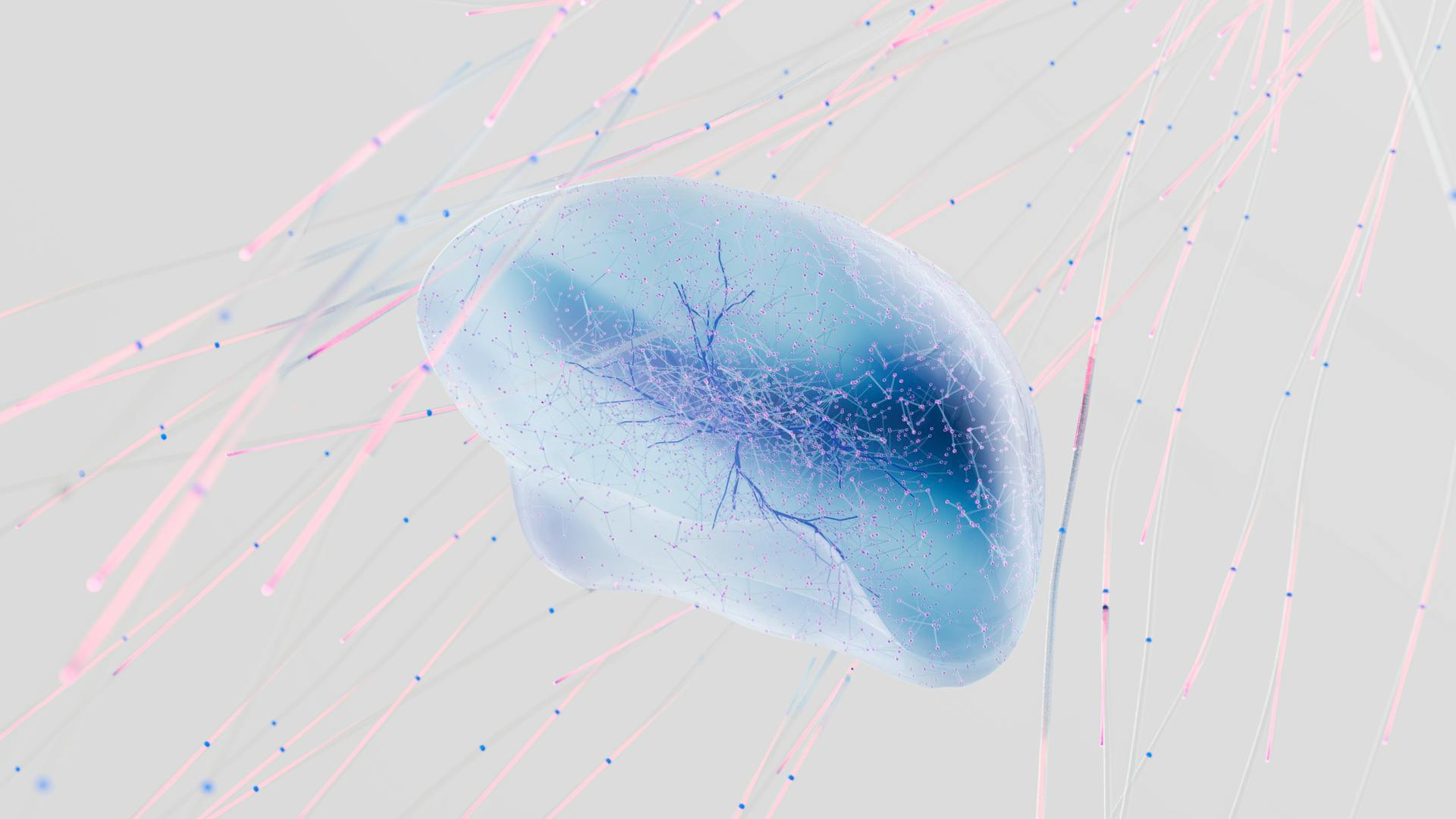
Transfer learning is a powerful technique that allows us to tap into the knowledge gained by others in machine learning models. This means we can use pre-trained models and fine-tune them for our specific problem.
