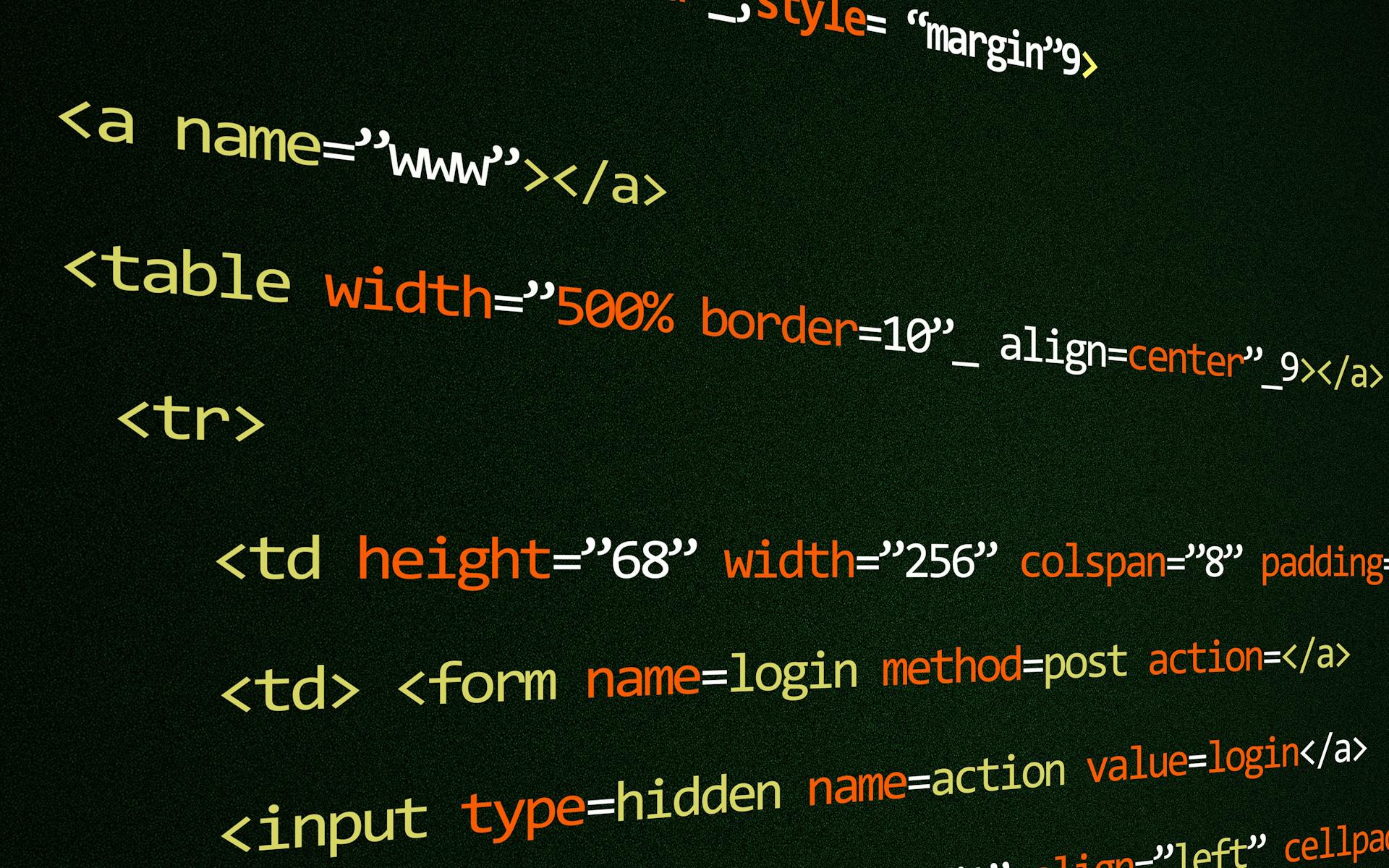
Code Llama can be fine-tuned with your own data and dataset to improve its performance on specific tasks. This process involves adapting the model's weights to better fit your data.
