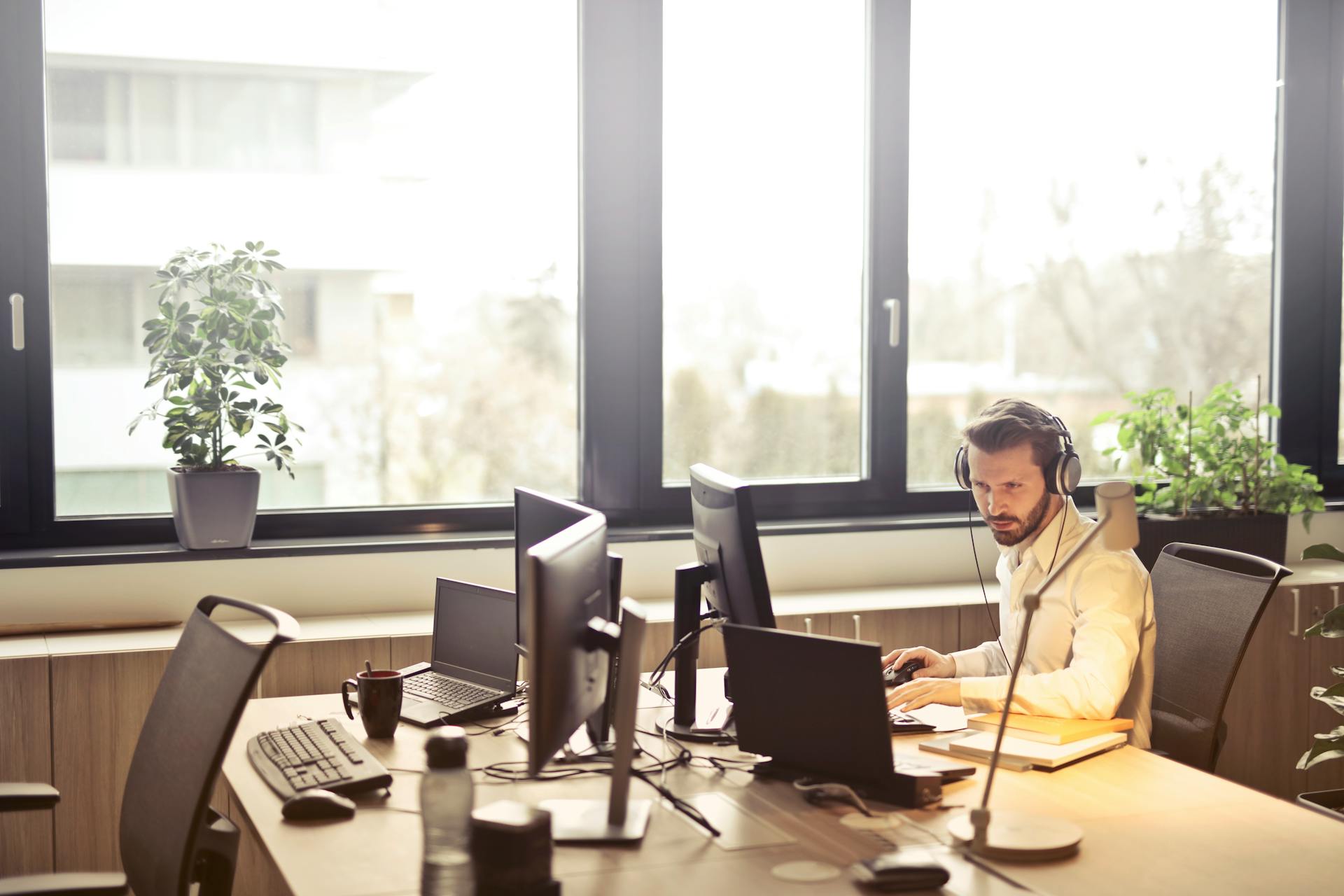
AP Computer Science is a challenging course, but with the right approach, you can succeed. The course covers a wide range of topics, including algorithms, data structures, object-oriented programming, and software engineering.