By leveraging pre-trained models, we can significantly reduce the amount of data and computational resources required for training, making it a more efficient and cost-effective approach. This is particularly useful in real-world applications where data is limited or expensive to collect.
For instance, a model pre-trained on a large dataset of images can be fine-tuned for a specific task like image classification or object detection. This approach has been successfully applied in various domains, including computer vision and natural language processing.
The pre-trained models can be used as a starting point, and then we can add or modify layers to suit our specific needs. This flexibility is one of the key advantages of transfer learning, allowing us to adapt to different problem domains and tasks.
Check this out: Learning with Errors
What Is Transfer Learning
Transfer learning is a machine learning method that uses a pre-trained model as the basis for training a new one. This approach leverages the knowledge gained from solving a source task in the source domain and applies it to a target task or domain.
The traditional supervised learning paradigm breaks down when we don't have sufficient labeled data for the task or domain we care about. In such cases, transfer learning comes to the rescue by allowing us to reuse an existing model trained on a related task or domain.
Transfer learning can be applied when the domain of the training and test data is the same, but the task is different. For example, a model trained to recognize objects in images can be fine-tuned for detecting pedestrians on night-time images.
However, the model's performance may deteriorate or collapse when applied to a new domain, as it has inherited the bias of its training data. To overcome this challenge, transfer learning enables us to store the knowledge gained in solving the source task in the source domain and apply it to our problem of interest.
For your interest: Tunet and Domain Adaptation
In practice, we seek to transfer as much knowledge as we can from the source setting to our target task or domain. This knowledge can take on various forms depending on the data, such as how objects are composed to identify novel objects or general words people use to express their opinions.
Transfer learning can also be applied to natural language processing tasks, where a model trained for sentiment analysis in English can be used for building a model for the same task in German or Spanish.
The Typical Workflow
Instantiating a base model with pre-trained weights is the first step in a typical transfer learning workflow. This can be done using Keras.
To implement this workflow, you need to freeze all layers in the base model by setting trainable = False. This is crucial to prevent the base model from being modified during training.
There are two typical transfer learning workflows. The first one involves instantiating a base model, loading pre-trained weights, freezing the base model, creating a new model on top of the output of one or several layers from the base model, and training your new model on your new dataset.
On a similar theme: Neural Network Hidden Layer
The second workflow is faster and cheaper, but it doesn't allow you to dynamically modify the input data of your new model during training. This is a limitation when doing data augmentation, which is essential when your new dataset has too little data to train a full-scale model from scratch.
The Typical Workflow
To implement transfer learning in Keras, you need to instantiate a base model and load pre-trained weights into it. This is the first step in the typical transfer learning workflow.
You then freeze all layers in the base model by setting trainable to False. This prevents the base model's weights from being updated during training.
Next, you create a new model on top of the output of one or several layers from the base model. This is where you build your custom model that will be trained on your new dataset.
The typical transfer learning workflow involves training your new model on your new dataset. This is the final step in the process.
