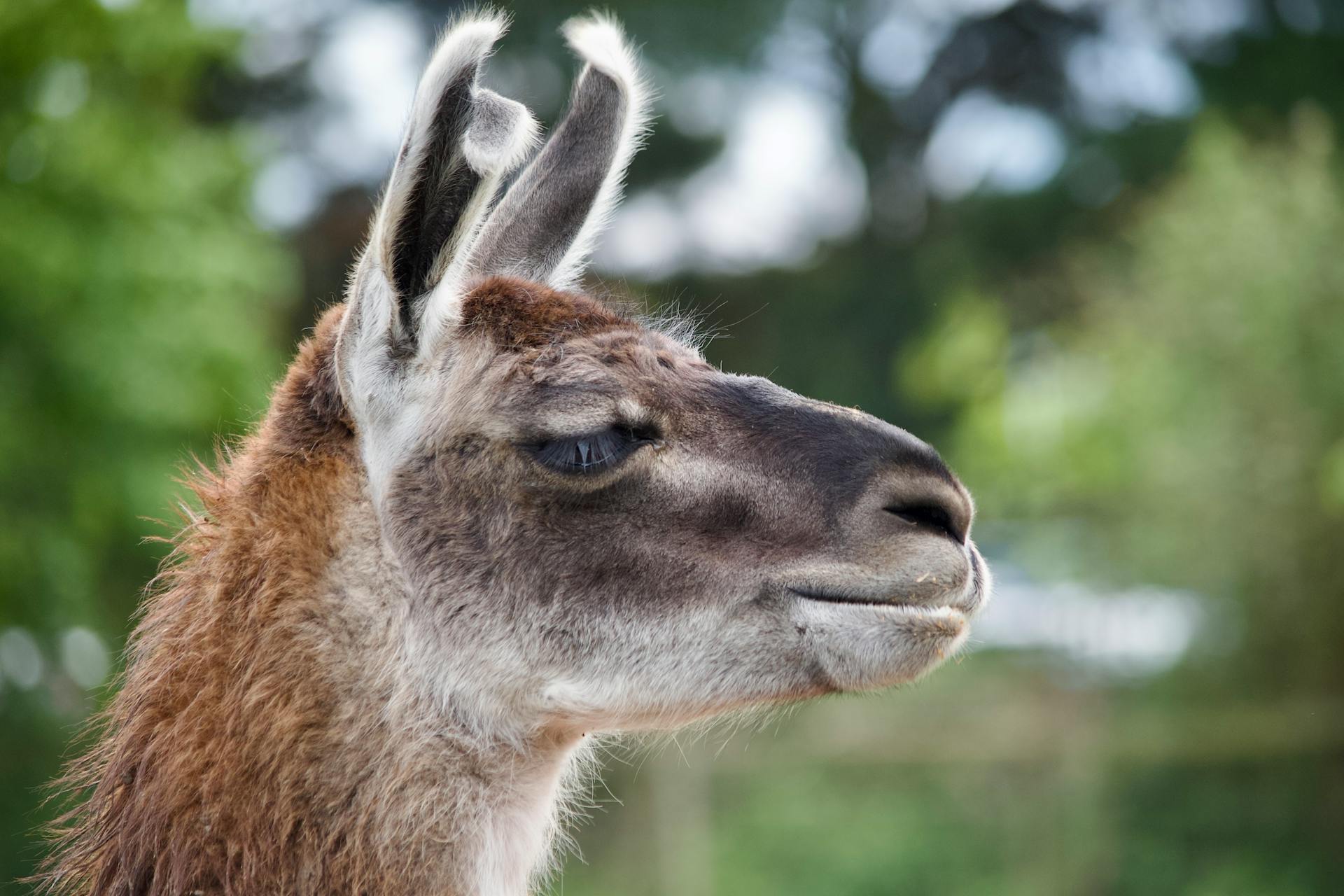
The ClassTransformers library includes models like LlamaTokenizer, LlamaTokenizerFast, LlamaModel, and LlamaForTokenClassification, each with its own unique features and capabilities. For example, LlamaTokenizer can be used to tokenize text, while LlamaModel can be used to perform tasks like language translation and text summarization.
One of the key features of ClassTransformers is its ability to handle a range of languages and tasks. For example, LlamaTokenizer can handle languages like English, Spanish, French, and many others. Additionally, ClassTransformers provides a range of pre-trained models that can be fine-tuned for specific tasks, making it a powerful tool for natural language processing.
Here are some of the key features of ClassTransformers:
- LlamaTokenizer: This tokenizer can be used to tokenize text in a range of languages, including English, Spanish, French, and many others.
- LlamaTokenizerFast: This tokenizer is similar to LlamaTokenizer, but it is optimized for speed and can handle large volumes of text.
- LlamaModel: This model can be used to perform tasks like language translation, text summarization, and question answering.
- LlamaForTokenClassification: This model can be used for tasks like named entity recognition and sentiment analysis.
In conclusion, ClassTransformers is a powerful library that provides a range of pre-trained transformer models for natural language processing tasks. Its ability to handle a range of languages and tasks makes it a valuable tool for researchers and practitioners in the field of natural language processing.
Transformer Layer Options
The Transformer Layer Options are a crucial part of the Transformer Engine's implementation, and understanding them can help you optimize your model's performance.