You'll learn how to write clear and concise code, and how to use design patterns to solve complex problems. The course also covers the basics of computer science, including data structures such as arrays, linked lists, and trees.
To succeed in AP Computer Science, you'll need to have a strong foundation in programming concepts and data structures. This includes understanding how to use recursion, iteration, and loops to solve problems.
The course also covers the AP Computer Science exam, which consists of two parts: multiple-choice questions and free-response questions. You'll need to be prepared to write clear and concise code to answer the free-response questions.
For more insights, see: Education Programming
Exam and Scheduling
The AP Computer Science exams have set dates, so be sure to mark your calendars for the regularly scheduled dates for the AP Computer Science A Exam and the AP Computer Science Principles Exam.
There are two core documents for the AP Computer Science courses: the AP Computer Science A Course and Exam Description and the AP Computer Science Principles Course and Exam Description. These documents provide a clear outline of the course content and describe the exam and AP Program in general.
See what others are reading: Ap Comp Sci Principles Exam Calculator
Exam Date
The exam date is a crucial piece of information for students preparing for their exams.
The AP Computer Science Principles Exam is scheduled on a regularly scheduled date, which is typically announced in advance.
This means that students can plan ahead and make sure they're available on the exam day.
For another approach, see: Ap Comp Sci Principles Practice Exam
Exam Description
The AP Computer Science A Exam has a regularly scheduled date, which is mentioned in the exam description.
The exam description is also the core document for the course, clearly laying out the course content and describing the exam and AP Program in general.
For students taking the AP Computer Science A Exam, it's essential to know that the exam description is the go-to resource for understanding the course and exam.
The AP Computer Science Principles Exam also has a regularly scheduled date, which is stated in the exam description.
The exam description for AP Computer Science Principles is the core document for the course, outlining the course content and providing an overview of the exam and AP Program.
In both AP Computer Science A and AP Computer Science Principles, the exam description is a crucial resource for students, teachers, and administrators.
Course Content
You'll learn the fundamentals of Java, a programming language, as well as other foundational concepts for coding. This will provide a solid base for your future studies in computer science.
In the course, you'll explore how real-world interactions can be expressed digitally by organizing behaviors and attributes into classes. This involves understanding the makeup of a class, including whether attributes are public or private.
Here are some key concepts you'll cover in the course:
- The makeup of a class, including whether attributes are public or private
- Setting an object’s attributes using constructors
- Using comments to describe the functionality of code
- Defining behaviors of an object using non-void, void, and static methods
- Where variables can be used in program code
- Breaking problems into smaller parts by creating methods to solve individual subproblems
- Intellectual property and ethical concerns in programming
Java Quick Reference
In this course, you'll learn the fundamentals of Java, a programming language, as well as other foundational concepts for coding. The curriculum covers a wide range of topics, but we'll focus on the Java Quick Reference, which is a crucial component of the course.
The Java Quick Reference is included in the Bluebook testing app and lists the accessible methods from the Java library that may be included on the exam. This version should be used in conjunction with released free-response questions from 2020 and later.
As you work through the course, you'll learn about primitive data types, including int, double, and Boolean, which are fundamental concepts in Java programming. You'll also learn how to evaluate arithmetic expressions in program code.
Here are some key concepts you'll cover in the Java Quick Reference:
- Primitive data types: int, double, and Boolean
- Evaluating arithmetic expressions in program code
- Using assignment operators to produce a value
- How variables and operators are sequenced and combined in an expression to create a result
These concepts will help you understand how to write effective Java code and prepare you for the exam. By the end of this course, you'll have a solid grasp of Java fundamentals and be well-prepared to tackle more advanced topics.
Algorithms and Programming
Algorithms and programming are the backbone of computer science, and understanding them is crucial for any aspiring programmer. You'll learn how to use algorithms and abstractions to create programs that solve problems or express your own creativity.
Developing algorithms is a key part of programming, and you'll explore how to create efficient and effective algorithms to tackle complex problems. You'll also learn about simulations, which can be used to model real-world systems and make predictions.