Readers also liked: Hidden Layers in Neural Networks Code Examples Tensorflow
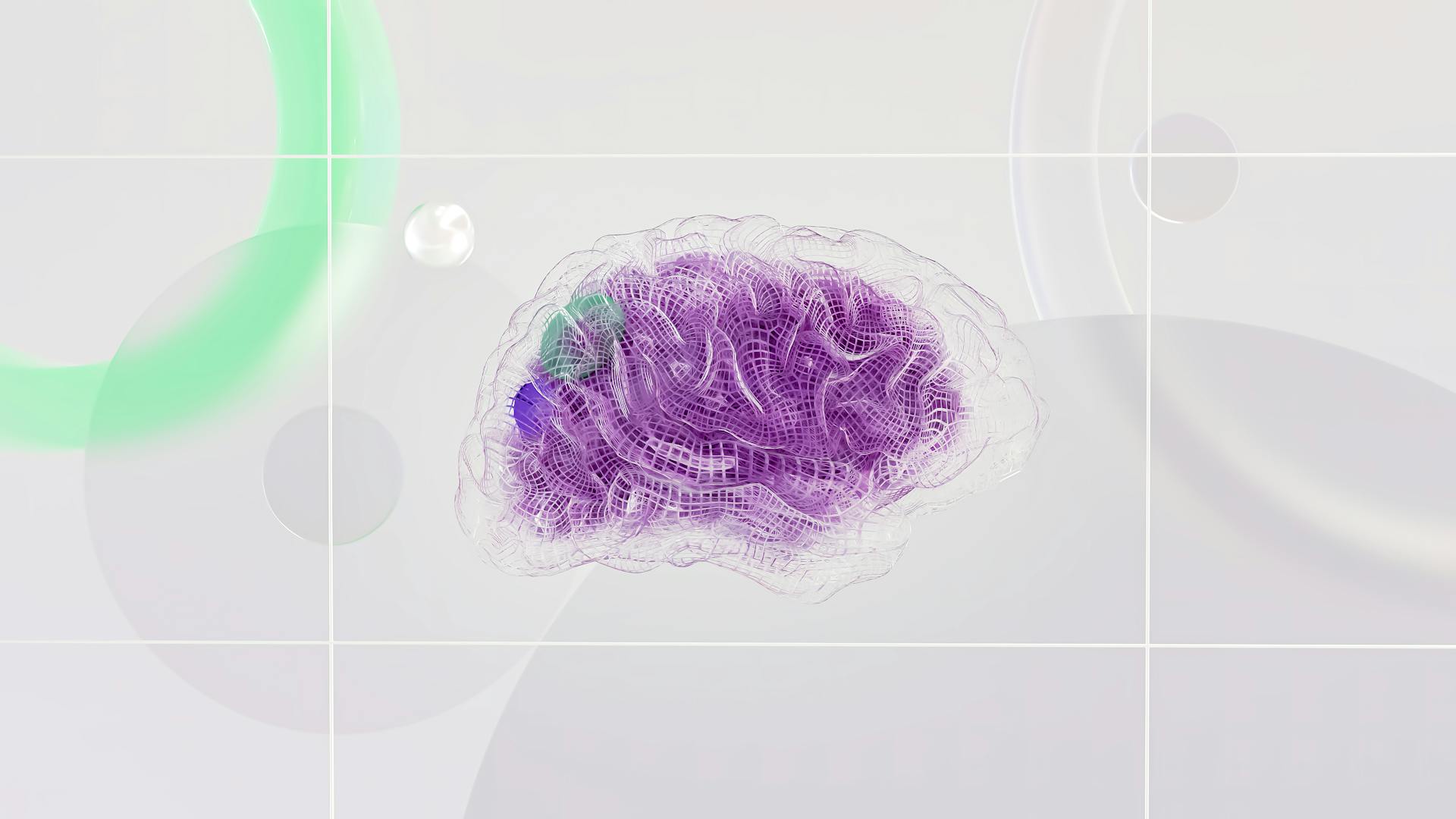
Here's a summary of the typical transfer learning workflow:
- Instantiate a base model and load pre-trained weights into it.
- Freeze all layers in the base model by setting trainable to False.
- Create a new model on top of the output of one or several layers from the base model.
- Train your new model on your new dataset.
Preparing Sets
Let's get started with preparing our datasets.
We need to load our datasets, which in this case are 12,500 images of dogs and cats, with 6,250 images per category.
To load the datasets, we use the following code snippet, which loads all the images in our original training data folder.
We can verify that we have 12,500 images for each category, which is confirmed by the output.
To build our smaller dataset, we need to select 3,000 images for training, 1,000 images for validation, and 1,000 images for our test dataset, with equal representation for the two animal categories.
We then write our datasets out to our disk in separate folders, so that we can easily access them in the future.
Now that our datasets are prepared, we need to load and prepare them for modeling.