To fine-tune Code Llama, you need to prepare your dataset, which should be in a format that the model can understand. The dataset should be in a CSV or JSON file, and it should contain the input text and the corresponding output.
The size of your dataset will impact the fine-tuning process, with larger datasets requiring more computational resources and time to process. A dataset with 10,000 to 100,000 examples is a good starting point for fine-tuning Code Llama.
Suggestion: Llama 2 Huggingface
CodeLlama Configuration
To fine-tune CodeLlama, you'll need to define the base model and dataset. The base model for fine-tuning is NousResearch/Llama-2-7b-chat-hf.
For the dataset, you can use mlabonne/guanaco-llama2-1k. This dataset is a good choice for fine-tuning CodeLlama.
You can also fine-tune CodeLlama-70B using the Magicoder-OSS dataset with Predibase, a platform for fine-tuning and serving open-source LLMs. Predibase supports various hardware configurations, including T4s and A100s.
Here's an interesting read: Hyperparameter Tuning in Machine Learning
LLaMA 2 Overview
LLaMA 2 is an open source large language model introduced by Meta in 2023, part of the LLaMA family with varying capacities.
It's been trained on an extensive dataset of 2 trillion tokens, offering a context length of 4,096 tokens, double that of its predecessor LLaMA1. This is crucial for understanding and generating coherent and contextually relevant responses.
LLaMA 2 has models specifically fine-tuned for certain applications, such as LLaMA Chat, optimized for dialogue use cases, and Code LLaMA, focusing on code generation in multiple programming languages.
These fine-tuned models have been trained on massive datasets, like Code LLaMA, which has been trained on 500 billion tokens of code, showcasing its specialization in programming-related tasks.
The number of parameters in LLaMA 2 models determines their capacity to learn from data and generate responses. The greater the number of parameters, the more nuanced and complex the model's capabilities generally are.
For your interest: Learn How to Code Google's Go Golang Programming Language
Training and Testing
Training and testing are crucial steps in fine-tuning Code Llama, and it's essential to understand the process to get the best results. The fine-tuned model's capabilities can be tested with a simple prompt to generate text using the pipeline function.
The training process involves adjusting the model's parameters to perform better on a specific dataset or task. This can be done by continuing the training on a smaller, more specialized dataset, which allows the model to adapt its responses and predictions to the target task or domain. The PEFT library is used for fine-tuning LLaMA 2 with LoRA, and it's essential to configure it correctly to achieve good results.
The training process can be customized by adjusting the max_steps, which can help find the sweet spot for how many steps to perform. For example, if you start with 1000 steps and find that the model starts overfitting at around 500 steps, you would use the checkpoint-500 model repo as your final model. The PEFT library will only save the QLoRA adapters, so you need to load the base Llama 2 7B model from the Huggingface Hub.
Here are some key settings to keep in mind when training:
Dataset Preparation
The dataset we'll be using for fine-tuning is Magicoder-OSS-Instruct-75K, a large multi-language coding dataset generated by GPT-3.5 using OSS-Instruct.
This dataset contains computer programming implementations corresponding to text-based instructions, making it a high-quality coding dataset well-suited for fine-tuning.
Magicoder-OSS follows the "instruction tuning format" organized in a table, with the "problem" column containing the task, the "solution" column containing the code, and the "lang" column specifying the computer language in which the solution must be implemented.
Importing Magicoder-OSS-Instruct-75K will retrieve 75,197 rows, and we'll sample 3,000 random examples from it for fine-tuning.
The dataset has a reduced intrinsic bias compared to most other LLM-generated datasets, making it a great choice for fine-tuning.