Additional reading: How to Learn to Code for Beginners
Algorithmic efficiency is critical in programming, and you'll learn how to optimize your algorithms to run faster and use less resources. This will help you to create programs that can handle large amounts of data and perform complex tasks.
Here's a breakdown of what you can expect to learn about algorithms and programming:
You'll also learn about the importance of data in programming, and how to extract information from it. This will help you to make sense of large datasets and create programs that can analyze and visualize data.
By mastering algorithms and programming, you'll be able to create programs that can solve complex problems and make a real impact in the world. So, let's dive in and explore the world of algorithms and programming!
Related reading: Programming Education
GridWorld Case Study (2008-2014)
The GridWorld Case Study was a computer program case study used with the AP Computer Science program from 2008 to 2014.
It was written in Java and served as an example of object-oriented programming (OOP).
For another approach, see: Ap Comp Sci Study Guide
The GridWorld framework was designed and implemented by Cay Horstmann, based on the Marine Biology Simulation Case Study, which was used from 2000-2007.
The narrative was produced by Chris Nevison and Barbara Cloud Wells, Colgate University.
Students were expected to be familiar with the classes and interfaces (and how they interact) before taking the exam.
The case study was divided into five sections, the last of which was only tested on the AB exam.
Roughly five multiple-choice questions in Section I were devoted to the GridWorld Case Study, and it was the topic of one free response question in Section II.
GridWorld has been discontinued and replaced with a set of labs for the 2014-2015 school year.
Systems and Networks
In this course, you'll delve into the world of computer systems and networks, and I'm excited to share some of the key concepts with you.
You'll explore how computer systems and networks work, and you'll learn about parallel and distributed computing, a technique that can speed up processes by dividing tasks across multiple computers.
Additional reading: Machine Learning in Computer Networks
The Internet is a fundamental part of modern computer systems and networks, and understanding how it works is crucial for anyone interested in technology.
Here are some key concepts you'll learn about in this section:
- Parallel computing: dividing tasks across multiple computers to speed up processes
- Distributed computing: using multiple computers to work together on a single task
These concepts will give you a solid understanding of how computer systems and networks work, and you'll be able to apply this knowledge in real-world scenarios.
Big Idea 5: Computing Impact
In the course, you'll explore the significant impact of computing on societies, economies, and cultures. The digital divide is a pressing issue that affects many people's access to technology and information.
Computing bias is another crucial topic that programmers need to consider. It refers to the unfair treatment of certain groups in the digital world, often due to biased algorithms.
Safe computing practices are essential to avoid online threats and protect personal data. This includes being cautious when clicking on links or downloading attachments from unknown sources.
The course will help you understand the legal and ethical responsibilities of programmers, including addressing computing bias and ensuring safe computing practices.
Frequently Asked Questions
Is computer science an AP hard?
AP Computer Science A is challenging, but manageable with proper support and practice. With the right resources, students can master the Java programming language and prepare for the AP CSA Exam.
What are the 5 big ideas of AP CSP?
The 5 Big Ideas of AP CSP are the foundational concepts that guide the study of computer science, including Creative Development, Data, Algorithms and Programming, Computer Systems and Networks, and the Impact of Computing. Understanding these Big Ideas is essential for developing a deep understanding of computer science principles and their applications.
Is AP Computer Science Java or Python?
AP Computer Science focuses on Java programming, but may also cover other foundational concepts and languages, including Python. Java is the primary language taught in this course.
Is AP Computer Science A hard?
AP Computer Science A is not hard, as the course provides students with support to learn and practice programming concepts. With the right resources, students can master the Java programming language and prepare for the AP CSA Exam.
What does AP Comp Sci do?
AP Comp Sci focuses on object-oriented programming in Java, emphasizing problem-solving with data structures and algorithms
Sources
- https://apstudents.collegeboard.org/courses/ap-computer-science-a
- https://www.aralia.com/helpful-information/difference-ap-computer-science-a-principles/
- https://apstudents.collegeboard.org/courses/ap-computer-science-principles
- https://en.wikipedia.org/wiki/AP_Computer_Science
- https://en.wikipedia.org/wiki/AP_Computer_Science_A
Featured Images: pexels.com