We load our datasets using the following code snippet, which shows that we have 3,000 training images and 1,000 validation images.
You might like: Clustering Algorithms Unsupervised Learning
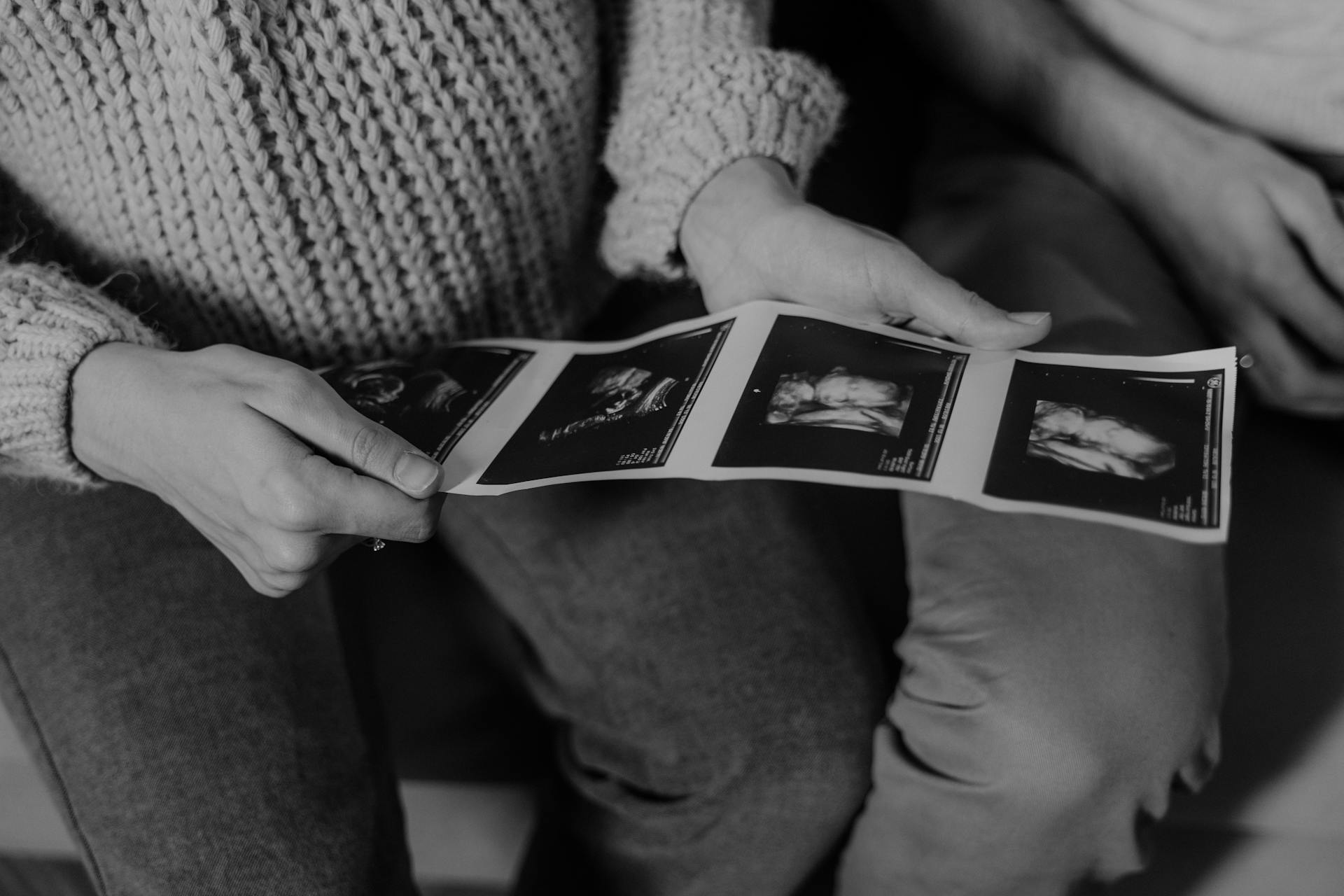
Each image is of size 150 x 150 and has three channels for red, green, and blue (RGB), giving each image the (150, 150, 3) dimensions.