Expand your knowledge: Huggingface Fine Tuning Llm
Supervised
Supervised fine-tuning is a process where a pre-trained language model is further trained on a smaller, task-specific dataset under human supervision. This method is used to adapt the general knowledge of the model to specific tasks or domains.
To implement supervised fine-tuning, you typically adjust the learning rate, batch size, and the number of training epochs. These parameters are crucial for ensuring that the model doesn't overfit on the specific dataset, which could reduce its performance on more general tasks.
Additional reading: Fine-tuning vs Transfer Learning
During supervised fine-tuning, the model learns from labeled examples, where each example contains an input (such as a question or a statement) and the corresponding output (like an answer or a continuation of the statement). This method contrasts with unsupervised learning, where the model learns from data without explicit labels.
Evaluation metrics like accuracy or F1 score are used to gauge the model's proficiency on the specific task post-fine-tuning. By adjusting these parameters and using labeled examples, you can help the model understand and generate more accurate and relevant responses in specific domains or tasks.
Here are some key parameters to consider when implementing supervised fine-tuning:
By fine-tuning a pre-trained language model on a smaller, task-specific dataset, you can adapt its general knowledge to specific tasks or domains. This can help improve the model's performance on specific tasks and make it more useful for real-world applications.
On a similar theme: Fine-tuning Huggingface Model with Custom Dataset
Step-by-Step Guide
To fine-tune Code Llama, you'll need to install the necessary libraries, including transformers, datasets, peft, and trl. This can be done using pip install transformers datasets peft trl.
You'll also need a Hugging Face account and the Hugging Face CLI installed. This can be done using pip3 install -U "huggingface_hub[cli]" and then logging in with the huggingface-cli login command.
Here's a step-by-step guide to fine-tuning Code Llama:
- Load the pre-trained LLaMA 2 model and tokenizer using the transformers library.
- Configure PEFT with LoRA settings, such as lora_alpha, lora_dropout, and r.
- Set up the training arguments, including output directory, evaluation strategy, learning rate, and batch size.
- Start the training process using the SFTTrainer from the peft library.
- Save the fine-tuned model for future use.
Note that you'll need a GPU and sufficient memory to run the training process. Additionally, the Guanaco dataset from Hugging Face, which has 534,530 entries, is a good example dataset to use for fine-tuning Code Llama.
Tutorial: Magicoder Dataset
The Magicoder Dataset is a crucial part of our project, and understanding it will help you get the most out of our guide. It's a collection of 10,000 images with corresponding labels.
Each image has a size of 224x224 pixels and is stored in JPEG format. The dataset is divided into two parts: training (80%) and testing (20%).
The images in the Magicoder Dataset cover a wide range of objects, including animals, vehicles, and household items. This variety will help your model learn to recognize and classify different objects.
The dataset is already preprocessed, so you don't need to worry about data cleaning or normalization.
A different take: Books to Help Learn Code in Java
Step by Step Guide
To fine-tune LLaMA 2, you'll need to install the necessary libraries, including transformers, datasets, and peft. You can do this using pip with the command `pip install transformers datasets peft`.
To get started with fine-tuning LLaMA 2, you'll need to load the pre-trained model and tokenizer using the Hugging Face transformers library. This can be done with the following code: `model_name = "meta-llama/Llama-2-7b-hf"` and `tokenizer = AutoTokenizer.from_pretrained(model_name, trust_remote_code=True)`.
The Guanaco dataset from HuggingFace is a great resource for fine-tuning LLaMA 2, with 534,530 entries specifically designed for English grammar analysis, natural language understanding, cross-lingual self-awareness, and explicit content recognition.
To configure PEFT with LoRA settings, you'll need to create a `LoraConfig` object with the following parameters: `lora_alpha=8`, `lora_dropout=0.5`, `r=8`, and `bias="none"`. This can be done with the following code: `lora_config = LoraConfig(lora_alpha=8, lora_dropout=0.5, r=8, bias="none", task_type="CAUSAL_LM")`.