The TransformerLayer has several options that can be tweaked to suit your needs, including hidden_size, which determines the size of each input sample.
You can also adjust the number of attention heads, num_attention_heads, to control how many attention heads are used in the transformer layer.
Another important option is bias, which allows you to add additive biases to the submodule layers.
Additionally, you can play with layernorm_epsilon, which is a value added to the denominator of layer normalization for numerical stability, and is set to 1e-5 by default.
Hidden dropout and attention dropout probabilities can also be adjusted, with hidden_dropout and attention_dropout defaulting to 0.1.
There's also an option to fuse qkv params, which enables optimizations such as QKV fusion without concatenations/splits.
The normalization type can be changed to a different type, and the activation function used in the MLP block can also be customized.
Take a look at this: Huggingface Transformer Introductions
Lastly, you can control the format of the intermediate hidden states with attn_input_format, which can be either 'bshd' or 'sbhd'.
Here's a summary of the TransformerLayer options:
Comparison and Replacement
Replacing HF's LlamaDecoderLayer with TE's TransformerLayer is a potential improvement. This can be achieved by swapping the basic layers like Linear and LayerNorm with larger modules like MultiheadAttention and LayerNormMLP.
The Transformer Engine offers a full TransformerLayer that combines MultiheadAttention and LayerNormMLP layers, which could replace LlamaDecoderLayer and provide a speedup. This requires careful mapping of the weights since the name of the weights are different for those two layers.
Using Transformer Engine's TransformerLayer in FP8 precision can improve performance. This is seen in the HF Llama model implementation where most of the LlamaDecoderLayers have been swapped with Transformer Engine implementation.
Replacing HF's LlamaDecoderLayer with TE's TransformerLayer in BF16 precision can also provide a speedup. This is due to the use of Transformer Engine's TransformerLayer, which combines MultiheadAttention and LayerNormMLP layers.
Limitations and Dependencies
To run Llama 2 with Hugging Face, you need to install the necessary dependencies. This includes the transformers library, which provides APIs for pretrained Transformer models, and torch, a GPU-Ready Tensor library.
For CPU-support only, you can install both libraries in one command line using `pip install torch transformers`. Alternatively, you can install the transformers library with torch support using `pip install transformers[torch]`.
To download the library, you'll need a Hugging Face API token, which you can obtain by creating an account and copying your token. Once you have the token, you can use the `save_pretrained()` function to download a file to a specific local path, and then use the `from_pretrained()` function to download the model offline.
Here are the essential libraries you need to install:
- transformers: Hugging Face Transformers provides APIs to quickly download and use pretrained Transformer models.
- torch: PyTorch provides Tensors that can live either on the CPU or the GPU.
Dependencies
Dependencies are a crucial aspect of any project, and it's essential to understand what you need to get started. You'll need to install the transformers and torch libraries to run Llama 2 with Hugging Face locally.
These libraries are the backbone of Hugging Face Transformers, providing APIs to quickly download and use pre-trained Transformer models. PyTorch is a GPU-Ready Tensor library that's used in conjunction with transformers.
To install these libraries, you can use pip install torch transformers, which will install both packages. Alternatively, you can use pip install transformers[torch], which will install a variant of the transformers package that contains support for torch.
If you're using Git, you may need to authenticate your requests to the Hugging Face API using your token. This can be done by running a command in your terminal or notebook that prompts you to enter your Hugging Face token.
Here's a list of the dependencies required for this tutorial:
- te_llama.py
- utils.py
- media/
- pytorch
- transformer_engine
- accelerate
- transformers
- peft
- datasets
Limitations and Bias
We all know that AI models like LLaMA-2-7B-32K are not perfect and can make mistakes. As with all language models, LLaMA-2-7B-32K may generate incorrect or biased content.
It's essential to remember that these models can perpetuate existing biases, which can be problematic. This is a limitation that we need to be aware of when using the model.
Keep in mind that the accuracy and reliability of the content generated by LLaMA-2-7B-32K can vary. This is a crucial consideration when relying on the model for important decisions or information.
Curious to learn more? Check out: How to Use Models from Huggingface
Conclusion
Using the Transformer Engine's TransformerLayer module as a substitute for Hugging Face's LlamaDecoderLayer can provide a significant speedup.
This approach requires careful initialization of the model to ensure the model weights are correctly mapped to their counterparts in TE's TransformerLayer.
Even with BF16 precision, TransformerLayer offers a speedup over the baseline implementation, which is a notable improvement.
The speedup is even more pronounced when using FP8 precision, making it a worthwhile consideration for those looking to optimize their models.
Frequently Asked Questions
Can I use llama 2 for commercial use?
Yes, Llama 2 is free for commercial use. It's designed to be accessible for both research and business applications.
Sources
- https://medium.com/@fradin.antoine17/3-ways-to-set-up-llama-2-locally-on-cpu-part-3-hugging-face-cd06e0440a5b
- https://huggingface.co/docs/transformers/main/en/model_doc/llama
- https://huggingface.co/docs/transformers/en/model_doc/llama2
- https://huggingface.co/togethercomputer/LLaMA-2-7B-32K
- https://docs.nvidia.com/deeplearning/transformer-engine/user-guide/examples/te_llama/tutorial_accelerate_hf_llama_with_te.html
Featured Images: pexels.com