We scale each image with pixel values between (0, 255) to values between (0, 1), as deep learning models work well with small input values.
This is confirmed by the output, which shows one of the sample images from our training dataset.
Optimization
Optimization is a crucial step in transfer learning. Fine-tuning is an optional last step that can give you incremental improvements, but it can also lead to quick overfitting.
To fine-tune a model, you need to unfreeze all or part of the base model and retrain the whole model end-to-end with a very low learning rate. This is only possible after the model with frozen layers has been trained to convergence.
It's essential to use a very low learning rate during fine-tuning, as you're training a much larger model than in the first round of training, and you're at risk of overfitting quickly. The learning rate should be so low that it only allows for incremental updates to the pre-trained weights.
A unique perspective: Fine Tune vs Incontext Learning
Here are some key things to keep in mind when fine-tuning:
- BatchNormalization layers contain 2 non-trainable weights that get updated during training. These are the variables tracking the mean and variance of the inputs.
- When you set bn_layer.trainable = False, the BatchNormalization layer will run in inference mode and not update its mean & variance statistics.
- When unfreezing a model with BatchNormalization layers, keep them in inference mode by passing training=False when calling the base model.
Optimization
Fine-tuning is an optional last step that can give you incremental improvements, but it also comes with the risk of quick overfitting.
The key to fine-tuning is to only do it after the model with frozen layers has been trained to convergence. If you mix randomly-initialized trainable layers with trainable layers that hold pre-trained features, the randomly-initialized layers will cause very large gradient updates during training, which will destroy your pre-trained features.
You should use a very low learning rate at this stage, because you are training a much larger model than in the first round of training, on a dataset that is typically very small. This is how to implement fine-tuning of the whole base model.
Calling compile() on a model is meant to "freeze" the behavior of that model, implying that the trainable attribute values at the time the model is compiled should be preserved throughout the lifetime of that model, until compile is called again.
On a similar theme: Model Fine Tune
BatchNormalization layers are a special case and contain 2 non-trainable weights that get updated during training, which track the mean and variance of the inputs. When you set bn_layer.trainable = False, the BatchNormalization layer will run in inference mode, and will not update its mean & variance statistics.
To fine-tune a model that contains BatchNormalization layers, you should keep the BatchNormalization layers in inference mode by passing training=False when calling the base model. This will prevent the updates applied to the non-trainable weights from destroying what the model has learned.
Here are a few things to keep in mind when working with BatchNormalization layers:
- BatchNormalization contains 2 non-trainable weights that get updated during training.
- When you set bn_layer.trainable = False, the BatchNormalization layer will run in inference mode.
- When you unfreeze a model that contains BatchNormalization layers, keep the BatchNormalization layers in inference mode by passing training=False.
Maximizing Efficiency
Using available data more effectively can greatly enhance model performance. By leveraging unsupervised or semi-supervised learning, we can extract information from unlabeled data, reducing the reliance on labeled samples.
Regularization can also help reduce overfitting by giving the model access to other features inherent in the data. This can be achieved by adding dropout after each hidden dense layer, like in the CNN Model with Regularization example, where 30% of the units in the dense layers are randomly masked.
A higher learning rate provided by transfer learning can also help models train faster and achieve target performance more quickly. This is evident in the Higher learning rate example, where transfer learning allows for a faster learning process.
However, it's worth noting that larger datasets may not have the expected effect on tasks that require them, as the initial weights become irrelevant with more iterations. This is a limitation of transfer learning, as seen in the Larger datasets example.
To maximize efficiency, we can also look for data in unexpected places, such as user-generated content, annotator disagreement, or user behavior like eye tracking or keystroke dynamics. These sources can provide valuable information for NLP tasks, as mentioned in the Using available data more effectively example.