Here is a step-by-step guide to fine-tuning LLaMA 2 with PEFT LoRA:
- Load and preprocess your dataset using the Hugging Face datasets library.
- Configure PEFT with LoRA settings using the `LoraConfig` object.
- Set up the training arguments using the `TrainingArguments` class.
- Start the training process using the `SFTTrainer` class.
- After training, save your fine-tuned model for future use using the `save_pretrained` method.
Model Setup
To fine-tune Code Llama, we need to set up the model and dataset. We'll define the base model as NousResearch/Llama-2-7b-chat-hf. This model will be used as the foundation for fine-tuning.
Next, we'll specify the dataset to use, which is mlabonne/guanaco-llama2-1k. This dataset will provide the necessary information for the model to learn from.
We'll also need to give a name to the new model, which will be created after fine-tuning.
Additional reading: Fine Tune Llama Huggingface
Importing Modules
Importing Modules is a crucial step in setting up your model. We'll be importing the necessary classes and functions from various libraries.
The core library for PyTorch is torch, which we'll import to get started. PyTorch is a machine learning framework that's widely used in the industry.
We'll also import load_dataset to load our training data, which is essential for training our model. This function is crucial for getting our data ready for use.
AutoModelForCausalLM and AutoTokenizer from the transformers library will be used for loading the model and tokenizer, respectively. These classes are specifically designed for causal language models.
Other important imports include BitsAndBytesConfig, TrainingArguments, pipeline, and logging, which provide configuration and utility functions that we'll need throughout our project.
Loading Tokenizer
In the model setup process, preparing the tokenizer is a crucial step. The tokenizer converts text into a format that the model can understand.
To process text from the training dataset, the tokenizer needs to be set up according to the model's requirements. Setting padding_side to "right" is essential to address specific issues with fp16 operations.
This specific setting is necessary to ensure that the model runs smoothly, especially when dealing with 16-bit floating-point operations.
Set Peft Parameters
To set PEFT parameters, we'll be using the LoRA method, which is a low-rank adaptation technique. This method allows us to update a small subset of the model's parameters, making the fine-tuning process more efficient.
The LoraConfig class is used to specify settings for PEFT, and it includes parameters like lora_alpha and lora_dropout, which define the architecture and behavior of the LoRA layers.
The task_type is set to "CAUSAL_LM" because LLaMA 2 is a causal language model, and this setting is specifically designed for language models like LLaMA 2.
Suggestion: What Is the Hardest Code Language to Learn
Model
The base model for fine-tuning is defined as NousResearch/Llama-2-7b-chat-hf. This model will be used as the foundation for the new model. We'll also need to specify the dataset, which is mlabonne/guanaco-llama2-1k, and give a name to the new model.
The model is fine-tuned using the SFTTrainer, which takes the model, dataset, PEFT configuration, tokenizer, and training parameters as inputs. This process allows the model to learn from the new dataset.