Domain Adaptation
Domain adaptation is a common requirement in machine learning, especially in vision and natural language processing. It's like trying to teach a model to recognize bikes in a picture, but the training data is biased towards cars.
The marginal probabilities between the source and target domains are often different, meaning the data distribution of the source and target domains has shifted. This requires tweaks to transfer the learning, such as adapting to different text types.
For instance, a classifier trained on movie-review sentiment would see a different distribution if utilized to classify product reviews. Domain adaptation techniques are utilized in transfer learning in these scenarios.
To adapt to new domains, we can learn domain-invariant representations, which are similar to pre-trained CNN features. These representations are learned using stacked denoising autoencoders and have seen success in natural language processing and vision.
We can also make representations more similar by actively encouraging the representations of both domains to be more similar to each other. This can be achieved by applying certain pre-processing steps directly to the representations themselves.
Another way to ensure similarity between the representations of both domains is to add another objective to an existing model that encourages it to confuse the two domains. This is done by reversing the gradients that flow from the loss to the rest of the network.
Domain adaptation is crucial in scenarios where the data where labeled information is easily accessible is different from the data that we actually care about. It's not just about adapting to new domains, but also about adapting to individual users and minorities to ensure that everyone's voice is heard.
A different take: Unsupervised Domain Adaptation
Methods and Techniques
Transfer learning has a long history of research, and various techniques exist to tackle different scenarios. The advent of Deep Learning has led to new approaches, some of which we'll review.
Using pre-trained models can be an effective strategy. There are several such models available, so it's essential to do some research and choose the right one for your problem. The number of new trainable layers and reused layers will depend on the task at hand.
Pre-trained models can be used in two popular ways: as a feature extractor or by fine-tuning the model. This can be a game-changer for complex computer vision tasks, such as solving a cat versus dog classifier with fewer images.
A pre-trained model like the VGG-16, created by the Visual Geometry Group at the University of Oxford, is an excellent example of a feature extractor. It has learned a robust hierarchy of features from over a million images belonging to 1,000 different categories, making it a good starting point for new images.
Here's a brief overview of how pre-trained models can be used:
- Using a pre-trained model as a feature extractor
- Fine-tuning the pre-trained model
Getting the
Getting the data is a crucial step in transfer learning. You can use the utility keras.utils.image_dataset_from_directory to generate a labeled dataset from a set of images on disk filed into class-specific folders.
If you have a small dataset, transfer learning is most useful. To keep your dataset small, you can use 40% of the original training data for training, 10% for validation, and 10% for testing.
The first 9 images in the training dataset are all different sizes, which can make it challenging to work with them. However, using a pre-trained model can help you get around this issue.
Here are some popular ways to use pre-trained models:
- Using a pre-trained model as a feature extractor
- Fine-tuning the pre-trained model
Pre-trained models like VGG-16 have learned a robust hierarchy of features that can be useful for a wide range of tasks.
Augmentation
Augmentation is a technique used to artificially introduce sample diversity in a dataset, especially when it's small. This can be done by applying random yet realistic transformations to the training images, such as random horizontal flipping or small random rotations.
You might enjoy: Conditional Random Fields
Applying random transformations can help expose the model to different aspects of the training data, which slows down overfitting. For example, in Example 1, we see that random data augmentation is used to introduce sample diversity in the training images.
Random horizontal flipping and small random rotations are just a few examples of the many transformations that can be applied to the training images. These transformations can be used to simulate different angles, lighting conditions, and other variations that may occur in real-world images.
To optimize loading speed, batching the data and using prefetching are also recommended. This is demonstrated in Example 1, where we see that the data is batched and prefetching is used to optimize loading speed.
Here are some common data augmentation techniques that can be used:
- Random horizontal flipping
- Small random rotations
- Random zooming
- Random color jittering
These techniques can be used to artificially increase the size of the dataset, which can be especially helpful when working with small datasets. By applying these transformations, we can expose the model to a wider range of variations, which can lead to better performance.
Applications
Transfer learning has been successfully applied to various machine learning tasks, including text classification, digit recognition, and medical imaging. In fact, transfer learning has been used to improve the accuracy of neural networks and convolutional neural networks in both natural language processing and computer vision.
Transfer learning can be applied to different domains, such as text classification, where it has been used to improve the performance of models for sentiment analysis and document classification. This is achieved by transferring knowledge from pre-trained models to new tasks.
In addition to text classification, transfer learning has also been applied to audio and speech recognition tasks. For example, models developed for English speech recognition have been successfully used to improve speech recognition performance for other languages, such as German.
Transfer learning can also be applied to image recognition tasks, such as object recognition and identification. In fact, a model trained to recognize horses can be further applied to detect zebras.
Recommended read: Machine Learning Facial Recognition Security and Surveillance Systems
Some specific examples of transfer learning applications include:
- Textual data: Embeddings like Word2vec and FastText have been prepared using different training datasets and used in different tasks, such as sentiment analysis and document classification.
- Audio/Speech: Automatic Speech Recognition (ASR) models developed for English have been successfully used to improve speech recognition performance for other languages, such as German.
- Image recognition: A model trained to recognize horses can be further applied to detect zebras.
- Computer Vision: Deep learning has been successfully used for various computer vision tasks, such as object recognition and identification, using different CNN architectures.
These applications demonstrate the potential of transfer learning in improving the performance of machine learning models across different domains and tasks.
Benefits and Advantages
Transfer learning offers numerous benefits and advantages that make it an attractive approach in machine learning.
Transfer learning enables us to build more robust models that can perform a wide variety of tasks.
One of the key advantages of transfer learning is its ability to help solve complex real-world problems with several constraints.
It also tackles problems like having little or almost no labeled data availability.
Transfer learning makes it easier to transfer knowledge from one model to another based on domains and tasks.
This path can even lead us towards achieving Artificial General Intelligence some day in the future.
Here are some of the specific benefits of transfer learning:
- Helps solve complex real-world problems with several constraints
- Tackle problems like having little or almost no labeled data availability
- Ease of transferring knowledge from one model to another based on domains and tasks
- Provides a path towards achieving Artificial General Intelligence some day in the future!
Challenges and Limitations
Transfer learning has immense potential, but it's not without its challenges. Negative transfer is a major issue, where the transfer of knowledge from the source to the target task leads to a drop in performance.
There are various reasons for negative transfer, including when the source task is not sufficiently related to the target task, or if the transfer method can't leverage the relationship between the tasks well. This can happen even with brute-force transfer, as shown in the work of Rosenstien and co-authors, who found that such transfer can degrade performance in target tasks when the source and target are too dissimilar.
Bayesian approaches and clustering-based solutions are being researched to avoid negative transfers.
Lack of Time
Lack of time can be a major obstacle in machine learning. Teaching some machine learning models takes too long.
You might need to use a similar, pre-trained model when you don't have enough time to build a new one. This can save you a significant amount of time and effort.
Unrelated
Working with unrelated datasets can be a challenge. The features transfer poorly if the datasets are not similar.
This requires restricting the parameters that can be trained and removing some layers, which can lead to overfitting. It's very hard and time-consuming to determine how many layers can be removed without overfitting.
Challenges
Transfer learning has immense potential, but it's not without its challenges. Negative transfer is a major issue, where knowledge transfer from a source task to a target task actually causes a drop in performance.
There are various reasons for negative transfer, such as when the source task is not sufficiently related to the target task. This can lead to a degradation in performance, as seen in the work of Rosenstien and their co-authors.
To avoid negative transfer, researchers are exploring Bayesian approaches and clustering-based solutions to identify relatedness. These techniques aim to better leverage the relationship between the source and target tasks.
Quantifying the transfer in transfer learning is also crucial, as it affects the quality of the transfer and its viability. Researchers are using techniques such as Kolmogorov complexity to prove theoretical bounds and measure relatedness between tasks.