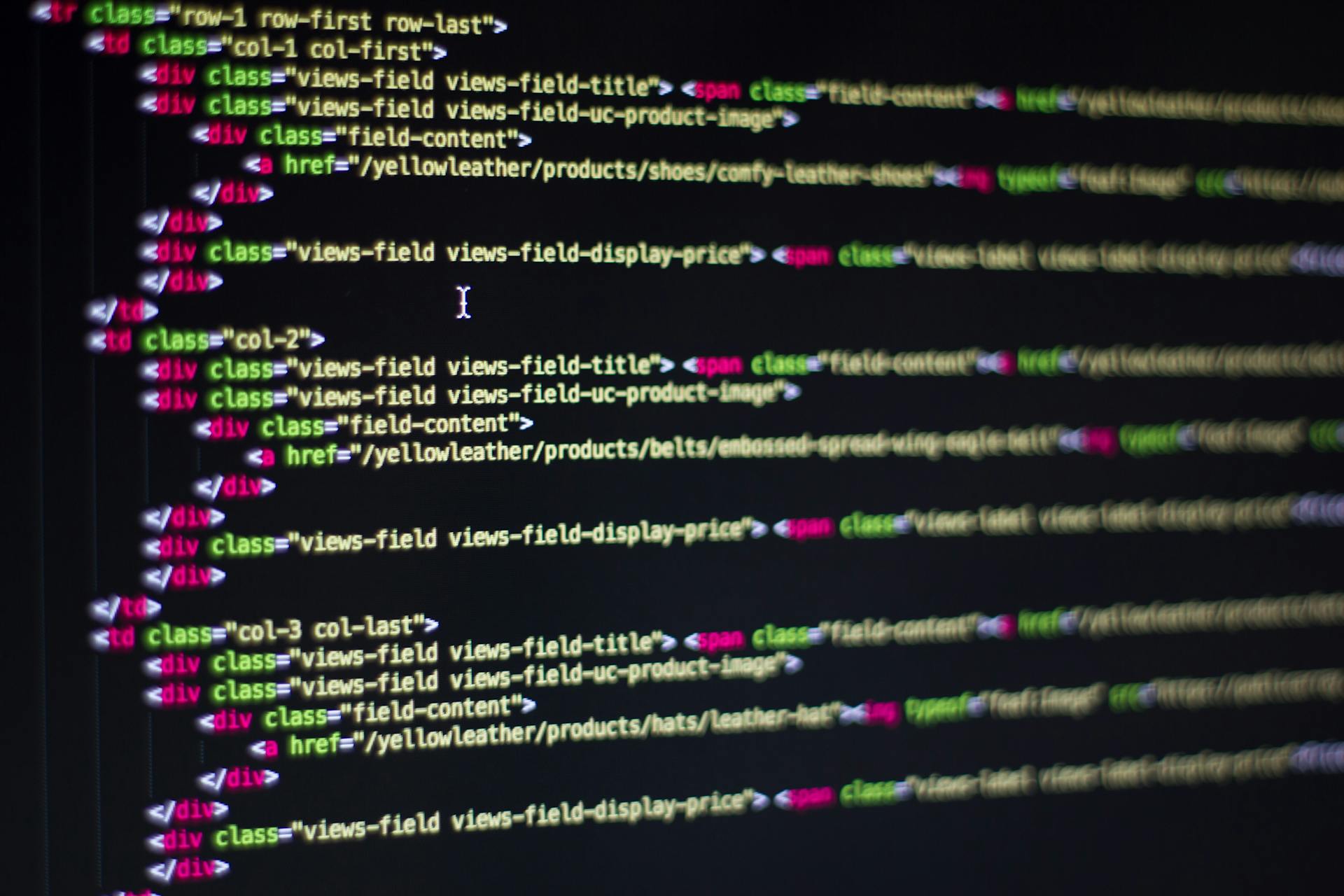
The fine-tuned model is then tested with a simple prompt to generate text. This is done using the pipeline function, which is a high-level utility for text generation.
The pre-trained Llama model is loaded using the LlamaForCausalLM class from the Hugging Face Transformers library. The load_in_8bit=True parameter is used to load the model using 8-bit quantization, reducing memory usage and improving inference speed.
The Llama 2 7B model is loaded using 4-bit quantization, a more efficient method than 8-bit quantization.
Check this out: Can I Generate Code Using Generative Ai Models
Sources
- https://predibase.com/blog/how-to-efficiently-fine-tune-codellama-70b-instruct-with-predibase
- https://www.run.ai/guides/generative-ai/llama-2-fine-tuning
- https://www.mlexpert.io/blog/alpaca-fine-tuning
- https://www.brev.dev/blog/fine-tuning-llama-2-your-own-data
- https://www.acorn.io/resources/learning-center/fine-tuning-llama-2
Featured Images: pexels.com