Here are some key challenges associated with transfer learning:
- Negative Transfer: occurs when transfer learning leads to a drop in performance
- Transfer Bounds: quantifying the transfer in transfer learning is essential to gauge its quality and viability
These challenges require careful investigation and exploration to overcome, and researchers are working on developing new techniques to address them.
Software
In transfer learning, pre-trained models can be fine-tuned for specific tasks using software tools like TensorFlow and PyTorch.
These frameworks provide a range of pre-trained models that can be adapted for new tasks, such as image classification and object detection.
The pre-trained models are often trained on large datasets and can be used as a starting point for new projects, saving time and computational resources.
Software tools also enable researchers to easily experiment with different models and architectures, allowing for faster development and testing of new ideas.
By leveraging pre-trained models and software tools, researchers can focus on the specific task at hand, rather than spending time building a model from scratch.
A different take: Ai Training Software
Case Studies and Examples
Transfer learning has been successfully applied in various domains, including image classification. ImageNet is a large-scale image classification dataset that has been used to train many pre-trained models.
One notable example is the VGG16 model, which achieved a top-5 error rate of 7.1% on the ImageNet validation set. This model was pre-trained on the ImageNet dataset and then fine-tuned for object detection tasks.
Expand your knowledge: Binary Classification
AlexNet, another pre-trained model, was trained on a dataset of over 1.2 million images and achieved a top-5 error rate of 15.3% on the ImageNet validation set. This model was used as a starting point for fine-tuning on a dataset of street scenes.
The pre-trained model can be fine-tuned for a specific task by updating the last layer to match the output shape of the target task. This process is known as transfer learning, where the pre-trained model is transferred to a new task.
Worth a look: Transfer Learning vs Fine Tuning
Conclusion and Future Work
Transfer learning is a game-changer in the machine learning industry, and its adoption is only going to grow. It's one of the key drivers for mainstream success in deep learning.
Transfer learning is a technique that enables us to build more intelligent systems, and with it, we can make the world a better place. The author of this article hopes to see more pre-trained models and innovative case studies that leverage transfer learning.
The author has already written a book on the topic, "Hands on Transfer Learning with Python", which is available on the Packt website and Amazon. This book provides a comprehensive coverage of transfer learning concepts and strategies.
Transfer learning is not just limited to image classification, it can also be applied to other areas like NLP, audio data, and generative deep learning. The author plans to cover these topics in future articles.
Here are some potential future articles on transfer learning:
- Transfer Learning for NLP
- Transfer Learning on Audio Data
- Transfer Learning for Generative Deep Learning
- More complex Computer Vision problems like Image Captioning
The author is excited to see more success stories around transfer learning and deep learning, which will enable us to build more intelligent systems and drive our personal goals.
Hands-On with Python
Deep learning can be simplified by transferring prior learning using the Python deep learning ecosystem.
The Python deep learning ecosystem is a powerful tool for machine learning tasks, thanks in part to Francois Chollet's amazing book 'Deep Learning with Python'.
Using Python for deep learning tasks can save a lot of time and effort, especially when working with complex neural networks.
Francois Chollet's book 'Deep Learning with Python' is a great resource for learning about deep learning with Python.
By leveraging the Python deep learning ecosystem, you can quickly and easily implement transfer learning in your machine learning projects.
Frequently Asked Questions
What is the difference between CNN and transfer learning?
Transfer learning is a technique that reuses knowledge from one task, whereas a Convolutional Neural Network (CNN) is a type of neural network architecture designed to process images. While CNNs can be used for transfer learning, not all transfer learning involves CNNs
Sources
- https://keras.io/guides/transfer_learning/
- https://www.ruder.io/transfer-learning/
- https://en.wikipedia.org/wiki/Transfer_learning
- https://serokell.io/blog/guide-to-transfer-learning
- https://towardsdatascience.com/a-comprehensive-hands-on-guide-to-transfer-learning-with-real-world-applications-in-deep-learning-212bf3b2f27a
Featured Images: pexels.com